Key Takeaways
1. Domain modeling is the foundation of clean, maintainable software architecture
A domain model is not a data model—we're trying to capture the way the business works: workflow, rules around state changes, messages exchanged; concerns about how the system reacts to external events and user input.
Domain-Driven Design (DDD) emphasizes creating a rich domain model that reflects the business logic and processes. This approach separates core business rules from infrastructure concerns, making the system more flexible and easier to maintain. Key concepts include:
- Entities: Objects with a distinct identity that persists over time
- Value Objects: Immutable objects defined by their attributes
- Aggregates: Clusters of related objects treated as a unit for data changes
- Domain Events: Represent significant occurrences within the domain
By focusing on the domain model, developers can create a shared language with stakeholders, improving communication and ensuring the software accurately represents the business requirements.
2. Repository and Unit of Work patterns decouple the domain from infrastructure
The Repository pattern is an abstraction over persistent storage, allowing us to decouple our model layer from the data layer.
Repository pattern provides a collection-like interface for accessing domain objects, hiding the details of data access. Unit of Work pattern maintains a list of objects affected by a business transaction and coordinates the writing out of changes. Together, they offer several benefits:
- Separation of concerns: Domain logic remains pure, free from infrastructure details
- Testability: Easier to mock or fake for unit testing
- Flexibility: Ability to switch between different storage mechanisms without affecting the domain
These patterns create a clear boundary between the domain and data access layers, allowing each to evolve independently and promoting a more modular architecture.
3. Service Layer pattern orchestrates use cases and defines system boundaries
The Service Layer pattern is an abstraction over domain logic that defines the application's use cases and what they require from the domain model.
Service Layer acts as a facade for the domain model, encapsulating application-specific logic and orchestrating the execution of use cases. It provides several advantages:
- Clear API: Defines the operations the application can perform
- Separation of concerns: Keeps domain logic separate from application logic
- Testability: Enables high-level unit tests without need for integration tests
By implementing a Service Layer, developers can create a clear boundary between the application's external interfaces (e.g., API, CLI) and its internal domain logic, making the system easier to understand and maintain.
4. Event-driven architecture enables loose coupling and scalability
Events can help us to keep things tidy by separating primary use cases from secondary ones. We also use events for communicating between aggregates so that we don't need to run long-running transactions that lock against multiple tables.
Event-driven architecture uses events to trigger and communicate between decoupled services. This approach offers several benefits:
- Loose coupling: Services can evolve independently
- Scalability: Easier to scale individual components
- Flexibility: Simplifies adding new features or changing business processes
Key components of event-driven systems include:
- Domain Events: Represent significant changes in the domain
- Message Bus: Routes events to appropriate handlers
- Event Handlers: React to specific events and perform actions
This architecture enables systems to handle complex workflows and integrate multiple services while maintaining modularity and scalability.
5. Command-Query Responsibility Segregation (CQRS) optimizes read and write operations
Reads and writes are different, so they should be treated differently (or have their responsibilities segregated, if you will).
CQRS separates the read and write models of an application, allowing each to be optimized independently. This pattern is particularly useful for complex domains or high-performance systems. Benefits include:
- Performance optimization: Read and write models can be scaled separately
- Simplified models: Each model focuses on a single responsibility
- Flexibility: Enables use of different data stores for reads and writes
Implementation strategies:
- Separate read and write models
- Use different databases for reads and writes
- Implement eventual consistency between read and write sides
While CQRS adds complexity, it can significantly improve performance and scalability in the right scenarios.
6. Dependency Injection promotes flexibility and testability
Dependency injection (DI) is a technique whereby an object's dependencies are provided to it, rather than the object itself creating or managing those dependencies.
Dependency Injection is a design pattern that improves code modularity, testability, and flexibility. Key benefits include:
- Loose coupling: Objects don't need to know how their dependencies are created
- Testability: Easy to swap real implementations with test doubles
- Flexibility: Simplifies changing implementations without modifying dependent code
Implementing DI:
- Constructor injection: Dependencies provided through the constructor
- Property injection: Dependencies set through public properties
- Method injection: Dependencies provided as method parameters
By using DI, developers can create more modular and maintainable code, especially when combined with other patterns like Repository and Unit of Work.
7. Validation at the system's edge ensures data integrity and simplifies the domain
Validate at the edge when possible. Validating required fields and the permissible ranges of numbers is boring, and we want to keep it out of our nice clean codebase. Handlers should always receive only valid messages.
Edge validation involves verifying inputs at the system's entry points before they reach the domain logic. This approach offers several advantages:
- Clean domain model: Domain logic focuses on business rules, not input validation
- Improved security: Catches malformed or malicious inputs early
- Better user experience: Provides immediate feedback on invalid inputs
Types of validation:
- Syntactic: Ensures correct data structure and types
- Semantic: Verifies the meaning and consistency of data
- Pragmatic: Applies business rules in the context of the operation
By implementing thorough validation at the system's edge, developers can create more robust and maintainable applications while keeping the domain model focused on core business logic.
Last updated:
FAQ
What's Architecture Patterns with Python about?
- Focus on Software Architecture: The book delves into architectural patterns tailored for Python, emphasizing Test-Driven Development (TDD), Domain-Driven Design (DDD), and event-driven microservices.
- Real-World Application: It uses practical examples from the authors' experiences at MADE.com to illustrate managing complexity in software systems.
- Framework Agnostic: While discussing frameworks like Flask and SQLAlchemy, the principles are applicable across various technologies and languages.
Why should I read Architecture Patterns with Python?
- Improve Software Design: The book offers insights into structuring applications for enhanced testability and maintainability, crucial for long-term software health.
- Learn from Experts: Authored by experienced software architects Harry Percival and Bob Gregory, it provides practical advice grounded in real-world scenarios.
- Comprehensive Coverage: It covers a range of architectural patterns, making it valuable for both beginners and experienced developers.
What are the key takeaways of Architecture Patterns with Python?
- Understanding Architectural Patterns: Readers learn about patterns like Repository, Unit of Work, and Event-Driven Architecture, and their implementation in Python.
- Emphasis on TDD and DDD: The book highlights the importance of TDD and DDD in managing complexity and ensuring accurate business logic representation.
- Event-Driven Systems: It discusses building systems that respond to events, allowing for flexible and decoupled architectures.
What are the best quotes from Architecture Patterns with Python and what do they mean?
- "Events are simple dataclasses...": This quote emphasizes the role of events in capturing and communicating changes within an event-driven architecture.
- "We use events as our data structure...": It illustrates how events serve as both input data and a means to trigger internal processes, enhancing modularity.
- "Our ongoing objective with these architectural patterns...": This underscores managing complexity in software design, ensuring applications remain manageable as they grow.
What is the Repository pattern as described in Architecture Patterns with Python?
- Abstraction Over Data Storage: The Repository pattern abstracts data access, keeping the domain model independent of the underlying data storage technology.
- Simplifies Testing: By using repositories, developers can easily mock data access in tests, leading to faster and more reliable unit tests.
- Encourages Separation of Concerns: This pattern maintains a clean separation between domain logic and data access logic, making the codebase easier to manage.
How does the Unit of Work pattern work in Architecture Patterns with Python?
- Atomic Operations: The Unit of Work pattern groups multiple operations into a single transaction, ensuring all changes are committed or rolled back together.
- Context Manager: Implemented as a context manager in Python, it simplifies the management of database sessions and transactions.
- Integration with Repositories: It collaborates with repositories to provide a cohesive interface for data access while maintaining transactional integrity.
What is Domain-Driven Design (DDD) and how is it applied in Architecture Patterns with Python?
- Focus on Business Logic: DDD emphasizes modeling software based on the business domain, ensuring code reflects real-world processes and rules.
- Ubiquitous Language: The book stresses using a common language between developers and domain experts to avoid misunderstandings and ensure clarity.
- Aggregates and Consistency Boundaries: DDD introduces aggregates, clusters of related objects treated as a single unit for data changes, maintaining consistency.
What are Domain Events and how are they used in Architecture Patterns with Python?
- Capturing State Changes: Domain Events represent changes in the system, allowing different application parts to react without tight coupling.
- Event-Driven Architecture: The book illustrates using events to trigger workflows and actions across system components, promoting a decoupled architecture.
- Integration with Message Bus: Events are published to a message bus, routing them to handlers, enabling clean separation of concerns and asynchronous processing.
How does Architecture Patterns with Python address testing strategies?
- Test Pyramid Concept: The authors advocate for a healthy test pyramid, emphasizing a majority of unit tests, fewer integration tests, and minimal end-to-end tests.
- Service Layer Testing: The book demonstrates testing service layer functions using fakes and mocks, allowing for fast and reliable tests focusing on business logic.
- Encouraging Refactoring: By structuring tests around the service layer and using events, the book encourages developers to refactor code without fear of breaking functionality.
What are some best practices for implementing architectural patterns in Python according to Architecture Patterns with Python?
- Start Simple: Begin with simple implementations of patterns and gradually refactor as the system grows in complexity.
- Use Context Managers: For managing resources like database sessions, context managers are encouraged for their clarity and ease of use.
- Decouple Components: Keep components loosely coupled, allowing for easier testing and maintenance, and enabling system evolution without significant rewrites.
What is the significance of event-driven architecture in Architecture Patterns with Python?
- Decoupled Systems: Event-driven architecture promotes decoupled systems where components communicate through events, leading to greater flexibility and scalability.
- Asynchronous Processing: It allows for asynchronous event processing, enabling systems to handle high loads and improve responsiveness.
- Improved Maintainability: Using events to trigger actions makes the architecture more maintainable, as changes to one component do not directly impact others.
What are the challenges of using an event-driven architecture as discussed in Architecture Patterns with Python?
- Complexity in Handling Events: Event-driven systems introduce complexity in managing event flows and ensuring correct system responses.
- Potential for Circular Dependencies: The book warns about the risk of circular dependencies between event handlers, leading to difficult-to-debug issues.
- Performance Considerations: The authors discuss trade-offs between synchronous and asynchronous processing, highlighting the need to balance responsiveness with complexity.
Review Summary
Architecture Patterns with Python receives high praise for its practical approach to Domain-Driven Design and software architecture. Readers appreciate its clear explanations, real-world examples, and balanced perspective on different patterns. The book is commended for its focus on Test-Driven Development and its engaging writing style. Many find it valuable for both beginners and experienced developers, offering insights into building scalable and maintainable Python applications. Some readers note that the approach may be overly complex for certain situations, but overall, the book is highly recommended for those interested in improving their software design skills.
Similar Books
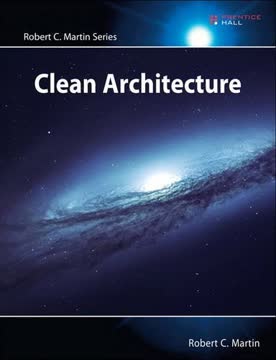
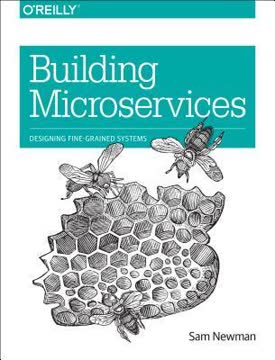
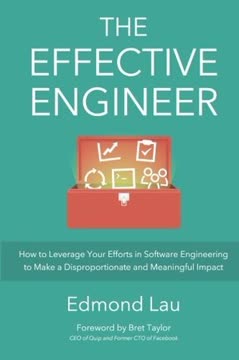
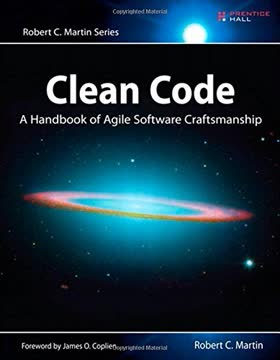

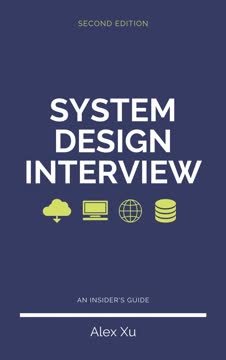
Download PDF
Download EPUB
.epub
digital book format is ideal for reading ebooks on phones, tablets, and e-readers.