Key Takeaways
1. React revolutionized UI development with its declarative approach and virtual DOM
React is called React for a reason: it enables our web applications to react to things.
Declarative paradigm. React introduced a revolutionary approach to building user interfaces by allowing developers to describe what they want to see, rather than imperatively specifying how to achieve it. This declarative paradigm simplifies the development process and makes code more predictable and easier to debug.
Component-based architecture. React encourages breaking down UIs into reusable, self-contained components. This modular approach promotes code reuse, separation of concerns, and easier maintenance. Components can be composed to create complex UIs, with each component managing its own state and lifecycle.
Advantages of React's approach:
- Improved code organization
- Enhanced readability
- Easier testing and debugging
- Scalability for large applications
2. JSX: The innovative syntax that marries HTML and JavaScript
JSX is a syntax extension for JavaScript that allows you to write HTML-like code within your JavaScript code.
Intuitive syntax. JSX allows developers to write HTML-like code directly in their JavaScript files, making it easier to visualize and create UI components. This syntax is more intuitive for developers familiar with HTML and reduces the mental overhead of switching between markup and logic.
Powerful expressions. JSX supports embedding JavaScript expressions within curly braces, enabling dynamic content and logic within the markup. This feature allows for powerful, flexible component creation.
Key features of JSX:
- Familiar HTML-like syntax
- JavaScript expressions support
- Compile-time optimizations
- Type-checking capabilities with TypeScript
3. Virtual DOM: React's secret weapon for efficient updates
The virtual DOM is a programming concept that represents the real DOM but as a JavaScript object.
Efficient updates. The virtual DOM is a lightweight in-memory representation of the actual DOM. React uses this to perform efficient updates by first applying changes to the virtual DOM, then calculating the minimal set of changes needed to update the real DOM.
Performance optimization. By batching DOM updates and minimizing direct manipulation, the virtual DOM significantly improves performance, especially for complex UIs with frequent updates.
Benefits of the virtual DOM:
- Reduced DOM manipulation
- Improved application performance
- Cross-platform compatibility
- Simplified development process
4. Reconciliation: How React efficiently updates the DOM
Reconciliation is the process of comparing the old virtual DOM tree with the new virtual DOM tree and determining which parts of the actual DOM need to be updated.
Efficient diffing. React's reconciliation algorithm efficiently compares the previous and new virtual DOM trees to identify the minimal set of changes required to update the actual DOM. This process, known as diffing, ensures that only necessary updates are applied, minimizing performance overhead.
Key-based optimization. React uses keys to optimize list rendering, allowing it to efficiently track and update list items. This approach significantly improves performance when dealing with dynamic lists.
Key aspects of reconciliation:
- Tree comparison algorithms
- Component-level updates
- Batched DOM mutations
- Key-based list optimization
5. Hooks: Revolutionizing state management and side effects in React
Hooks are a new addition in React 16.8. They let you use state and other React features without writing a class.
Simplified state management. Hooks introduce a more intuitive way to manage state and side effects in functional components. useState and useEffect are the most commonly used hooks, allowing developers to add state and lifecycle-like functionality to functional components.
Reusable logic. Custom hooks enable developers to extract and share stateful logic between components without changing the component hierarchy. This promotes code reuse and helps keep components focused on their primary responsibilities.
Popular React hooks:
- useState: Managing component-level state
- useEffect: Handling side effects
- useContext: Accessing context values
- useReducer: Complex state management
- useMemo and useCallback: Performance optimizations
6. Server-side rendering: Enhancing performance and SEO
Server-side rendering (SSR) is a technique that allows you to render React components on the server rather than in the browser.
Improved initial load time. SSR enables faster initial page loads by rendering the initial HTML on the server, allowing users to see content more quickly. This is particularly beneficial for content-heavy sites and applications targeting users with slower internet connections.
SEO benefits. By rendering content on the server, SSR makes it easier for search engines to crawl and index your pages, potentially improving search engine rankings and discoverability.
Advantages of server-side rendering:
- Faster time-to-content
- Improved SEO performance
- Better user experience on slow connections
- Consistent rendering across devices
7. React Server Components: The future of server-client integration
React Server Components (RSCs) introduce a new type of component that "runs" on the server and is otherwise excluded from the client-side JavaScript bundle.
Optimized performance. RSCs allow developers to keep large dependencies and data fetching on the server, reducing the amount of JavaScript sent to the client. This results in smaller bundle sizes and faster load times for end-users.
Seamless integration. RSCs can be seamlessly integrated with client-side components, allowing developers to create hybrid applications that leverage the strengths of both server-side and client-side rendering.
Key features of React Server Components:
- Reduced client-side JavaScript
- Improved performance
- Seamless server-client integration
- Enhanced data fetching capabilities
8. React frameworks: Simplifying development with opinionated solutions
Frameworks provide a predefined structure and solutions to common problems, allowing developers to focus on what's unique about their application, rather than getting bogged down with boilerplate code.
Streamlined development. React frameworks like Next.js and Remix provide opinionated solutions for common challenges such as routing, server-side rendering, and data fetching. These frameworks can significantly accelerate development and improve productivity.
Best practices enforcement. Frameworks often incorporate best practices and conventions, helping developers create more maintainable and scalable applications. They provide a consistent structure that makes it easier for teams to collaborate and onboard new members.
Popular React frameworks:
- Next.js: Full-stack React framework
- Remix: Web framework with focus on web standards
- Gatsby: Static site generator for React
- Create React App: Simplified React bootstrapping
9. React alternatives: Exploring reactive programming models
Reactive programming models offer some compelling benefits, particularly when it comes to automatically managing dependencies and updates.
Fine-grained reactivity. Frameworks like Vue, Svelte, and Solid use reactive primitives (e.g., signals) to achieve fine-grained updates. This approach can lead to more efficient rendering and simpler state management compared to React's coarse-grained model.
Different paradigms. Each framework offers its own unique approach to building user interfaces, with varying levels of abstraction, performance characteristics, and developer experience.
Notable React alternatives:
- Vue.js: Progressive JavaScript framework
- Svelte: Compile-time framework
- Solid: Fine-grained reactivity framework
- Angular: Full-featured framework by Google
- Qwik: Resumable framework for instant loading
10. The evolution of React: Adapting to changing paradigms
React's model of handling state and updates provides an excellent balance between control and convenience.
Continuous innovation. React has consistently evolved to address developer needs and industry trends. From the introduction of hooks to the development of React Server Components, the library continues to adapt and improve.
Performance focus. While React's core model differs from newer reactive approaches, the team is actively working on performance optimizations. Projects like React Forget aim to provide similar benefits to signals without changing React's fundamental model.
Recent and upcoming React features:
- Concurrent rendering
- Suspense for data fetching
- React Server Components
- Automatic batching of state updates
- React Forget: Compiler-based optimizations
Last updated:
Review Summary
Fluent React receives mixed reviews, with an overall rating of 4.18/5. Readers appreciate its in-depth coverage of React internals, Fiber, and reconciliation algorithms. However, some find certain chapters irrelevant or biased. The book is praised for its comprehensive approach but criticized for including both beginner and advanced content. While valuable for understanding React's inner workings, it's not recommended for newcomers. Reviewers suggest it's an essential read for experienced React developers looking to deepen their knowledge, despite some structural and content criticisms.
Similar Books
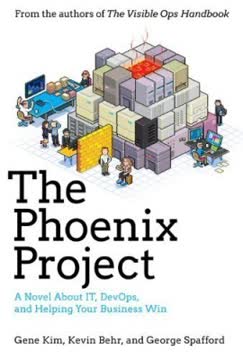
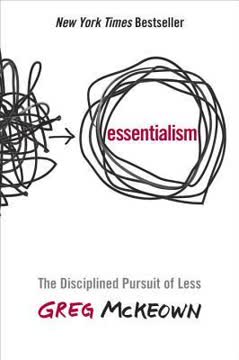
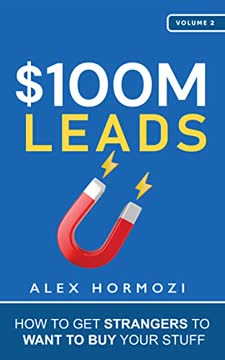
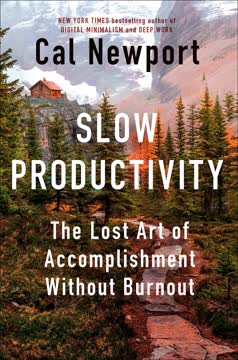
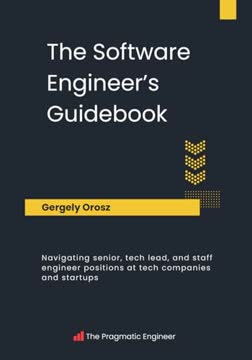
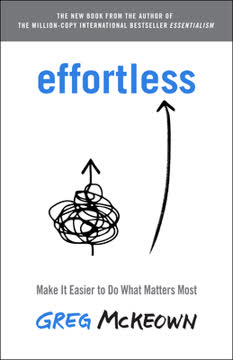
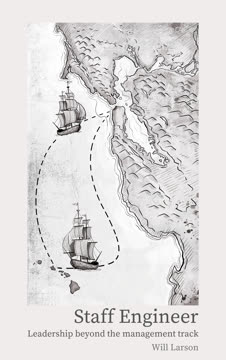
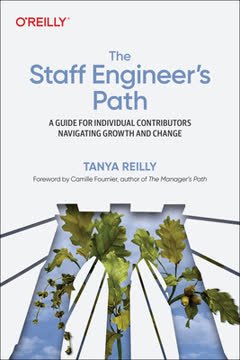
Download PDF
Download EPUB
.epub
digital book format is ideal for reading ebooks on phones, tablets, and e-readers.