Key Takeaways
1. Java's Foundation: Classes, Objects, and the JVM
Kathy and Bert’s ‘Head First Java’ transforms the printed page into the closest thing to a GUI you’ve ever seen.
Classes and Objects. Java is fundamentally object-oriented, meaning everything revolves around classes and objects. A class serves as a blueprint, defining the structure and behavior of objects. Objects, on the other hand, are instances of a class, each possessing its own unique state (data) and the ability to perform actions (methods).
The Java Virtual Machine. The JVM is the engine that executes Java bytecode, the compiled form of Java source code. This bytecode is platform-independent, allowing Java applications to run on any device with a JVM. The JVM is responsible for memory management, including garbage collection, which automatically reclaims memory occupied by objects that are no longer in use.
Compilation and Execution. The Java development process involves writing source code in .java
files, compiling it into bytecode using the javac
compiler, and then running the bytecode on the JVM using the java
command. The JVM interprets the bytecode and translates it into machine code that the underlying operating system can understand and execute.
2. Variables: Primitives, References, and Memory
Variables come in two flavors: primitive and reference.
Primitive Types. Java offers eight primitive data types for storing basic values: boolean
, char
, byte
, short
, int
, long
, float
, and double
. Each primitive type has a specific size and range of values it can hold. Primitive variables store the actual values directly in memory.
Reference Variables. Reference variables, on the other hand, do not store the object itself. Instead, they hold a reference, a pointer, or an address that indicates where the object is located in memory (on the heap). Multiple reference variables can point to the same object.
The Heap and the Stack. Objects are created and stored on the heap, a region of memory managed by the JVM's garbage collector. Local variables, including method parameters, are stored on the stack, a region of memory used for method invocations and temporary data. Understanding the distinction between the heap and the stack is crucial for understanding memory management and object lifecycles in Java.
3. Methods: Behavior, Arguments, and Return Types
Methods use object state (bark different).
Methods and Object Behavior. Methods define the actions that an object can perform. They operate on the object's state, represented by its instance variables. The behavior of a method can vary depending on the object's state.
Arguments and Parameters. Methods can accept arguments, which are values passed into the method when it is called. The method declares parameters, which are local variables that receive the argument values. Java is pass-by-value, meaning that the argument value is copied into the parameter variable.
Return Types. Methods can also return values, which are sent back to the caller of the method. The return type of a method must be declared in the method signature. If a method does not return a value, its return type is void
.
4. Leveraging the Java API: Pre-Built Classes and Packages
You don’t have to reinvent the wheel if you know how to find what you need from the Java library, commonly known as the Java API.
The Java API. The Java API is a vast collection of pre-built classes and interfaces that provide a wide range of functionality, from basic data structures to networking and GUI components. By leveraging the API, developers can avoid reinventing the wheel and focus on building custom features for their applications.
Packages. Classes in the Java API are organized into packages, which are namespaces that help prevent naming collisions and provide a logical structure for the library. To use a class from the API, you must either import the package containing the class or use the fully-qualified name of the class.
Exploring the API. The Java API documentation is an invaluable resource for learning about the available classes and methods. The documentation provides detailed information about each class, including its purpose, methods, and usage examples. Reference books and online resources can also be helpful for exploring the API.
5. Object-Oriented Design: Inheritance and Polymorphism
When you get on the Polymorphism Plan, you’ll learn the 5 steps to better class design, the 3 tricks to polymorphism, the 8 ways to make flexible code, and if you act now—a bonus lesson on the 4 tips for exploiting inheritance.
Inheritance. Inheritance allows you to create new classes (subclasses) that inherit the properties and behaviors of existing classes (superclasses). This promotes code reuse and reduces redundancy. Subclasses can add new methods and instance variables, as well as override methods inherited from the superclass.
Polymorphism. Polymorphism enables you to treat objects of different classes in a uniform way. This is achieved through inheritance and interfaces. A superclass reference can refer to an object of any of its subclasses, and you can call methods on that reference without knowing the exact type of the object.
IS-A Relationship. The IS-A test is a useful guideline for determining whether inheritance is appropriate. If it makes sense to say "A is a B," then class A should inherit from class B. For example, a Dog
IS-A Animal
, so Dog
should extend Animal
.
6. Abstract Classes and Interfaces: Achieving Flexibility
Inheritance is just the beginning.
Abstract Classes. Abstract classes cannot be instantiated directly. They serve as blueprints for subclasses, defining common properties and behaviors. Abstract classes can contain both abstract methods (methods without implementation) and concrete methods (methods with implementation).
Interfaces. Interfaces define a contract that classes can implement. An interface specifies a set of methods that a class must implement if it claims to implement the interface. Interfaces cannot contain instance variables or concrete methods (except for default methods, introduced in Java 8).
Polymorphism with Interfaces. Interfaces provide a powerful way to achieve polymorphism. You can declare a reference variable of an interface type and assign it an object of any class that implements that interface. This allows you to write code that works with objects of different classes in a uniform way.
7. Exception Handling: Managing Risky Operations
Exceptions say “something bad may have happened...”
Exceptions. Exceptions are events that disrupt the normal flow of a program's execution. They typically indicate errors or exceptional conditions that the program cannot handle in the usual way. Java provides a robust exception-handling mechanism to deal with these situations.
Try-Catch Blocks. To handle exceptions, you can enclose risky code in a try
block. If an exception occurs within the try
block, the program jumps to the corresponding catch
block, where you can take appropriate action, such as logging the error, displaying a message to the user, or attempting to recover from the problem.
Checked and Unchecked Exceptions. Java distinguishes between checked and unchecked exceptions. Checked exceptions must be either handled in a catch
block or declared in the method signature using the throws
keyword. Unchecked exceptions, which are subclasses of RuntimeException
, do not require explicit handling or declaration.
8. Swing and GUIs: Creating Interactive Applications
Face it, you need to make GUIs.
Swing Components. Swing is a GUI toolkit that provides a rich set of components for building interactive applications. Swing components are lightweight, platform-independent, and highly customizable.
Layout Managers. Layout managers are responsible for arranging components within a container, such as a JFrame or JPanel. Different layout managers have different policies for determining the size and position of components. Common layout managers include BorderLayout, FlowLayout, and BoxLayout.
Event Handling. Event handling is the process of responding to user actions, such as button clicks, mouse movements, and keyboard input. To handle events, you must implement a listener interface, register the listener with the event source, and define the event-handling method.
9. Data Structures: Collections and Generics
Sorting is a snap in Java.
Collections Framework. The Java Collections Framework provides a set of interfaces and classes for storing and manipulating groups of objects. Common collection types include List, Set, and Map.
Generics. Generics allow you to specify the type of objects that a collection can hold. This improves type safety and reduces the need for casting. For example, ArrayList<String>
declares a list that can hold only String objects.
Sorting. The Collections.sort()
method provides a convenient way to sort lists. To sort objects of a custom class, the class must implement the Comparable
interface or you must provide a custom Comparator
.
10. Packaging and Deployment: Releasing Your Code
It’s time to let go.
JAR Files. A JAR (Java Archive) file is a compressed archive that bundles all the class files, resources, and metadata for a Java application into a single file. JAR files make it easier to distribute and deploy Java applications.
Executable JARs. To make a JAR file executable, you must include a manifest file that specifies the main class of the application. The manifest file is a simple text file that contains key-value pairs.
Java Web Start. Java Web Start (JWS) is a technology that allows you to deploy stand-alone Java applications over the Web. JWS applications are launched from a web browser and run outside the browser sandbox, providing access to system resources.
11. Distributed Computing: RMI and Remote Services
Being remote doesn’t have to be a bad thing.
Remote Method Invocation (RMI). RMI is a Java API that allows you to invoke methods on objects running in a different JVM, potentially on a different machine. RMI simplifies the process of building distributed applications.
RMI Architecture. RMI involves several key components: the remote interface, the remote implementation, the stub (client-side proxy), and the skeleton (server-side proxy). The client interacts with the stub, which handles the communication with the server. The server receives the request and invokes the method on the remote implementation.
RMI Registry. The RMI registry is a naming service that allows clients to locate remote objects. The server registers its remote objects with the registry, and clients can look up those objects by name.
Last updated:
FAQ
1. What is "Head First Java" by Kathy Sierra about?
- Engaging Java introduction: "Head First Java" by Kathy Sierra is an interactive, visually rich guide to learning Java programming, designed to make complex concepts accessible and memorable.
- Comprehensive coverage: The book covers Java fundamentals, object-oriented programming, GUI development, exception handling, networking, collections, generics, and deployment.
- Active learning approach: It uses puzzles, stories, quizzes, and hands-on projects to reinforce learning, based on cognitive science and educational psychology principles.
- Real-world applications: Readers build practical projects like chat clients, music sequencers, and service browsers, gaining skills for real Java development.
2. Why should I read "Head First Java" by Kathy Sierra?
- Unique teaching style: The book transforms learning into an interactive experience, using humor, visuals, and analogies to make Java concepts stick.
- Covers essential topics: It goes beyond syntax, teaching design principles, object-oriented thinking, and practical skills needed for professional Java programming.
- Hands-on learning: Numerous exercises, code puzzles, and projects help readers apply concepts immediately and retain knowledge.
- Industry praise: Java experts and leaders recommend it as an essential resource for both beginners and those looking to deepen their Java skills.
3. What are the key takeaways from "Head First Java" by Kathy Sierra?
- Think like a Java programmer: The book emphasizes understanding how Java works under the hood, including memory management, object lifecycle, and design patterns.
- Master core Java concepts: Readers gain a solid foundation in object-oriented programming, exception handling, GUI development, collections, and multithreading.
- Practical application focus: Through real-world projects and exercises, the book ensures readers can build, debug, and deploy Java applications confidently.
- Active, metacognitive learning: The teaching approach encourages readers to reflect on their learning process, promoting deeper understanding and long-term retention.
4. How does "Head First Java" by Kathy Sierra explain object-oriented programming concepts?
- Classes and objects: The book defines classes as blueprints for objects, which have state (instance variables) and behavior (methods), and explains how objects are created and interact.
- Inheritance and polymorphism: It covers how subclasses inherit from superclasses, enabling code reuse, and how polymorphism allows objects to be treated as their superclass or interface type.
- Encapsulation: The importance of hiding data with private variables and accessing them through public getters and setters is stressed to protect object integrity.
- Design principles: The book introduces the IS-A test for inheritance and encourages using interfaces for shared behavior across unrelated classes.
5. What does "Head First Java" by Kathy Sierra teach about variables, data types, and memory management?
- Primitive vs. reference types: Variables can be primitives (like int, boolean) holding actual values, or references pointing to objects on the heap.
- Type safety: Java enforces strict type declarations, preventing errors like assigning a Giraffe to a Rabbit variable, and uses generics for safer collections.
- Stack vs. heap: Local variables live on the stack and disappear after method execution, while objects and instance variables reside on the heap until no references remain.
- Garbage collection: The book explains how Java automatically reclaims memory for objects that are no longer referenced, helping manage resources efficiently.
6. How does "Head First Java" by Kathy Sierra explain methods, parameters, and encapsulation?
- Methods and object state: Methods operate on an object's instance variables, and their behavior can change based on the object's current state.
- Parameters and return values: Methods can accept parameters (arguments) and return values, with Java using pass-by-value for both primitives and object references.
- Getters and setters: The book advocates using getter and setter methods to access and modify private instance variables, supporting encapsulation and data validation.
- Static and final keywords: It explains static methods/variables as class-level (shared) and final as unchangeable, with static final used for constants.
7. What practical advice does "Head First Java" by Kathy Sierra give about inheritance, interfaces, and design?
- IS-A test for inheritance: Only use inheritance when a true "is-a" relationship exists between subclass and superclass to maintain logical hierarchies.
- Interfaces for roles: Interfaces define method contracts for shared behavior, allowing unrelated classes to implement common capabilities without multiple inheritance.
- Abstract classes: Abstract classes provide a template with both abstract and concrete methods, serving as a base for subclasses but cannot be instantiated directly.
- Avoiding inheritance abuse: The book warns against extending classes just for code reuse if the IS-A relationship doesn't hold, suggesting composition or interfaces instead.
8. How does "Head First Java" by Kathy Sierra approach exception handling and risky behavior?
- Checked vs. unchecked exceptions: The book distinguishes between checked exceptions (which must be handled or declared) and unchecked exceptions (like RuntimeException) that do not require explicit handling.
- Try/catch/finally structure: It teaches wrapping risky code in try blocks, handling exceptions in catch blocks, and using finally for cleanup tasks that must always run.
- Ducking exceptions: Methods can declare exceptions in their signatures to pass responsibility up the call stack, but some code must eventually handle them.
- Polymorphic exception handling: Catch blocks can use superclass types for exceptions, but writing specific catch blocks for different exception types is recommended for precise handling.
9. What does "Head First Java" by Kathy Sierra teach about Java GUI programming with Swing?
- Swing components and layout managers: The book introduces essential Swing components like JFrame, JPanel, JButton, and layout managers such as BorderLayout and BoxLayout for building user interfaces.
- Event-driven programming: It explains how to use event listeners (like ActionListener) and inner classes to handle user actions such as button clicks.
- Custom graphics and animation: Readers learn to create custom drawing panels by subclassing JPanel and overriding paintComponent(), enabling advanced graphics and interactive animations.
- Combining GUI and logic: The book demonstrates integrating GUI events with program logic, such as updating graphics in response to user actions.
10. How does "Head First Java" by Kathy Sierra explain Java Collections and Generics?
- Collections overview: The book covers key collection types like ArrayList, HashSet, TreeSet, and HashMap, explaining their use cases for storing, sorting, and managing data.
- Generics for type safety: Generics allow collections to enforce compile-time type checks, preventing runtime errors and eliminating the need for casting.
- Common operations: Readers learn to use methods like add(), remove(), size(), and isEmpty() for manipulating collections, and how to use generics with collections for safer code.
- Sorting and comparators: The book explains implementing Comparable and Comparator interfaces to sort custom objects by different attributes.
11. What networking and multithreading concepts does "Head First Java" by Kathy Sierra cover?
- Socket programming basics: The book explains how to create client and server sockets, read and write data streams, and build simple chat applications.
- Thread creation and management: It teaches creating threads by implementing Runnable, starting them with Thread objects, and managing thread states and scheduling.
- Synchronization and thread safety: The importance of using the synchronized keyword to protect shared data and prevent race conditions is emphasized.
- Combining networking and threads: Practical examples show how to use threads in networked applications to keep GUIs responsive and handle concurrent communication.
12. What are the best quotes and notable advice from "Head First Java" by Kathy Sierra, and what do they mean?
- On inheritance: "Roses are red, violets are blue. Square is-a Shape, the reverse isn’t true." — Highlights the one-way nature of inheritance; subclasses inherit from superclasses, not vice versa.
- On generics: "Generics means more type-safety... code that makes the compiler stop you from putting a Dog into a list of Ducks." — Emphasizes the importance of generics for preventing type errors.
- On synchronization: "Use the synchronized keyword to modify a method so that only one thread at a time can access it." — Stresses the need for synchronization in multithreaded code.
- On packages: "Package structure is like the full name of a class, technically known as the fully-qualified name." — Explains the role of packages in organizing and uniquely identifying classes.
- On atomicity: "Think Newton, not Einstein, when you hear the word ‘atomic’ in the context of threads or transactions." — Encourages thinking of atomicity as indivisible operations, not complex physics.
Review Summary
Head First Java receives overwhelmingly positive reviews for its unique, visual approach to teaching Java programming. Readers praise its engaging style, clear explanations, and effective learning techniques. Many find it ideal for beginners and those transitioning from other languages. The book's use of humor, graphics, and unconventional methods helps concepts stick. While some critics find it too basic or distracting, most appreciate its ability to make complex topics accessible and enjoyable. Reviewers often mention retaining information long after reading and recommend it as an excellent introduction to Java.
Similar Books
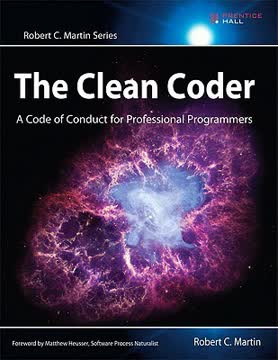
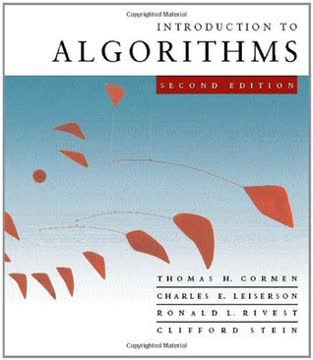
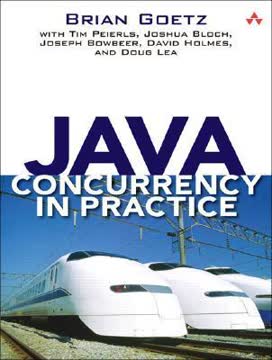
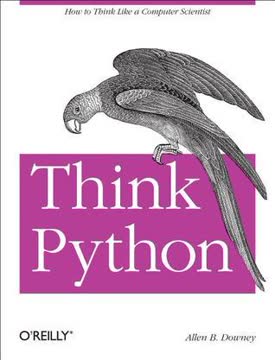
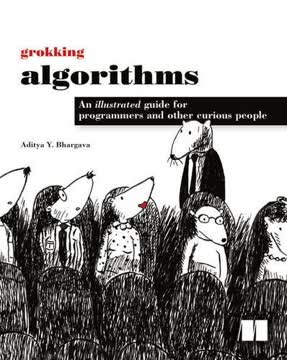

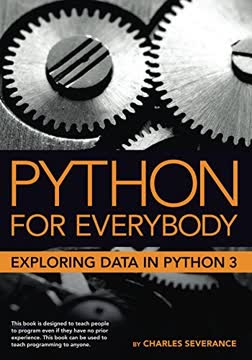
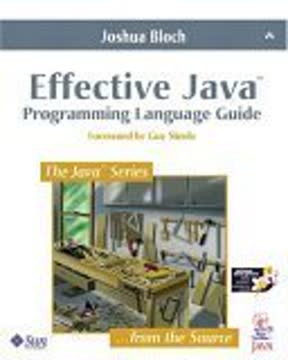
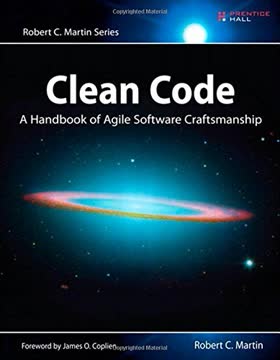
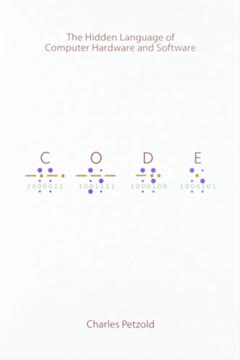
Download PDF
Download EPUB
.epub
digital book format is ideal for reading ebooks on phones, tablets, and e-readers.