Key Takeaways
1. Regular expressions are powerful tools for text processing and pattern matching
Regular expressions are the key to powerful, flexible, and efficient text processing.
Versatile pattern matching: Regular expressions provide a concise and flexible means to "match" a particular pattern of characters within a string. They are used in a wide range of applications, including:
- Text editors for search and replace operations
- Data validation in forms and input fields
- Parsing and extracting information from structured text
- Log file analysis and system administration tasks
- Natural language processing and text mining
Universally supported: Most modern programming languages and text processing tools incorporate regex support, making them a fundamental skill for developers and data analysts. Examples include:
- Perl, Python, Java, JavaScript, and Ruby
- Unix command-line tools like grep, sed, and awk
- Database systems for advanced string matching and manipulation
2. Understanding regex engines: NFA vs DFA approaches
The two basic technologies behind regular-expression engines have the somewhat imposing names Nondeterministic Finite Automaton (NFA) and Deterministic Finite Automaton (DFA).
NFA (Nondeterministic Finite Automaton):
- Regex-directed approach
- Used in most modern languages (Perl, Python, Java, .NET)
- Allows for powerful features like backreferences and lookaround
- Performance can vary based on regex construction
DFA (Deterministic Finite Automaton):
- Text-directed approach
- Used in traditional Unix tools (awk, egrep)
- Generally faster and more consistent performance
- Limited feature set compared to NFA engines
Understanding the differences between these engines is crucial for writing efficient and effective regular expressions, as the same regex can behave differently depending on the underlying implementation.
3. Mastering regex syntax: Metacharacters, quantifiers, and anchors
The metacharacter rules change depending on whether you're in a character class or not.
Core regex components:
- Metacharacters: Special characters with unique meanings (e.g., . * + ? |)
- Character classes: Sets of characters to match (e.g., [a-z], [^0-9])
- Quantifiers: Specify repetition of preceding elements (* + ? {n,m})
- Anchors: Match positions rather than characters (^ $ \b)
- Grouping and capturing: Parentheses for logical grouping and text extraction
Context-sensitive behavior: The interpretation of certain characters changes based on their context within the regex. For example:
- A hyphen (-) is a literal character outside a character class, but denotes a range inside one
- A caret (^) means "start of line" outside a class, but "negation" at the start of a class
Mastering these nuances allows for precise and powerful pattern matching across various regex flavors and implementations.
4. Crafting efficient regexes: Balancing correctness and performance
Writing a good regex involves striking a balance among several concerns.
Key considerations:
- Correctness: Accurately matching desired patterns while avoiding false positives
- Readability: Creating expressions that are maintainable and understandable
- Efficiency: Optimizing for speed and resource usage, especially for large-scale processing
Balancing strategies:
- Use specific patterns over overly general ones when possible
- Avoid unnecessary backtracking by careful ordering of alternatives
- Leverage regex engine optimizations (e.g., anchors, literal text exposure)
- Break complex patterns into multiple simpler regexes when appropriate
- Benchmark and profile regex performance with representative data sets
Remember that the most efficient regex is not always the most readable or maintainable. Strive for a balance that fits the specific requirements of your project and team.
5. Optimization techniques: Exposing literal text and anchors
Expose Literal Text
Exposing literal text:
- Helps regex engines apply optimizations like fast substring searches
- Improves performance by allowing early failure for non-matching strings
Techniques:
- Factor out common prefixes: th(?:is|at) instead of this|that
- Use non-capturing groups (?:...) to avoid unnecessary capturing overhead
- Rearrange alternations to prioritize longer, more specific matches
Utilizing anchors:
- Anchors (^ $ \A \Z \b) provide positional context for matches
- Enable regex engines to quickly rule out non-matching positions
Best practices:
- Add ^ or \A to patterns that must match at the start of input
- Use $ or \Z for patterns that must match at the end
- Employ word boundaries \b to prevent partial word matches
By exposing literal text and leveraging anchors, you can significantly improve regex performance, especially for complex patterns applied to large datasets.
6. Advanced regex concepts: Lookaround, atomic grouping, and possessive quantifiers
Lookaround constructs are similar to word-boundary metacharacters like \b or the anchors ^ and $ in that they don't match text, but rather match positions within the text.
Lookaround:
- Positive lookahead (?=...) and lookbehind (?<=...)
- Negative lookahead (?!...) and lookbehind (?<!...)
- Allows for complex assertions without consuming characters
Atomic grouping (?>...):
- Prevents backtracking within the group
- Improves performance by committing to a match once found
Possessive quantifiers (*+ ++ ?+):
- Similar to atomic grouping, but applied to quantifiers
- Matches as much as possible and never gives back
These advanced features provide powerful tools for creating precise and efficient regular expressions:
- Use lookaround for complex matching conditions without altering the match boundaries
- Apply atomic grouping to prevent unnecessary backtracking in alternations
- Employ possessive quantifiers when backtracking is not needed (e.g., parsing well-formed data)
While not supported in all regex flavors, these concepts can dramatically improve both the expressiveness and performance of your patterns when available.
7. Unrolling the loop: A technique for optimizing complex patterns
Unrolling the loop
The unrolling technique:
- Transforms repetitive patterns like (this|that|...)* into more efficient forms
- Especially useful for optimizing matches with alternation inside quantifiers
Steps to unroll a loop:
- Identify the repeating pattern and its components
- Separate "normal" and "special" cases within the pattern
- Reconstruct the regex using the general form: normal+(special normal+)*
Benefits of unrolling:
- Reduces backtracking in many common scenarios
- Can transform "catastrophic" regexes into manageable ones
- Often results in faster matching, especially for non-matching cases
Example transformation:
- Original: "(\.|[^"\])*"
- Unrolled: "[^"\](\.[^"\])*"
The unrolled version can be orders of magnitude faster for certain inputs, particularly when there's no match. This technique requires a deep understanding of regex behavior and the specific pattern being optimized, but can yield substantial performance improvements for complex, frequently-used expressions.
Last updated:
FAQ
What's Mastering Regular Expressions about?
- Comprehensive Guide: Mastering Regular Expressions by Jeffrey E.F. Friedl is a detailed exploration of regular expressions (regex), covering their syntax, mechanics, and practical applications across various programming languages.
- Regex Engines: The book discusses different regex engines, focusing on Traditional NFA and DFA engines, explaining their operation and implications on performance.
- Practical Techniques: It provides practical techniques for crafting efficient regex patterns, emphasizing the importance of understanding backtracking and optimization strategies.
Why should I read Mastering Regular Expressions?
- Deep Understanding: This book is essential for anyone looking to gain a deep understanding of regex, whether for programming, data processing, or text manipulation.
- Real-World Examples: Friedl includes numerous real-world examples and exercises that help solidify the concepts, making it easier to apply regex in practical scenarios.
- Performance Insights: The book offers insights into performance issues and optimizations, crucial for writing efficient regex patterns that can handle large datasets or complex text processing tasks.
What are the key takeaways of Mastering Regular Expressions?
- Regex Mechanics: Understanding the mechanics of regex engines, including how they process patterns and match text, is crucial for effective use.
- Efficiency Techniques: The book provides techniques for crafting efficient expressions, helping you avoid common pitfalls that can lead to performance issues.
- Tool-Specific Information: It covers specific implementations in popular programming languages like Perl, Java, and .NET, allowing you to apply your knowledge in various contexts.
What are the best quotes from Mastering Regular Expressions and what do they mean?
- "To master regular expressions is to master your data.": This quote highlights the importance of regular expressions in effectively managing and manipulating data, emphasizing their power.
- "Regular expressions are an idea—one that is implemented in various ways by various utilities.": This reflects the versatility of regular expressions and how understanding the core concept can help you adapt to different tools and languages.
- "Understanding backtracking is perhaps the most important facet of NFA efficiency.": This statement stresses the importance of grasping how backtracking works in NFA engines, as it directly affects the performance and efficiency of regex operations.
How does Mastering Regular Expressions explain the mechanics of regex engines?
- DFA vs. NFA Engines: The book explains the differences between Deterministic Finite Automaton (DFA) and Nondeterministic Finite Automaton (NFA) engines, detailing how they process regex.
- Impact on Performance: It discusses how the choice of regex engine can affect performance and matching behavior, providing insights into crafting effective expressions.
- Practical Implications: The author emphasizes the importance of understanding the underlying mechanics of regex engines to optimize regex usage in programming.
What are the different types of regex engines discussed in Mastering Regular Expressions?
- Traditional NFA: This engine type is commonly used in many programming languages and is characterized by its backtracking behavior, which can lead to inefficiencies if not carefully managed.
- DFA (Deterministic Finite Automaton): DFA engines process regex patterns in a more linear fashion, making them faster for certain types of matches, but they lack some features like backreferences.
- POSIX NFA: This variant adheres to the POSIX standard, requiring the longest match to be found, which can lead to performance issues due to extensive backtracking.
How does backtracking affect regex performance in Mastering Regular Expressions?
- Increased Workload: Backtracking can significantly increase the workload of regex engines, especially in NFA implementations, as they may need to explore multiple paths to find a match.
- Exponential Matches: Certain regex patterns can lead to exponential backtracking, where the number of possible matches grows rapidly, causing the engine to take an impractically long time to return a result.
- Optimization Strategies: The book discusses various strategies to minimize backtracking, such as using possessive quantifiers and atomic grouping, which can help improve performance.
What are some practical techniques for writing efficient regex patterns in Mastering Regular Expressions?
- Use Non-Capturing Parentheses: When capturing is not needed, using non-capturing parentheses can reduce overhead and improve performance.
- Avoid Unnecessary Backtracking: Techniques such as reordering alternatives and using anchors can help avoid unnecessary backtracking, leading to faster matches.
- Leverage Atomic Grouping: Using atomic grouping can prevent the regex engine from backtracking into previously matched states, which can enhance efficiency.
What regex features are unique to Perl as discussed in Mastering Regular Expressions?
- Rich Regex Flavor: Perl's regex flavor includes features like non-capturing parentheses, lookahead, and lookbehind constructs, which enhance its expressive power.
- Modifiers for Flexibility: The book explains how Perl allows for modifiers that can change the behavior of regex patterns, such as case insensitivity and free-spacing.
- Integration with Perl Code: The author discusses how regex can be integrated with Perl code, allowing for dynamic and powerful text processing capabilities.
How does Mastering Regular Expressions address performance issues?
- Regex Compilation: The book explains how regex compilation can impact performance, particularly in languages like Perl. It discusses the use of the /o modifier to cache compiled regex for efficiency.
- Memory Usage: It highlights the importance of understanding memory usage when working with regex, especially with large strings. The author provides strategies to minimize memory overhead.
- Benchmarking Techniques: The book includes methods for benchmarking regex performance, allowing readers to measure and compare the efficiency of different regex patterns.
How can I optimize my regex patterns according to Mastering Regular Expressions?
- Use the /o Modifier: The book recommends using the /o modifier to compile regex patterns only once, which can significantly improve performance in repeated matches.
- Avoid Naughty Variables: It advises against using variables like $&, $', and $' as they can lead to unnecessary memory overhead due to pre-match copies.
- Benchmark Your Patterns: The author emphasizes the importance of benchmarking regex patterns to identify performance bottlenecks, allowing for refinement and optimization.
Review Summary
Mastering Regular Expressions is highly regarded as an essential book for programmers learning regex. Readers praise its comprehensive coverage, from basics to advanced techniques, and its clear explanations of regex engines. Many found it demystified a challenging topic, though some non-programmers found it difficult. The book is particularly valued for teaching efficient regex thinking and implementation. While some content may be outdated, it remains a go-to reference. Criticisms include occasional verbosity and dated examples, but overall, it's considered the definitive work on regular expressions.
Similar Books
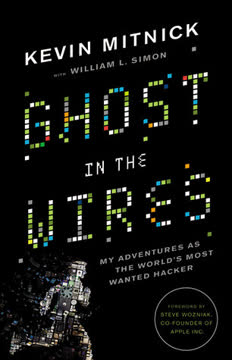
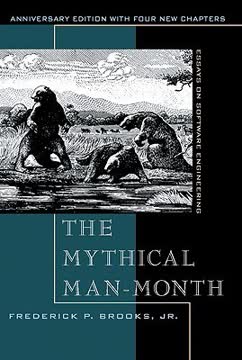
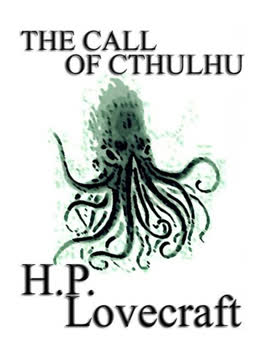
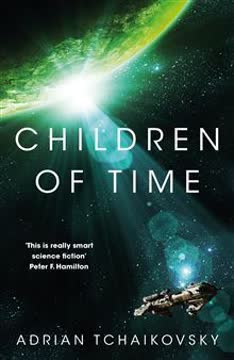
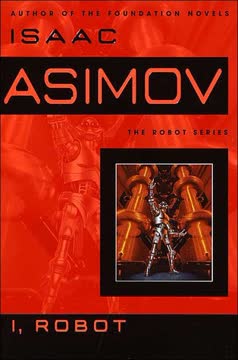
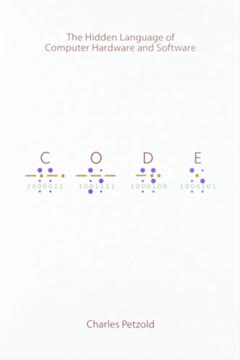
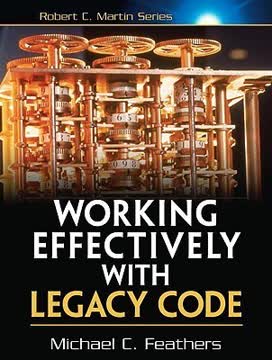
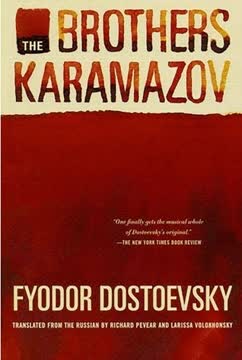
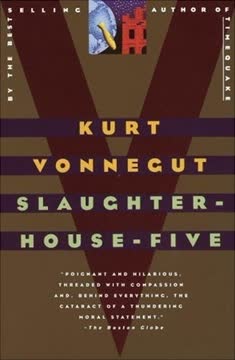
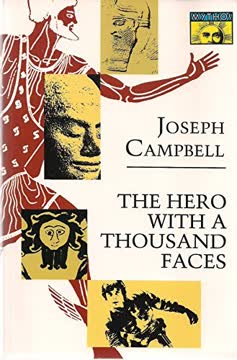
Download PDF
Download EPUB
.epub
digital book format is ideal for reading ebooks on phones, tablets, and e-readers.