Key Takeaways
1. Clean code is essential for maintainable software projects
Clean code is not just a nice thing to have or a luxury in software projects. It's a necessity.
Code readability matters. Clean code is fundamental to the long-term success of any software project. It goes beyond mere formatting and encompasses the overall structure, clarity, and maintainability of the codebase. Key aspects of clean code include:
- Consistent formatting and naming conventions
- Clear and concise documentation
- Modular and well-organized code structure
- Minimal code duplication (DRY principle)
- Ease of understanding and modification
Tools for code quality. To maintain clean code, developers should leverage various tools:
- Linters (e.g., Pylint) for style and error checking
- Type checkers (e.g., Mypy) for static type analysis
- Code formatters (e.g., Black) for consistent styling
- Automated testing frameworks for ensuring code correctness
2. Pythonic code leverages language-specific features for efficiency
Pythonic code is in general much better quality code.
Embrace Python's idioms. Writing Pythonic code means utilizing language-specific features and conventions to create more readable, efficient, and maintainable code. Key Pythonic concepts include:
- List comprehensions and generator expressions
- Context managers (with statements)
- Proper use of built-in functions and data structures
- Leveraging duck typing and dynamic nature of Python
Avoid common pitfalls. Non-Pythonic practices to avoid:
- Using mutable default arguments
- Improperly extending built-in types
- Overusing global variables
- Neglecting Python's standard library features
By adhering to Pythonic principles, developers can write code that is not only more efficient but also more aligned with the Python community's best practices.
3. Design principles promote flexibility and extensibility in software
The idea of inverting dependencies is that our code should not adapt to details or concrete implementations, but rather the other way around.
Separation of concerns. Good software design relies on several key principles that promote modularity, flexibility, and maintainability:
- Single Responsibility Principle (SRP): Each component should have one reason to change
- Open/Closed Principle (OCP): Open for extension, closed for modification
- Dependency Inversion Principle (DIP): Depend on abstractions, not concretions
Design for change. Implementing these principles leads to:
- Easier code maintenance and updates
- Improved testability and debugging
- Enhanced code reusability
- Reduced ripple effects when making changes
By adhering to these design principles, developers can create software that is more resilient to change and easier to extend over time.
4. SOLID principles guide robust object-oriented design
The SOLID principles are key guidelines for good object-oriented software design.
SOLID breakdown. The SOLID principles provide a framework for creating maintainable and scalable object-oriented systems:
- Single Responsibility Principle (SRP)
- Open/Closed Principle (OCP)
- Liskov Substitution Principle (LSP)
- Interface Segregation Principle (ISP)
- Dependency Inversion Principle (DIP)
Benefits of SOLID. Applying these principles leads to:
- More modular and loosely coupled code
- Easier testing and maintenance
- Improved code reusability
- Better adaptability to changing requirements
While these principles originated in static typing contexts, they can be adapted and applied effectively in Python's dynamic environment, leading to more robust and flexible object-oriented designs.
5. Decorators enhance code reusability and separation of concerns
Decorators are powerful tools in Python that can be applied to many things such as classes, methods, functions, generators, and many more.
Versatile code enhancement. Decorators provide a clean way to modify or extend the behavior of functions, methods, or classes without altering their source code. Common uses include:
- Adding logging or timing functionality
- Implementing caching or memoization
- Managing access control or authentication
- Transforming function arguments or return values
Best practices. When working with decorators:
- Use functools.wraps to preserve metadata of decorated functions
- Create decorators that can work with both functions and methods
- Avoid side effects in the body of the decorator
- Use class-based decorators for more complex scenarios
Decorators exemplify Python's "batteries included" philosophy, offering a powerful tool for code reuse and separation of concerns.
6. Descriptors provide powerful attribute management in Python
Descriptors are another distinctive feature of Python that takes object-oriented programming to another level.
Advanced attribute control. Descriptors allow fine-grained control over attribute access, modification, and deletion in Python objects. They are particularly useful for:
- Implementing computed or managed attributes
- Enforcing type checking or validation
- Creating reusable attribute behavior across multiple classes
Types of descriptors:
- Data descriptors: Implement set and/or delete
- Non-data descriptors: Implement only get
Descriptors are the underlying mechanism for many Python features, including properties, methods, and class methods. Understanding and utilizing descriptors can lead to more elegant and powerful object-oriented designs in Python.
7. Generators and coroutines enable efficient data processing and asynchronous programming
Generators are probably the best feature of Python.
Memory-efficient iteration. Generators provide a way to create iterators that produce values on-demand, rather than storing them all in memory. Benefits include:
- Reduced memory usage for large datasets
- Improved performance for processing sequences
- Simplified code for complex iterations
Asynchronous programming. Coroutines, built on top of generators, enable:
- Non-blocking I/O operations
- Concurrent programming without threads
- Efficient handling of many simultaneous operations
Key concepts:
- yield and yield from statements
- async and await syntax (for modern coroutines)
- Event loops and asynchronous frameworks (e.g., asyncio)
Mastering generators and coroutines allows developers to write more efficient and scalable Python applications, particularly for I/O-bound and data processing tasks.
8. Unit testing ensures code quality and facilitates refactoring
Unit tests (and any form of automatic tests, for that matter) are critical to software maintainability.
Quality assurance. Unit tests provide numerous benefits to software development:
- Early bug detection and prevention
- Documentation of expected behavior
- Confidence in code changes and refactoring
- Improved design through testability considerations
Testing best practices:
- Write tests before or alongside code (Test-Driven Development)
- Aim for high code coverage, but focus on critical paths
- Use mocking to isolate units of code
- Regularly run and maintain the test suite
Popular Python testing frameworks:
- unittest (built-in)
- pytest (third-party, with enhanced features)
Incorporating unit testing into the development process leads to higher quality, more maintainable code, and increased confidence in software reliability.
9. Design patterns offer reusable solutions to common software problems
Design patterns are key guidelines for good object-oriented software design.
Proven solutions. Design patterns represent best practices for solving common software design problems. They provide:
- A shared vocabulary for developers
- Tested and proven design solutions
- Improved code flexibility and maintainability
Categories of patterns:
- Creational patterns (e.g., Factory, Singleton)
- Structural patterns (e.g., Adapter, Decorator)
- Behavioral patterns (e.g., Observer, Strategy)
Python-specific considerations. While many design patterns are language-agnostic, Python's dynamic nature and built-in features can simplify or even obviate the need for some traditional patterns. For example:
- Python's duck typing can replace complex inheritance hierarchies
- First-class functions can simplify strategy and command patterns
- Context managers provide a Pythonic alternative to some structural patterns
When applying design patterns in Python, it's crucial to consider the language's unique features and idiomatic practices to create clean, efficient, and truly Pythonic code.
Last updated:
Review Summary
Clean Code in Python receives mostly positive reviews, with an average rating of 4.13/5. Readers appreciate its coverage of clean coding principles, Pythonic practices, and advanced topics like decorators and design patterns. Many find it valuable for improving OOP skills and code quality. Some readers note the book's depth and practical examples, while a few criticize its writing style and lack of emphasis on certain topics. Overall, it's recommended for intermediate to advanced Python developers seeking to enhance their coding practices and deepen their understanding of the language.
Similar Books
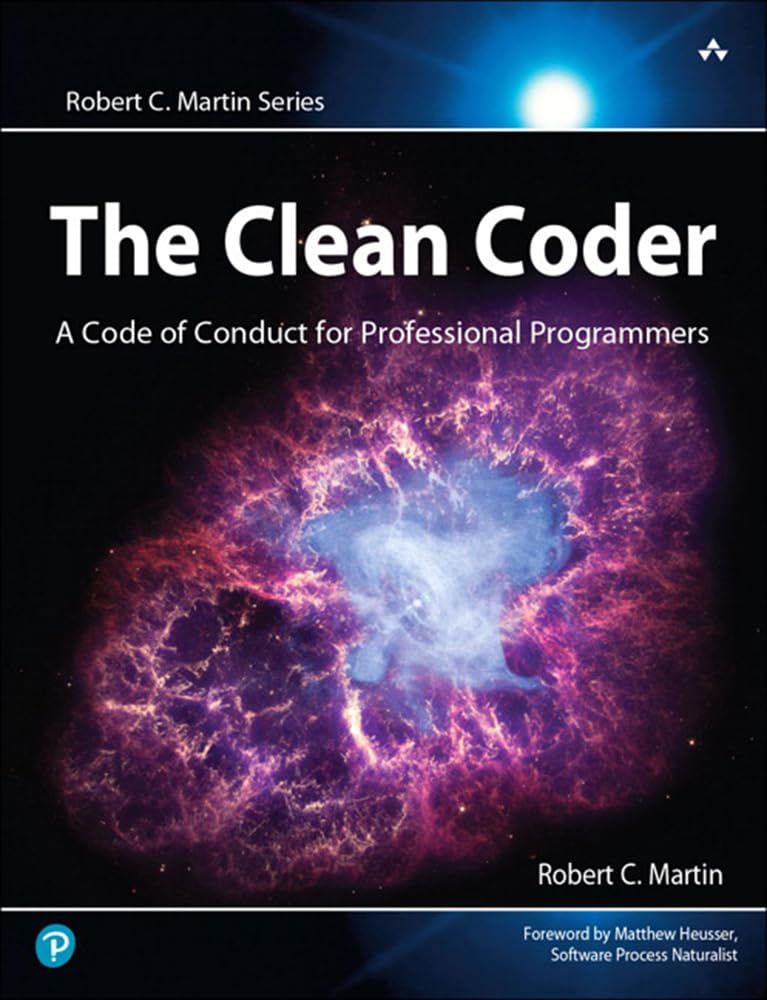
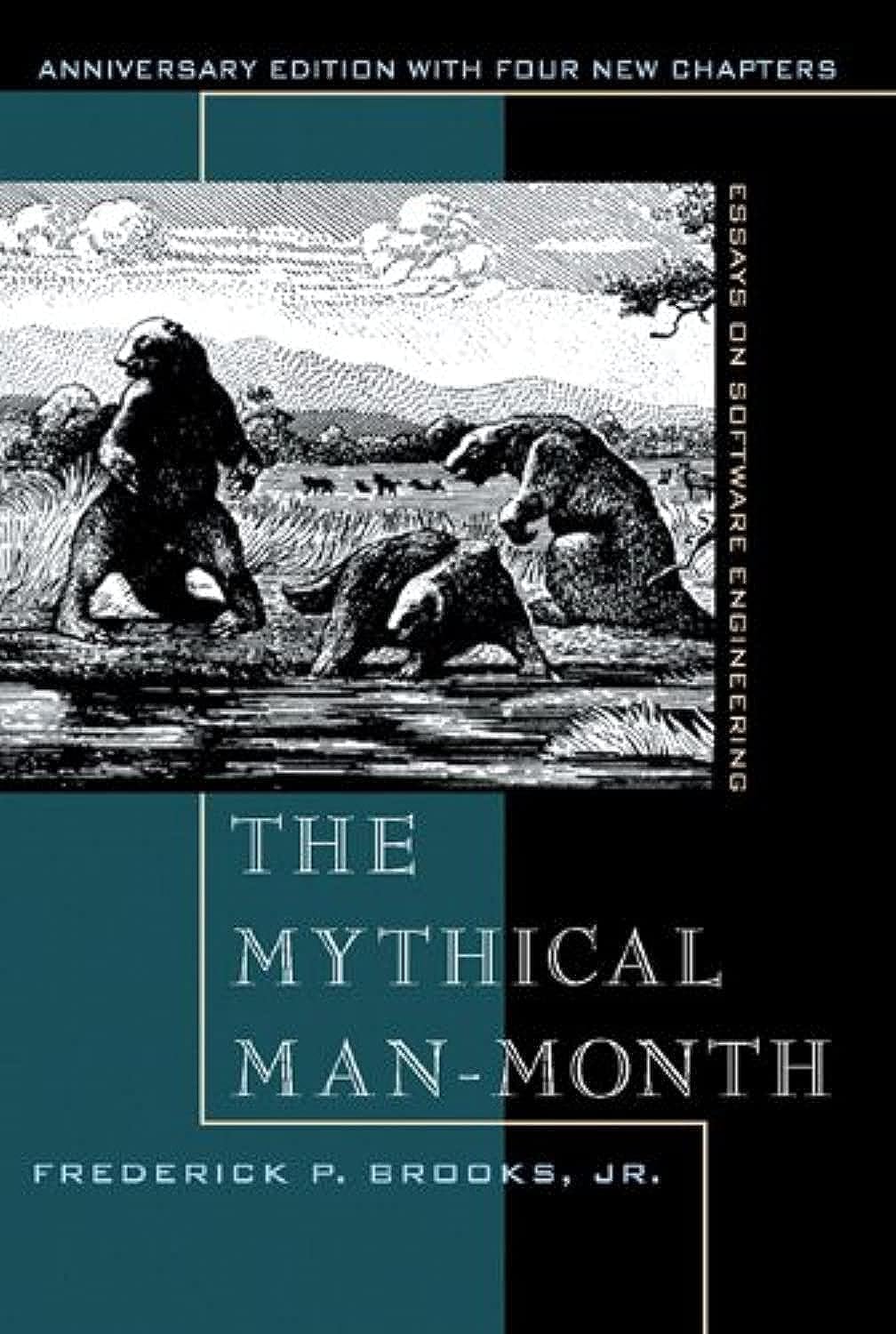
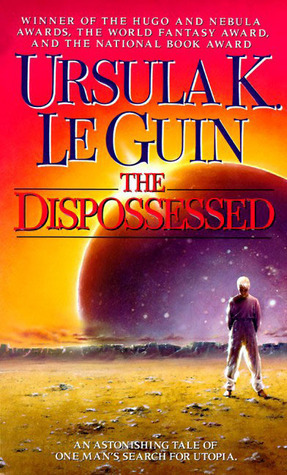
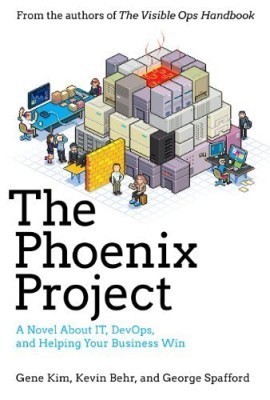
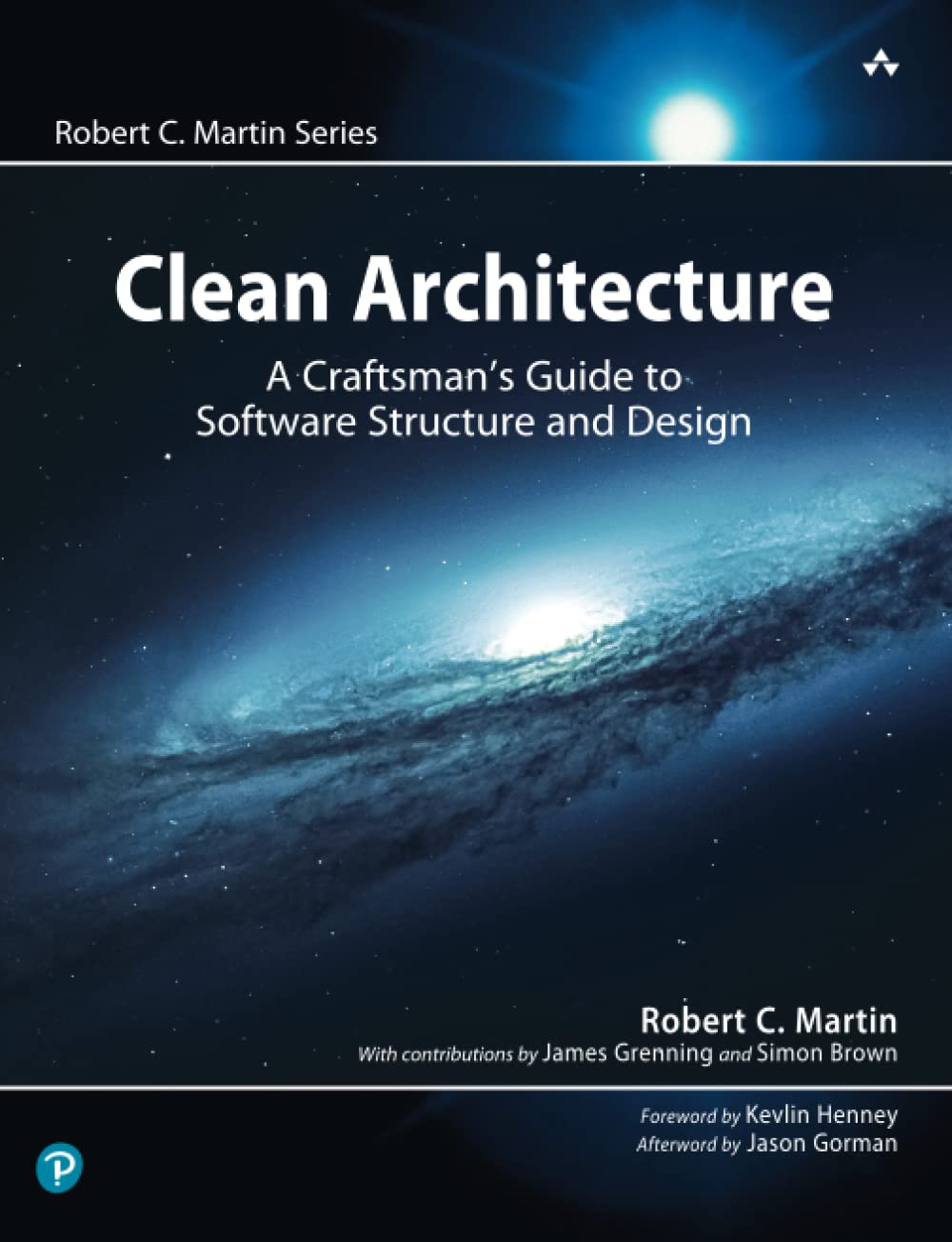
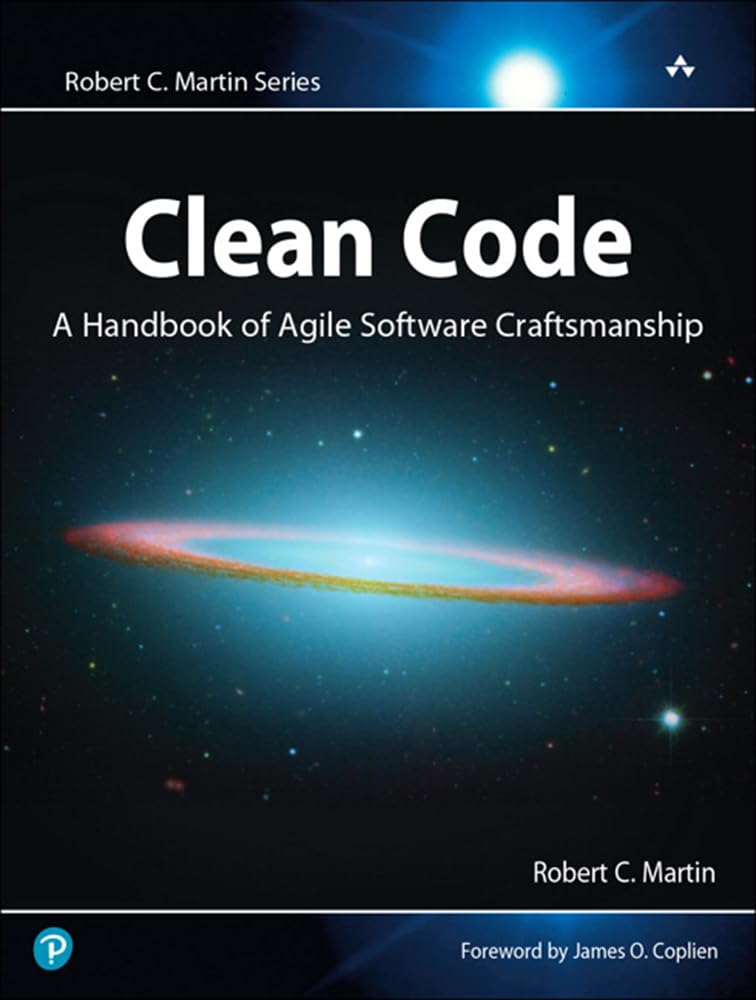
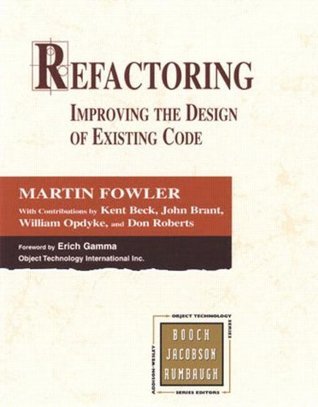
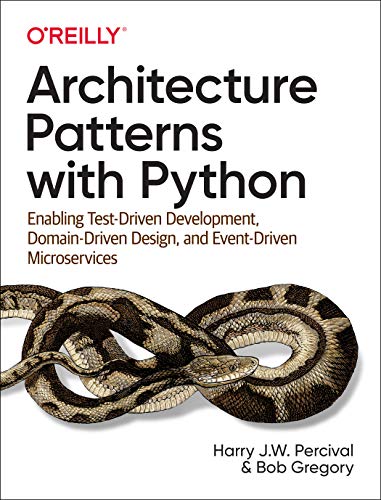
Download PDF
Download EPUB
.epub
digital book format is ideal for reading ebooks on phones, tablets, and e-readers.