Key Takeaways
1. Algorithms: The bedrock of efficient problem-solving.
An algorithm is a set of instructions for accomplishing a task.
Algorithms defined. At its core, an algorithm is simply a well-defined procedure or set of rules designed to solve a specific problem. They are the fundamental building blocks of computer programs, dictating how data is processed and manipulated to achieve a desired outcome. The choice of algorithm significantly impacts the efficiency and performance of a program, making algorithm selection a critical aspect of software development.
Algorithm examples. Algorithms are not limited to the realm of computer science; they are prevalent in everyday life. For example:
- A recipe for baking a cake is an algorithm.
- Driving directions from one location to another is an algorithm.
- The steps for assembling furniture is an algorithm.
Importance of algorithms. Understanding algorithms is crucial for programmers because it enables them to write efficient and effective code. By selecting the right algorithm for a given task, developers can optimize performance, reduce resource consumption, and improve the overall user experience.
2. Binary Search: A logarithmic time marvel.
With binary search, you guess the middle number and eliminate half the remaining numbers every time.
Binary search defined. Binary search is an efficient algorithm for finding a specific value within a sorted list. It works by repeatedly dividing the search interval in half. If the middle element matches the target value, the search is successful. Otherwise, the search continues in either the left or right half of the interval, depending on whether the target value is less than or greater than the middle element.
Logarithmic time complexity. The key advantage of binary search is its logarithmic time complexity, denoted as O(log n). This means that the number of steps required to find the target value increases logarithmically with the size of the list. For example:
- A list of 1,024 elements requires at most 10 steps.
- A list of 1,048,576 elements requires at most 20 steps.
Practical applications. Binary search is widely used in various applications where efficient searching is essential, including:
- Searching for a word in a dictionary.
- Finding a contact in a phone book.
- Looking up data in a sorted database index.
3. Arrays vs. Linked Lists: Choosing the right structure.
With an array, all your elements are stored right next to each other.
Arrays and linked lists defined. Arrays and linked lists are two fundamental data structures used to store collections of elements. Arrays store elements in contiguous memory locations, while linked lists store elements in non-contiguous locations, with each element pointing to the next element in the sequence. The choice between arrays and linked lists depends on the specific requirements of the application.
Arrays advantages. Arrays offer fast random access to elements, meaning that any element can be accessed directly using its index in O(1) time. This makes arrays suitable for applications where frequent element lookups are required.
Linked lists advantages. Linked lists excel at insertions and deletions, particularly in the middle of the list. Inserting or deleting an element in a linked list only requires updating the pointers of the adjacent elements, which can be done in O(1) time. This makes linked lists suitable for applications where frequent insertions and deletions are required.
4. Recursion: Elegance in self-reference.
Recursion is where a function calls itself.
Recursion defined. Recursion is a powerful programming technique where a function calls itself within its own definition. This allows complex problems to be broken down into smaller, self-similar subproblems, which can be solved recursively until a base case is reached.
Base case and recursive case. Every recursive function must have two essential components:
- A base case, which specifies when the recursion should stop.
- A recursive case, which defines how the function should call itself with a smaller subproblem.
Call stack. Recursive functions rely on the call stack to keep track of the state of each function call. Each time a function calls itself, a new frame is added to the call stack, storing the function's variables and execution context. When the base case is reached, the function returns, and the frames are popped off the call stack in reverse order.
5. Quicksort: Divide, conquer, and sort efficiently.
Quicksort is a sorting algorithm.
Quicksort defined. Quicksort is a popular and efficient sorting algorithm that employs the divide-and-conquer paradigm. It works by selecting a "pivot" element from the array and partitioning the other elements into two sub-arrays, according to whether they are less than or greater than the pivot. The sub-arrays are then recursively sorted, and the sorted sub-arrays are combined with the pivot to produce the final sorted array.
Divide and conquer. Quicksort exemplifies the divide-and-conquer strategy, which involves breaking down a problem into smaller, self-similar subproblems, solving the subproblems recursively, and then combining the solutions to solve the original problem.
Average case performance. Quicksort has an average time complexity of O(n log n), making it one of the fastest sorting algorithms in practice. However, its worst-case time complexity is O(n^2), which can occur when the pivot is consistently chosen poorly. To mitigate this risk, it is common to choose the pivot randomly.
6. Hash Tables: The power of key-value lookups.
A hash table maps keys to values.
Hash tables defined. Hash tables are a powerful data structure that allows for efficient storage and retrieval of key-value pairs. They work by using a hash function to map each key to an index in an array, where the corresponding value is stored. This allows for constant-time (O(1)) average-case lookup, insertion, and deletion operations.
Hash functions. The performance of a hash table depends heavily on the quality of the hash function. A good hash function should distribute keys evenly across the array to minimize collisions, which occur when two or more keys map to the same index.
Use cases. Hash tables are widely used in various applications where efficient key-value lookups are essential, including:
- Implementing dictionaries and symbol tables.
- Caching data for faster retrieval.
- Indexing databases for efficient searching.
7. Breadth-First Search: Navigating networks with ease.
Breadth-first search tells you if there’s a path from A to B.
Breadth-first search defined. Breadth-first search (BFS) is a graph traversal algorithm that explores a graph level by level, starting from a given source node. It systematically visits all the neighbors of the source node, then all the neighbors of those neighbors, and so on, until the target node is found or the entire graph has been explored.
Shortest path. BFS is guaranteed to find the shortest path between two nodes in an unweighted graph, where the shortest path is defined as the path with the fewest edges.
Queues. BFS uses a queue to keep track of the nodes to be visited. The queue ensures that nodes are visited in the order they were discovered, which is essential for finding the shortest path.
8. Dijkstra's Algorithm: Finding the shortest weighted path.
Dijkstra’s algorithm is used to calculate the shortest path for a weighted graph.
Dijkstra's algorithm defined. Dijkstra's algorithm is a graph search algorithm that solves the single-source shortest path problem for a graph with non-negative edge weights, producing a shortest path tree. This means, given a source node, the algorithm finds the shortest path between that node and every other.
Weighted graphs. Unlike breadth-first search, Dijkstra's algorithm can handle weighted graphs, where each edge has a numerical value associated with it, representing its cost or distance.
Directed acyclic graphs. Dijkstra's algorithm only works with directed acyclic graphs (DAGs), which are graphs that have no cycles and where all edges have a direction.
9. Greedy Algorithms: Quick, approximate solutions.
Greedy algorithms optimize locally, hoping to end up with a global optimum.
Greedy algorithms defined. Greedy algorithms are a simple and intuitive problem-solving approach that involves making the locally optimal choice at each step, with the hope of finding a globally optimal solution. They are often used when finding the exact solution is computationally expensive or impossible.
Approximation algorithms. Greedy algorithms are often used as approximation algorithms, which provide a solution that is close to the optimal solution but may not be exactly optimal.
Set covering problem. A classic example of a problem where greedy algorithms are useful is the set covering problem, which involves finding the smallest set of subsets that cover all the elements in a given set.
10. Dynamic Programming: Optimizing through subproblems.
Dynamic programming is useful when you’re trying to optimize something given a constraint.
Dynamic programming defined. Dynamic programming is a powerful problem-solving technique that involves breaking down a complex problem into smaller, overlapping subproblems, solving each subproblem only once, and storing the solutions in a table or memo to avoid recomputation. This approach can significantly improve the efficiency of algorithms for problems that exhibit optimal substructure and overlapping subproblems.
Knapsack problem. A classic example of a problem that can be solved using dynamic programming is the knapsack problem, which involves selecting a subset of items with maximum value that can fit into a knapsack with a limited capacity.
Grid. Every dynamic-programming solution involves a grid. The values in the cells are usually what you’re trying to optimize. Each cell is a subproblem, so think about how you can divide your problem into subproblems.
11. K-Nearest Neighbors: Learning from your neighbors.
KNN is used for classification and regression and involves looking at the k-nearest neighbors.
K-nearest neighbors defined. K-nearest neighbors (KNN) is a simple yet effective machine learning algorithm that can be used for both classification and regression tasks. It works by finding the k nearest data points to a given query point and then predicting the class or value of the query point based on the majority class or average value of its neighbors.
Classification and regression. KNN can be used for both classification (categorizing data points into different classes) and regression (predicting a continuous value).
Feature extraction. Feature extraction is the process of converting raw data into a set of numerical features that can be used by the KNN algorithm. The choice of features is crucial for the performance of the algorithm.
Last updated:
Review Summary
Grokking Algorithms An Illustrated Guide For Programmers and Other Curious People receives high praise for its approachable, visual approach to teaching algorithms. Readers appreciate its simplicity, engaging illustrations, and clear explanations, making it an excellent introduction for beginners and a refresher for experienced programmers. The book covers fundamental algorithms and data structures, with many finding it enjoyable and easy to understand. Some criticisms include uneven difficulty levels, occasional errors, and a lack of depth in certain topics. Overall, it's widely recommended as a friendly, accessible guide to algorithms.
Similar Books
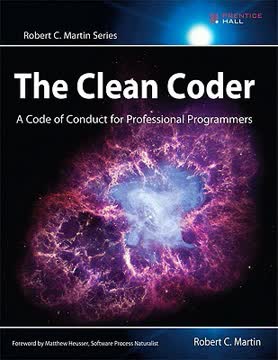
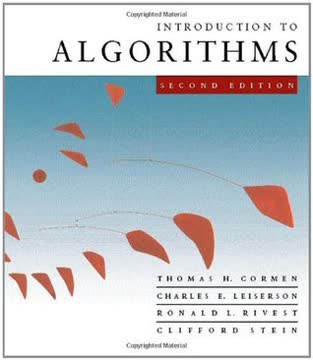
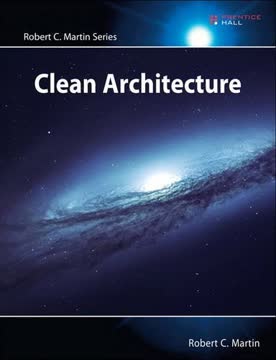


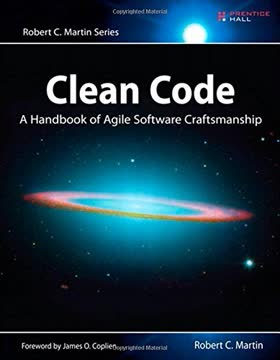
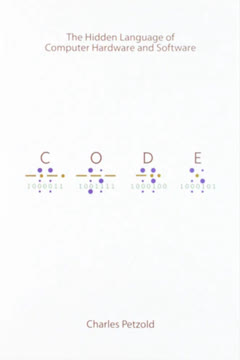
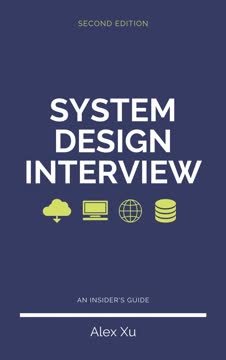
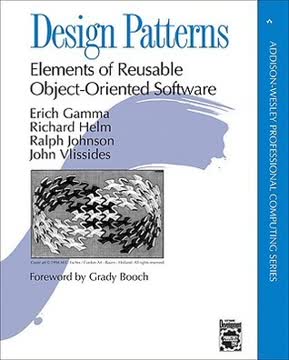