Key Takeaways
1. Unit testing is essential for code quality and developer confidence
A unit test is an automated piece of code that invokes the unit of work being tested, and then checks some assumptions about a single end result of that unit.
Definition of unit testing. Unit testing involves writing automated code to verify the behavior of small, isolated pieces of functionality within a software system. It helps developers catch bugs early, refactor with confidence, and document expected behavior. By focusing on testing individual units of work, developers can ensure that each component functions correctly before integration.
Benefits of unit testing:
- Improves code quality by catching bugs early
- Enables safer refactoring and code changes
- Serves as living documentation for code behavior
- Increases developer confidence in the codebase
2. NUnit provides a robust framework for writing and running unit tests
NUnit uses an attribute scheme to recognize and load tests.
Key features of NUnit. NUnit is a popular testing framework for .NET that simplifies the process of writing and running unit tests. It provides attributes to mark test methods and classes, assertion methods to verify expected outcomes, and a test runner to execute tests and report results.
Core NUnit concepts:
- [Test] attribute for marking test methods
- [TestFixture] attribute for test classes
- Assert class for verifying test outcomes
- Test runners for executing tests (GUI, console, or IDE integration)
3. Stubs and mocks are crucial for isolating dependencies in tests
A stub is a controllable replacement for an existing dependency (or collaborator) in the system.
Understanding test doubles. Stubs and mocks are types of test doubles used to isolate the code under test from its dependencies. Stubs provide predetermined responses to method calls, while mocks allow verification of interactions between objects.
Key differences:
- Stubs: Return fixed values, don't fail tests
- Mocks: Verify method calls, can cause test failures
- Use stubs for state testing, mocks for interaction testing
- Limit to one mock per test to maintain focus
4. Isolation frameworks simplify the creation of test doubles
An isolation framework is a set of programmable APIs that makes creating fake objects much simpler, faster, and shorter than hand-coding them.
Benefits of isolation frameworks. These tools automate the creation of stubs and mocks, reducing the need for manual implementation. They offer powerful APIs for configuring behavior and verifying interactions, making tests more concise and easier to maintain.
Popular isolation frameworks:
- Moq: Open-source, lambda-based API
- NSubstitute: Simple, readable syntax
- Typemock Isolator: Commercial, supports legacy code
- FakeItEasy: Intuitive API, good for beginners
5. Test organization and hierarchy are key to maintainable test suites
Mapping tests to projects, classes, and methods is crucial for maintainability and readability.
Structuring test projects. Organize tests to mirror the structure of the production code, making it easy to locate and manage related tests. Use clear naming conventions and create a hierarchy that reflects the system under test.
Test organization best practices:
- One test project per production project
- One test class per production class
- Test method names describe the scenario and expected outcome
- Group related tests using test categories or nested classes
6. Good unit tests are trustworthy, maintainable, and readable
Without readability the tests you write are almost meaningless.
Characteristics of quality tests. Effective unit tests should be reliable, easy to update, and comprehensible to other developers. Focus on writing tests that clearly communicate their purpose and expectations.
Guidelines for better tests:
- Use descriptive test names (method_scenario_expectedOutcome)
- Follow the Arrange-Act-Assert pattern
- Avoid logic and multiple assertions in tests
- Keep tests isolated and independent
- Use meaningful variable names and clear assertions
7. Implementing unit testing in an organization requires careful strategy
There are two main ways an organization or team can start changing a process: bottom up or top down (and sometimes both).
Approaches to adoption. Introducing unit testing to an organization can be challenging. It requires a combination of technical skills, cultural change, and management support. Choose an approach that fits your organization's culture and constraints.
Implementation strategies:
- Start with a pilot project or team
- Provide training and resources
- Set clear goals and metrics
- Address common objections and concerns
- Celebrate successes and learn from failures
8. Legacy code can be made testable with the right approach and tools
Designing for testability becomes a way of thinking.
Tackling untested code. Legacy code often lacks tests and can be difficult to modify. By using specific techniques and tools, developers can gradually introduce tests and improve the code's testability.
Strategies for legacy code:
- Use characterization tests to document existing behavior
- Apply the "Sprout Method" technique to add new, tested functionality
- Leverage unconstrained mocking frameworks for hard-to-isolate dependencies
- Refactor gradually to improve testability
- Prioritize high-risk or frequently changing areas
9. Designing for testability enhances overall code quality
Testable designs usually only matter in static languages, such as C# or VB.NET, where testability depends on proactive design choices that allow things to be replaced.
Benefits of testable design. Designing code with testability in mind often leads to better overall architecture. It promotes loose coupling, clear interfaces, and separation of concerns, which are valuable beyond just testing.
Testability design principles:
- Dependency injection for easier mocking
- Interface-based design for flexibility
- Single Responsibility Principle for focused classes
- Avoid static methods and global state
- Use factory methods or abstract factories for object creation
Last updated:
FAQ
What's The Art of Unit Testing about?
- Focus on Unit Testing: The Art of Unit Testing by Roy Osherove is a comprehensive guide to unit testing, particularly in .NET and C#. It covers the basics, advanced techniques, and best practices for writing and maintaining unit tests.
- Test-Driven Development (TDD): The book emphasizes TDD, explaining how writing tests first can lead to better design and more reliable code. It includes personal experiences and lessons from the author.
- Practical Examples: It provides practical examples and code snippets, making it easier for readers to understand and apply the concepts discussed, equipping developers with the skills needed to write effective unit tests.
Why should I read The Art of Unit Testing?
- Improve Code Quality: The book teaches how to write effective unit tests that catch bugs early and ensure code behaves as expected, enhancing overall code quality.
- Learn Best Practices: It shares best practices for writing, organizing, and maintaining unit tests, applicable across various programming languages and frameworks.
- Real-World Insights: Filled with real-world insights and anecdotes, the book provides valuable lessons to help avoid common pitfalls in unit testing.
What are the key takeaways of The Art of Unit Testing?
- Unit Testing Fundamentals: Understanding what constitutes a good unit test is crucial. The book defines a unit test as "an automated piece of code that invokes the unit of work being tested, and then checks some assumptions about a single end result of that unit."
- Isolation Techniques: It discusses techniques for isolating code under test from its dependencies, such as using stubs and mocks, ensuring tests are reliable and maintainable.
- Integration into Development: Emphasizes integrating unit testing into the development process and organizational culture, providing strategies for overcoming resistance within teams.
How does The Art of Unit Testing define a unit test?
- Definition of a Unit Test: A unit test is "a piece of code that invokes a unit of work and checks one specific end result of that unit of work," focusing on specific outcomes rather than general behavior.
- Unit of Work: The unit of work can range from a single method to multiple classes, allowing for comprehensive testing of complex interactions.
- Failing Tests: If assumptions about the end result are incorrect, the unit test fails, providing a clear pass/fail criterion essential for effective testing.
What is the importance of writing good unit tests according to The Art of Unit Testing?
- Automated and Repeatable: Good unit tests should be automated and repeatable, allowing frequent runs without manual intervention, ensuring code changes don't introduce new bugs.
- Easy to Implement: The book stresses that unit tests should be easy to implement, encouraging developers to write tests alongside their code, reducing the likelihood of neglecting testing.
- Consistent Results: A good unit test should consistently return the same results if the code under test hasn’t changed, building trust in the testing process.
What are the core techniques for writing unit tests discussed in The Art of Unit Testing?
- Using Stubs and Mocks: Introduces stubs and mocks as essential tools for isolating code under test from dependencies, with stubs simulating behavior and mocks verifying interactions.
- Dependency Injection: Discusses methods of dependency injection, like constructor and property injection, to facilitate testing and control dependencies' behavior during tests.
- Refactoring for Testability: Emphasizes refactoring code to make it more testable, including creating interfaces and using design patterns that support easier testing.
How does The Art of Unit Testing suggest integrating unit testing into an organization?
- Be an Agent of Change: Encourages being an agent of change to promote unit testing, addressing concerns and resistance from team members.
- Visible Progress: Suggests tracking metrics and sharing successes to demonstrate the value of unit testing, making progress visible to gain support.
- Specific Goals: Recommends setting specific goals for unit testing adoption to guide the process and measure success, leading to more effective integration.
What is the "safe green zone" mentioned in The Art of Unit Testing?
- Defined Area for Unit Tests: The "safe green zone" is a designated area in the codebase where only unit tests reside, ensuring they run quickly and reliably.
- Encourages Frequent Testing: Isolating unit tests encourages frequent runs, leading to quicker feedback on code changes and maintaining high confidence in the codebase.
- Avoids False Positives: Minimizes the risk of false positives from integration tests, ensuring test failures indicate real issues needing attention.
How does The Art of Unit Testing recommend handling legacy code?
- Prioritize Components: Recommends creating a test-feasibility table to prioritize components to test based on complexity and dependency levels, focusing efforts where most impactful.
- Use Integration Tests First: Advises writing integration tests before refactoring legacy code to establish a baseline of functionality, ensuring changes don't break existing behavior.
- Leverage Isolation Frameworks: Discusses using isolation frameworks like Typemock Isolator to test legacy code without extensive refactoring, allowing gradual design improvement.
What are the pillars of good unit tests according to The Art of Unit Testing?
- Trustworthiness: Tests should be reliable, consistently passing or failing based on code correctness, helping developers feel confident in their changes.
- Maintainability: Tests should be easy to maintain and update as code evolves, preventing developers from ignoring them and undermining the testing process.
- Readability: Tests should be easy to read and understand, with clear naming conventions and structured assertions contributing to readability.
What is the "template test class pattern" mentioned in The Art of Unit Testing?
- Abstract Test Class: Involves creating an abstract test class defining a set of tests to be implemented by derived classes, ensuring consistent application across similar classes.
- Encourages Consistency: Promotes consistency in testing practices by enforcing a standard set of tests for related classes, useful in large codebases.
- Facilitates Code Reuse: Allows for code reuse in test setups and assertions, reducing duplication and making tests easier to maintain.
How does The Art of Unit Testing recommend naming unit tests?
- Three-Part Naming Convention: Suggests a naming convention including the method being tested, the scenario, and the expected behavior, clarifying what the test verifies.
- Improves Readability: Consistent naming patterns help developers quickly understand a test's purpose without reading its implementation, enhancing test suite readability.
- Avoids Ambiguity: Clear, descriptive names prevent confusion about a test's purpose, reducing misinterpretation or miscommunication among team members.
Review Summary
The Art of Unit Testing receives generally positive reviews, with readers praising its comprehensive coverage of unit testing concepts and practices. Many find it helpful for beginners, while some experienced developers note its usefulness in refining their skills. The book's practical examples and clear explanations are appreciated. However, some criticize its heavy focus on C# and outdated information. Readers value the insights on test organization, mocking, and integrating testing into development processes. A few reviewers find the content repetitive or contradictory to other sources.
Similar Books
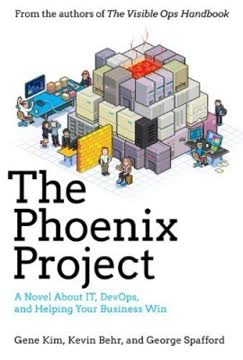
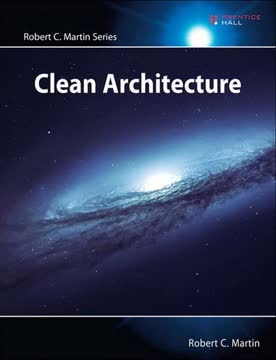
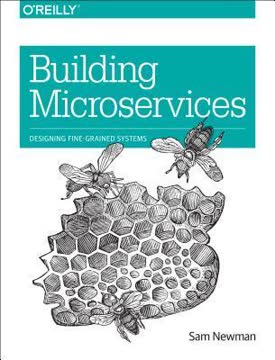
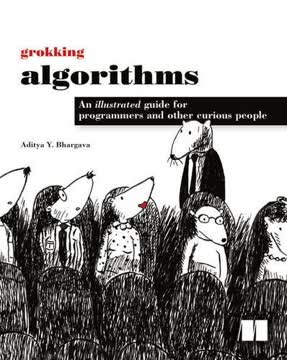
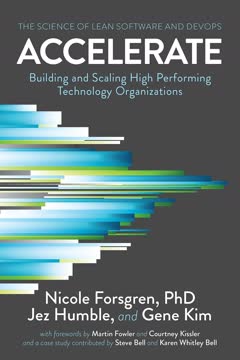
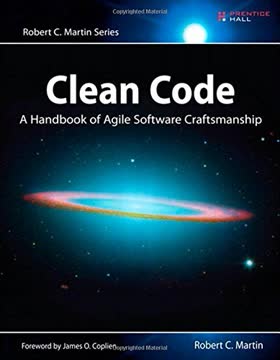
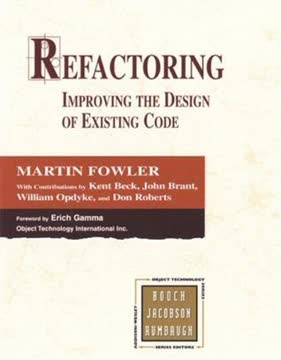
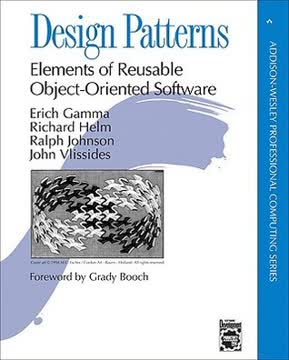
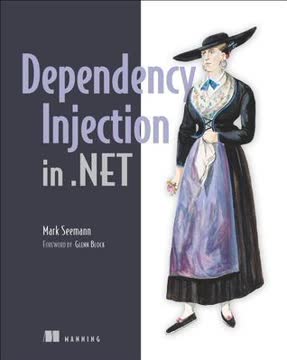
Download PDF
Download EPUB
.epub
digital book format is ideal for reading ebooks on phones, tablets, and e-readers.