Key Takeaways
1. Lambda expressions revolutionize Java programming with concise, functional-style code
Lambda expressions are deceivingly concise and it's easy to carelessly duplicate them in code.
Concise syntax: Lambda expressions allow developers to write more expressive and readable code in Java. They provide a way to pass behavior as an argument to methods, enabling functional programming paradigms.
Improved readability: By reducing boilerplate code, lambda expressions make it easier to understand the intent of the code at a glance. This is particularly useful for operations on collections, event handling, and callback implementations.
Functional programming in Java: Lambda expressions facilitate a more functional style of programming, encouraging immutability and side-effect-free functions. This leads to code that is easier to reason about, test, and maintain.
2. Functional interfaces enable seamless integration of lambda expressions
A functional interface is an interface with one abstract—unimplemented—method.
Single abstract method: Functional interfaces are the foundation for lambda expressions in Java. They define a single abstract method, which allows them to be implemented using lambda syntax.
Built-in functional interfaces: Java 8 introduces several built-in functional interfaces in the java.util.function package, such as:
- Predicate<T>: Represents a boolean-valued function of one argument
- Function<T,R>: Represents a function that accepts one argument and produces a result
- Consumer<T>: Represents an operation that accepts a single input argument and returns no result
- Supplier<T>: Represents a supplier of results
Custom functional interfaces: Developers can create their own functional interfaces to suit specific needs, enabling the use of lambda expressions in domain-specific contexts.
3. Streams provide powerful data processing capabilities with lazy evaluation
Streams have two types of methods: intermediate and terminal, which work together.
Declarative data processing: Streams allow developers to express complex data processing operations in a declarative manner. This leads to more readable and maintainable code compared to imperative approaches.
Lazy evaluation: Stream operations are lazily evaluated, meaning that computation on the data is only performed when necessary. This can lead to significant performance improvements, especially when dealing with large datasets.
Composition of operations: Streams support a fluent API for composing multiple operations:
- filter(): Selects elements based on a predicate
- map(): Transforms elements
- reduce(): Combines elements to produce a single result
- collect(): Gathers elements into a collection or other data structure
4. Method references offer a shorthand syntax for lambda expressions
Method references can also refer to static methods and methods that take parameters.
Concise alternative: Method references provide a more compact way to write lambda expressions when the lambda simply calls an existing method.
Types of method references:
- Static method reference: ClassName::staticMethodName
- Instance method reference of a particular object: objectReference::instanceMethodName
- Instance method reference of an arbitrary object of a particular type: ClassName::instanceMethodName
- Constructor reference: ClassName::new
Improved readability: In many cases, method references can make code more readable by clearly indicating the intent to use an existing method rather than defining new behavior.
5. The Collections API is enhanced with functional-style operations
We can easily find the oldest person in the list.
Stream operations on collections: The Collections API now includes methods to obtain streams, allowing for powerful data processing operations directly on collection objects.
Functional-style operations: New methods have been added to collection interfaces to support functional-style operations:
- forEach(): Performs an action for each element
- removeIf(): Removes all elements that match a predicate
- replaceAll(): Replaces each element with the result of applying a function
Improved performance: Many of these operations can be more efficient than their imperative counterparts, especially when dealing with large collections or when parallelism is desired.
6. Functional programming concepts improve code quality and maintainability
We can achieve the same now, but with far less code.
Immutability: Functional programming encourages the use of immutable data structures, reducing the risk of side effects and making code easier to reason about and debug.
Higher-order functions: The ability to pass functions as arguments and return them as results enables powerful abstractions and code reuse.
Pure functions: Emphasizing functions without side effects leads to more predictable and testable code.
Benefits:
- Easier parallel processing
- Improved code organization
- Reduced complexity
- Enhanced testability
7. Design patterns are simplified and more expressive with lambda expressions
We can now realize this pattern with less effort in Java.
Simplified implementations: Many traditional design patterns can be implemented more concisely using lambda expressions and functional interfaces.
Examples of simplified patterns:
- Strategy pattern: Pass different behaviors as lambda expressions
- Observer pattern: Use functional interfaces for event listeners
- Decorator pattern: Compose behaviors using lambda expressions
- Template method pattern: Pass customizable behavior as method arguments
Improved flexibility: The functional approach often results in more flexible and composable designs compared to traditional object-oriented implementations.
8. Resource management becomes more efficient with functional techniques
We can now enjoy a lightweight syntax in Java with lambda expressions.
Try-with-resources: Java 7 introduced the try-with-resources statement for automatic resource management. Java 8 enhances this with lambda expressions.
Functional resource management: Lambda expressions enable more flexible and concise resource management patterns:
- Delayed initialization
- Lazy evaluation of resources
- Custom resource handling strategies
Benefits:
- Reduced boilerplate code
- Improved resource cleanup
- More expressive error handling
9. Recursion and memoization are optimized using functional approaches
Memoization is a simple yet smart technique to make recursive overlapping computations really fast.
Tail-call optimization: While Java doesn't support automatic tail-call optimization, functional techniques can be used to implement it manually, allowing for more efficient recursive algorithms.
Memoization: Functional programming facilitates easy implementation of memoization, a technique to cache results of expensive function calls and avoid redundant computations.
Benefits:
- Improved performance for recursive algorithms
- Reduced stack overflow risks
- Elegant solutions for dynamic programming problems
10. Parallel processing is greatly simplified with the new Java 8 features
We can easily parallelize the previous example to speed up the execution.
Parallel streams: Java 8 introduces parallel streams, allowing easy parallelization of data processing operations without explicit thread management.
Fork/Join framework integration: Parallel streams leverage the Fork/Join framework under the hood, providing efficient work-stealing for parallel tasks.
Parallelization considerations:
- Data size: Parallelization is most beneficial for large datasets
- Operation complexity: Computationally intensive operations benefit more from parallelization
- Thread safety: Ensure that operations are thread-safe when using parallel streams
</instructions>
Last updated:
FAQ
What's "Functional Programming in Java: Harnessing the Power of Java 8 Lambda Expressions" about?
- Focus on Java 8: The book explores the new features introduced in Java 8, particularly lambda expressions, and how they enable functional programming in Java.
- Functional Programming Concepts: It introduces core functional programming concepts such as immutability, statelessness, and functions as values.
- Practical Examples: The book provides practical examples and exercises to help readers understand and apply functional programming techniques in Java.
- Integration with OOP: It discusses how functional programming can complement object-oriented programming, enhancing Java's capabilities.
Why should I read "Functional Programming in Java" by Venkat Subramaniam?
- Enhance Java Skills: It helps Java developers upgrade their skills by learning functional programming, which is increasingly important in modern software development.
- Improve Code Quality: The book demonstrates how functional programming can lead to more concise, readable, and maintainable code.
- Performance Benefits: Readers will learn how functional programming can improve performance, especially with parallel processing.
- Real-World Applications: The book provides insights into applying functional programming in real-world Java applications, making it practical and relevant.
What are the key takeaways of "Functional Programming in Java"?
- Lambda Expressions: Understanding and using lambda expressions to write more concise and expressive Java code.
- Immutability and Statelessness: Emphasizing the importance of immutability and statelessness in reducing errors and improving code maintainability.
- Function Composition: Learning how to compose functions to create more modular and reusable code.
- Parallel Processing: Leveraging Java 8's Stream API for efficient parallel processing and improved performance.
How does "Functional Programming in Java" explain lambda expressions?
- Syntax and Usage: The book explains the syntax of lambda expressions and how they can replace anonymous inner classes.
- Functional Interfaces: It covers the concept of functional interfaces, which are essential for using lambda expressions in Java.
- Examples and Exercises: Practical examples demonstrate how to use lambda expressions in various scenarios, such as filtering and transforming collections.
- Integration with Streams: The book shows how lambda expressions work seamlessly with Java 8's Stream API for data processing.
What are the benefits of using functional programming in Java, according to the book?
- Conciseness and Clarity: Functional programming leads to more concise and clear code, reducing boilerplate and improving readability.
- Reduced Errors: By promoting immutability and statelessness, functional programming reduces the likelihood of bugs related to mutable state.
- Easier Parallelization: Functional code is easier to parallelize, allowing for better utilization of multicore processors.
- Improved Maintainability: The modular nature of functional programming makes code easier to maintain and extend.
How does "Functional Programming in Java" address the integration of functional and object-oriented programming?
- Complementary Paradigms: The book explains how functional programming can complement object-oriented programming, rather than replace it.
- Design Patterns: It discusses how traditional design patterns can be implemented more elegantly using functional programming techniques.
- Separation of Concerns: Functional programming encourages separating concerns, leading to cleaner and more modular code.
- Real-World Examples: The book provides examples of integrating functional and object-oriented programming in real-world Java applications.
What are some of the best quotes from "Functional Programming in Java" and what do they mean?
- "Programs must be written for people to read, and only incidentally for machines to execute." This quote emphasizes the importance of writing code that is easy for humans to understand, a key principle of functional programming.
- "Any intelligent fool can make things bigger, more complex, and more violent. It takes a touch of genius—and a lot of courage—to move in the opposite direction." This highlights the value of simplicity and elegance in programming, which functional programming promotes.
- "Progress is made by lazy men looking for easier ways to do things." This quote humorously underscores the efficiency gains from lazy evaluation, a concept in functional programming.
How does the book explain the concept of immutability and its importance?
- Definition of Immutability: Immutability means that once an object is created, its state cannot be changed, which helps prevent bugs.
- Benefits of Immutability: The book explains how immutability leads to safer, more predictable code and simplifies concurrent programming.
- Java Support: It discusses how Java supports immutability and how developers can enforce it in their code.
- Practical Examples: Examples in the book show how to implement immutability in Java applications effectively.
What is the role of Streams in Java 8, as explained in the book?
- Data Processing: Streams provide a high-level abstraction for processing sequences of elements, making data manipulation more intuitive.
- Lazy Evaluation: The book highlights how Streams use lazy evaluation to optimize performance by delaying computation until necessary.
- Parallel Processing: Streams make it easy to parallelize data processing, improving performance on multicore systems.
- Functional Operations: Streams support functional-style operations like map, filter, and reduce, which are central to functional programming.
How does "Functional Programming in Java" address performance concerns with functional programming?
- Efficient Execution: The book explains how functional programming can lead to efficient execution, especially with parallel processing.
- Compiler Optimizations: It discusses how Java 8's compiler optimizations and the invokedynamic instruction improve performance.
- Profiling and Evaluation: The book encourages profiling and evaluating code to identify performance bottlenecks and optimize them.
- Real-World Considerations: It provides practical advice on when to use functional programming for performance gains in real-world applications.
What are some practical examples of functional programming in Java provided in the book?
- Collection Manipulation: Examples include filtering, transforming, and reducing collections using Streams and lambda expressions.
- Concurrency and Parallelism: The book demonstrates how to use functional programming for concurrent and parallel processing.
- Design Patterns: It shows how to implement design patterns like strategy and decorator using functional programming techniques.
- Resource Management: Examples illustrate how to manage resources and handle exceptions using functional programming constructs.
How can developers successfully adopt functional programming in Java, according to the book?
- Gradual Transition: The book suggests starting with small, manageable changes and gradually adopting functional programming techniques.
- Practice and Experimentation: It encourages developers to practice and experiment with functional programming to build confidence and expertise.
- Community and Collaboration: Engaging with the developer community and collaborating with peers can accelerate learning and adoption.
- Continuous Learning: The book emphasizes the importance of continuous learning and staying updated with the latest developments in Java and functional programming.
Review Summary
Functional Programming in Java receives mostly positive reviews, with an average rating of 4.16/5. Readers appreciate its concise introduction to functional programming concepts and Java 8 features. Many find it helpful for understanding lambdas and their practical applications. The author's enthusiastic style is praised, though some find it verbose. The book is considered particularly useful for beginners transitioning from imperative to functional programming. Some readers note that while it provides a good overview, it may lack depth for more experienced developers.
Similar Books
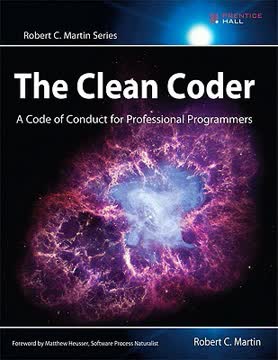

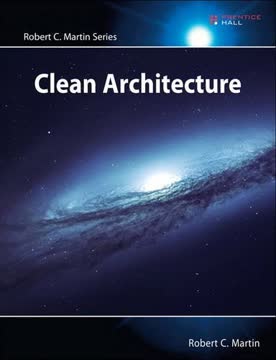
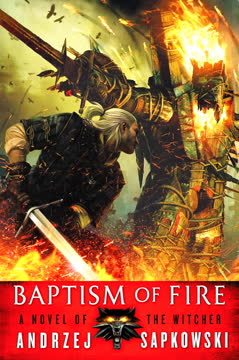
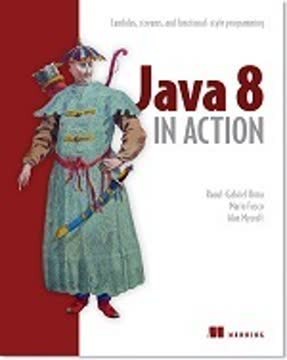
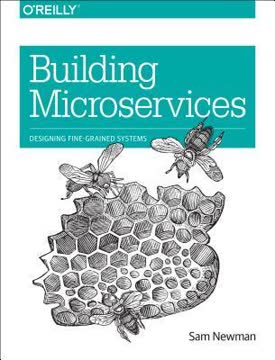

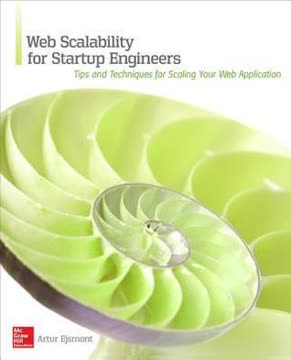
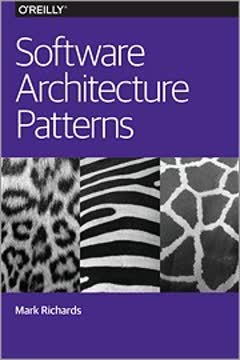
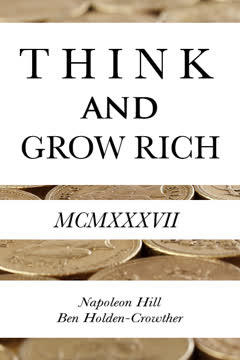
Download PDF
Download EPUB
.epub
digital book format is ideal for reading ebooks on phones, tablets, and e-readers.