Key Takeaways
1. Java 8 introduces lambda expressions and functional interfaces for concise, flexible code
Lambda expressions can make your code more readable and flexible.
Concise syntax. Lambda expressions provide a way to pass behavior as a method argument, enabling more flexible and reusable code. They are essentially anonymous functions that can be used wherever a functional interface is expected. Functional interfaces are interfaces with a single abstract method, such as Predicate, Function, and Consumer.
Improved readability. By using lambda expressions, you can write more expressive and readable code. For example, instead of verbose anonymous inner classes, you can use compact lambda syntax:
- Before: new Comparator<Apple>() { public int compare(Apple a1, Apple a2) { return a1.getWeight().compareTo(a2.getWeight()); } }
- After: (Apple a1, Apple a2) -> a1.getWeight().compareTo(a2.getWeight())
Method references. Java 8 also introduces method references as a shorthand notation for lambdas that simply call an existing method. This further enhances code readability and reusability.
2. The Streams API enables declarative, parallelizable data processing
Streams can be processed in parallel transparently, without you having to write any multithreaded code!
Declarative programming. The Streams API allows you to express complex data processing queries in a declarative way. Instead of writing how to perform operations step-by-step, you describe what you want to achieve. This leads to more concise and readable code.
Parallelism. Streams can be easily parallelized to take advantage of multicore processors. By simply changing stream() to parallelStream(), you can potentially improve performance without changing the logic of your code.
Lazy evaluation. Stream operations are lazily evaluated, meaning that computation on the source data is only performed when the terminal operation is initiated. This can lead to significant performance improvements, especially when dealing with large datasets.
Key Stream operations include:
- Intermediate: filter, map, flatMap, distinct, sorted, limit
- Terminal: forEach, count, collect, reduce, find, match
3. Default methods in interfaces allow API evolution without breaking existing implementations
Default methods help library designers evolve APIs in a backward-compatible way.
API evolution. Default methods allow you to add new methods to interfaces without breaking existing implementations. This feature was primarily introduced to evolve the Java collections API, enabling the addition of new methods like stream() to existing interfaces.
Multiple inheritance of behavior. With default methods, Java now supports a limited form of multiple inheritance of behavior. Interfaces can provide implementation code, not just method signatures.
Resolution rules. When a class implements multiple interfaces with conflicting default methods, Java 8 provides clear resolution rules:
- Classes always win over interfaces
- More specific interfaces win over less specific ones
- If there's still a conflict, the class must explicitly override the method
4. Optional class improves null handling and API design
Using Optional can help you design better APIs in which, just by reading the signature of a method, users can tell whether to expect an optional value.
Null alternative. The Optional class provides a better way to represent the absence of a value, rather than using null references. This helps prevent NullPointerExceptions and makes code more readable.
API design. By using Optional in method signatures, you explicitly communicate that a value might be absent. This leads to better API design and forces users to consider the case where a value is not present.
Optional provides several methods for safe handling:
- isPresent() to check if a value exists
- ifPresent(Consumer<T>) to execute an action if a value is present
- orElse(T) to provide a default value
- orElseGet(Supplier<T>) to lazily provide a default value
- map(Function<T,U>) and flatMap(Function<T,Optional<U>>) for transformations
5. New Date and Time API addresses shortcomings of previous date/time classes
The date-time objects of the new Date and Time API are all immutable.
Immutability. The new java.time package introduces immutable date-time classes, which are thread-safe and prevent many common programming errors associated with mutable date-time classes.
Separation of concerns. The API clearly separates:
- Human-readable time (LocalDate, LocalTime, LocalDateTime)
- Machine time (Instant)
- Time zones (ZonedDateTime)
- Time periods (Duration, Period)
Fluent API. The new classes provide a fluent API for performing operations, making date and time manipulation more intuitive and less error-prone. For example:
java
LocalDate date = LocalDate.of(2023, Month.MAY, 15)
.plusDays(7)
.minusMonths(1);
6. CompletableFuture facilitates asynchronous and reactive programming
CompletableFuture allows you to express complex asynchronous computations in a declarative way.
Asynchronous programming. CompletableFuture extends the Future interface, providing a way to perform asynchronous computations and combine their results. This is particularly useful for building responsive applications and efficiently utilizing system resources.
Composability. CompletableFuture allows you to chain multiple asynchronous operations, making it easy to express complex workflows. Methods like thenApply, thenCompose, and thenCombine enable you to transform and combine results of asynchronous computations.
Key features:
- Non-blocking operations
- Exception handling with exceptionally and handle methods
- Ability to combine multiple futures with allOf and anyOf
- Support for timeout handling
7. Functional programming concepts enhance Java's expressiveness and maintainability
Functional-style programming promotes side-effect-free methods and declarative programming.
Immutability. Functional programming emphasizes the use of immutable data structures, which leads to more predictable and maintainable code. In Java 8, this is supported through the use of final variables and immutable collections.
Higher-order functions. Java 8 introduces the ability to pass functions as arguments and return them as results, enabling powerful abstractions and code reuse. This is achieved through lambda expressions and method references.
Declarative style. Functional programming encourages a declarative style of programming, where you describe what you want to achieve rather than how to achieve it. This often leads to more concise and readable code.
Key functional programming concepts in Java 8:
- Pure functions (methods without side effects)
- Function composition
- Lazy evaluation (as seen in the Streams API)
- Recursion as an alternative to iteration
8. Scala comparison highlights Java 8's functional programming capabilities and limitations
Scala provides a larger set of features around these ideas compared to Java 8.
Syntactic differences. Scala offers more concise syntax for many operations, such as creating and working with collections. For example, creating a map in Scala:
scala
val authorsToAge = Map("Raoul" -> 23, "Mario" -> 40, "Alan" -> 53)
Advanced functional features. Scala provides more advanced functional programming features, including:
- Pattern matching
- Lazy evaluation
- Currying
- Traits (similar to Java interfaces but with more capabilities)
Type system. Scala has a more advanced type system, including features like:
- Type inference
- Higher-kinded types
- Implicit conversions
While Java 8 introduces many functional programming concepts, it still maintains backward compatibility and a more gradual learning curve compared to Scala's more comprehensive functional programming approach.
Last updated:
FAQ
What’s Java 8 in Action about?
- Focus on Java 8 Features: Java 8 in Action by Raoul-Gabriel Urma explores the significant changes introduced in Java 8, such as lambdas, streams, and functional programming.
- Practical Examples: The book includes practical examples and exercises to help readers apply these new features in real-world scenarios.
- Evolution of Java: It discusses the evolution of Java and the importance of these changes for modern programming practices.
Why should I read Java 8 in Action?
- Enhance Programming Skills: The book helps Java developers upgrade their skills with the latest features in Java 8, making them more proficient.
- Comprehensive Guide: It serves as a comprehensive guide with clear explanations, suitable for both beginners and experienced programmers.
- Stay Updated: Understanding Java 8 is crucial for staying relevant in the evolving tech landscape, and this book ensures you are up-to-date.
What are the key takeaways of Java 8 in Action?
- Lambdas and Streams: Learn how to use lambda expressions and the Streams API for writing concise and efficient Java code.
- Behavior Parameterization: The book emphasizes writing flexible and reusable code through behavior parameterization.
- Functional Programming Concepts: Gain insights into integrating functional programming techniques into Java.
How does Java 8 in Action explain lambda expressions?
- Concise Syntax: Lambda expressions provide a concise way to represent anonymous functions, allowing code to be passed as arguments.
- Functional Interfaces: They are used in the context of functional interfaces, which define a single abstract method that the lambda implements.
- Type Inference: Java compiler infers parameter types in lambda expressions, making the code cleaner and more readable.
What is the Streams API in Java 8 in Action?
- Data Processing: The Streams API processes sequences of elements in a functional style, supporting operations like filtering, mapping, and reducing.
- Lazy Evaluation: Streams are evaluated lazily, performing computations only when necessary, which can improve performance.
- Parallel Processing: The API supports parallel processing, allowing developers to leverage multicore processors efficiently.
How does Java 8 in Action address Optional types?
- Avoiding Nulls: The Optional class represents optional values without using null references, preventing NullPointerExceptions.
- Clearer API Design: Using Optionals indicates when a value may be absent, improving code readability and maintainability.
- Functional Operations: Methods like map, flatMap, and orElse are used to work with Optionals in a functional style.
What is behavior parameterization in Java 8 in Action?
- Definition: Behavior parameterization allows passing blocks of code as parameters to methods, enabling flexible behavior.
- Real-World Examples: The book illustrates this with examples like filtering and sorting collections, adapting to changing requirements.
- Improved Code Flexibility: This approach creates methods that are more adaptable and easier to maintain.
How can I implement asynchronous programming with CompletableFuture in Java 8 in Action?
- Creating CompletableFutures: Use the supplyAsync method to run tasks asynchronously, useful for non-blocking operations.
- Chaining Operations: Chain asynchronous operations with methods like thenApply and thenCompose for a declarative programming style.
- Error Handling: Handle errors with methods like handle and exceptionally, ensuring graceful management of exceptions.
What are some best practices for using streams in Java 8 in Action?
- Prefer Internal Iteration: Use streams for internal iteration, simplifying code and allowing potential parallel processing.
- Avoid Side Effects: Ensure lambdas in streams are stateless and avoid modifying external variables to maintain clarity.
- Use Method References: Leverage method references for cleaner code when passing existing methods as arguments in streams.
How does Java 8 in Action explain the significance of CompletableFuture?
- Asynchronous Programming: CompletableFuture is a tool for handling asynchronous programming, allowing non-blocking code.
- Composing Tasks: Compose multiple asynchronous tasks with methods like thenCompose and thenCombine for managing workflows.
- Error Handling: The book covers handling exceptions in asynchronous computations, crucial for robust applications.
What are the differences between parallel streams and CompletableFutures in Java 8 in Action?
- Parallel Streams: Designed for processing collections in parallel, best for compute-bound tasks with evenly distributed workloads.
- CompletableFutures: More flexible for asynchronous programming, handling I/O-bound tasks without blocking.
- Use Cases: Parallel streams are ideal for data processing, while CompletableFutures excel in managing asynchronous tasks and dependencies.
What are the best quotes from Java 8 in Action and what do they mean?
- "NullPointerException is by far the most common exception in Java.": Highlights the need for better practices like using Optional to avoid null references.
- "Lambda expressions can make your code more readable and flexible.": Emphasizes the benefit of lambdas in simplifying code and enabling behavior parameterization.
- "Functional programming is about writing code that is easier to reason about.": Underlines the core benefit of functional programming—reducing complexity and improving maintainability.
Review Summary
Java 8 in Action receives high praise for its comprehensive coverage of Java 8 features, particularly lambdas and streams. Readers appreciate its clear explanations, practical examples, and insights into functional programming. Many find it helpful for updating their Java knowledge and bridging the gap between older versions and modern Java. The book's focus on Java 8 is both a strength and weakness, as some content becomes outdated with newer Java releases. Despite this, it remains highly recommended for Java developers looking to enhance their understanding of functional programming concepts and Java 8 features.
Similar Books
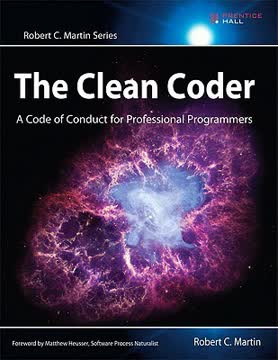
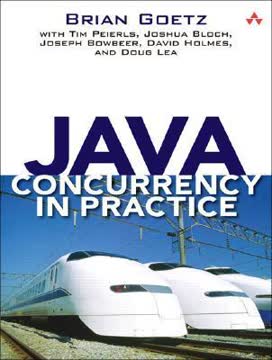
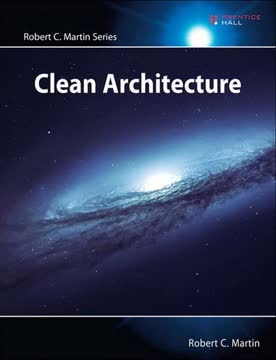
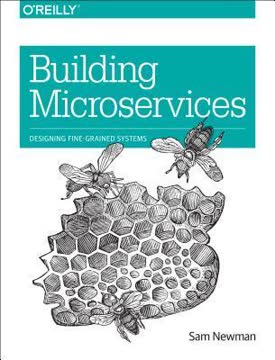
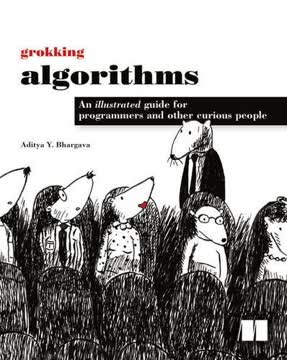
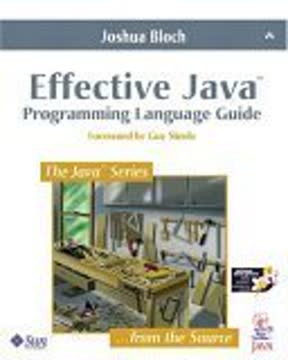
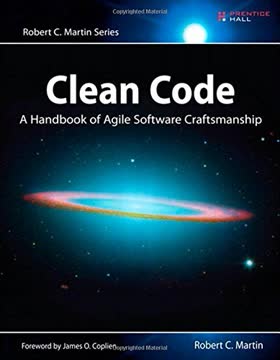
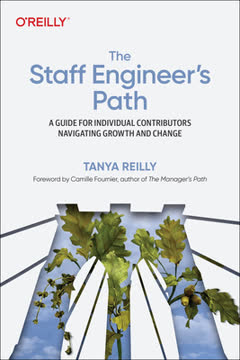
Download PDF
Download EPUB
.epub
digital book format is ideal for reading ebooks on phones, tablets, and e-readers.