Key Takeaways
1. Master fundamental data structures and algorithms for technical interviews
The programming questions are generally difficult. If everyone (or even most people) answered a particular question quickly, the company would stop asking it because it wouldn't tell them anything about the applicants.
Core competencies. Technical interviews focus on assessing candidates' understanding of fundamental data structures and algorithms. Common topics include linked lists, trees, graphs, sorting algorithms, and search techniques. Interviewers use these problems to evaluate problem-solving skills, coding ability, and knowledge depth.
Problem types. Expect questions on implementing data structures from scratch, manipulating existing structures, and applying algorithms to solve specific problems. Examples include reversing a linked list, finding the lowest common ancestor in a binary tree, or implementing depth-first search on a graph.
Preparation strategy.
- Review core data structures: arrays, linked lists, stacks, queues, trees, and graphs
- Practice common algorithms: sorting, searching, traversal, and recursion
- Implement data structures and algorithms from scratch to solidify understanding
- Solve practice problems focusing on time and space complexity optimization
2. Approach programming problems methodically and communicate your thought process
Interactivity is key. The code you write in the interview is probably the only example of your code that your interviewer sees. If you write ugly code, your interviewer will assume you always write ugly code.
Structured approach. Develop a systematic method for tackling interview problems. Start by clarifying the problem statement and requirements. Break down the problem into smaller components and consider edge cases. Discuss potential solutions and their trade-offs before diving into coding.
Clear communication. Articulate your thought process throughout the interview. Explain your reasoning, assumptions, and decision-making as you work through the problem. This demonstrates your problem-solving skills and allows the interviewer to provide guidance if needed.
Problem-solving steps:
- Understand the problem thoroughly
- Clarify any ambiguities or constraints
- Consider multiple approaches and discuss trade-offs
- Choose an approach and outline the solution
- Implement the solution, explaining your code as you write
- Test your solution with example inputs and edge cases
- Analyze the time and space complexity of your solution
3. Implement and manipulate linked lists efficiently
Deletion and insertion require a pointer or reference to the element immediately preceding the deletion or insertion location.
Fundamental operations. Linked lists are a common focus in technical interviews due to their simplicity and ability to test pointer manipulation skills. Master basic operations such as insertion, deletion, and traversal for both singly and doubly linked lists.
Common challenges. Be prepared to handle edge cases like empty lists, single-element lists, and operations at the head or tail of the list. Practice implementing more complex operations such as reversing a linked list, detecting cycles, or finding the nth-to-last element.
Key techniques:
- Use dummy nodes to simplify head and tail operations
- Implement the runner technique (slow and fast pointers) for cycle detection and finding middle elements
- Master recursive approaches for problems like reversing a linked list or merging sorted lists
- Understand when to use singly vs. doubly linked lists based on problem requirements
4. Understand tree structures and traversal algorithms
Many tree operations can be implemented recursively. The recursive implementation may not be the most efficient, but it's usually the best place to start.
Tree fundamentals. Familiarize yourself with different types of trees, including binary trees, binary search trees (BSTs), and heaps. Understand the properties and advantages of each structure. Master tree traversal algorithms: in-order, pre-order, and post-order.
Common problems. Practice solving typical tree-related problems such as finding the height of a tree, checking if a tree is balanced, and implementing level-order traversal. Be prepared to work with BSTs for searching, insertion, and deletion operations.
Key concepts:
- Recursive vs. iterative implementations of tree traversals
- Balanced vs. unbalanced trees and their impact on performance
- Tree rotations for maintaining balance in BSTs
- Heap operations: insertion, deletion, and heapify
- Trie data structure for efficient string operations
5. Apply graph concepts to solve complex problems
Graphs are commonly used to model real-world problems that are difficult to model with other data structures.
Graph representations. Understand different ways to represent graphs, including adjacency lists and adjacency matrices. Know when to use each representation based on the problem requirements and graph characteristics (sparse vs. dense).
Graph algorithms. Master fundamental graph algorithms such as depth-first search (DFS), breadth-first search (BFS), and shortest path algorithms (Dijkstra's, Bellman-Ford). Be prepared to apply these algorithms to solve problems like finding connected components, detecting cycles, or topological sorting.
Graph problem-solving strategies:
- Identify whether the problem requires a directed or undirected graph
- Consider weighted vs. unweighted graphs for distance or cost-related problems
- Use DFS for problems involving path finding or cycle detection
- Apply BFS for shortest path problems in unweighted graphs
- Implement union-find data structure for disjoint set problems
6. Optimize solutions using Big-O analysis and space-time trade-offs
Although this process involves two passes through the list, it's still O(n). It requires only a few variables' worth of storage, so this method is a significant improvement over the previous attempt.
Runtime analysis. Develop proficiency in analyzing the time and space complexity of your solutions using Big-O notation. Understand the implications of different complexity classes (e.g., O(1), O(log n), O(n), O(n log n), O(n^2)) on algorithm performance.
Optimization techniques. Learn to identify and apply common optimization strategies such as using appropriate data structures, eliminating unnecessary work, and leveraging problem-specific properties. Be prepared to discuss trade-offs between time and space complexity.
Key optimization approaches:
- Use hash tables for O(1) average-case lookup, insertion, and deletion
- Apply binary search on sorted data for O(log n) search time
- Implement dynamic programming to avoid redundant computations
- Utilize two-pointer techniques for array and string problems
- Consider amortized analysis for data structures like dynamic arrays
7. Practice recursion and iterative approaches for tree and graph problems
Sometimes recursive algorithms can be replaced with iterative algorithms that accomplish the same task in a fundamentally different manner using different data structures.
Recursive solutions. Develop a strong understanding of recursive problem-solving, particularly for tree and graph traversals. Practice identifying base cases and recursive steps. Be aware of the potential drawbacks of recursion, such as stack overflow for deep recursion.
Iterative alternatives. Learn to convert recursive solutions to iterative ones using explicit stack data structures. This skill is valuable for optimizing space complexity and handling large inputs that might cause stack overflow in recursive implementations.
Balancing approaches:
- Use recursion for its simplicity and elegance in initial problem-solving
- Convert to iterative solutions when dealing with large inputs or limited stack space
- Implement tail recursion optimization when applicable
- Understand the trade-offs between recursive and iterative solutions in terms of readability, maintainability, and performance
- Practice both approaches to develop flexibility in problem-solving
Last updated:
FAQ
1. What is Programming Interviews Exposed: Secrets to Landing Your Next Job by John Mongan about?
- Comprehensive interview guide: The book is a step-by-step manual for preparing for technical programming interviews, focusing on real-world problems and the entire hiring process.
- Emphasis on problem-solving: It teaches readers how to approach, analyze, and solve programming problems, rather than just memorizing answers.
- Covers full interview journey: From self-assessment and market research to technical questions, nontechnical questions, and offer negotiation, the book provides a holistic view.
- Practical coding focus: Examples use C, C++, and Java, but the emphasis is on data structures and algorithms, making it accessible to most programmers.
2. Why should I read Programming Interviews Exposed by John Mongan?
- Effective interview preparation: The book demystifies the unique challenges of programming interviews and equips readers with proven strategies and mindsets.
- Insight into interviewer expectations: It reveals what interviewers look for, including communication, problem-solving approach, and handling of tricky or ambiguous questions.
- Broad and deep coverage: Topics range from algorithms and data structures to concurrency, OOP, design patterns, databases, and even nontechnical questions.
- Ongoing learning support: The book encourages continuous practice and offers additional resources like a mailing list and app for further preparation.
3. What are the key takeaways from Programming Interviews Exposed by John Mongan?
- Problem-solving over memorization: Success comes from understanding problem types and developing a systematic approach, not just rote learning.
- Mastery of fundamentals: Deep knowledge of data structures, algorithms, and core programming concepts is essential for most interview questions.
- Communication is critical: Explaining your thought process clearly and methodically is as important as arriving at the correct answer.
- Nontechnical skills matter: Fit, experience, and the ability to answer behavioral questions can be decisive, even for technically strong candidates.
4. How does Programming Interviews Exposed by John Mongan recommend preparing before starting a job search?
- Know yourself: Assess your programming interests, strengths, and career goals to target roles that fit your passions and skills.
- Understand the market: Research current job trends, in-demand technologies, and outsourcing risks using social networks, job sites, and professional courses.
- Develop marketable skills: Build credentials through education, certifications, side projects, and internships to stand out to recruiters.
- Manage your online profile: Keep LinkedIn and other professional profiles up-to-date and positive, as recruiters often check these before interviews.
5. What strategies does Programming Interviews Exposed by John Mongan suggest for solving programming problems in interviews?
- Clarify and understand: Always ask questions to fully understand the problem and work through simple examples before coding.
- Choose the right tools: Analyze possible solutions, discuss trade-offs, and select the most efficient algorithms and data structures, explaining your choices.
- Communicate and iterate: Talk through your thought process, write clean code, test with examples, and handle edge cases, showing adaptability and openness to hints.
- Demonstrate critical thinking: Interviewers value candidates who can analyze performance, consider alternatives, and respond well to feedback.
6. How does Programming Interviews Exposed by John Mongan cover linked lists and their importance in interviews?
- Fundamental data structure: Linked lists test understanding of pointers, dynamic memory, and are especially relevant in C and C++ interviews.
- Varieties and operations: The book covers singly, doubly, and circular linked lists, focusing on traversal, insertion, deletion, and pointer management.
- Common interview problems: Examples include implementing stacks, deleting nodes, finding mth-to-last elements, and detecting cycles, with detailed solutions.
- Emphasis on edge cases: Mastery of linked list operations and special cases is essential for success in technical interviews.
7. What does Programming Interviews Exposed by John Mongan teach about trees and graphs for interviews?
- Tree fundamentals: The book explains hierarchical structures, especially binary trees and binary search trees, and covers recursive algorithms for traversals and searches.
- Graph complexity: It introduces graphs as generalizations of trees, discussing representations, BFS/DFS searches, and practical problems like the "Six Degrees of Kevin Bacon."
- Algorithmic focus: Emphasis is placed on recursive and iterative solutions, runtime analysis, and handling cycles in graphs.
- Interview relevance: Understanding these concepts is crucial for tackling complex data structure problems in interviews.
8. How are arrays and strings addressed in Programming Interviews Exposed by John Mongan?
- Array properties: The book discusses O(1) access, O(n) insertion/deletion, static vs. dynamic arrays, and language-specific behaviors.
- String handling: It covers mutability, encoding (ASCII/Unicode), and efficient manipulation techniques, highlighting differences across languages.
- Common pitfalls: Issues like bounds checking, memory management, and off-by-one errors are explained with practical advice.
- Problem examples: Classic interview problems such as finding the first nonrepeated character, reversing words, and integer-string conversions are included.
9. What guidance does Programming Interviews Exposed by John Mongan provide on recursion and its use in interviews?
- Recursion basics: The book defines recursion, explains base and recursive cases, and uses factorial as a simple example.
- Recursive vs. iterative: While recursion can be elegant, iterative solutions are often more efficient; the book advises starting with recursion and considering alternatives.
- Practical applications: It covers recursive algorithms for binary search, permutations, combinations, and telephone words, emphasizing clear understanding and implementation.
- Avoiding pitfalls: The importance of reaching base cases and preventing infinite recursion is stressed.
10. Which sorting algorithms and concepts are explained in Programming Interviews Exposed by John Mongan?
- Classic algorithms: Selection sort, insertion sort, quicksort, and merge sort are explained with their mechanisms, time complexities, and trade-offs.
- Advanced topics: The book covers multi-key sorting, stable vs. unstable sorts, and optimized quicksort implementations for edge cases.
- Algorithm selection: Guidance is given on choosing the right sort based on data size, memory, order, and stability requirements.
- Special problems: Pancake sorting and merging sorted lists are discussed to illustrate algorithmic thinking beyond standard sorts.
11. How does Programming Interviews Exposed by John Mongan address concurrency, object-oriented programming, and design patterns?
- Concurrency fundamentals: The book explains threads, synchronization (monitors, semaphores), deadlocks, and classic problems like Dining Philosophers, with practical code examples.
- OOP principles: It reviews classes, encapsulation, inheritance, polymorphism, interfaces vs. abstract classes, and virtual methods, clarifying their roles in interviews.
- Design patterns: Creational (Singleton, Builder, Factory), behavioral (Iterator, Observer), and structural (Decorator) patterns are summarized, with advice on when and why to use them.
- Practical relevance: Understanding these concepts helps candidates discuss architecture and design decisions confidently with interviewers.
12. What nontechnical, database, and bit manipulation topics are covered in Programming Interviews Exposed by John Mongan?
- Nontechnical questions: The book stresses the importance of experience, fit, goals, and salary discussions, offering strategies for positive, specific answers.
- Database fundamentals: It covers relational concepts, SQL commands (SELECT, JOIN, GROUP BY), transactions (ACID), and tricky SQL problems.
- Bit manipulation: Binary notation, bitwise operators, shift operations, and classic problems like counting 1 bits and determining endianness are explained with efficient algorithms.
- Résumé and profile tips: Guidance is provided on writing effective technical résumés and managing your online presence for job search success.
Review Summary
Programming Interviews Exposed is highly recommended for job-seeking programmers and recent graduates. Readers praise its comprehensive coverage of data structures, algorithms, and interview preparation. The book offers detailed explanations and step-by-step problem-solving approaches, making it valuable for technical interview preparation. While some find it outdated or lacking in certain areas, many credit it for helping them land jobs at top tech companies. The book is particularly useful for reviewing fundamental concepts and developing problem-solving skills. Some readers suggest supplementing it with other resources for a more complete interview preparation.
Similar Books
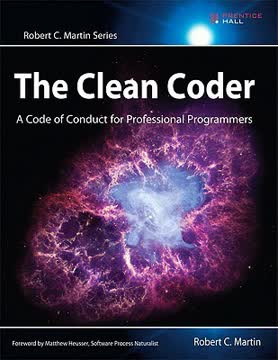
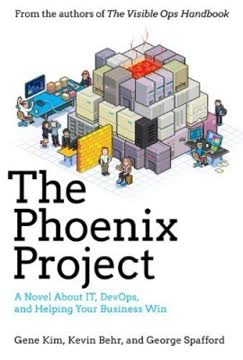
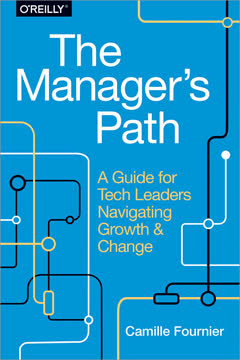
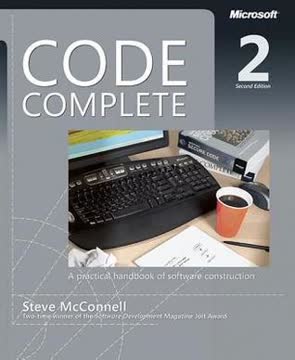
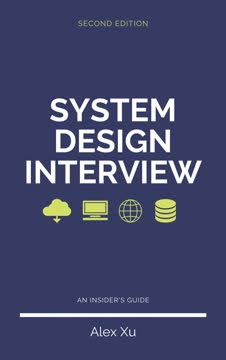
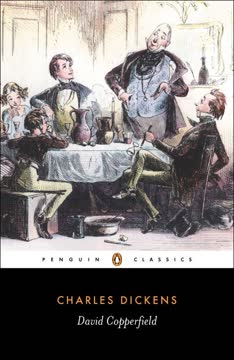
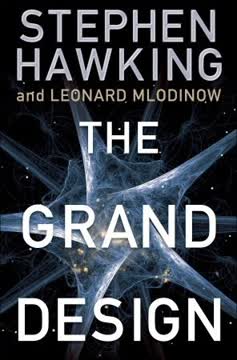
Download PDF
Download EPUB
.epub
digital book format is ideal for reading ebooks on phones, tablets, and e-readers.