Key Takeaways
1. TypeScript is a superset of JavaScript, offering optional static typing
TypeScript is a bit unusual as a language in that it neither runs in an interpreter (as Python and Ruby do) nor compiles down to a lower-level language (as Java and C do). Instead, it compiles to another high-level language, JavaScript.
TypeScript enhances JavaScript with optional static typing, allowing developers to catch errors early and improve code quality. It maintains full compatibility with JavaScript, making it an ideal choice for gradual adoption in existing projects. The TypeScript compiler performs type checking during development but generates plain JavaScript for runtime execution. This approach provides the benefits of static typing without sacrificing JavaScript's flexibility and ecosystem.
Key features of TypeScript include:
- Optional static typing
- Enhanced IDE support with intelligent code completion
- Advanced object-oriented programming features
- Compatibility with existing JavaScript code
- Ability to use latest ECMAScript features regardless of target environment
2. Understand type inference and use it effectively
TypeScript aims to make your life easier, but TypeScript with lots of any types can be harder to work with than untyped JavaScript because you have to fix type errors and still keep track of the real types in your head.
Leverage type inference to write cleaner, more concise code while maintaining type safety. TypeScript's type inference system is sophisticated and can often deduce types without explicit annotations. This reduces boilerplate and makes the code more readable. However, it's crucial to understand how inference works to avoid unexpected behavior and maintain type safety.
Best practices for type inference:
- Use const for variables that won't be reassigned to help TypeScript infer more specific types
- Let TypeScript infer return types for functions when possible
- Use explicit type annotations for function parameters to improve readability and catch errors
- Be aware of how context affects type inference, especially in callbacks and higher-order functions
3. Design robust types that represent valid states
Types that represent both valid and invalid states are likely to lead to confusing and error-prone code.
Create precise types that accurately model your domain and prevent invalid states. Well-designed types act as a form of documentation and help catch logical errors at compile-time. This approach leads to more maintainable and less error-prone code. When designing types, focus on representing only valid states and use TypeScript's features to enforce constraints.
Techniques for robust type design:
- Use union types to represent mutually exclusive states
- Leverage literal types to create more specific type constraints
- Implement discriminated unions for complex state representations
- Use readonly modifiers to prevent accidental mutations
- Employ conditional types and mapped types for advanced type transformations
4. Leverage TypeScript's structural typing system
TypeScript models this behavior, and it can sometimes lead to surprising results because the type checker's understanding of a type may be broader than what you had in mind.
Embrace structural typing to write more flexible and reusable code. TypeScript's structural typing system focuses on the shape of objects rather than their nominal types. This approach allows for greater code reuse and flexibility but requires a different mindset compared to nominally typed languages like Java or C#.
Key aspects of structural typing:
- Objects are compatible if they have the same shape, regardless of their declared type
- Interfaces can be implemented implicitly, without explicit declaration
- Excess property checks are performed on object literals but not on variables
- Use index signatures to define objects with arbitrary properties
- Be aware of the differences between fresh and stale object literals in type checking
5. Use advanced type features judiciously
By adopting TypeScript you're trusting the judgment of the team that builds it.
Apply advanced features carefully to solve specific problems without overcomplicating your code. TypeScript offers a rich set of advanced type features, such as conditional types, mapped types, and type operators. While these can be powerful tools for expressing complex type relationships, they can also make code harder to understand and maintain if overused.
Guidelines for using advanced type features:
- Start with simple types and only introduce complexity when necessary
- Use conditional types to create flexible, reusable type definitions
- Leverage mapped types to transform existing types systematically
- Employ type operators like keyof and typeof to create derived types
- Document complex type constructs to aid understanding for other developers
6. Write modular code with TypeScript
TypeScript tends to be quite good at tracking types through conditionals. Think twice before adding an assertion—it might be onto something that you're not!
Organize code into modules to improve maintainability and reusability. TypeScript's module system, based on ECMAScript modules, allows for better code organization and encapsulation. Properly structured modules make it easier to reason about code, manage dependencies, and refactor large codebases.
Best practices for modular TypeScript:
- Use ECMAScript module syntax (import/export) instead of namespace
- Export only what's necessary from a module to maintain encapsulation
- Leverage barrel files to simplify imports from complex module structures
- Use dynamic imports for code splitting and improved performance
- Organize related functionality into cohesive modules
7. Migrate JavaScript projects to TypeScript incrementally
TypeScript's type system is gradual and optional: gradual because you can add types to your code bit by bit and optional because you can disable the type checker whenever you like.
Adopt TypeScript gradually in existing JavaScript projects to minimize disruption and maximize benefits. TypeScript's design allows for incremental adoption, making it possible to migrate large codebases without a complete rewrite. This approach lets teams experience the benefits of TypeScript while continuing to deliver features.
Steps for incremental migration:
- Set up TypeScript in the project and configure the build process
- Enable JavaScript files in the TypeScript compilation (allowJs option)
- Gradually rename .js files to .ts, starting with isolated modules
- Add type annotations incrementally, focusing on function signatures
- Enable stricter compiler options over time (e.g., noImplicitAny, strictNullChecks)
8. Embrace modern JavaScript features in TypeScript
TypeScript lets you write modern JavaScript whatever your runtime environment.
Utilize modern JavaScript features to write cleaner, more expressive code in TypeScript. TypeScript supports the latest ECMAScript features and can transpile them to older versions of JavaScript for compatibility. This allows developers to use modern language constructs regardless of the target runtime environment.
Key modern JavaScript features to use in TypeScript:
- Arrow functions for concise function syntax and lexical this binding
- Destructuring assignments for cleaner object and array access
- Async/await for more readable asynchronous code
- Template literals for easier string interpolation
- Optional chaining and nullish coalescing for safer property access
- Class fields and private fields for cleaner class definitions
9. Optimize TypeScript configurations for better type safety
TypeScript's motto is "JavaScript that scales." A key part of "scales" is the language services, which are a core part of the TypeScript experience.
Fine-tune compiler options to enhance type safety and catch more potential errors. TypeScript offers numerous compiler options that affect type checking strictness and code generation. Configuring these options appropriately can significantly improve code quality and developer experience.
Important compiler options to consider:
- Enable strict mode (strict: true) for the most comprehensive type checking
- Use noImplicitAny to catch untyped expressions
- Enable strictNullChecks to handle null and undefined more safely
- Set strictFunctionTypes for stricter function type checking
- Use noUncheckedIndexedAccess to catch potential undefined access on indexed types
- Enable esModuleInterop for better interoperability with CommonJS modules
Last updated:
FAQ
What's Effective TypeScript about?
- Focus on Improvement: Effective TypeScript by Dan Vanderkam is a guide designed to enhance your TypeScript skills through 62 specific strategies.
- Practical Guidance: It offers practical, actionable advice for developers with some JavaScript and TypeScript experience, aiming to elevate their expertise.
- Common Pitfalls: The book addresses common pitfalls and traps, helping readers build a solid understanding of TypeScript and its ecosystem.
Why should I read Effective TypeScript?
- Skill Enhancement: The book helps transition from beginner or intermediate to expert in TypeScript, improving coding practices and language understanding.
- Practical Recipes: It provides practical advice and recipes that can be directly applied to your work, making it valuable for both new and seasoned developers.
- Deep Understanding: Offers insights into TypeScript’s powerful features, helping you write safer and more efficient code.
What are the key takeaways of Effective TypeScript?
- 62 Specific Ways: The book outlines 62 ways to improve TypeScript usage, covering topics from basic syntax to advanced type manipulation.
- Practical Focus: Each item provides actionable advice for day-to-day work, making it a practical guide.
- Type Relationships: Understanding the relationship between TypeScript and JavaScript, and how TypeScript models JavaScript’s runtime behavior, is a significant takeaway.
How does Effective TypeScript address type safety?
- Type Annotations: Emphasizes using type annotations to ensure type safety, catching errors at compile time rather than runtime.
- Strict Null Checks: Advocates for enabling strict null checks to identify potential null or undefined values, leading to safer code.
- Generics: Highlights the use of generics for creating reusable and type-safe components, maintaining type safety across various types.
What is the significance of type inference in Effective TypeScript?
- Automatic Detection: Type inference allows TypeScript to automatically determine variable types, reducing the need for explicit annotations.
- Contextual Understanding: Emphasizes that TypeScript uses context to infer types, which can lead to surprises if not properly understood.
- Avoid Redundancy: Understanding type inference helps avoid unnecessary type annotations, focusing on clear and concise code.
How does Effective TypeScript recommend handling optional properties?
- Optional Properties Use: Discusses defining properties that may or may not be present, useful for modeling real-world scenarios.
- Avoid Ambiguity: Stresses the importance of clarity in handling cases where optional properties are not provided.
- Type Safety: Encourages using TypeScript’s type system to enforce checks on optional properties, ensuring robust code.
How does Effective TypeScript suggest structuring types for valid states?
- Design Valid States: Emphasizes designing types that only represent valid states, preventing errors and simplifying code understanding.
- Tagged Unions: Suggests using tagged unions to model different states explicitly, ensuring well-defined state transitions.
- Avoid Invalid Combinations: Structuring types to avoid invalid combinations simplifies logic and reduces runtime errors.
What are tagged unions, and why are they important in Effective TypeScript?
- Definition: Tagged unions, or discriminated unions, define a type that can be one of several types, each with a common "tag" property.
- Type Safety: They improve type safety by ensuring only valid property combinations are allowed, preventing errors from invalid states.
- Practical Examples: The book provides examples of implementing tagged unions, aiding in managing complex data structures and control flow.
How does Effective TypeScript address the use of the any
type?
- Limit
any
Use: Advises against overusingany
, as it silences the type checker and can mask real problems. - Encourages Specific Types: Recommends using specific types that accurately represent data, enhancing readability and maintainability.
- Understanding Risks: Highlights risks of
any
, such as breaking function signature contracts and losing type system benefits.
What are some specific methods or advice from Effective TypeScript?
- Push Null Values: Advises structuring types to handle null values cleanly, improving readability and reducing errors.
- Avoid
any
: Strongly advises againstany
types, suggesting more specific types orunknown
for type safety. - Use JSDoc: Recommends JSDoc-style comments for documentation, enhancing developer experience with better tooltips and documentation.
How can I improve my TypeScript code using Effective TypeScript?
- Follow 62 Items: The book's 62 items provide actionable advice for gradually improving TypeScript skills and code quality.
- Practice with Examples: Implement concepts in real-world projects to reinforce understanding and internalize best practices.
- Engage with Community: Encourages participation in the TypeScript community for additional insights and support.
What are the best quotes from Effective TypeScript and what do they mean?
- "TypeScript is a superset of JavaScript.": Emphasizes the foundational relationship, facilitating gradual TypeScript adoption.
- "Avoid using
any
.": Warns againstany
type pitfalls, which undermine TypeScript’s type system benefits. - "Design types that only represent valid states.": Encourages creating types that prevent invalid states, simplifying logic and reducing errors.
Review Summary
Effective TypeScript receives high praise for its comprehensive coverage of TypeScript concepts, from basics to advanced topics. Readers appreciate its clear explanations, practical examples, and insights into TypeScript's type system. Many found it helpful for both beginners and experienced developers, highlighting its value in improving coding practices. The book's structure of concise tips makes it easy to reference. While some readers felt certain sections were less relevant or outdated, most agree it's an essential resource for mastering TypeScript and enhancing JavaScript development skills.
Similar Books
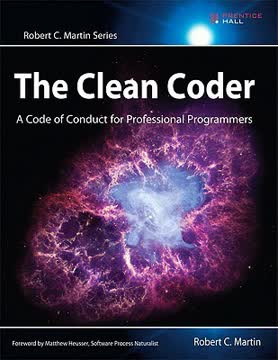
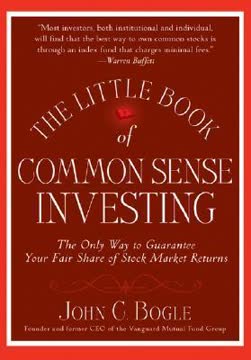
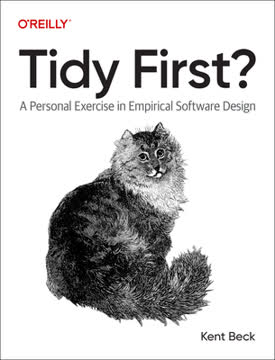
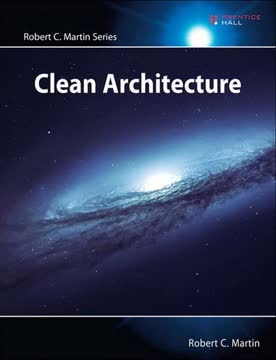
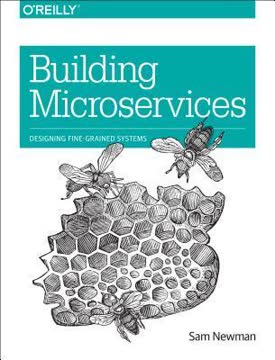
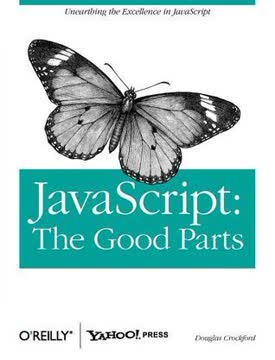
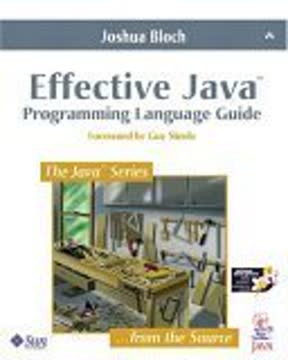
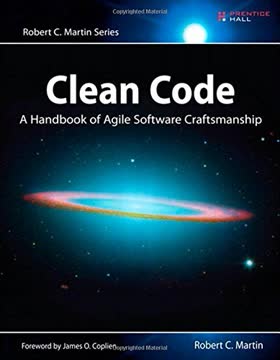
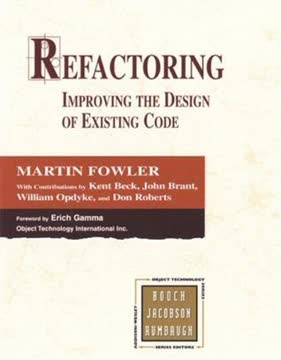
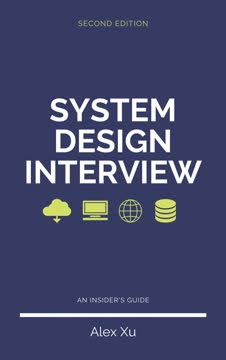
Download PDF
Download EPUB
.epub
digital book format is ideal for reading ebooks on phones, tablets, and e-readers.