Key Takeaways
1. React fundamentals: Components, JSX, and state management
Components are the foundation of every React application.
Building blocks of React. Components are the core building blocks of React applications, allowing developers to create reusable and modular UI elements. JSX, a syntax extension for JavaScript, enables the seamless integration of HTML-like code within JavaScript, making it easier to describe the structure of React components.
State and props. React's state management system, primarily through the useState hook, allows components to maintain and update their own data. Props, on the other hand, facilitate the passing of data and functions between components, enabling a unidirectional data flow. This combination of state and props forms the backbone of React's declarative programming model, where developers describe the desired UI state, and React efficiently updates the DOM to match.
2. Asynchronous data handling and side effects in React
React's useEffect Hook creates a memoized function every time its dependency array changes.
Managing side effects. The useEffect hook is crucial for handling side effects in React components, such as data fetching, subscriptions, or manually changing the DOM. It allows developers to perform these operations after the component has rendered, ensuring smooth user experiences and preventing unnecessary re-renders.
Asynchronous operations. When dealing with asynchronous operations like API calls, React provides patterns to manage loading states, error handling, and data updates. Techniques such as conditional rendering based on loading/error states and the use of async/await syntax within useEffect callbacks help create robust data fetching mechanisms.
- Key aspects of asynchronous data handling:
- Setting up loading and error states
- Using async/await for cleaner asynchronous code
- Implementing error boundaries for graceful error handling
- Cancelling ongoing requests when components unmount
3. Controlled components and forms in React
Forms aren't much different in React's JSX than in HTML.
Form management. React's approach to form handling revolves around the concept of controlled components. In this pattern, form elements like input fields, checkboxes, and select dropdowns are controlled by React state, allowing for more predictable and manageable form behavior.
Event handling. React provides a synthetic event system that wraps the browser's native event system, offering a consistent interface across different browsers. This system allows developers to easily attach event handlers to form elements and manage user interactions.
- Key aspects of form handling in React:
- Using the useState hook to manage form state
- Implementing onChange handlers to update state
- Preventing default form submission behavior
- Validating form inputs and displaying error messages
4. Performance optimization techniques for React applications
React's memo API checks whether the props of a component have changed.
Preventing unnecessary re-renders. React's default behavior of re-rendering child components when a parent component updates can sometimes lead to performance issues. The memo higher-order component and useMemo hook allow developers to optimize rendering by memoizing components and values, respectively.
Code splitting and lazy loading. As React applications grow in size, it becomes crucial to implement code splitting techniques. React.lazy and Suspense components enable developers to split their code into smaller chunks and load them on demand, significantly improving initial load times and overall application performance.
- Key performance optimization techniques:
- Using React.memo for function components
- Implementing useMemo for expensive computations
- Utilizing useCallback to memoize callback functions
- Employing React.lazy and Suspense for code splitting
- Implementing virtualization for long lists
5. Advanced React patterns: Custom hooks and memoization
Custom hooks encapsulate reusable logic in a way that's easy to share between components.
Creating custom hooks. Custom hooks allow developers to extract component logic into reusable functions, promoting code reuse and separation of concerns. They follow the same rules as React's built-in hooks and can utilize other hooks within their implementation.
Memoization techniques. Memoization is a powerful optimization technique in React, used to cache the results of expensive computations or to prevent unnecessary re-renders. The useMemo and useCallback hooks are primary tools for implementing memoization in functional components.
- Benefits of custom hooks and memoization:
- Improved code organization and reusability
- Enhanced performance through caching
- Better separation of concerns in components
- Easier testing of complex logic
6. Testing React applications: Unit, integration, and snapshot testing
React Testing Library adheres to a single core philosophy: instead of testing implementation details of React components, it tests how users interact with the application and if it works as expected.
Testing philosophy. The React community emphasizes testing user behavior rather than implementation details. This approach leads to more robust tests that are less likely to break due to internal refactoring.
Testing tools and techniques. React applications can be tested using a combination of unit tests for individual functions and components, integration tests for component interactions, and snapshot tests for catching unexpected UI changes. Popular testing libraries include Jest for the test runner and assertion library, and React Testing Library for rendering components and simulating user interactions.
- Key aspects of React testing:
- Writing unit tests for individual components and functions
- Creating integration tests to verify component interactions
- Using snapshot testing to catch unintended UI changes
- Mocking API calls and other external dependencies
- Implementing continuous integration for automated testing
7. Structuring and scaling React projects
There is no right way for folder/file structures.
Project organization. As React projects grow, proper organization becomes crucial for maintainability and scalability. While there's no one-size-fits-all approach, common patterns include organizing by feature, by type (components, hooks, utilities), or a combination of both.
Modular architecture. Implementing a modular architecture helps in managing complexity as the project scales. This involves creating reusable components, separating concerns, and establishing clear interfaces between different parts of the application.
- Best practices for structuring React projects:
- Implementing a consistent naming convention
- Creating a clear separation between presentational and container components
- Using index files for cleaner imports
- Implementing lazy loading for better performance
- Utilizing TypeScript for improved type safety and developer experience
Last updated:
Review Summary
The Road to React receives largely positive reviews, praised for its clarity, practical approach, and comprehensive coverage of React basics. Readers appreciate its step-by-step explanations, ES6 integration, and hands-on project. The book is recommended for beginners and those with some React experience. Some reviewers note its concise nature and lack of advanced topics. The author's writing style and additional resources are highly regarded. Most readers find it an excellent introduction to React, though a few desire more in-depth coverage of certain areas.
Similar Books
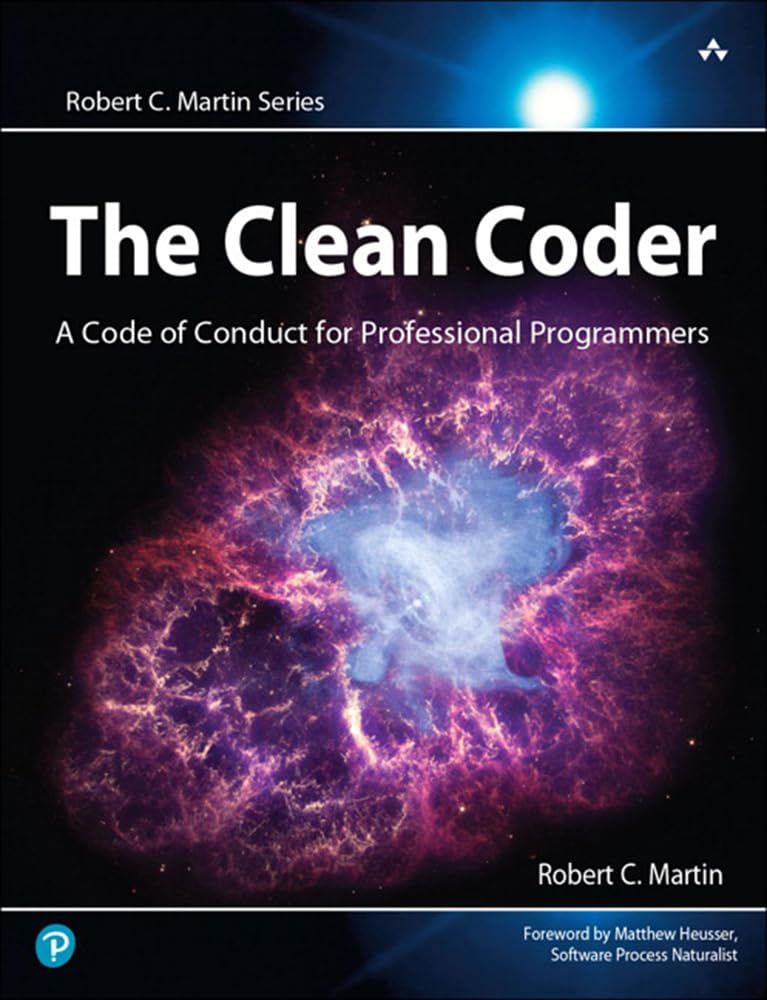
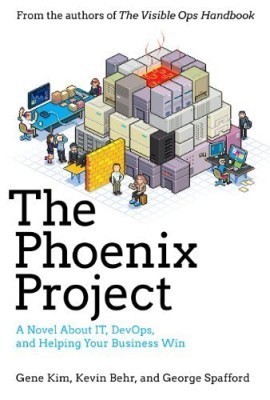
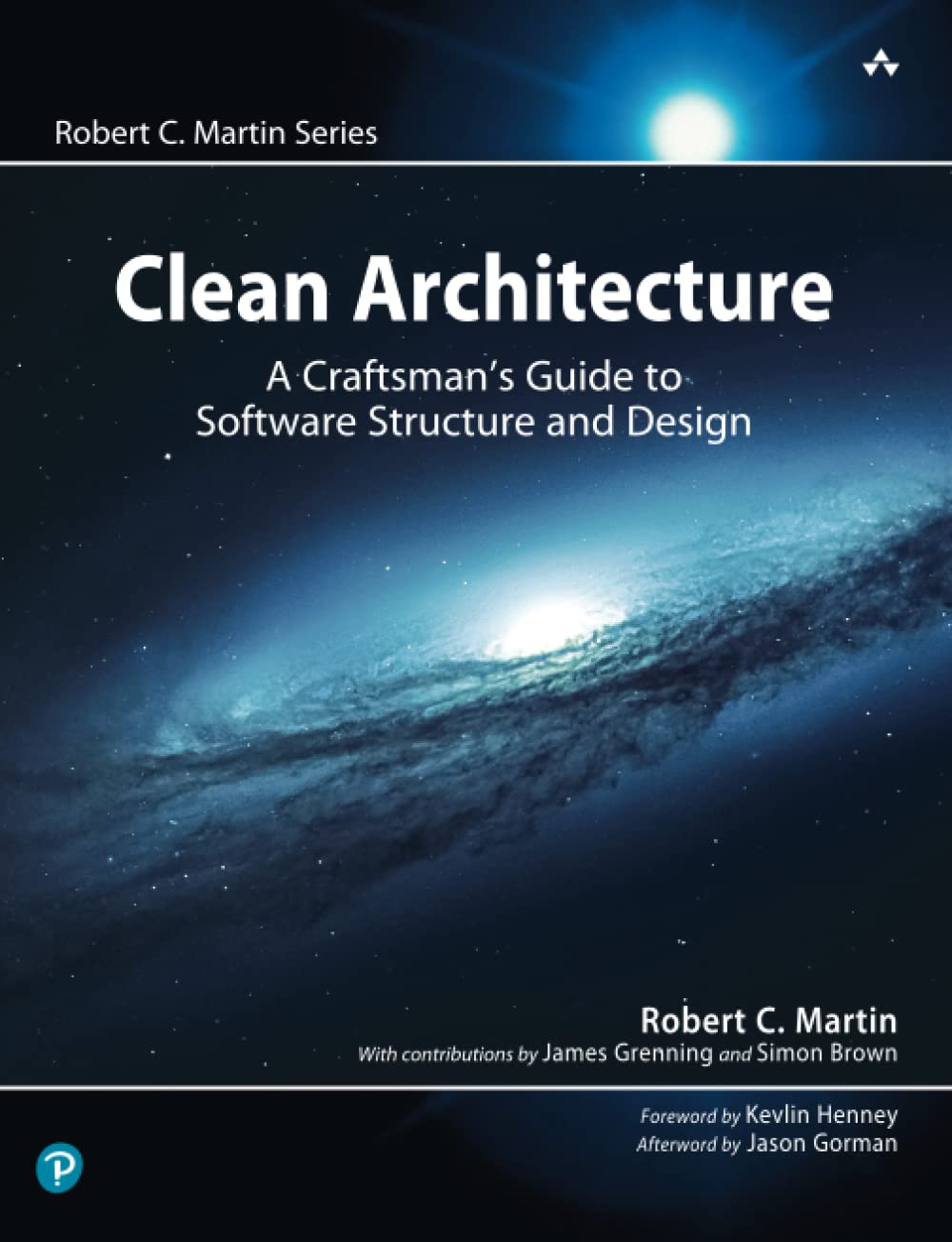
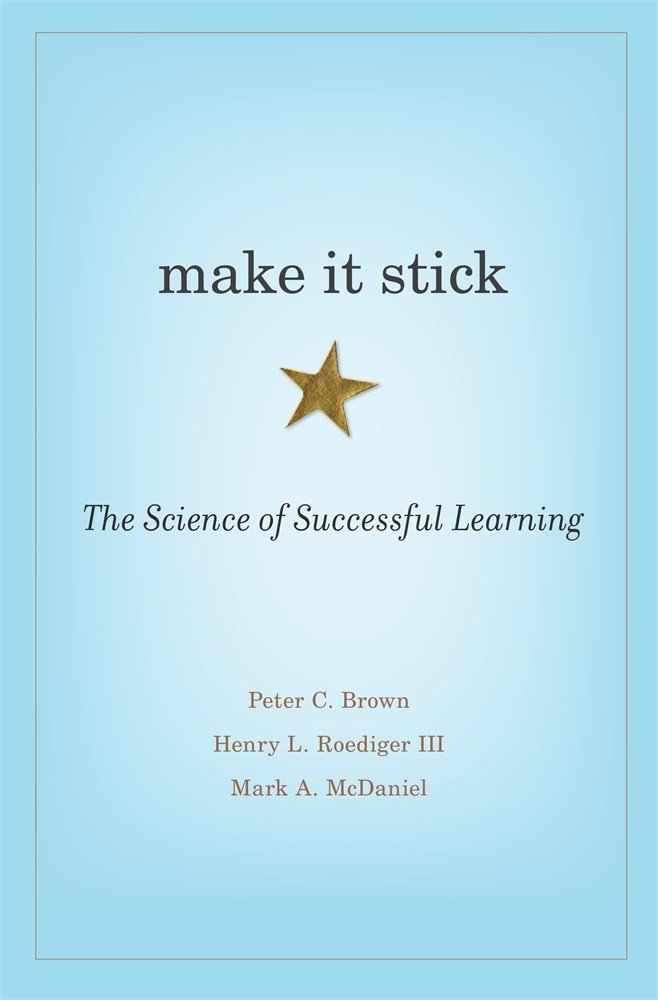
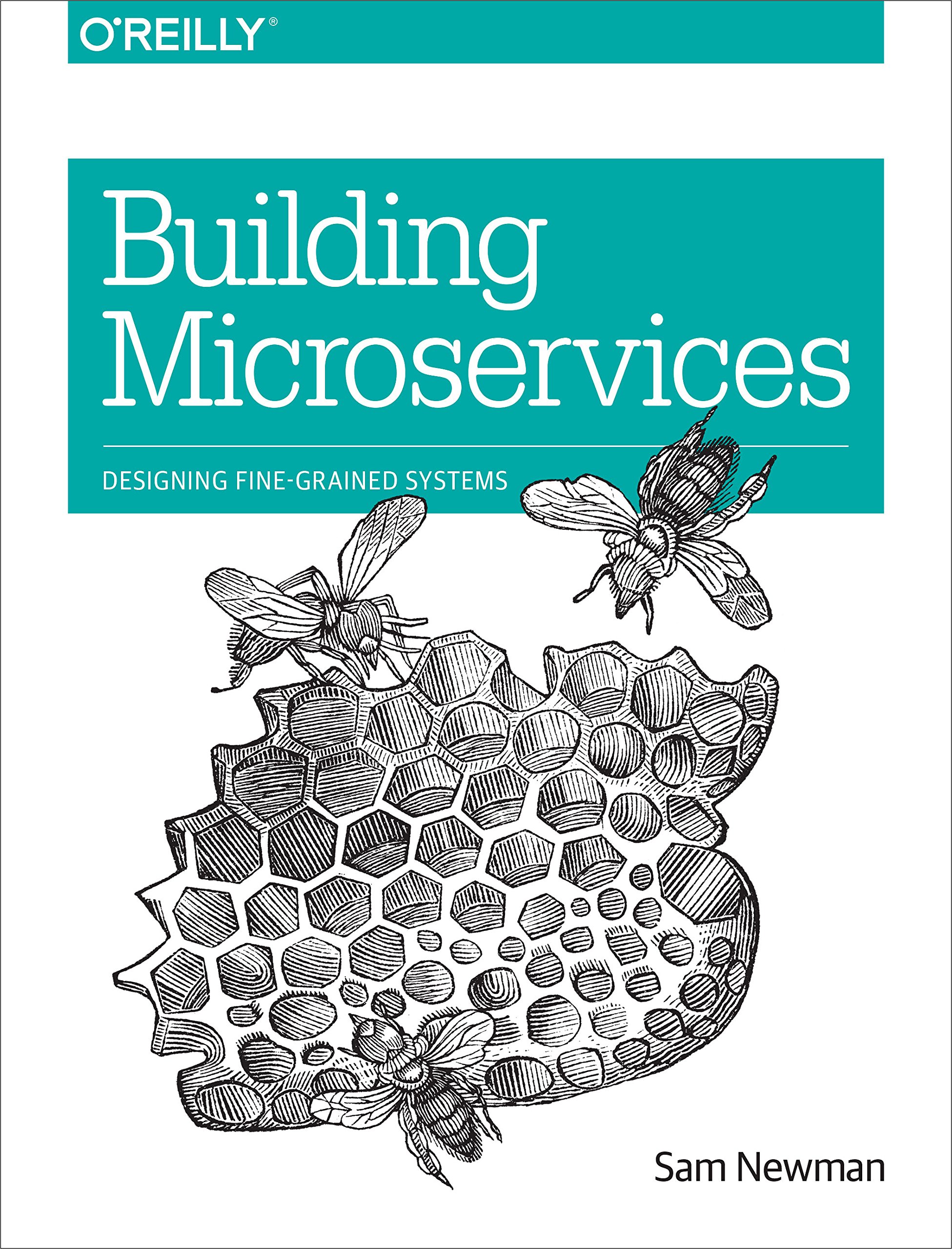
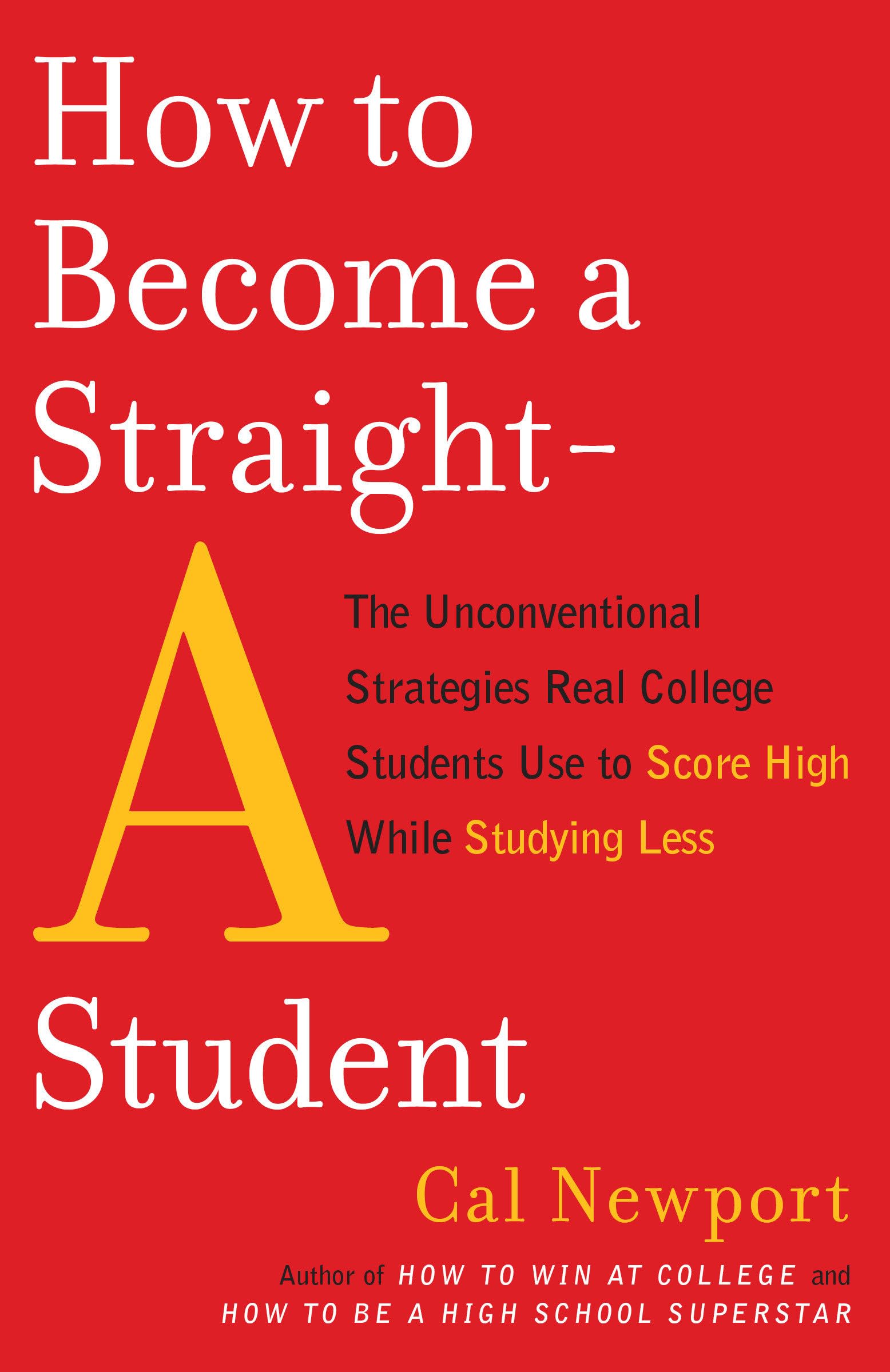
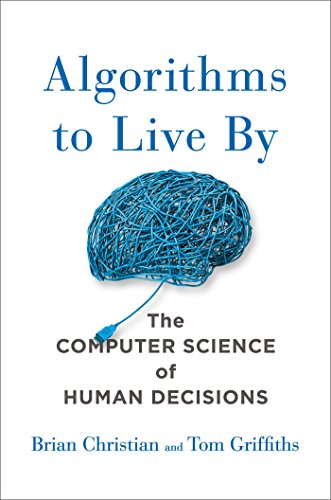
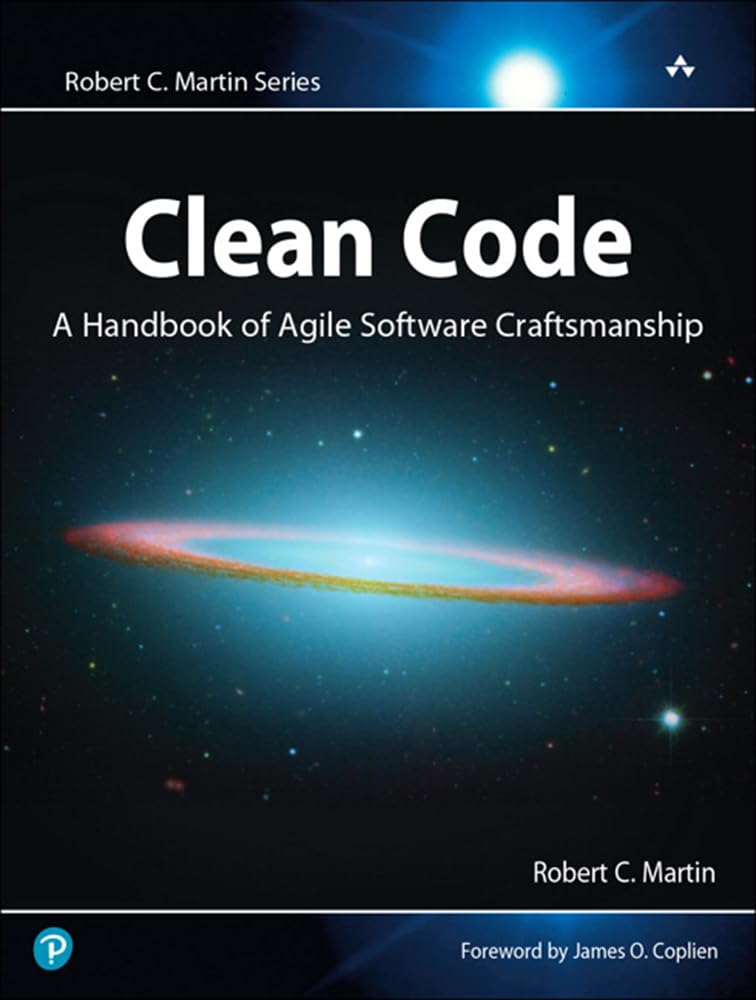
Download PDF
Download EPUB
.epub
digital book format is ideal for reading ebooks on phones, tablets, and e-readers.