Key Takeaways
1. Resource-oriented APIs provide a standardized approach for designing intuitive and scalable web services
Resource-oriented APIs are really just a special type of RPC-style APIs where each RPC follows a clear and standardized pattern: <StandardMethod><Resource>().
Standardization simplifies API design. Resource-oriented APIs focus on representing data as resources with a standard set of methods (GET, POST, PUT, DELETE) applied to them. This approach provides several benefits:
- Consistency: Users can quickly learn and understand the API structure
- Scalability: New resources can be easily added without changing the overall API design
- Predictability: Standard methods behave similarly across different resources
By organizing APIs around resources and standard methods, developers can create more intuitive and user-friendly interfaces that are easier to learn, use, and maintain over time.
2. Effective naming conventions and resource layouts are crucial for creating clear and maintainable APIs
Good names, like good APIs, are simple, expressive, and predictable.
Naming matters in API design. Proper naming conventions and resource layouts significantly impact an API's usability and maintainability:
- Use clear, concise, and descriptive names for resources and methods
- Follow consistent naming patterns across the API
- Avoid ambiguous or overly generic names
Resource layout considerations:
- Organize resources hierarchically when appropriate
- Use meaningful relationships between resources
- Avoid deep nesting that can lead to complex URLs
Well-designed names and layouts make APIs more intuitive, reducing the learning curve for new users and improving overall developer experience.
3. Data types and field definitions form the foundation of robust API design
While code is often private and out of sight in most software projects, design decisions in an API are front and center, shown to all of the users of the service.
Careful consideration of data types is crucial. Proper selection and definition of data types and fields impact API usability, performance, and future extensibility:
- Choose appropriate data types for each field (e.g., string, number, boolean)
- Consider size limitations and potential growth of data
- Use enumerations sparingly, opting for string fields with validation when possible
Key considerations:
- Nullable vs. optional fields
- Default values and their implications
- Handling of complex data structures (e.g., nested objects, arrays)
By defining clear and consistent data types, APIs become more predictable and easier to integrate with various client applications.
4. Standard methods offer consistency and predictability across API operations
Standard methods should (and likely will) get an API 90% of the way there. And for the rest of the scenarios, you have custom methods to explore in the next chapter.
Leverage standard methods for common operations. Standard methods (GET, POST, PUT, DELETE) provide a consistent interface for interacting with resources:
- GET: Retrieve resource information
- POST: Create new resources
- PUT: Update existing resources
- DELETE: Remove resources
Benefits of standard methods:
- Predictable behavior across different resources
- Simplified client integration
- Adherence to RESTful principles
By relying on standard methods for common operations, APIs become more intuitive and easier to use, reducing the learning curve for developers integrating with the service.
5. Custom methods and long-running operations extend API functionality beyond standard CRUD operations
Custom methods are not a mechanism for parameterization of standard methods.
Extend API capabilities with custom methods. When standard methods are insufficient, custom methods can provide additional functionality:
- Use for complex operations that don't fit the CRUD model
- Name custom methods clearly to indicate their purpose
- Avoid overusing custom methods, as they can increase API complexity
Long-running operations:
- Handle time-consuming tasks asynchronously
- Provide status updates and progress information
- Allow cancellation and resumption of operations
By carefully implementing custom methods and long-running operations, APIs can offer advanced functionality while maintaining overall simplicity and consistency.
6. Resource relationships and polymorphism enable complex data modeling in APIs
Polymorphism in APIs allows resources to take on varying types to avoid duplicating shared functionality.
Model complex relationships effectively. APIs often need to represent complex data relationships:
- One-to-many: Use nested resources or separate endpoints
- Many-to-many: Implement association resources or custom methods
- Polymorphism: Allow resources to take on multiple forms
Relationship modeling strategies:
- Cross-references: Link resources using identifiers
- Nested resources: Represent hierarchical relationships
- Polymorphic resources: Share common interfaces across different resource types
Effective modeling of resource relationships allows APIs to represent complex data structures while maintaining clarity and ease of use.
7. Batch operations and pagination facilitate efficient handling of large datasets
In short, pagination has to do with being able to consume data when the number of resources or the size of a single resource is simply too large for a single API response.
Optimize for large datasets. APIs often need to handle large amounts of data efficiently:
Batch operations:
- Allow multiple resources to be created, updated, or deleted in a single request
- Improve performance by reducing the number of API calls
- Maintain atomicity to ensure all-or-nothing execution
Pagination:
- Break large result sets into manageable chunks
- Use cursor-based pagination for better performance and consistency
- Provide clear indicators for the next page of results
By implementing batch operations and pagination, APIs can handle large datasets more efficiently, improving performance and user experience.
8. Security and versioning strategies ensure API reliability and backward compatibility
When error codes are not provided (or have overlap, or are intended for humans rather than computers), we end up in the scary situation where API users may begin to rely on the message contents to figure out what's wrong.
Prioritize security and compatibility. Implementing robust security measures and versioning strategies is crucial for API longevity:
Security considerations:
- Use proper authentication and authorization mechanisms
- Implement rate limiting to prevent abuse
- Provide clear error messages with specific error codes
Versioning strategies:
- Use semantic versioning to communicate changes
- Maintain backward compatibility when possible
- Clearly document breaking changes and migration paths
By focusing on security and versioning, APIs can maintain reliability and trust while evolving to meet changing requirements over time.
Last updated:
FAQ
What is API Design Patterns by J.J. Geewax about?
- Comprehensive API design guide: The book presents a structured collection of design patterns and principles for building web APIs, with a focus on resource-oriented APIs.
- Practical and accessible: It uses TypeScript-style API definitions and real-world examples, such as a Twitter-like API, to illustrate concepts for developers familiar with HTTP and serialization.
- Organized for learning: The content is divided into six parts, covering API basics, design patterns, resource relationships, collective operations, and safety/security concerns.
Why should I read API Design Patterns by J.J. Geewax?
- Improve API usability: The book helps designers create APIs that are operational, expressive, simple, and predictable, leading to better user experiences and higher adoption.
- Avoid common pitfalls: It distills years of best practices, helping readers sidestep mistakes like inconsistent naming, poor resource layout, and problematic versioning.
- Consistency and scalability: Following the patterns ensures APIs are easier to maintain, evolve, and integrate, benefiting both providers and consumers.
What are the key takeaways from API Design Patterns by J.J. Geewax?
- Patterns and anti-patterns: The book highlights both effective design patterns and common anti-patterns, such as offset-based pagination and in-line data, to guide better API decisions.
- Advanced concepts covered: It addresses complex topics like request deduplication, resource revisions, authentication, and versioning, equipping readers for real-world challenges.
- Balance of theory and practice: The book combines foundational principles with practical advice, code examples, and exercises to reinforce learning.
What makes a "good" API according to API Design Patterns by J.J. Geewax?
- Operational and reliable: APIs should reliably do what users expect, meeting non-functional requirements like latency and accuracy.
- Expressive and simple: Users must be able to clearly express their intent without awkward workarounds, and the API should be as simple as possible.
- Predictable and consistent: Consistent naming, behavior, and patterns reduce surprises, making APIs easier to learn and use effectively.
How does API Design Patterns by J.J. Geewax define and use API design patterns?
- Reusable blueprints: API design patterns are reusable solutions to common interface design problems, focusing on structure rather than implementation.
- Addressing API rigidity: Since APIs are hard to change once public, patterns help get the design right from the start.
- Promoting uniformity: Patterns encourage consistency across APIs, reducing fragmentation and making APIs easier to learn within organizations.
What naming conventions and advice does API Design Patterns by J.J. Geewax provide for APIs?
- Names are critical: API names are public and long-lived, so choosing expressive, simple, and predictable names is essential to avoid confusion and costly changes.
- Avoid "API smells": Prepositions in names often signal deeper design issues, and units should be included in names for clarity (e.g., sizeBytes vs. sizeMegapixels).
- Consistency across the API: Good naming practices ensure uniformity and make APIs easier to use and maintain.
How does API Design Patterns by J.J. Geewax recommend structuring resources and their relationships?
- Thoughtful resource layout: Carefully choose resources and define only necessary relationships (references, hierarchies, many-to-many, self-references) to avoid complexity.
- Avoid anti-patterns: Don’t create resources for everything, avoid deep hierarchies, and refrain from in-lining all data to prevent confusion and inefficiency.
- Modeling relationships: Use association resources for many-to-many relationships and string ID fields for references, ensuring flexibility and data integrity.
What are the standard API methods and their characteristics in API Design Patterns by J.J. Geewax?
- Standard methods defined: The book emphasizes Get, List, Create, Update (PATCH), Delete, and Replace (PUT) as the core methods.
- Consistency is key: Each method should behave predictably and consistently across all resources to maximize learnability.
- Idempotency and side effects: Most methods are idempotent except Delete, which should fail if the resource doesn’t exist; side effects should be minimized for predictability.
How does API Design Patterns by J.J. Geewax handle partial updates and retrievals using field masks?
- Granular field selection: Field masks let clients specify which fields to retrieve or update, reducing unnecessary data transfer and supporting fine-grained updates.
- Transport and syntax: Field masks are sent as repeated query parameters and can address nested fields, but not array items by index.
- Defaults and limitations: By default, all fields are returned unless specified, and updates infer the mask from provided data.
When and how should custom methods be used in APIs according to API Design Patterns by J.J. Geewax?
- For nonstandard actions: Custom methods handle operations with side effects (e.g., sending emails, launching rockets) that don’t fit standard CRUD methods.
- Separation of concerns: They keep complex operations distinct from simple data manipulation, maintaining the purity of standard methods.
- Consistent implementation: Custom methods use POST and a colon in the URL (e.g., /resource:action) and should be applied consistently across the API.
How does API Design Patterns by J.J. Geewax address advanced topics like pagination, filtering, and versioning?
- Cursor-based pagination: The book recommends using page tokens as cursors instead of numeric offsets to avoid duplicate or missing results.
- Flexible filtering: Advocates for unstructured string filters (like SQL WHERE clauses) for usability and future flexibility, evaluating one resource at a time.
- Versioning strategies: Discusses perpetual stability, agile instability, and semantic versioning, each with trade-offs in stability and user satisfaction.
What security and reliability patterns does API Design Patterns by J.J. Geewax recommend (e.g., request deduplication, validation, authentication)?
- Request deduplication: Use client-generated request IDs to identify and deduplicate non-idempotent requests, caching responses for a limited time.
- Validation with
validateOnly
: ThevalidateOnly
flag allows clients to preview request outcomes without executing them, ensuring safety and correctness. - Authentication via digital signatures: Users register public keys with the API server and sign requests with private keys, ensuring integrity, authenticity, and nonrepudiation.
Review Summary
API Design Patterns receives mixed reviews, with an overall rating of 3.89 out of 5. Readers appreciate its comprehensive coverage of API design topics, practical insights, and structured approach. The book is praised for discussing complex patterns and providing valuable guidance for backend engineers and API designers. However, some criticize its verbosity, repetitiveness, and occasional lack of context. Despite these drawbacks, many readers consider it a must-read for those involved in API development, offering a good balance of theory and practical examples.
Similar Books
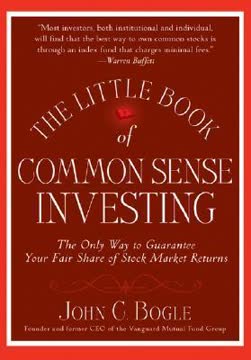
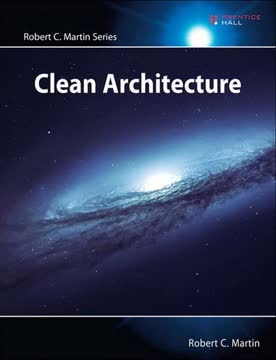
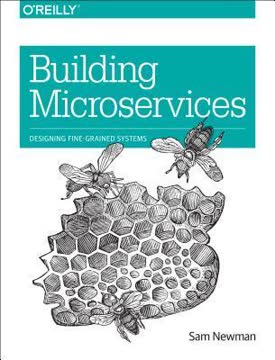
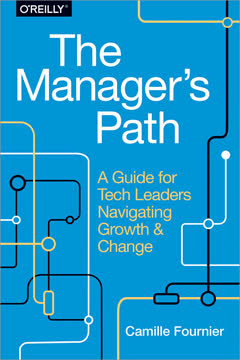
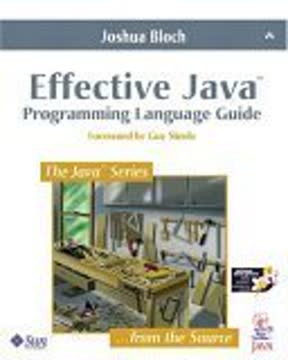
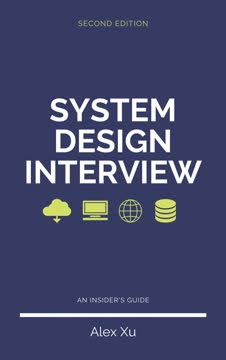
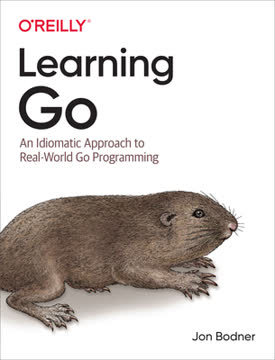
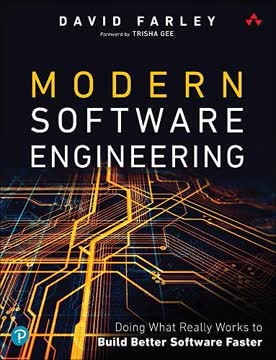
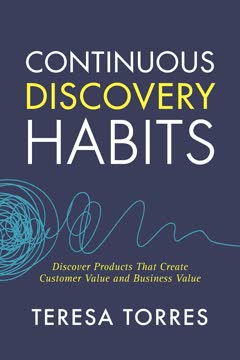
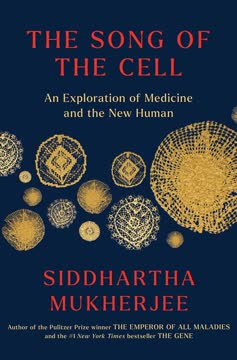
Download PDF
Download EPUB
.epub
digital book format is ideal for reading ebooks on phones, tablets, and e-readers.