Key Takeaways
1. Programming empowers creativity and problem-solving.
Writing programs (or programming) is a very creative and rewarding activity.
Creative outlet. Programming is not just about following instructions; it's a creative process. It allows individuals to express their ideas, solve problems, and build innovative solutions. It transforms abstract concepts into tangible realities.
Problem-solving skills. Learning to program cultivates critical thinking and problem-solving abilities. It teaches one to break down complex problems into smaller, manageable steps, and to develop logical solutions.
- Analyzing data
- Automating tasks
- Creating new tools
Beyond professional programming. The skills acquired through programming are valuable in various fields, enabling individuals to use technology effectively in their chosen professions, regardless of whether they become professional programmers.
2. Understanding computer hardware architecture is fundamental.
The Central Processing Unit (or CPU) is the part of the computer that is built to be obsessed with “what is next?”
Key components. A basic understanding of computer hardware architecture, including the CPU, main memory, secondary memory, and input/output devices, is essential for programmers. Knowing how these components interact helps in writing efficient and effective code.
CPU's role. The Central Processing Unit (CPU) is the brain of the computer, executing instructions at an incredibly fast pace. Understanding the CPU's role helps programmers appreciate the need for efficient algorithms and code optimization.
- 3.0 Gigahertz means the CPU asks "What next?" three billion times per second.
Memory hierarchy. Main memory provides fast access to data, but it's volatile. Secondary memory offers persistent storage, but it's slower. Understanding this trade-off helps programmers make informed decisions about data storage and retrieval.
3. Programming languages bridge human intent and machine execution.
If we knew this language, we could tell the computer to do tasks on our behalf that were repetitive.
High-level languages. Programming languages like Python are designed to be relatively easy for humans to read and write. They abstract away the complexities of machine language, allowing programmers to focus on problem-solving.
Interpreters and compilers. Translators, such as interpreters and compilers, convert high-level code into machine language that the CPU can understand. This translation process enables programmers to write code that is portable across different types of hardware.
- Python is an interpreter.
- C is a compiled language.
Problem-solving skills. Learning a programming language involves mastering its vocabulary and grammar, as well as developing the ability to "tell a story" through code, conveying an idea to the computer in a logical and meaningful way.
4. Variables, expressions, and statements form the core of Python code.
A statement is a unit of code that the Python interpreter can execute.
Fundamental building blocks. Variables, expressions, and statements are the fundamental building blocks of Python code. Understanding how to use them correctly is essential for writing functional programs.
Variables and types. Variables are names that refer to values, and each value has a specific type (e.g., integer, string, float). Choosing meaningful variable names and understanding data types are crucial for code readability and correctness.
- Mnemonic variable names help remember why the variable was created.
Operators and expressions. Operators are special symbols that perform computations, and expressions are combinations of values, variables, and operators. Understanding operator precedence and how to construct valid expressions is essential for performing calculations.
5. Conditional execution enables programs to make decisions.
The boolean expression after the if statement is called the condition.
Controlling program flow. Conditional statements (if, elif, else) allow programs to execute different blocks of code based on certain conditions. This enables programs to make decisions and respond dynamically to different inputs.
Boolean expressions. Conditional statements rely on boolean expressions, which evaluate to either True or False. Comparison operators (e.g., ==, !=, >, <) and logical operators (e.g., and, or, not) are used to construct boolean expressions.
x > 0 and x < 10
is true only if x is greater than 0 and less than 10.
Error handling. The try
and except
blocks allow programs to gracefully handle errors and exceptions, preventing them from crashing. This is particularly useful when dealing with user input or external data sources.
6. Functions promote code reusability and modularity.
When you define a function, you specify the name and the sequence of statements.
Code organization. Functions are named sequences of statements that perform a specific task. They promote code reusability, modularity, and readability by breaking down large programs into smaller, manageable units.
Parameters and arguments. Functions can take arguments as input, which are assigned to parameters within the function. This allows functions to operate on different data each time they are called.
def greet(name):
name is the parameter.greet("Alice")
"Alice" is the argument.
Fruitful vs. void functions. Fruitful functions return a value, while void functions perform an action but don't return anything. Understanding the difference is crucial for using functions effectively.
7. Iteration automates repetitive tasks.
This type of flow is called a loop because the third step loops back around to the top.
Looping constructs. Iteration, using while
and for
loops, allows programs to automate repetitive tasks. This is essential for processing large amounts of data or performing actions multiple times.
while
loops. while
loops continue executing as long as a certain condition is true. It is important to ensure that the condition eventually becomes false to avoid infinite loops.
break
statement exits the loop.continue
statement skips to the next iteration.
for
loops. for
loops iterate over a sequence of items, such as a list or a string. This provides a convenient way to process each item in the sequence.
8. Strings are sequences of characters with powerful manipulation tools.
A string is a sequence of characters.
String operations. Strings are sequences of characters that can be manipulated using various operators and methods. Understanding string operations is essential for working with text data.
String methods. Python provides a rich set of string methods for tasks such as:
len()
: Getting the length of a stringfind()
: Searching for substringssplit()
: Breaking a string into a list of wordsupper()
: Converting to uppercaselower()
: Converting to lowercasestrip()
: Removing whitespace
Immutability. Strings are immutable, meaning they cannot be changed after they are created. Operations that appear to modify strings actually create new strings.
9. Files provide persistent data storage.
Secondary memory is not erased when the power is turned off.
Data persistence. Files provide a way to store data persistently, so it can be retrieved even after the program ends. This is essential for applications that need to save and load data.
File operations. To work with files, you must first open them using the open()
function, specifying the file name and mode (e.g., read, write). Then, you can read data from the file or write data to it.
open('filename.txt', 'r')
: Opens a file for reading.open('filename.txt', 'w')
: Opens a file for writing (overwrites existing content).open('filename.txt', 'a')
: Opens a file for appending (adds to existing content).
Error handling. It's important to use try
and except
blocks to handle potential errors when working with files, such as file not found or permission denied.
10. Lists, dictionaries, and tuples are versatile data structures.
A list is a sequence of values.
Data organization. Lists, dictionaries, and tuples are versatile data structures that allow you to organize and store collections of data. Choosing the right data structure is crucial for efficient data manipulation.
Lists. Lists are mutable sequences of values, indexed by integers. They support various operations such as appending, inserting, deleting, and sorting.
append()
: Adds an element to the end of the list.insert()
: Inserts an element at a specific position.remove()
: Removes the first occurrence of a specific element.sort()
: Sorts the list in ascending order.
Dictionaries. Dictionaries are mappings between keys and values. They provide fast lookups based on keys and are useful for storing data in a structured way.
Tuples. Tuples are immutable sequences of values. They are similar to lists, but they cannot be modified after they are created.
11. Regular expressions enable sophisticated pattern matching.
The power of the regular expressions comes when we add special characters to the search string that allow us to more precisely control which lines match the string.
Pattern recognition. Regular expressions are a powerful tool for searching and extracting substrings that match a particular pattern. They provide a concise and flexible way to define complex search criteria.
Special characters. Regular expressions use special characters to represent various patterns, such as:
^
: Matches the beginning of a line$
: Matches the end of a line.
: Matches any character\s
: Matches a whitespace character\S
: Matches a non-whitespace character*
: Matches zero or more occurrences+
: Matches one or more occurrences
findall()
method. The findall()
method searches a string for all substrings that match a regular expression and returns them as a list.
12. Networked programs facilitate communication and data retrieval.
A socket is much like a file, except that a single socket provides a two-way connection between two programs.
Sockets. Sockets provide a low-level way to establish network connections between two programs. They allow programs to send and receive data over the Internet.
HTTP protocol. The Hypertext Transfer Protocol (HTTP) is the network protocol that powers the web. Understanding HTTP is essential for building web applications and retrieving data from web servers.
GET
command requests a document from a web server.
urllib
library. The urllib
library provides a higher-level way to interact with web servers, handling the details of the HTTP protocol and making it easier to retrieve web pages and other resources.
13. Web services offer structured data exchange via APIs.
When an application makes a set of services in its API available over the web, we call these web services.
APIs. Application Programming Interfaces (APIs) define contracts between applications, specifying how they can interact with each other. Web services expose APIs over the web, allowing programs to access data and functionality from other programs.
Data formats. XML and JSON are common formats for exchanging data between applications. JSON is quickly becoming the format of choice due to its simplicity and direct mapping to native data structures.
- XML is better suited for document-style data.
- JSON is better suited for exchanging dictionaries, lists, or other internal information.
Security. API keys and OAuth are used to secure web services and control access to data.
14. Object-oriented programming manages complexity through modularity.
In a way, object oriented programming is a way to arrange your code so that you can zoom into 50 lines of the code and understand it while ignoring the other 999,950 lines of code for the moment.
Code organization. Object-oriented programming (OOP) is a paradigm that organizes code into objects, which encapsulate data (attributes) and behavior (methods). This promotes code reusability, modularity, and maintainability.
Classes and objects. Classes are templates for creating objects. They define the attributes and methods that objects of that class will have. Objects are instances of classes.
class PartyAnimal:
defines a class.an = PartyAnimal()
creates an object.
Inheritance. Inheritance allows you to create new classes (child classes) by extending existing classes (parent classes). This promotes code reuse and allows you to build complex hierarchies of objects.
Last updated:
FAQ
1. What is "Python for Everybody: Exploring Data in Python 3" by Charles Severance about?
- Beginner-friendly Python introduction: The book is a comprehensive guide to learning Python 3, focusing on data exploration and analysis rather than traditional computer science theory.
- Real-world data applications: It emphasizes practical skills like reading files, parsing data, web scraping, and working with databases, making it ideal for those interested in data science.
- Open educational resource: Authored by Charles Severance, it is based on "Think Python" but adapted for hands-on data handling, and is freely available under a Creative Commons license.
- Stepwise learning: Concepts are introduced gradually, from basic programming to advanced topics like object-oriented programming and data visualization.
2. Why should I read "Python for Everybody" by Charles Severance?
- Practical data skills: The book teaches you to use Python to automate tasks, analyze data, and solve real-world problems, even if you don’t plan to become a professional programmer.
- Hands-on learning: It provides numerous code examples and exercises using real datasets, such as emails, text files, and web data, to reinforce learning.
- Broad applicability: Skills learned are useful in many fields—biology, economics, law, management—where data handling is essential.
- Emphasis on creativity and productivity: Programming is presented as a creative, rewarding activity that enhances your ability to use technology effectively.
3. What are the key programming concepts covered in "Python for Everybody" by Charles Severance?
- Core constructs: The book covers variables, expressions, statements, input/output, conditional execution, loops, and functions as the building blocks of Python programs.
- Data structures: Lists, dictionaries, and tuples are explained in detail, including their use in data manipulation and analysis.
- Object-oriented programming: It introduces classes, objects, inheritance, and constructors to help organize larger programs.
- Advanced topics: Regular expressions, web scraping, and database integration are included for more complex data tasks.
4. How does "Python for Everybody" by Charles Severance explain variables, expressions, and statements?
- Variables as named storage: Variables are symbolic names for values stored in memory, created or updated with assignment statements.
- Expressions and operators: The book details how to combine values, variables, and operators (+, -, *, /, %, **) to compute results, following standard precedence rules.
- Data types and input: It covers basic types like integers, floats, and strings, and demonstrates how to use the input() function and type conversion.
- Statements as instructions: Each line of code is a statement that tells the computer what to do, forming the backbone of Python programs.
5. What are the fundamental building blocks of programs in "Python for Everybody" by Charles Severance?
- Input and output: Programs interact with users and data sources through input and output operations.
- Sequential and conditional execution: Code runs in order, but can branch using if, elif, and else statements based on conditions.
- Loops and reuse: Repeated execution is handled with while and for loops, and code reuse is achieved through functions.
- Composition: The art of programming is in combining these elements to create useful, sophisticated programs.
6. How does "Python for Everybody" by Charles Severance teach conditional execution and exception handling?
- Boolean logic: The book explains how to use boolean expressions (True/False) to control program flow with if, elif, and else statements.
- Alternative and chained conditionals: It covers simple and complex branching for handling multiple scenarios.
- Exception handling: Try and except blocks are introduced to catch and handle runtime errors gracefully, making programs more robust.
- Practical examples: Real-world scenarios, such as file not found errors, are used to illustrate these concepts.
7. How are functions introduced and used in "Python for Everybody" by Charles Severance?
- Reusable code blocks: Functions are named sequences of statements that can be called multiple times, improving code organization and reducing repetition.
- Parameters and return values: Functions can accept arguments and return results, supporting both fruitful (returning values) and void (no return) functions.
- Flow of execution: Calling a function temporarily diverts program flow to execute its body, then returns to the caller.
- Modular programming: Functions enable breaking down complex problems into manageable pieces.
8. What iteration techniques are taught in "Python for Everybody" by Charles Severance?
- While loops: Used for indefinite iteration, repeating as long as a condition is true, with break and continue for finer control.
- For loops: Ideal for definite iteration over sequences like lists or files, executing the loop body once per item.
- Common loop patterns: The book covers counting, summing, finding maximum/minimum values, and debugging strategies like "debugging by bisection."
- Practical examples: Real data processing tasks are used to illustrate loop usage.
9. How does "Python for Everybody" by Charles Severance handle strings and their manipulation?
- Strings as sequences: Strings are treated as sequences of characters, accessible by index and supporting slicing.
- Immutability and methods: Strings cannot be changed in place, but methods like upper(), lower(), find(), startswith(), and strip() provide powerful manipulation tools.
- Parsing and formatting: The book covers extracting substrings using find and slicing, and formatting strings with the % operator.
- Real-world parsing: Examples include analyzing text files and extracting information from lines of data.
10. What file handling and data input/output concepts are covered in "Python for Everybody" by Charles Severance?
- Reading and writing files: The book teaches how to open, read, write, and close files, including handling both text and binary data.
- Searching and processing: It demonstrates searching for lines matching conditions, filtering with string methods, and handling user input for file names.
- Error handling: Try-except blocks are used to manage file-related errors, such as missing or malformed files, ensuring program stability.
- Practical applications: Examples include reading email logs, saving images, and parsing large datasets.
11. How are lists, dictionaries, and tuples used for data handling in "Python for Everybody" by Charles Severance?
- Lists as mutable sequences: Lists can store heterogeneous elements, support indexing, slicing, and methods like append(), extend(), sort(), and remove().
- Dictionaries as key-value stores: Dictionaries provide fast lookup, support methods like get(), keys(), and values(), and are ideal for counting and frequency analysis.
- Tuples for immutability: Tuples are immutable and can be used as dictionary keys or for multiple assignment in loops.
- Combined usage: The book demonstrates using tuples as dictionary keys, iterating with tuple unpacking, and sorting dictionary items by values.
12. What advanced topics—such as regular expressions, web scraping, and databases—are covered in "Python for Everybody" by Charles Severance?
- Regular expressions: The book explains regex syntax and usage for powerful pattern matching and data extraction, including greedy vs. non-greedy matching.
- Web scraping: It covers using sockets, urllib, and BeautifulSoup to fetch and parse web content, with advice on respectful scraping practices.
- Database integration: SQLite is introduced for storing and querying data, with examples of creating tables, normalization, and using SQL within Python.
- Data visualization: The book presents projects that visualize data using OpenStreetMap, D3.js, and JSON, integrating database and web data for interactive analysis.
Review Summary
Python for Everybody receives mostly positive reviews, with an average rating of 4.28/5. Readers praise its beginner-friendly approach, clear explanations, and practical examples. Many find it an excellent companion to the author's Coursera course. The book is commended for its coverage of fundamentals and introduction to data analysis. Some criticisms include a steep difficulty increase in later chapters and insufficient depth in certain topics. Overall, it's considered a valuable resource for those new to programming or Python, especially when paired with additional materials.
Similar Books
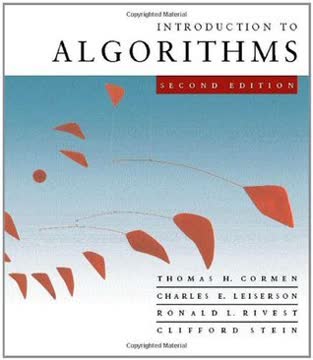
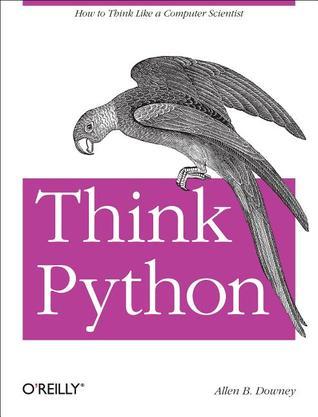
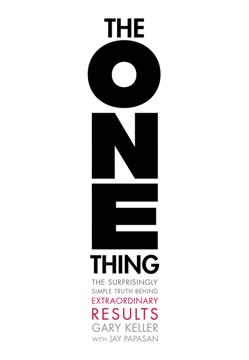
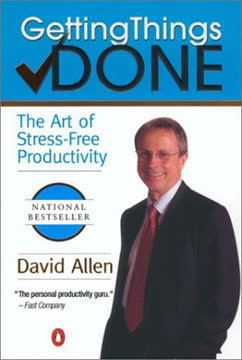
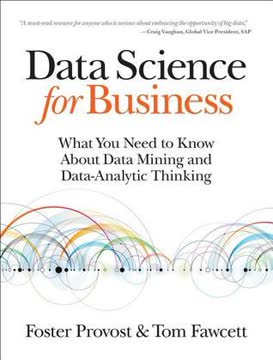
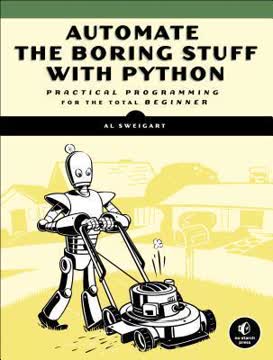


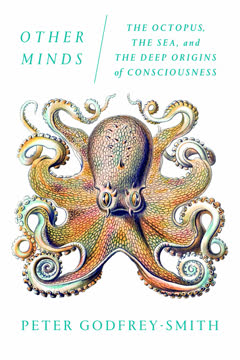

Download PDF
Download EPUB
.epub
digital book format is ideal for reading ebooks on phones, tablets, and e-readers.