Key Takeaways
1. Laravel simplifies web application development with elegant syntax and powerful features
"Laravel is, at its core, about equipping and enabling developers. Its goal is to provide clear, simple, and beautiful code and features that help developers quickly learn, start, and develop, and write code that's simple, clear, and will last."
Framework philosophy. Laravel focuses on developer happiness and productivity. It achieves this through convention over configuration, allowing developers to get started quickly without excessive boilerplate. The framework provides a robust set of tools and libraries that cover common web development tasks, from routing and database access to authentication and caching.
Elegant syntax. Laravel's syntax is designed to be expressive and intuitive. This is evident in various aspects of the framework:
- Fluent database queries:
User::where('active', true)->orderBy('name')->get()
- Simple route definitions:
Route::get('/users', [UserController::class, 'index'])
- Expressive validation rules:
'email' => 'required|email|unique:users'
Powerful features. Laravel includes a wide range of built-in features that accelerate development:
- Eloquent ORM for database interactions
- Blade templating engine for views
- Artisan command-line tool for common tasks
- Built-in authentication and authorization systems
- Queue system for background job processing
- Event broadcasting and WebSocket integration
2. Routing and controllers form the backbone of Laravel's request handling
"Controllers are essentially classes that are responsible for routing user requests through to the application's services and data, and returning some form of useful response back to the user."
Routing system. Laravel's routing system allows developers to define how the application responds to HTTP requests. Routes can be defined for different HTTP methods (GET, POST, PUT, DELETE, etc.) and can include parameters for dynamic segments of the URL.
Controller organization. Controllers in Laravel provide a structured way to group related request handling logic. They can be organized into:
- Resource controllers for RESTful APIs
- Single action controllers for focused functionality
- Invokable controllers for simple, single-purpose actions
Middleware. Laravel's middleware provides a convenient mechanism for filtering HTTP requests entering the application:
- Authentication checks
- CSRF protection
- API rate limiting
- Custom business logic
3. Blade templating engine provides a clean and expressive way to create views
"Blade is Laravel's templating engine. Its primary focus is a clear, concise, and expressive syntax with powerful inheritance and extensibility."
Template inheritance. Blade allows developers to create reusable layouts:
- Define master layouts with
@yield
directives - Extend layouts in child views with
@extends
- Override sections using
@section
and@endsection
Directives and control structures. Blade provides a clean syntax for common PHP control structures:
- Conditionals:
@if
,@else
,@elseif
,@unless
- Loops:
@foreach
,@for
,@while
- Including sub-views:
@include
- Custom directives for extending functionality
Data display and escaping. Blade makes it easy to display data while protecting against XSS attacks:
- Echo data with automatic escaping:
{{ $variable }}
- Echo unescaped data (use with caution):
{!! $variable !!}
- Access nested data easily:
{{ $user->profile->name }}
4. Eloquent ORM simplifies database interactions with an intuitive ActiveRecord implementation
"Eloquent is Laravel's ActiveRecord ORM, which makes it easy to relate a Post class (model) to the posts database table, and get all records with a call like Post::all()."
Model definition. Eloquent models represent database tables and provide an intuitive interface for interacting with data:
- Define relationships between models (hasMany, belongsTo, etc.)
- Set up accessors and mutators for data transformation
- Implement model events for hooks into the lifecycle
Query building. Eloquent provides a fluent interface for building database queries:
- Retrieving data:
User::where('active', true)->get()
- Inserting records:
User::create(['name' => 'John', 'email' => 'john@example.com'])
- Updating records:
$user->update(['status' => 'active'])
- Deleting records:
$user->delete()
Advanced features. Eloquent includes powerful features for complex database operations:
- Eager loading to solve the N+1 query problem
- Soft deletes for archiving records
- Model factories and seeders for testing and development
- Query scopes for reusable query logic
5. Laravel offers robust authentication and authorization out of the box
"Laravel comes with the default User model, the create_users_table migration, the auth controllers, and the auth scaffold, Laravel comes with a full user authentication system out of the box."
Authentication system. Laravel provides a complete authentication system that can be set up with minimal configuration:
- User registration and login
- Password reset functionality
- Remember me feature
- Email verification
Authorization. The framework includes a powerful authorization system:
- Define policies for model-specific authorization logic
- Use Gates for simple closures to determine if a user is authorized
- Implement middleware for route-level authorization
Customization. While the default authentication system is comprehensive, Laravel allows for easy customization:
- Modify authentication views and logic
- Implement multi-authentication with guards
- Integrate third-party authentication providers
6. Artisan command-line tool enhances productivity and simplifies common tasks
"Artisan is the tool that makes it possible to interact with Laravel applications from the command line."
Built-in commands. Artisan comes with a wide range of useful commands:
- Generate boilerplate code for models, controllers, migrations, etc.
- Run database migrations and seeders
- Clear various application caches
- Manage the queue system
Custom commands. Developers can create their own Artisan commands:
- Generate command files with
php artisan make:command
- Define command signature and description
- Implement command logic in the
handle
method
Task scheduling. Artisan includes a task scheduler that allows for easy management of recurring tasks:
- Define a schedule in the
app/Console/Kernel.php
file - Use expressive syntax to define task frequency
- Run a single Cron entry on the server to manage all scheduled tasks
7. Laravel's ecosystem includes powerful tools for testing, queues, and event broadcasting
"Laravel provides a series of tools for implementing queues, queued jobs, events, and WebSocket event publishing. We'll also cover Laravel's scheduler, which makes cron a thing of the past."
Testing. Laravel includes tools for both unit and feature testing:
- PHPUnit integration out of the box
- DatabaseMigrations and DatabaseTransactions traits for database testing
- Mocking facades and services for isolated unit tests
- Browser testing with Laravel Dusk
Queue system. Laravel's queue system allows for the deferral of time-consuming tasks:
- Multiple queue drivers (database, Redis, Amazon SQS, etc.)
- Job classes for encapsulating queue logic
- Failed job handling and retry mechanisms
Event broadcasting. Laravel makes it easy to implement real-time features:
- Define and fire events within the application
- Broadcast events over WebSockets
- Integrate with services like Pusher or use Laravel Echo for client-side listening
Ecosystem tools. Laravel's ecosystem includes additional tools that extend its capabilities:
- Laravel Forge for server management and deployment
- Laravel Nova for rapid admin panel development
- Laravel Vapor for serverless deployment on AWS Lambda
By leveraging these powerful features and tools, developers can build robust, scalable, and maintainable web applications with Laravel. The framework's focus on developer experience, combined with its extensive functionality, makes it an excellent choice for projects of all sizes.
Last updated:
FAQ
What's Laravel: Up and Running by Matt Stauffer about?
- Comprehensive Guide: The book serves as a comprehensive introduction to Laravel, a popular PHP framework for building modern web applications.
- Practical Focus: It emphasizes practical application with real-world examples and best practices, making it suitable for both beginners and experienced developers.
- Framework Features: Key features such as routing, middleware, Eloquent ORM, and Blade templating are thoroughly explained, along with advanced topics like queues and events.
Why should I read Laravel: Up and Running by Matt Stauffer?
- Expert Insights: Matt Stauffer, a respected figure in the Laravel community, shares insights that help avoid common pitfalls and enhance understanding.
- Structured Learning: The book is organized to build knowledge progressively, with each chapter reinforcing and introducing new concepts logically.
- Hands-On Practice: It includes hands-on examples that allow readers to practice immediately, enhancing retention and understanding of the material.
What are the key takeaways of Laravel: Up and Running by Matt Stauffer?
- Laravel's Philosophy: Emphasizes Laravel's focus on developer happiness and speed, stating that "Happy developers make the best code."
- Framework Benefits: Discusses the benefits of using frameworks like Laravel, which provide a structured way to build applications efficiently.
- Ecosystem Tools: Introduces Laravel's ecosystem, including tools like Homestead and Forge, which enhance the development experience.
What are the best quotes from Laravel: Up and Running by Matt Stauffer and what do they mean?
- "Happy developers make the best code.": Highlights Laravel's philosophy that a positive developer experience leads to better quality code.
- "Laravel helps you bring your ideas to reality with no wasted code.": Emphasizes Laravel's efficiency and focus on rapid application development.
- "The best frameworks will not only provide you with a solid foundation, but also give you the freedom to customize.": Reflects Laravel's balance between convention and flexibility.
How does Laravel: Up and Running by Matt Stauffer explain the MVC architecture?
- MVC Overview: The book emphasizes the Model-View-Controller (MVC) architecture, which is foundational for effectively using Laravel.
- Separation of Concerns: MVC separates application logic, user interface, and data handling, promoting organized and maintainable code.
- Practical Examples: Provides examples of how MVC is implemented in Laravel, helping readers understand its practical application.
What is Eloquent ORM in Laravel: Up and Running by Matt Stauffer and how is it used?
- Active Record Pattern: Eloquent is Laravel's built-in ORM that follows the Active Record pattern, simplifying database interactions.
- Model Relationships: Simplifies defining relationships between models, such as one-to-many and many-to-many, making data handling intuitive.
- Query Building: Offers a fluent query builder for constructing complex queries easily, enhancing database operation efficiency.
How does Laravel: Up and Running by Matt Stauffer address user authentication?
- Built-In System: Laravel provides a robust authentication system out of the box, including user registration, login, and password reset functionalities.
- Auth Controllers: Details the role of controllers like RegisterController and LoginController in managing authentication processes.
- Authorization Features: Discusses Laravel's authorization features, including policies and gates, for managing user permissions and access control.
How does Laravel: Up and Running by Matt Stauffer explain middleware?
- Middleware Overview: Introduces middleware as a way to filter HTTP requests, defining behavior before or after request processing.
- Common Middleware: Covers built-in middleware like authentication and CSRF protection, essential for securing applications.
- Custom Middleware: Provides guidance on creating custom middleware to handle specific application needs, enhancing request handling flexibility.
How does Laravel: Up and Running by Matt Stauffer cover routing?
- Simple Definitions: Explains straightforward route definitions using the
Route
facade for organizing application URLs. - Dynamic Parameters: Describes how to define dynamic route parameters for flexible URL structures and easy parameter access.
- Named Routes: Discusses named routes for simplifying URL generation and redirection, enhancing route management.
What is the role of migrations in Laravel: Up and Running by Matt Stauffer?
- Schema Management: Migrations allow developers to define and manage database schema structure using code, facilitating version control.
- Up and Down Methods: Each migration file includes methods to apply and revert changes, ensuring database changes can be rolled back.
- Artisan Commands: Explains using Artisan commands to run migrations, making database schema changes straightforward.
How does Laravel: Up and Running by Matt Stauffer cover testing?
- Importance of Testing: Emphasizes testing in Laravel applications, providing examples for writing tests for routes, controllers, and models.
- Authentication Testing: Covers testing authentication routes and user registration processes to ensure system functionality.
- Using PHPUnit: Discusses integrating PHPUnit for testing, highlighting Laravel's framework for simplifying test writing and execution.
What are queues in Laravel: Up and Running by Matt Stauffer and how are they used?
- Asynchronous Processing: Queues allow deferring time-consuming tasks, like sending emails, to keep applications responsive.
- Job Creation: Describes creating jobs with Artisan commands, encapsulating task logic for queue processing.
- Queue Workers: Explains running queue workers to process jobs, ensuring tasks are handled efficiently as they are added.
Review Summary
Laravel by Matt Stauffer is highly praised by readers, with an overall rating of 4.47/5. Many reviewers found it comprehensive, covering both basics and advanced topics. It's recommended for beginners and experienced developers alike. Readers appreciate its detailed explanations, practical examples, and test sessions. Some consider it an excellent reference guide. A few readers noted it doesn't follow a guided project approach and may be outdated for the latest Laravel versions. Overall, it's seen as a valuable resource for learning and mastering the Laravel framework.
Similar Books
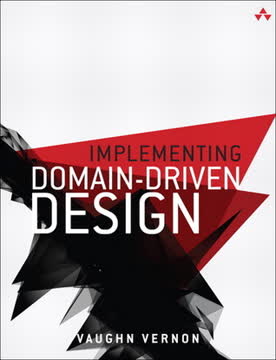
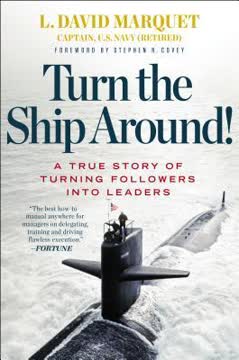
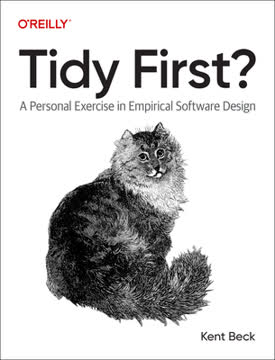
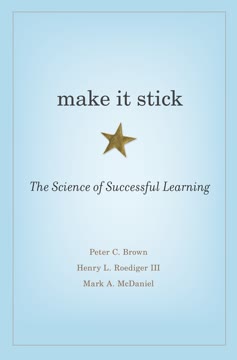

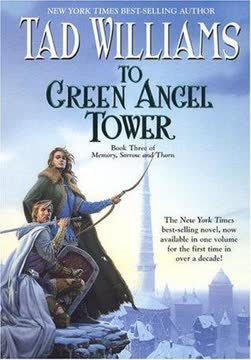
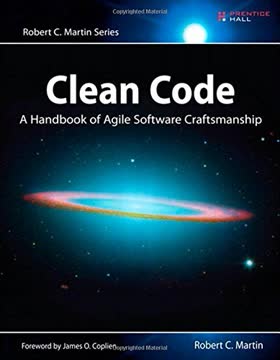
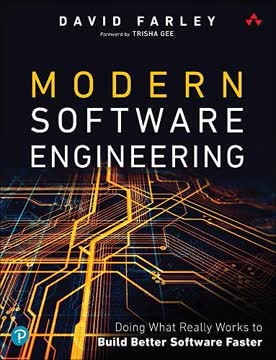
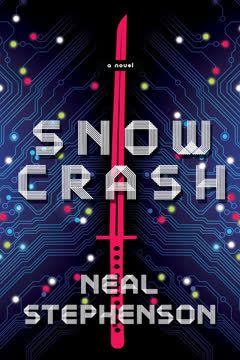
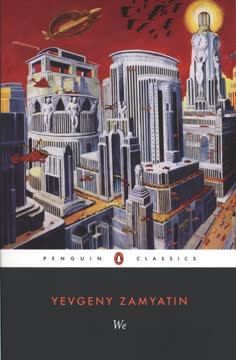
Download PDF
Download EPUB
.epub
digital book format is ideal for reading ebooks on phones, tablets, and e-readers.