Key Takeaways
1. Tidying First: A Strategic Approach to Software Design
"Software design is an exercise in human relationships."
Tidying first is a strategic approach to software design that focuses on making small, safe structural changes to code before implementing behavior changes. This approach aims to improve code readability, maintainability, and flexibility. Tidyings are mini-refactorings that can be applied quickly and easily, such as:
- Guard clauses
- Explaining variables and constants
- Extracting helper functions
- Normalizing symmetries
The goal of tidying first is to make future behavior changes easier and more efficient. By investing in code structure, developers create options for future changes and reduce the cost of software development over time.
2. The Economics of Software Design: Time Value and Optionality
"A dollar today is worth more than a dollar tomorrow, so earn sooner and spend later."
Time value of money and optionality are two key economic concepts that influence software design decisions. The time value of money suggests that earning revenue sooner and delaying expenses is generally preferable. However, optionality emphasizes the value of creating future possibilities.
In software design, this translates to:
- Balancing immediate behavior changes (revenue) with structural improvements (investment)
- Creating options for future changes by improving code structure
- Recognizing that uncertainty and volatility can increase the value of options
Understanding these economic principles helps developers make informed decisions about when and how much to invest in software design.
3. Coupling and Cohesion: Fundamental Principles of Software Design
"cost(software) ~= coupling"
Coupling and cohesion are fundamental principles that drive the cost and quality of software. Coupling refers to the interdependence between different elements of a system, while cohesion describes how well the elements within a module work together.
Key points:
- High coupling increases the cost of changes and maintenance
- Low cohesion makes code harder to understand and modify
- Reducing coupling and increasing cohesion are primary goals of software design
- Decoupling isn't free and involves trade-offs
Effective software design seeks to minimize coupling between modules while maximizing cohesion within modules. This approach leads to more flexible, maintainable, and less expensive software systems.
4. Reversible Structure Changes: The Power of Flexible Design
"What's the difference between a bad haircut and a bad tattoo? The bad haircut grows out, but the bad tattoo is forever."
Reversible structure changes are a key advantage of software design compared to many other engineering disciplines. Most software design decisions can be easily undone, which allows for more experimentation and iteration.
Benefits of reversible changes:
- Reduced risk in making design decisions
- Encourages experimentation and learning
- Allows for incremental improvements
When making design decisions, consider:
- Is this change easily reversible?
- If not, can we make it reversible through feature flags or other techniques?
- How can we minimize the impact of potentially irreversible changes?
By focusing on reversible changes, developers can make bolder design decisions and adapt more quickly to changing requirements.
5. Balancing Tidying and Behavior Changes
"Tidy first? Likely yes. Just enough. You're worth it."
Balancing tidying and behavior changes is crucial for effective software development. While tidying improves code structure and creates options for future changes, it's important not to get carried away and delay delivering value through behavior changes.
Guidelines for balancing:
- Tidy first when it will make the immediate behavior change easier or cheaper
- Tidy after when you've gained new insights from implementing a behavior change
- Tidy later when you have a backlog of improvements that don't directly impact current work
- Consider the economic trade-offs between immediate behavior changes and long-term structural improvements
Remember that the ultimate goal is to deliver value through working software. Tidying is a means to that end, not an end in itself.
6. Managing Tidying: Rhythm, Batch Sizes, and Chaining
"Tidyings are like potato chips. You eat one, and you'll want another."
Managing tidying effectively involves finding the right rhythm, batch sizes, and chaining of tidyings. This ensures that tidying remains productive and doesn't interfere with delivering value.
Key aspects of managing tidying:
- Rhythm: Aim for short bursts of tidying (minutes to hours) interspersed with behavior changes
- Batch sizes: Keep tidying batches small to reduce risk and improve review processes
- Chaining: Be aware of how one tidying can lead to another, but resist the urge to go down rabbit holes
Best practices:
- Separate tidying commits from behavior change commits
- Consider not requiring reviews for small, low-risk tidyings
- Be prepared to "get untangled" if tidying and behavior changes become mixed
Effective management of tidying helps maintain a healthy balance between improving code structure and delivering new features.
7. Software Design as an Exercise in Human Relationships
"Software design is preparation for change; change of behavior."
Software design as a human activity emphasizes the importance of relationships and communication in the development process. This perspective shifts the focus from purely technical considerations to the human aspects of software development.
Key relationships in software design:
- Individual developers with their code
- Developers within a team
- Development teams with business stakeholders
Implications:
- Design decisions affect how easily others can understand and modify the code
- Effective communication is crucial for making and explaining design choices
- Empathy and consideration for future developers (including your future self) should guide design decisions
By viewing software design through the lens of human relationships, developers can create more maintainable, collaborative, and valuable software systems.
Last updated:
FAQ
What's "Tidy First?" about?
- Focus on Design: "Tidy First?" by Kent Beck emphasizes the importance of software design over tools and technology, advocating for a focus on creating clean, readable code.
- Practical Tips: The book provides practical tips for developers at any level to improve their codebases, making them easier to understand and modify.
- Incremental Improvement: It introduces a realistic approach to incrementally improving production code, moving away from traditional top-down design theories.
- Human Relationships: Beck highlights that software design is an exercise in human relationships, starting with the programmer's relationship with themselves.
Why should I read "Tidy First?" by Kent Beck?
- Expert Insights: Kent Beck is a renowned figure in software design, and his insights are valuable for anyone interested in improving their coding practices.
- Practical Application: The book offers actionable advice that can be applied immediately to make codebases more manageable and maintainable.
- Empirical Approach: It presents an empirical approach to software design, focusing on real-world application rather than theoretical concepts.
- Personal Development: Reading this book can help developers enhance their skills, making them more effective and efficient in their work.
What are the key takeaways of "Tidy First?" by Kent Beck?
- Tidy First Concept: The book introduces the concept of tidying code before making changes to improve clarity and ease of modification.
- Small Changes, Big Impact: It emphasizes making small, incremental changes that can lead to significant improvements in code quality.
- Balancing Design and Behavior: Beck discusses the balance between investing in code structure and changing system behavior for optimal results.
- Human-Centric Design: The book underscores the importance of considering human relationships in software design, starting with the programmer's self-care.
What is the "Tidy First" method in Kent Beck's book?
- Initial Tidying: The method involves tidying messy code before making changes to its functionality, ensuring a cleaner and more understandable codebase.
- Safe and Efficient: It provides guidelines on how to tidy code safely and efficiently, minimizing the risk of introducing errors.
- Stopping Tidying: The book also addresses when to stop tidying, preventing over-investment in code cleanup at the expense of delivering features.
- Why Tidying Works: Beck explains the benefits of tidying, such as improved code readability and easier future modifications.
How does Kent Beck define software design in "Tidy First?"
- Beneficially Relating Elements: Beck defines software design as the process of beneficially relating elements within a codebase to improve its structure and functionality.
- Focus on Relationships: The definition emphasizes the relationships between code elements and how they can be organized to reduce complexity.
- Human-Centric Approach: It highlights the importance of considering human factors in design, such as how code changes affect team dynamics and individual productivity.
- Incremental Improvement: The definition supports the idea of making small, incremental improvements to code structure to enhance overall design.
What are some practical tips from "Tidy First?" by Kent Beck?
- Guard Clauses: Use guard clauses to simplify nested conditions and improve code readability.
- Delete Dead Code: Remove unused code to reduce clutter and potential confusion in the codebase.
- Normalize Symmetries: Ensure consistent patterns in code to make it easier to read and understand.
- Explaining Variables: Use well-named variables to clarify the purpose of complex expressions.
What is the significance of coupling and cohesion in "Tidy First?" by Kent Beck?
- Coupling Definition: Coupling refers to the degree of interdependence between code elements, with high coupling leading to more complex and costly changes.
- Cohesion Definition: Cohesion measures how closely related the responsibilities of a single module or element are, with high cohesion indicating a well-organized codebase.
- Cost of Software: Beck explains that the cost of software is closely tied to the level of coupling, as it affects the ease of making changes.
- Design Strategy: The book advocates for reducing coupling and increasing cohesion to make code easier to maintain and modify.
How does "Tidy First?" by Kent Beck address the economics of software design?
- Time Value of Money: The book discusses the concept that a dollar today is worth more than a dollar tomorrow, influencing decisions on when to tidy code.
- Optionality: Beck highlights the value of creating options in software design, allowing for future changes and adaptations.
- Balancing Act: The book explores the balance between spending money on tidying now versus the potential future benefits of having a cleaner codebase.
- Economic Incentives: It encourages developers to consider economic incentives when deciding whether to tidy code, balancing immediate costs with long-term gains.
What are the best quotes from "Tidy First?" by Kent Beck and what do they mean?
- "Software design is an exercise in human relationships." This quote emphasizes the importance of considering the human aspect of software design, including how code changes affect team dynamics and individual productivity.
- "Tidy first is a bit of an exception. When you tidy first, you know you will realize the value of tidying immediately." This highlights the immediate benefits of tidying code before making changes, such as improved readability and easier modifications.
- "The structure of the system doesn’t matter to its behavior. One big function, a whole bunch of itty bitties, same paycheck comes out." This underscores the idea that while structure doesn't affect behavior, it significantly impacts the ease of making future changes.
- "Coupling drives the cost of software." This quote points to the critical role of coupling in determining the cost and complexity of software development and maintenance.
How does "Tidy First?" by Kent Beck suggest managing tidying in a development workflow?
- Separate Tidying: Beck advises separating tidying from behavior changes in pull requests to make reviews more manageable and focused.
- Chaining Tidyings: The book discusses how tidyings can lead to further tidyings, creating a chain of improvements that enhance code quality.
- Batch Sizes: It explores the trade-offs of batching tidyings together versus handling them separately, considering factors like review latency and integration costs.
- Rhythm of Tidying: Beck emphasizes finding a rhythm for tidying that balances immediate needs with long-term code quality goals.
What is the role of reversibility in "Tidy First?" by Kent Beck?
- Reversible Decisions: Beck highlights that most structure changes in software are reversible, allowing for experimentation without long-term commitment.
- Contrast with Behavior Changes: Unlike structure changes, behavior changes can have irreversible consequences, making careful consideration essential.
- Design Strategy: The book encourages treating reversible decisions differently, focusing on quick iterations and learning from mistakes.
- Reducing Risk: By emphasizing reversibility, Beck suggests reducing the risk associated with design changes, making it easier to adapt and improve code.
How does "Tidy First?" by Kent Beck address the timing of tidying?
- First, After, Later, Never: The book categorizes tidying into four timing strategies, each with its own set of considerations and trade-offs.
- Tidy First: This approach is recommended when tidying can immediately simplify a behavior change, making it more efficient.
- Tidy After: Beck suggests tidying after a change when it can enhance future modifications without delaying current work.
- Tidy Later: The book acknowledges that some tidyings can be postponed, especially when immediate benefits are not apparent.
Review Summary
Tidy First? receives mixed reviews, with an average rating of 3.95/5. Readers appreciate its concise insights on code refactoring and software design economics. Many find the first part on "tidyings" useful for beginners but basic for experienced developers. The book's strength lies in its discussion of when and why to tidy code, offering a unique perspective on software design using financial concepts. Some criticize its brevity and high price, suggesting it could have been a blog post. Overall, it's valued for its practical advice and thought-provoking ideas on software development.
Similar Books
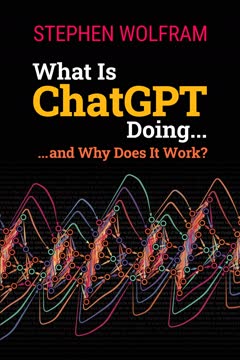
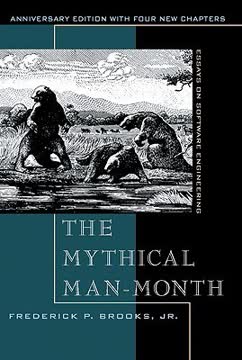
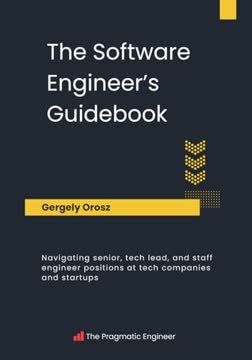
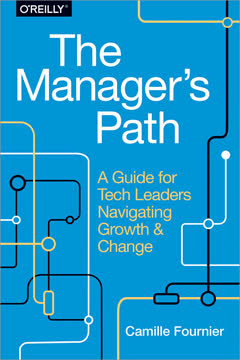
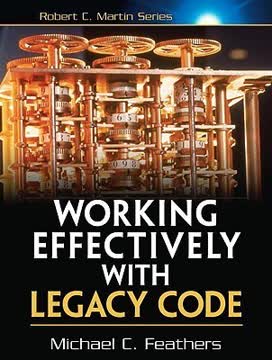
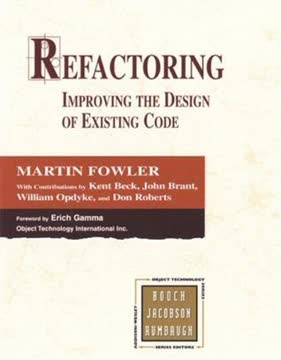
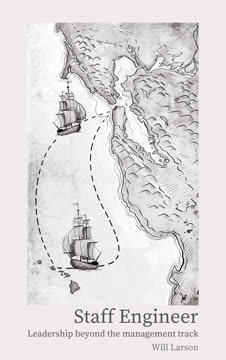
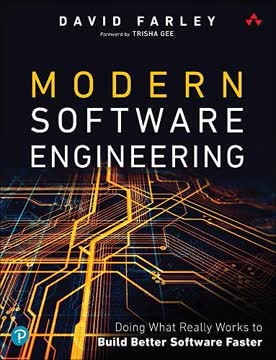
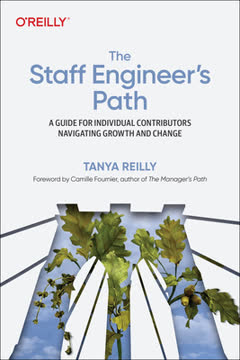
Download PDF
Download EPUB
.epub
digital book format is ideal for reading ebooks on phones, tablets, and e-readers.