Key Takeaways
1. Frameworks enforce security and reliability across applications
Frameworks enable code reuse: rather than accounting for all of the security and reliability aspects affecting a given functionality or feature, developers only need to customize a specific building block.
Standardized protection. Frameworks provide a consistent approach to addressing common security and reliability concerns across multiple applications. By centralizing critical functions like authentication, authorization, and error handling, frameworks reduce the likelihood of vulnerabilities arising from inconsistent implementations. This approach allows domain experts to design and develop secure building blocks, benefiting all teams using the framework.
Increased productivity. Using frameworks leads to higher productivity for developers, as they can focus on business logic rather than reinventing security and reliability features. Frameworks often come with built-in tools for monitoring, logging, and performance optimization, further streamlining development processes. This standardization also makes it easier to reason about code security and reliability, as common patterns are consistently applied across projects.
2. Simplicity in code design enhances security and maintainability
Avoid YAGNI code leads to improved reliability, and simpler code leads to fewer security bugs, fewer opportunities to make mistakes, and less developer time spent maintaining unused code.
Reducing complexity. Simpler code is easier to understand, review, and maintain. By following principles like YAGNI (You Aren't Gonna Need It) and avoiding over-engineering, developers can create more robust and secure systems. Simpler code has fewer potential points of failure and is less likely to contain hidden vulnerabilities.
Improving maintainability. Regular refactoring and addressing technical debt are crucial for maintaining code simplicity over time. This ongoing process helps prevent the accumulation of complex, hard-to-understand code that can lead to security and reliability issues. Tools like linters and code health metrics can help teams identify areas for improvement and maintain high code quality standards.
3. Strong typing and sanitization prevent common vulnerabilities
Using strong types protects your code from errors of this type that a compiler doesn't capture.
Type safety. Strong typing helps catch errors at compile-time rather than runtime, preventing many common vulnerabilities before they reach production. By using specific types for different contexts (e.g., SafeHtml for HTML content, SafeUrl for URLs), developers can enforce security properties by design. This approach makes it much harder to introduce vulnerabilities like SQL injection or cross-site scripting (XSS).
Input validation. Proper input sanitization is crucial for preventing security vulnerabilities. By using dedicated libraries and frameworks for handling user input, developers can ensure that all data is properly validated and sanitized before being used in sensitive operations. This includes:
- Escaping special characters in user-generated content
- Validating and sanitizing URLs and file paths
- Enforcing strict type checking for all inputs
4. Unit testing verifies individual components' behavior
Unit tests typically run locally as part of engineering workflows to provide fast feedback to developers before they submit changes to the codebase.
Granular verification. Unit tests focus on verifying the behavior of individual software components in isolation. This approach allows developers to catch bugs early in the development process and ensures that each component functions correctly before integration. Effective unit testing practices include:
- Writing tests alongside or before the actual code (Test-Driven Development)
- Covering both normal and edge cases
- Using mocks and stubs to isolate dependencies
Continuous feedback. By integrating unit tests into the development workflow, teams can catch regressions and unintended side effects quickly. This continuous feedback loop helps maintain code quality and prevents the introduction of new bugs. Automated CI/CD pipelines can run unit tests on every code change, providing immediate feedback to developers.
5. Integration testing evaluates system-wide interactions
Integration testing moves beyond individual units and abstractions, replacing fake or stubbed-out implementations of abstractions like databases or network services with real implementations.
System-level verification. Integration tests evaluate how different components of a system work together, uncovering issues that may not be apparent when testing units in isolation. These tests help ensure that the system functions correctly as a whole, including interactions with external dependencies like databases and APIs. Key considerations for integration testing include:
- Setting up realistic test environments
- Managing test data securely
- Handling asynchronous operations and timing issues
Balancing coverage and speed. While integration tests provide valuable insights into system behavior, they are typically slower and more resource-intensive than unit tests. Teams need to find the right balance between comprehensive integration testing and maintaining fast feedback loops. Strategies like selective integration testing based on risk assessment can help optimize test coverage without sacrificing development velocity.
6. Dynamic analysis tools detect runtime errors and vulnerabilities
Dynamic program analysis analyzes software by running programs, potentially in virtualized or emulated environments, for purposes beyond just testing.
Runtime error detection. Dynamic analysis tools like sanitizers can detect various runtime errors and vulnerabilities that may not be apparent through static analysis or regular testing. These tools instrument the code during compilation to add runtime checks for issues such as:
- Memory leaks and buffer overflows
- Use-after-free errors
- Data races and deadlocks
- Undefined behavior
Performance considerations. While dynamic analysis tools provide valuable insights, they can significantly impact performance. Teams often run these tools as part of nightly builds or dedicated testing pipelines to balance the need for thorough analysis with development speed. The insights gained from dynamic analysis can help teams prioritize bug fixes and improve overall code quality.
7. Fuzz testing uncovers edge cases and unexpected inputs
Fuzzing can be useful for testing service resilience.
Automated input generation. Fuzz testing involves generating large numbers of random or semi-random inputs to uncover bugs and vulnerabilities. This technique is particularly effective at finding edge cases and unexpected behaviors that manual testing might miss. Fuzz testing can help identify:
- Memory corruption issues
- Input validation flaws
- Denial-of-service vulnerabilities
- Parsing errors in file formats or network protocols
Continuous fuzzing. Integrating fuzz testing into continuous integration pipelines allows teams to constantly probe their systems for new vulnerabilities. As the codebase evolves, fuzzing can uncover regressions or new issues introduced by changes. Tools like libFuzzer and AFL (American Fuzzy Lop) make it easier to implement fuzz testing for various types of software.
8. Continuous validation ensures ongoing system resilience
Carefully designed test suites can evaluate the correctness of different pieces of software that perform the same task.
Proactive resilience. Continuous validation goes beyond traditional testing by constantly exercising the system under various conditions. This approach helps ensure that the system remains resilient to failures and attacks over time. Continuous validation techniques include:
- Chaos engineering experiments
- Regular disaster recovery drills
- Automated security scanning and penetration testing
- Performance stress testing under realistic load conditions
Evolving threat models. As systems grow and evolve, new vulnerabilities and failure modes may emerge. Continuous validation helps teams stay ahead of these challenges by regularly reassessing their systems' resilience. This process should include updating threat models, revising test scenarios, and incorporating lessons learned from real-world incidents and near-misses.
Last updated:
FAQ
What's Building Secure and Reliable Systems about?
- Focus on Integration: The book emphasizes integrating security and reliability into the entire lifecycle of system design, implementation, and maintenance.
- Practical Guidance: It offers practical advice and best practices for organizations of all sizes, from startups to large enterprises.
- Cultural Aspects: The authors discuss the importance of fostering a culture of security and reliability within organizations.
Why should I read Building Secure and Reliable Systems?
- Comprehensive Resource: It serves as a guide for professionals involved in system design and operations, offering insights from experienced practitioners at Google.
- Real-World Examples: The book shares case studies and examples, making the concepts relatable and applicable to various organizational contexts.
- Improving Practices: Readers can learn how to improve their organization's security posture and reliability practices.
What are the key takeaways of Building Secure and Reliable Systems?
- Integration of Principles: Security and reliability should be integrated into every stage of the system lifecycle.
- Crisis Management: Effective crisis management strategies, including incident response planning, are outlined.
- Cultural Importance: A culture that prioritizes security and reliability is essential for resilient systems.
What are the best quotes from Building Secure and Reliable Systems and what do they mean?
- "Security and reliability are inherent properties of a system.": These aspects should be integrated from the beginning.
- "A culture of inevitability can help teams have the appropriate frame of mind to build secure and reliable systems.": Accepting that failures can happen leads to better resilience.
- "Everyone is responsible for security and reliability.": Security and reliability should be a collective effort across the organization.
What is the principle of least privilege in Building Secure and Reliable Systems?
- Definition: Users should have the minimum access necessary to accomplish their tasks.
- Reducing Risk: Limiting access minimizes the potential for mistakes or malicious actions.
- Implementation Strategies: Strategies include using small functional APIs and advanced authorization controls.
How does Building Secure and Reliable Systems define adversaries?
- Understanding Adversaries: Adversaries are individuals or groups that may exploit system vulnerabilities.
- Motivations: Motivations include financial gain, activism, and espionage.
- Frameworks for Analysis: The book provides frameworks for analyzing adversaries, aiding in designing resilient systems.
What role does culture play in security and reliability according to Building Secure and Reliable Systems?
- Cultural Shift: Building a culture of security and reliability is essential for long-term success.
- Empowerment and Training: Training employees and empowering them to take ownership of security practices is crucial.
- Collaboration and Communication: Open communication and collaboration help identify potential issues early.
What is the role of the incident commander (IC) in crisis management as described in Building Secure and Reliable Systems?
- Leadership During Incidents: The IC leads the incident response team and makes critical decisions.
- Maintaining Morale: The IC focuses on maintaining team morale during high-pressure situations.
- Handover Processes: The IC manages handovers between shifts to ensure continuity.
How does Building Secure and Reliable Systems address the trade-offs between security and reliability?
- Balancing Act: Decisions in one area can impact the other, requiring careful consideration.
- Design Considerations: Designers should ensure security measures do not compromise reliability.
- Real-World Examples: Examples show how organizations navigate these trade-offs effectively.
What are some best practices for designing secure systems in Building Secure and Reliable Systems?
- Design for Understandability: Systems should be understandable to aid in security and reliability.
- Use of Proxies: Safe proxies manage access and enforce security policies.
- Continuous Improvement: Regularly review and update security practices based on new insights.
What are the advanced mitigation strategies discussed in Building Secure and Reliable Systems?
- Defense in Depth: Implement multiple defensive measures to protect systems.
- Automated Response Mechanisms: Use automated systems for quick incident response.
- Regular Testing and Updates: Continuously test and update security measures to adapt to threats.
How does Building Secure and Reliable Systems suggest handling legacy code?
- Consolidate Exemption Mechanisms: Simplify the codebase to reduce vulnerabilities.
- Refactor Regularly: Improve security and reliability through regular refactoring.
- Avoid Overengineering: Promote the YAGNI principle to keep the codebase manageable.
Review Summary
Building Secure and Reliable Systems receives mixed reviews, with an average rating of 3.90 out of 5. Readers appreciate its comprehensive coverage of security and reliability principles, particularly for large organizations. Many find it valuable for training and as a reference guide. However, some criticize its structure, verbosity, and occasional lack of clarity. The book is praised for integrating security and reliability concepts but is also noted for being Google-centric. While some chapters are considered too theoretical or detailed, others are highly practical. Overall, it's recommended for security professionals and SREs.
Similar Books
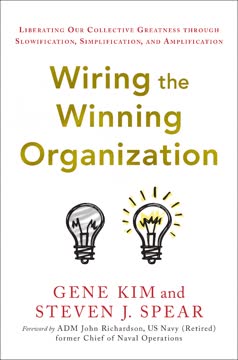
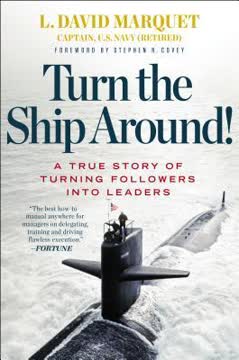
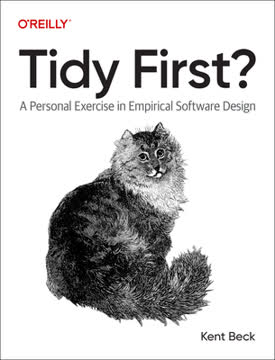
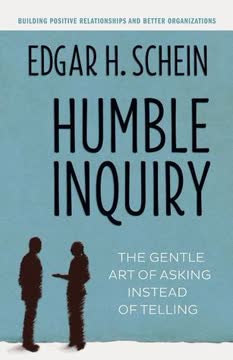
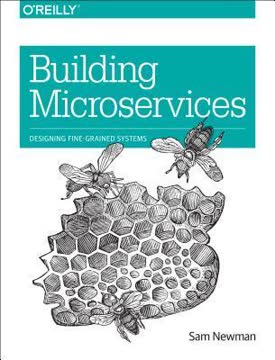
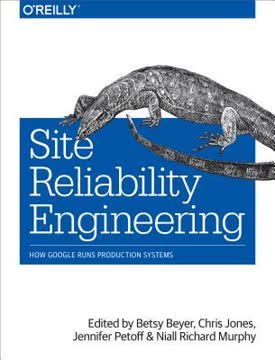
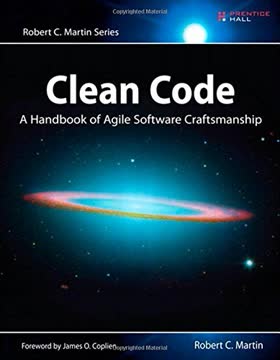
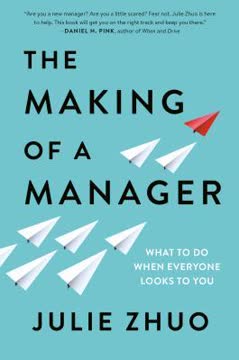
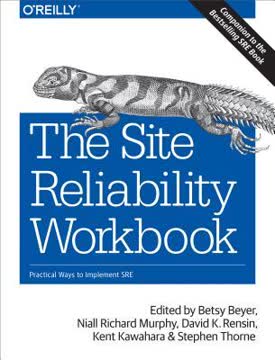
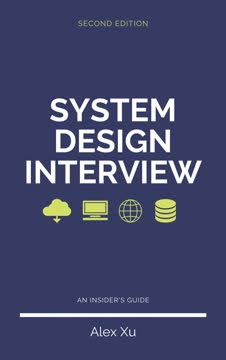
Download PDF
Download EPUB
.epub
digital book format is ideal for reading ebooks on phones, tablets, and e-readers.