Key Takeaways
1. C++: A Language for Elegant & Efficient Abstractions
C++ is a language for developing and using elegant and efficient abstractions.
Dual Nature. C++ is designed to bridge the gap between low-level hardware access and high-level problem domains. It inherits C's efficiency for systems programming while adding powerful abstraction mechanisms inspired by Simula. The goal is to provide a language that is both performant and expressive, allowing programmers to represent concepts directly in code.
- Direct hardware mapping
- Affordable abstraction mechanisms
- General-purpose language
- Bias towards systems programming
Zero Overhead. A core principle of C++ design is the "zero-overhead principle": you don't pay for what you don't use. Language features and fundamental abstractions are designed to be as efficient as hand-written code. This is crucial for performance-critical applications and infrastructure software.
- Efficiency is paramount
- No implicit costs for unused features
- Enables use in resource-constrained environments
Expressing Ideas. The language aims to help programmers express ideas clearly and directly in code. This involves representing concepts as types, relationships as hierarchies or parameterizations, and independent ideas independently. The synthesis of different programming styles is encouraged to achieve the best solutions.
- Express ideas directly
- Represent relationships
- Keep independent ideas separate
- Simple ideas simply
2. Modern C++ (C++11) is a Better Language
C++ feels like a new language.
Significant Evolution. The C++ language has undergone dramatic improvements over the years, most notably with the C++11 standard. These changes have made C++ a far more powerful and polished tool for writing quality software. Modern C++ enables clearer, simpler, and more direct expression of ideas.
- C++11 vs C++98
- Enhanced expressiveness
- Improved safety and performance
Key Features. C++11 introduced a wealth of features that fundamentally changed how C++ is written and used. These include better support for concurrency, resource management, and generic programming, as well as syntactic conveniences that reduce boilerplate.
- Concurrency support (
std::thread
,std::mutex
) - Move semantics (
std::move
, rvalue references) - Smart pointers (
unique_ptr
,shared_ptr
) - Lambdas
auto
andconstexpr
- Initializer lists
Adopting Modern Styles. Programmers are strongly encouraged to adopt modern C++ features and styles. Sticking to older C++98 or C styles will result in lower-quality, less maintainable, and potentially less performant code. The standard guarantees backward compatibility, but progress happens.
3. Classes: The Foundation of Abstraction
The central language feature of C++ is the class.
User-Defined Types. Classes are the primary mechanism for creating user-defined types that represent concepts from the problem domain directly in code. A well-chosen set of classes makes a program easier to understand, reason about, and modify.
- Represent concepts as types
- Foundation of abstraction mechanisms
- Improve code clarity and maintainability
Encapsulation. Classes allow distinguishing between the public interface (what users see and use) and the private implementation details (data members and helper functions). This enforces data hiding, guarantees consistent use of data, and allows changing the implementation without affecting user code.
- Public interface
- Private implementation
- Data hiding
- Separation of concerns
Constructors and Destructors. Constructors define how objects are initialized, ensuring they are in a valid state upon creation. Destructors define cleanup actions performed when an object is destroyed, crucial for releasing resources. This constructor/destructor pair is fundamental to resource management techniques like RAII.
- Guaranteed initialization
- Resource acquisition (constructors)
- Resource release (destructors)
- Class invariants
4. Resource Management: RAII and Smart Pointers
The constructor/destructor combination is the basis of many elegant techniques.
RAII Principle. Resource Acquisition Is Initialization (RAII) is a fundamental C++ technique where resource acquisition is tied to object initialization (constructors) and resource release to object destruction (destructors). This ensures resources are properly managed even when exceptions are thrown or functions are exited early.
- Resource acquisition in constructors
- Resource release in destructors
- Automatic cleanup on scope exit
- Exception safety
Avoiding Leaks. RAII helps prevent resource leaks (like memory leaks) and other resource management errors (premature deletion, double deletion). By encapsulating resource ownership within objects, the compiler handles cleanup automatically.
- Eliminates "naked"
new
anddelete
- Prevents memory leaks
- Handles files, locks, threads, etc.
Smart Pointers. unique_ptr
and shared_ptr
are standard library smart pointers that use RAII to manage dynamically allocated memory. unique_ptr
provides exclusive ownership, while shared_ptr
provides shared ownership via reference counting. They are preferred over raw pointers for managing heap-allocated objects.
unique_ptr
(exclusive ownership)shared_ptr
(shared ownership)- Automatic memory deallocation
- Avoids manual
delete
calls
5. Templates: Enabling Generic Programming
A template is a class or a function that we parameterize with a set of types or values.
Compile-Time Parameterization. Templates allow defining classes, functions, and type aliases that are parameterized by types, values, or other templates. This enables writing code that works with a variety of types without sacrificing performance.
- Parameterize by type or value
- Compile-time polymorphism
- Code generation
Generic Programming. Templates are the foundation of generic programming in C++, focusing on designing algorithms and data structures that work with any type meeting specific requirements (concepts). This allows writing highly reusable code that is both type-safe and efficient.
- Design general algorithms
- Work with various types
- Concepts (requirements on arguments)
Efficiency. Templates are a compile-time mechanism, meaning their use typically incurs no run-time overhead compared to equivalent hand-written code. This is achieved through techniques like inlining and compile-time computation.
- Zero run-time overhead
- Enables inlining
- Compile-time computation
6. The Standard Library: Your Essential Toolkit
No significant program is written in just a bare programming language.
Essential Components. The standard library provides a comprehensive set of fundamental components that are essential for almost any C++ program. These include containers, algorithms, I/O facilities, utilities, and support for concurrency.
- Containers (vector, list, map, set)
- Algorithms (sort, find, copy, unique)
- I/O Streams (cin, cout, cerr)
- Utilities (pair, tuple, smart pointers, time)
- Concurrency support
Foundation. The standard library is written in C++ itself, demonstrating the language's power and serving as a model for good C++ design. It acts as a common foundation for other libraries and applications, promoting portability and interoperability.
- Written in C++
- Model for good design
- Promotes portability
- Enables interoperability
Prefer Standard. Programmers are strongly encouraged to use standard library components whenever possible rather than reinventing them. Standard components are well-designed, optimized, widely available, and well-known, reducing maintenance costs and improving code quality.
- Don't reinvent the wheel
- Well-designed and optimized
- Widely available and known
7. Concurrency: Built-in Support for Parallelism
Concurrency – the execution of several tasks simultaneously – is widely used to improve throughput (by using several processors for a single computation) or to improve responsiveness (by allowing one part of a program to progress while another is waiting for a response).
Modern Feature. C++ includes robust, portable, and type-safe support for concurrent programming, addressing the needs of modern multi-core hardware. This is a significant addition in C++11.
- Built-in support
- Portable and type-safe
- Addresses multi-core processors
Threads and Tasks. The library provides low-level thread management (std::thread
) and higher-level task-based abstractions (std::future
, std::async
). Threads share an address space, requiring careful synchronization to avoid data races.
std::thread
for system threads- Shared address space
- Task-based concurrency (
std::async
)
Synchronization. Mechanisms like mutexes (std::mutex
) and condition variables (std::condition_variable
) are provided to manage shared data access and synchronize threads. Atomic operations offer fine-grained, lock-free synchronization for simple types.
- Mutexes and locks
- Condition variables
- Atomic operations
- Avoiding data races
8. Mastering Types and Basic Facilities
Every name and every expression has a type associated with it.
Fundamental Building Blocks. Understanding C++'s built-in types (int
, double
, char
, bool
), how to declare variables, the rules of scope, and basic control flow statements (if
, switch
, for
, while
) is foundational. These elements, inherited and enhanced from C, form the basis of all C++ programs.
- Built-in types
- Declarations and scope
- Control flow statements
- Expressions and operators
Type Safety. C++ is a statically typed language, meaning types are checked at compile time. This helps catch errors early. Features like auto
for type deduction and constexpr
for compile-time constants enhance type safety and expressiveness.
- Static type checking
- Catch errors early
auto
for type deductionconstexpr
for compile-time constants
Pointers and References. C++ provides pointers and references for indirect access to memory. While powerful, they require careful management, especially when dealing with resource ownership. Smart pointers are preferred over raw pointers for managing heap memory.
- Pointers and references
- Indirect memory access
- Smart pointers for ownership
9. Combining Programming Styles for Effective Solutions
The best (most maintainable, most readable, smallest, fastest, etc.) solution to most nontrivial problems tends to be one that combines aspects of these styles.
Synthesis, Not Exclusivity. C++ is designed to support multiple programming styles: procedural, data abstraction, object-oriented, and generic programming. The language features allow these styles to be used in combination, and the most effective solutions often blend techniques from several paradigms.
- Procedural programming
- Data abstraction
- Object-oriented programming
- Generic programming
Avoid Dogma. Focusing exclusively on a single style or viewing C++ as a "hybrid" language misses the power of its synthesis. The language provides tools that can be used elegantly in combination to support a wide variety of techniques.
- Language supports combinations
- Avoid single-paradigm thinking
Practical Design. Effective C++ programming involves choosing the right tools (language features, standard library components) from the available set and combining them appropriately for the problem at hand. This requires understanding the strengths and weaknesses of different approaches and how they interact.
- Choose the right tools
- Combine features effectively
- Focus on design and techniques
10. Error Handling: Exceptions and Guarantees
The notion of an exception is provided to help get information from the point where an error is detected to a point where it can be handled.
Separating Concerns. Exceptions (throw
, catch
) provide a mechanism to separate the code that detects an error from the code that handles it. This is particularly useful in large programs and libraries where the detector doesn't know how to recover, and the handler cannot easily detect the error.
throw
to report errorscatch
to handle errors- Decouples detection and handling
Exception Safety. The standard library provides guarantees about the state of objects when exceptions are thrown. The basic guarantee ensures invariants are maintained and no resources are leaked. The strong guarantee ensures operations either succeed or have no effect.
- Basic guarantee (no leaks, valid state)
- Strong guarantee (all or nothing)
noexcept
specifier
Integration with RAII. Exception handling is tightly integrated with RAII (Resource Acquisition Is Initialization). This ensures that resources acquired by objects are properly released during stack unwinding when an exception propagates, preventing leaks and simplifying cleanup code.
- RAII for cleanup
- Destructors called during stack unwinding
Last updated:
Review Summary
The C++ Programming Language is widely regarded as the definitive reference for C++, written by the language's creator. While praised for its comprehensive coverage and valuable insights, many reviewers note it's not suitable for beginners. The book is described as dense, sometimes verbose, and better used as a reference than a learning tool. Experienced programmers appreciate its depth and technical details. Some criticize the writing style and organization, while others consider it essential for mastering C++. Overall, it's seen as a crucial resource for professionals but challenging for newcomers.
Similar Books
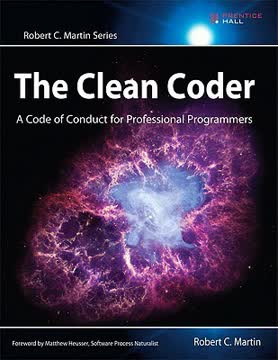
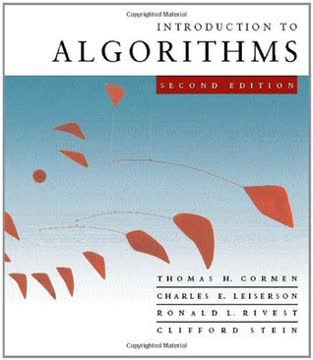
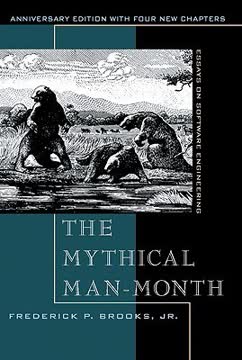
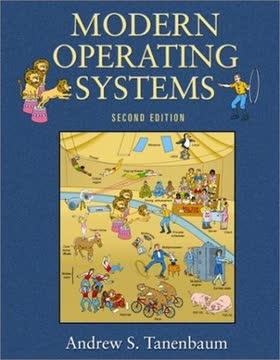
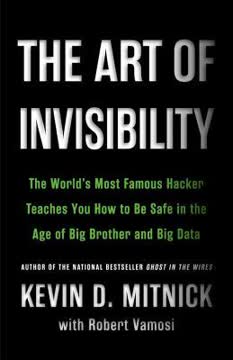
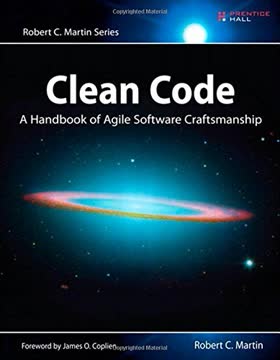
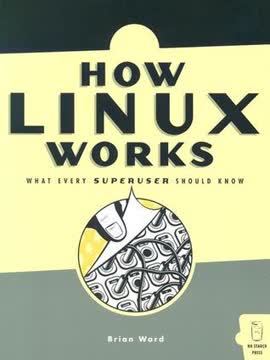
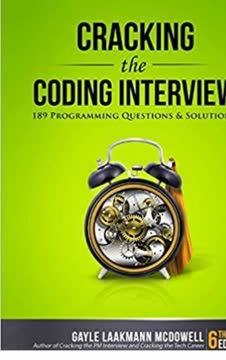
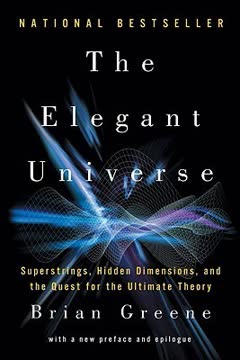
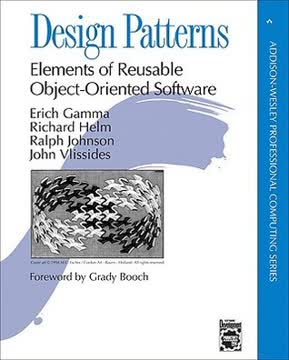
Download PDF
Download EPUB
.epub
digital book format is ideal for reading ebooks on phones, tablets, and e-readers.