Key Takeaways
1. Embrace Error Messages and Master the Command Line
As a programmer, being able to find answers on your own is far more important than any algorithms or data structure knowledge.
Error messages are your friends. Instead of ignoring error messages, learn to decipher them. Tracebacks pinpoint the location of the error, while the error message itself provides clues about the cause. Copy and paste error messages into search engines to find explanations and solutions.
Command line proficiency. The command line is a powerful tool for environment setup, running programs, and automating tasks. Learn essential commands like cd
, dir
(or ls
), copy
(or cp
), and python
to navigate the filesystem and execute code efficiently. Tab completion and command history can significantly reduce typing effort.
Environment variables and PATH. Understanding environment variables, especially the PATH variable, is crucial for running programs from the command line. Adding directories to PATH allows you to execute programs without specifying their full file paths.
2. Code Formatting with Black: Consistency is Key
Maintaining consistency and readability within a project, rather than adhering to any individual formatting rule, is the prime reason for enforcing style guides.
Readability is paramount. Code formatting is not merely cosmetic; it significantly impacts readability, which is essential for code maintenance and collaboration. Consistent formatting reduces cognitive load and makes it easier to understand the code's logic.
Black: The uncompromising formatter. Black automates code formatting, ensuring consistency across your projects. It adheres to a specific style guide, eliminating debates about spacing, line length, and other formatting preferences.
Horizontal and vertical spacing. Proper spacing within lines and between code blocks enhances readability. Use spaces around operators, after commas, and before comments. Employ blank lines to group related code and separate distinct sections.
3. Names Matter: Choose Identifiers Wisely
The two hardest problems in computer science are naming things, cache invalidation, and off-by-one errors.
Descriptive names are crucial. Choosing meaningful names for variables, functions, and classes is essential for code clarity. Names should be concise yet descriptive, avoiding abbreviations, jokes, and cultural references.
Naming conventions. Follow PEP 8's naming conventions for Python code. Use snake_case for variables and functions, PascalCase for classes, and UPPER_SNAKE_CASE for constants.
Appropriate length. Names should be long enough to be descriptive but not so long as to become unwieldy. Consider the scope of the name: local variables can have shorter names, while global variables require more descriptive names.
4. Sniff Out Code Smells to Prevent Bugs
A code smell doesn’t necessarily mean a problem exists, but it does mean you should investigate your program.
Duplicate code. The most common code smell is duplicate code, which makes maintenance difficult. Deduplicate code by placing it in functions or loops.
Magic numbers. Replace unexplained numeric values with named constants to improve readability and maintainability. This also applies to string literals.
Other code smells. Be wary of commented-out code, print debugging statements, variables with numeric suffixes, and classes that should just be functions or modules. These can indicate potential problems or areas for improvement.
5. Write Pythonic Code: Embrace the Language's Idioms
There should be one—and preferably only one—obvious way to do it.
Embrace Python's style. Pythonic code leverages the language's unique features and idioms to create concise and readable solutions. Learning these idioms is essential for writing effective Python code.
Commonly misused syntax. Avoid using range(len())
for iterating over sequences; use enumerate()
instead. Use the with
statement for file handling to ensure proper resource management. Compare with None
using is
instead of ==
.
String formatting. Use f-strings for string interpolation, as they offer a more readable and efficient alternative to older methods. Raw strings are useful for strings with many backslashes.
6. Measure Performance to Optimize Effectively
Premature optimization is the root of all evil.
Don't guess, measure. Before attempting to optimize code, measure its performance using the timeit
and cProfile
modules. This helps identify bottlenecks and ensures that your efforts are focused on the most impactful areas.
timeit
for small snippets. The timeit
module measures the execution time of small code snippets, allowing you to compare the performance of different approaches.
cProfile
for larger functions. The cProfile
module provides a detailed profile of function calls, revealing which functions consume the most time. Amdahl's Law states that improving the slowest parts of your code yields the greatest overall performance gains.
7. Object-Oriented Programming: Classes and Objects
Programs must be written for people to read, and only incidentally for machines to execute.
Classes as blueprints. Classes are blueprints for creating objects, which are instances of those classes. Classes encapsulate data (attributes) and behavior (methods) into a single unit.
Methods and __init__()
. Methods are functions associated with objects. The __init__()
method is a special method that initializes the object's attributes when it is created. The self
parameter refers to the object itself.
Attributes. Attributes are variables associated with an object. They can be accessed and modified using dot notation (e.g., object.attribute
).
8. Inheritance: Code Reuse with Caution
Placing objects in a neat hierarchy appeals to our sense of order; programmers do it just for fun.
Inheritance for code reuse. Inheritance allows you to create new classes (child classes) that inherit the methods and attributes of existing classes (parent classes). This promotes code reuse and reduces duplication.
Overriding methods. Child classes can override inherited methods to provide specialized behavior. The super()
function allows you to call the parent class's method from the child class's method.
Favor composition. Composition, which involves including objects of other classes as attributes, is often a more flexible alternative to inheritance. It avoids tight coupling between classes and promotes modularity.
9. Pythonic OOP: Properties and Dunder Methods
Please don’t anthropomorphize computers; they find it very annoying.
Properties for controlled attribute access. Properties provide a way to control how attributes are accessed, modified, and deleted. They allow you to add validation logic and prevent invalid states.
Dunder methods for operator overloading. Dunder methods (also called magic methods) allow you to define how your objects interact with Python's built-in operators (e.g., +
, -
, *
, ==
). This enables you to create more expressive and intuitive code.
Pythonic OOP. By using properties and dunder methods, you can create classes that are more Pythonic, readable, and maintainable. These features allow you to leverage Python's unique strengths and write code that feels natural and expressive.
Last updated:
Review Summary
Beyond the Basic Stuff with Python is highly praised for bridging the gap between beginner and intermediate Python programming. Readers appreciate its coverage of best practices, tools, and techniques, including code formatting, naming conventions, Git usage, and object-oriented programming. The book is commended for its clear explanations, practical examples, and focus on Pythonic code. Many reviewers found it helpful in advancing their skills and understanding of software development concepts. While some felt certain topics could have been explored more deeply, the overall consensus is that it's an excellent resource for Python learners looking to progress beyond the basics.
Similar Books
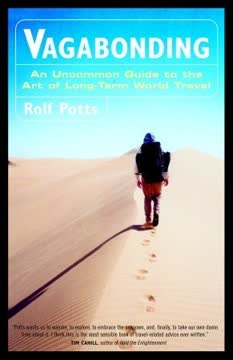
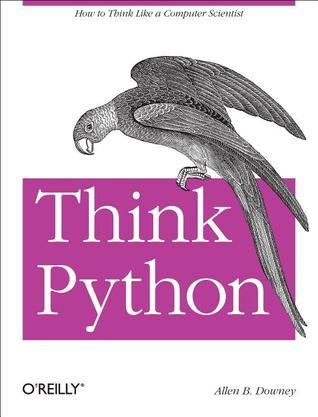
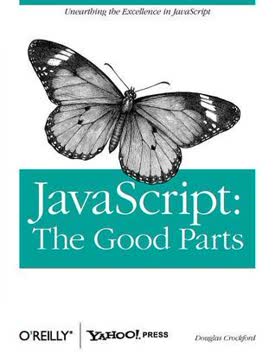
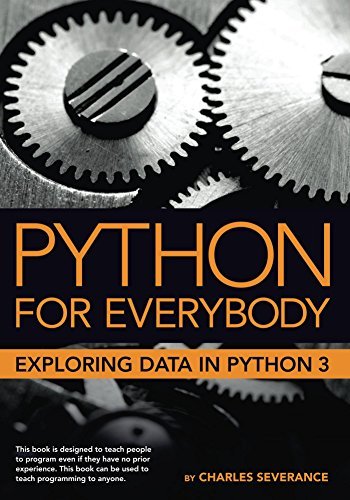
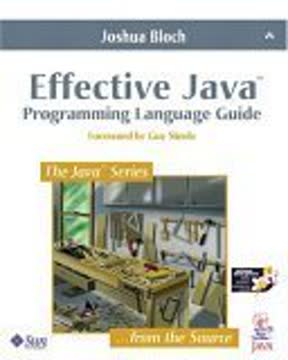
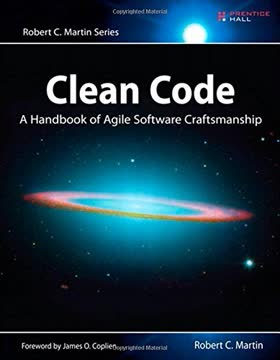
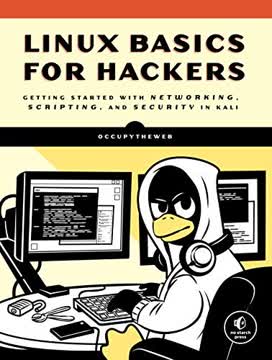
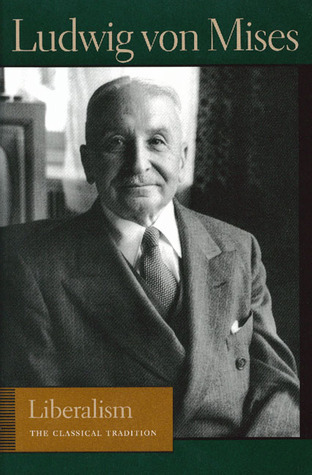
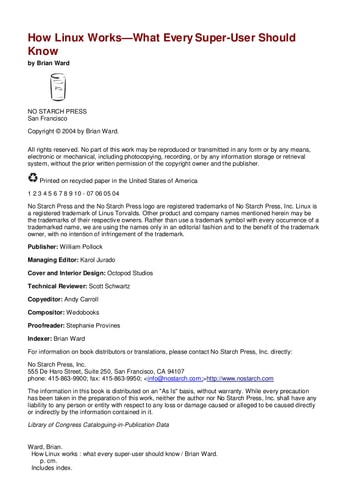
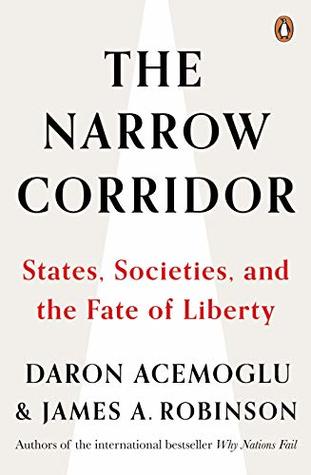
Download PDF
Download EPUB
.epub
digital book format is ideal for reading ebooks on phones, tablets, and e-readers.