Key Takeaways
1. JavaScript design patterns optimize code structure and solve common problems
Design patterns make it easy for us to build on the shoulders of developers who have defined solutions to challenging problems and architectures over several decades.
Reusable solutions. Design patterns in JavaScript provide tried-and-tested solutions to common programming challenges. They offer a shared vocabulary for developers to discuss and implement efficient code structures. By leveraging these patterns, developers can:
- Improve code organization and maintainability
- Enhance software flexibility and scalability
- Facilitate easier collaboration among team members
Pattern categories. Design patterns are typically categorized into three main groups:
- Creational: Dealing with object creation mechanisms
- Structural: Focusing on object composition and relationships
- Behavioral: Addressing object communication and responsibility distribution
Understanding and applying these patterns can significantly improve the quality and efficiency of JavaScript applications, leading to more robust and maintainable codebases.
2. Creational patterns: Constructor, Module, Singleton, and Factory
The Module pattern encapsulates the "privacy" state and organization using closures.
Object creation mechanisms. Creational patterns focus on efficient ways to create objects and manage object creation processes. Key creational patterns in JavaScript include:
- Constructor Pattern: Defines object initialization
- Module Pattern: Encapsulates private state and public interfaces
- Singleton Pattern: Ensures only one instance of a class exists
- Factory Pattern: Centralizes object creation logic
Modularity and encapsulation. These patterns promote:
- Code organization and reusability
- Data privacy and controlled access
- Flexible object instantiation
By employing creational patterns, developers can create more maintainable and scalable code structures, especially in large-scale applications where object creation and management can become complex.
3. Structural patterns: Decorator, Facade, Flyweight, and Mixin
The Decorator pattern aims to promote code reuse.
Composing objects. Structural patterns deal with object composition, providing ways to form larger structures from individual objects. Key structural patterns include:
- Decorator: Adds new functionality to objects dynamically
- Facade: Simplifies complex subsystems with a unified interface
- Flyweight: Shares common data across multiple objects
- Mixin: Adds properties and methods to objects
Flexibility and efficiency. These patterns offer:
- Enhanced code reusability
- Simplified interfaces for complex systems
- Optimized memory usage
- Flexible object composition
Structural patterns allow developers to create more flexible and efficient object structures, improving overall system design and performance.
4. Behavioral patterns: Observer, Mediator, and Command
The Observer pattern allows you to notify one object when another object changes without requiring the object to know about its dependents.
Object interactions. Behavioral patterns focus on communication between objects, defining how they operate together. Key behavioral patterns include:
- Observer: Defines a one-to-many dependency between objects
- Mediator: Centralizes complex communications between objects
- Command: Encapsulates a request as an object
Improved communication. These patterns provide:
- Loose coupling between objects
- Centralized control of object interactions
- Flexibility in executing and undoing operations
By implementing behavioral patterns, developers can create more robust and maintainable systems, especially in complex applications with numerous interacting components.
5. MVC, MVP, and MVVM architectural patterns for application organization
MVC relies on the Observer pattern for some of its core communication (something that, surprisingly, isn't covered in many articles about the MVC pattern).
Separation of concerns. These architectural patterns provide structures for organizing application logic:
- Model-View-Controller (MVC): Separates data, user interface, and control logic
- Model-View-Presenter (MVP): Similar to MVC, but with a more passive View
- Model-View-ViewModel (MVVM): Separates View development from business logic
Benefits of architectural patterns:
- Improved code organization and maintainability
- Enhanced testability of individual components
- Facilitated collaboration among developers
- Better scalability for large applications
These patterns help developers create more organized, maintainable, and scalable applications by providing clear structures for separating different aspects of application logic.
6. Modern JavaScript modules and asynchronous programming patterns
The async/await pattern uses async/await as the primary way to fetch data from Server Components.
Modular code organization. Modern JavaScript provides built-in module support, allowing developers to create more organized and maintainable codebases. Key concepts include:
- ES6 modules with import and export statements
- Dynamic imports for code-splitting and performance optimization
Asynchronous programming. Patterns for handling asynchronous operations include:
- Promises: Representing the eventual completion of an async operation
- Async/await: Syntactic sugar for working with Promises
- Observables: Handling streams of asynchronous data
These modern patterns enable developers to write cleaner, more efficient code for handling complex asynchronous operations and organizing large-scale applications.
7. React design patterns: Higher-Order Components, Render Props, and Hooks
Higher-Order Components allow us to keep logic we want to reuse all in one place.
Component composition. React offers several patterns for creating reusable and composable components:
- Higher-Order Components (HOCs): Enhance components with additional functionality
- Render Props: Share code between components using a prop whose value is a function
- Hooks: Add state and lifecycle features to functional components
Benefits of React patterns:
- Improved code reusability
- Enhanced component composition
- Simplified state management
- Reduced boilerplate code
These patterns enable developers to create more flexible, maintainable, and efficient React applications by promoting code reuse and separation of concerns.
8. Rendering patterns: Client-side, Server-side, Static, and Hybrid approaches
The PRPL pattern focuses on four primary performance considerations: Pushing critical resources efficiently, Rendering the initial route as soon as possible, Pre-caching assets in the background, and Lazily loading routes or assets.
Optimizing performance. Various rendering patterns aim to improve application performance and user experience:
- Client-side Rendering (CSR): Renders content in the browser
- Server-side Rendering (SSR): Generates HTML on the server
- Static Rendering: Pre-renders content at build time
- Hybrid Rendering: Combines multiple rendering approaches
Considerations for rendering patterns:
- Initial load time and Time to Interactive (TTI)
- Search Engine Optimization (SEO)
- Server load and scalability
- Content update frequency
Choosing the appropriate rendering pattern depends on the specific requirements of the application, balancing factors such as performance, SEO, and development complexity.
9. Application structure best practices for scalable React projects
Sticking to a defined pattern for structuring a project would help you explain it to other team members and prevent projects from getting disorganized and unnecessarily complicated.
Organized project structure. Implementing a clear and consistent project structure is crucial for maintaining scalable React applications. Key considerations include:
- Grouping files by feature or module
- Separating presentational and container components
- Implementing a clear naming convention
- Utilizing appropriate state management solutions
Best practices:
- Keep components small and focused
- Use meaningful directory names
- Implement lazy loading for optimized performance
- Maintain consistent coding standards
A well-structured React application improves code maintainability, facilitates collaboration among team members, and enhances overall project scalability.
Last updated:
Review Summary
Learning Javascript Design Patterns receives mixed reviews. Some praise its comprehensive coverage of design patterns and practical examples, while others find it outdated and overly focused on specific libraries. Positive reviews highlight its usefulness for intermediate developers and its enduring relevance. Criticisms include inconsistent editing, dated content, and overemphasis on jQuery. Many readers appreciate the book's explanation of common patterns but suggest it may not be the best choice for those seeking cutting-edge JavaScript techniques. Overall, it's seen as a solid introduction to design patterns, albeit with some limitations.
Similar Books
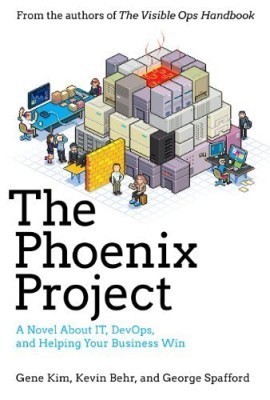
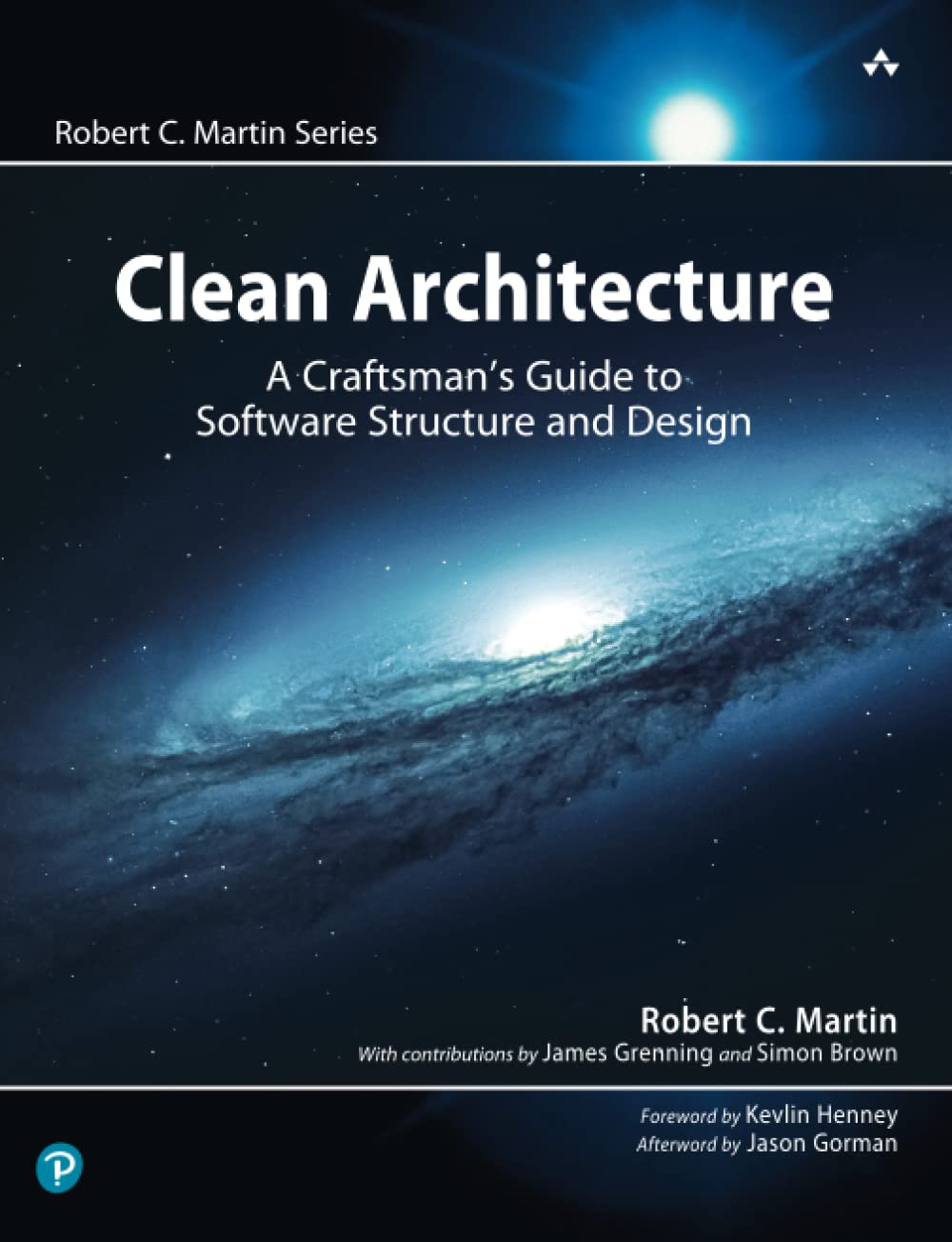
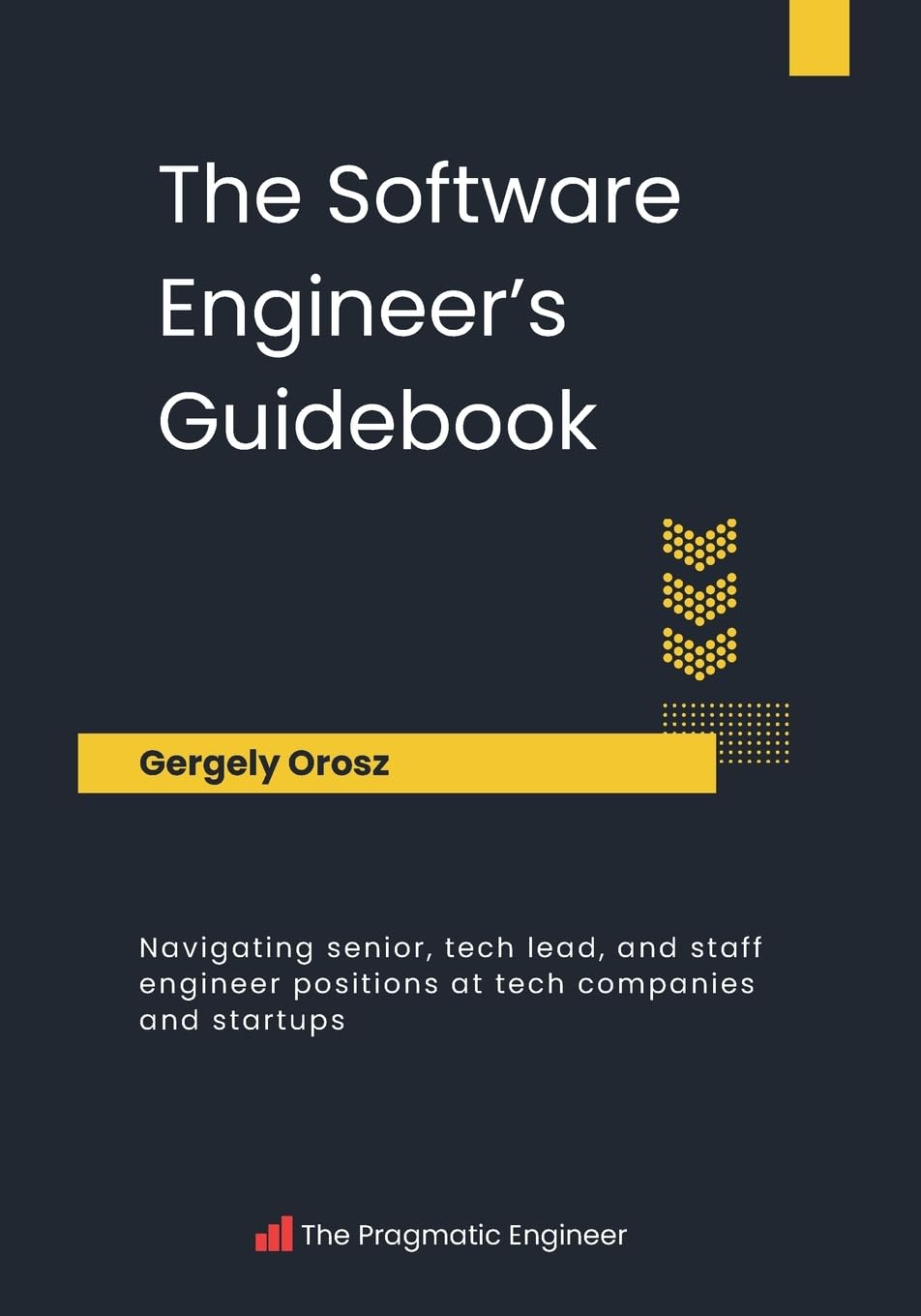
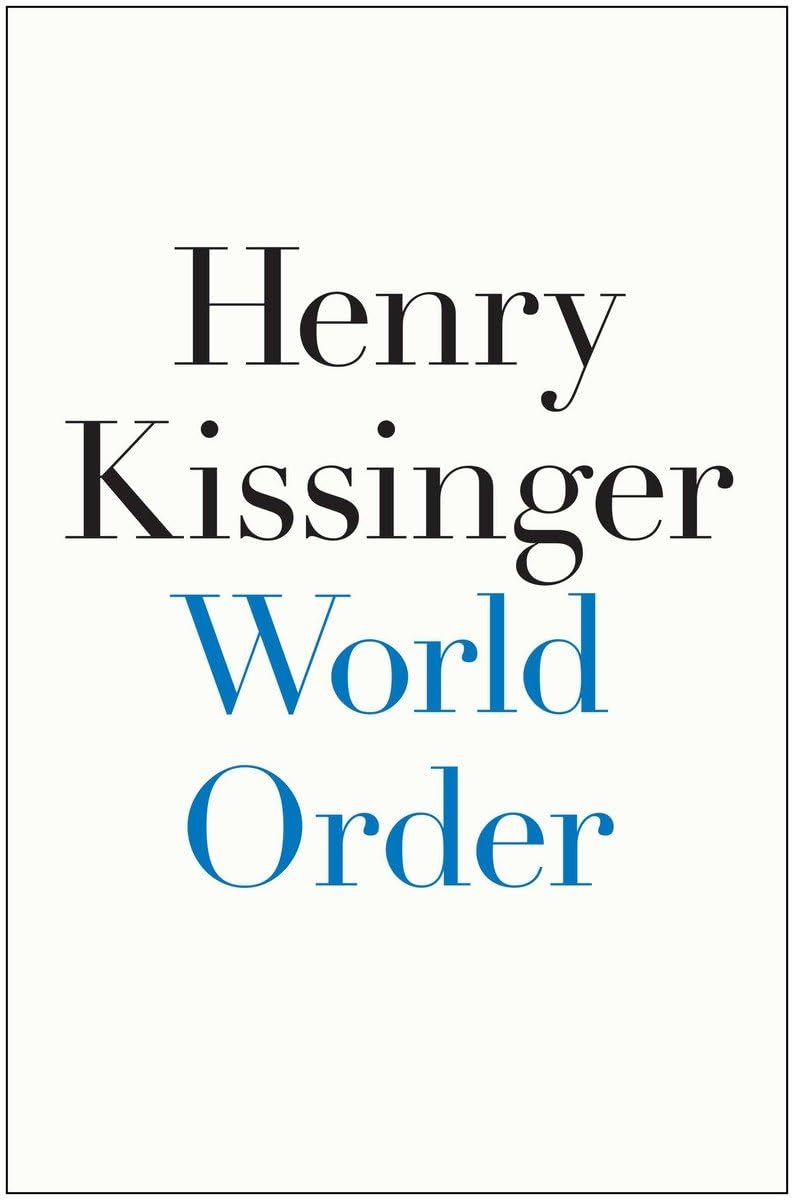
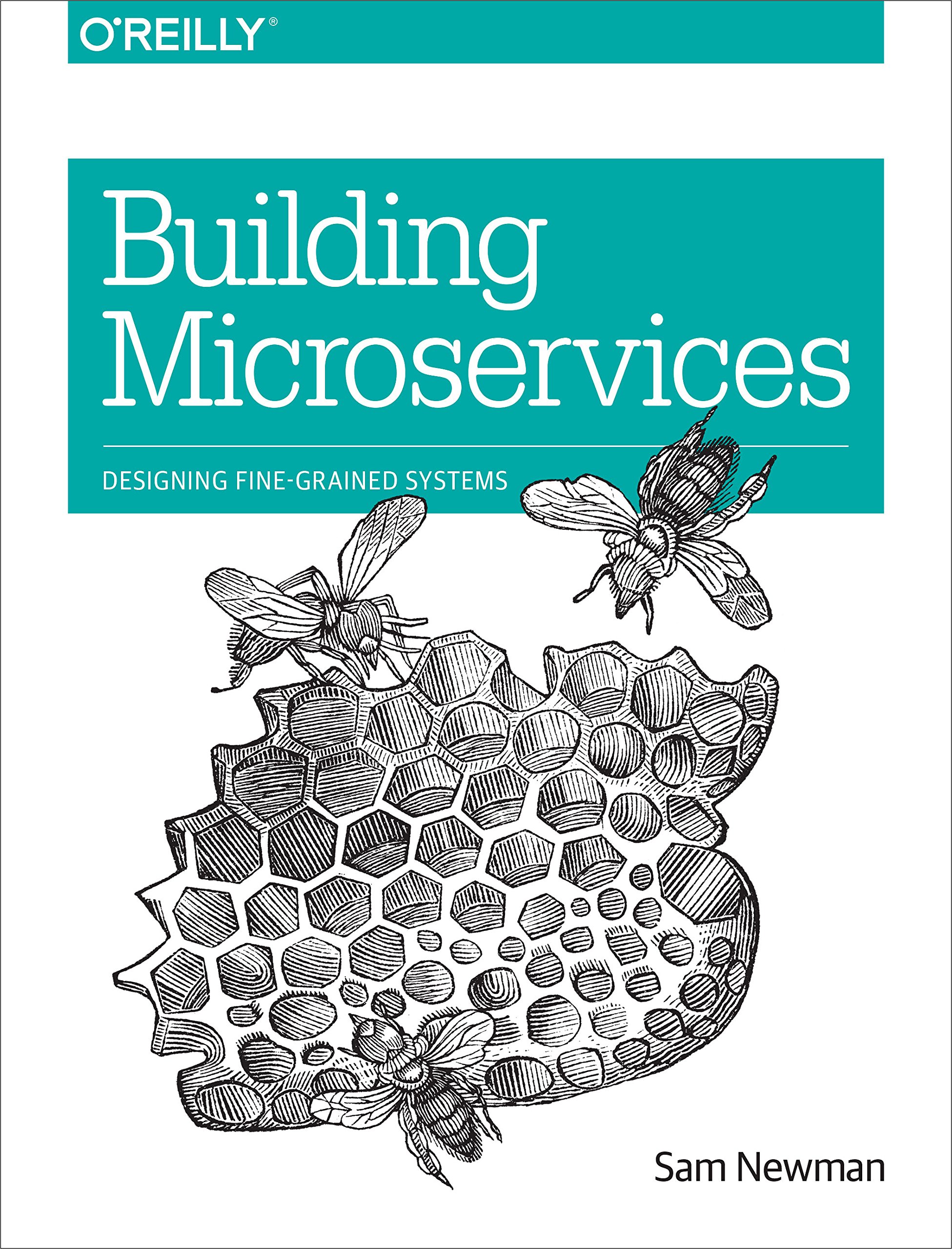
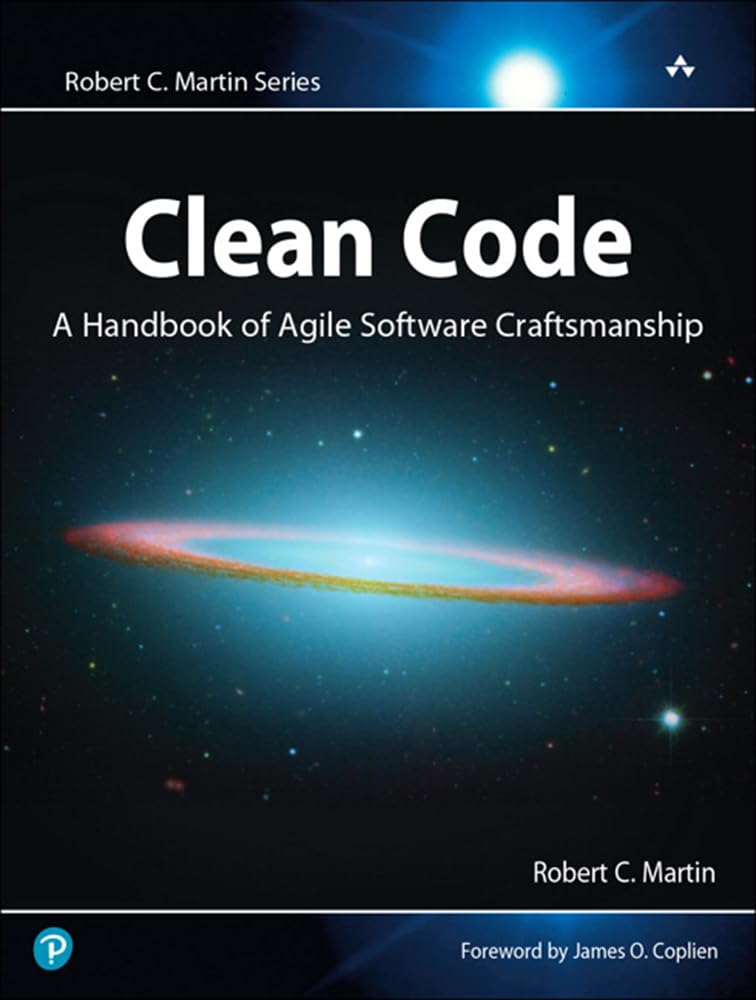
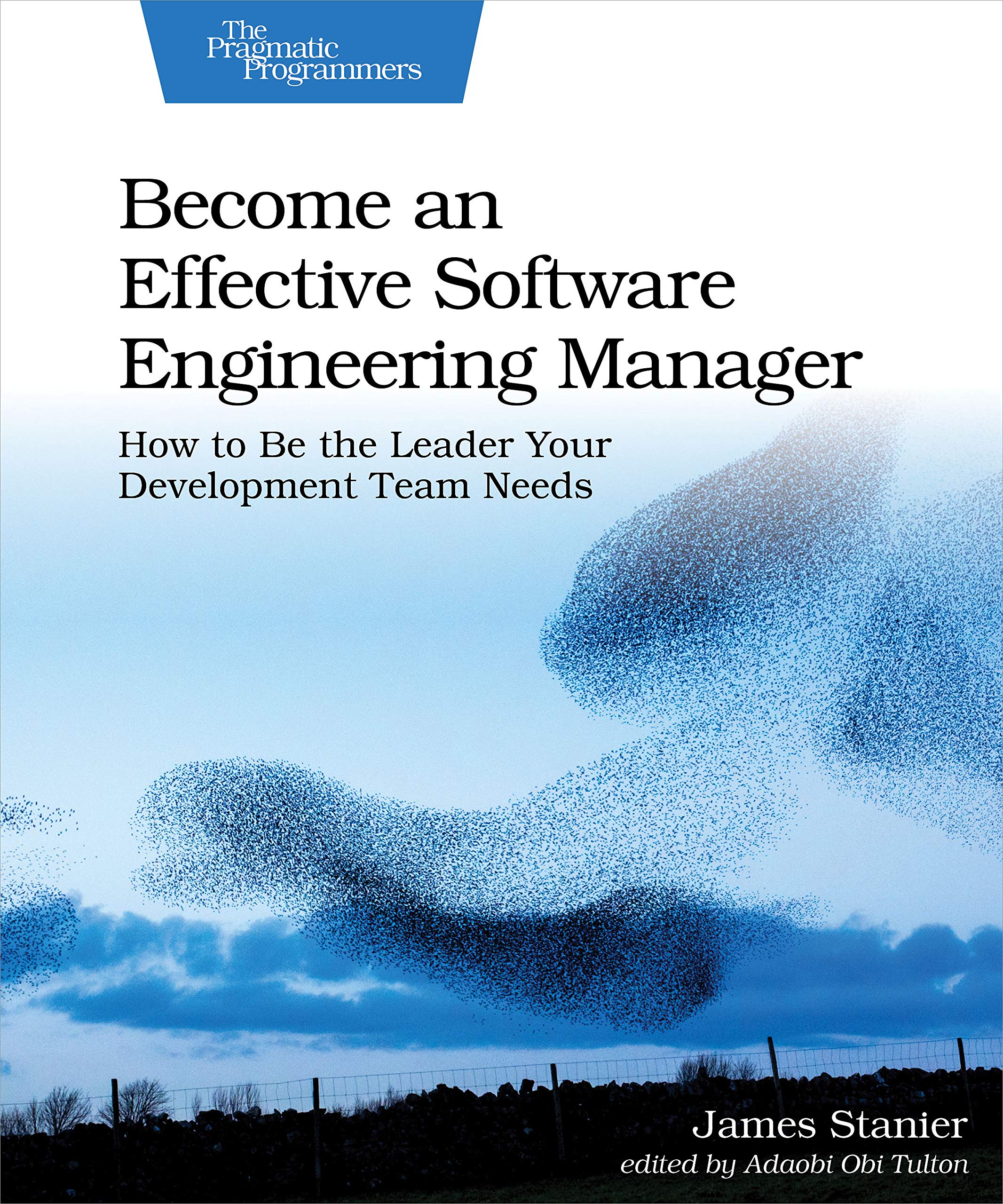

Download PDF
Download EPUB
.epub
digital book format is ideal for reading ebooks on phones, tablets, and e-readers.