Key Takeaways
1. React simplifies UI development with reusable components.
The goal of this book is to avoid confusion in the learning process by putting things in a sequence: a learning roadmap.
Component-based architecture. React's core strength lies in its component-based architecture, which allows developers to break down complex UIs into smaller, manageable, and reusable pieces. These components encapsulate both the structure (HTML) and behavior (JavaScript) of a UI element, making it easier to reason about and maintain.
Benefits of reusability. Reusable components promote code efficiency, reduce redundancy, and improve consistency across the application. By creating a library of well-defined components, developers can quickly assemble complex UIs with minimal effort. For example, a button component can be used throughout the application with different styles and functionalities, ensuring a consistent user experience.
Scalability and maintainability. The component-based approach also enhances the scalability and maintainability of React applications. When changes are needed, developers can focus on modifying individual components without affecting the entire application. This modularity makes it easier to add new features, fix bugs, and refactor code.
2. JSX offers a concise syntax for creating React elements.
Yes, in React, you write code that looks like HTML right in your JavaScript code.
Tag-based syntax. JSX (JavaScript XML) is a syntax extension that allows developers to write HTML-like code directly within their JavaScript files. This provides a more intuitive and readable way to define React elements, making it easier to visualize the structure of the UI.
Benefits of JSX:
- Improved readability: JSX code is easier to understand and maintain compared to raw JavaScript code for creating React elements.
- Concise syntax: JSX reduces the amount of code needed to define UI elements, making development faster and more efficient.
- Familiarity: JSX resembles HTML, making it easier for developers with web development experience to learn and use.
Babel transformation. JSX code is not directly understood by browsers. It needs to be transformed into standard JavaScript code using a tool like Babel. This transformation process converts JSX tags into React.createElement() calls, which the browser can then interpret and render.
3. Functional programming enhances React code readability and testability.
A nice side effect of working with React is that it can make you a stronger JavaScript developer by promoting patterns that are readable, reusable, and testable.
Emphasis on pure functions. Functional programming principles, such as immutability and pure functions, align well with React's component-based architecture. Pure functions, which always return the same output for a given input and have no side effects, make code more predictable and easier to test.
Benefits of functional programming in React:
- Improved readability: Functional code tends to be more concise and easier to understand.
- Enhanced testability: Pure functions are naturally testable because their output is solely determined by their input.
- Increased reusability: Functional components can be easily composed and reused throughout the application.
Data transformations. Functional programming emphasizes transforming data from one form to another using functions. This approach promotes immutability and avoids modifying data directly, leading to more predictable and maintainable code.
4. Hooks enable state management and side effects in functional components.
More recently in 2019, we saw the release of Hooks, a new way of adding and sharing stateful logic across components.
State and side effects. Hooks are a feature in React that allows functional components to manage state and perform side effects, which were previously only possible in class components. This simplifies component logic and promotes code reuse.
Commonly used Hooks:
- useState: Manages component state.
- useEffect: Performs side effects, such as data fetching or DOM manipulation.
- useContext: Accesses values from React context.
- useRef: Creates persistent references to DOM elements or values.
Custom Hooks. Custom Hooks allow developers to extract and reuse stateful logic across multiple components. This promotes code modularity and reduces redundancy. For example, a custom Hook can be created to handle form input validation or data fetching, and then reused in different components that require similar functionality.
5. React Router facilitates navigation in single-page applications.
After all, React was billed as a library: concerned with implementing a specific set of features, not providing a tool for every use case.
Client-side routing. React Router is a library that enables client-side routing in single-page applications (SPAs). It allows developers to define routes that map URLs to specific components, providing a seamless navigation experience without requiring full page reloads.
Key components of React Router:
- BrowserRouter: Provides the routing context for the application.
- Route: Defines a mapping between a URL path and a component.
- Link: Creates navigation links that update the URL without triggering a full page reload.
- useParams: Accesses parameters from the current URL.
Dynamic routing. React Router supports dynamic routing, which allows developers to define routes with parameters that can be used to pass data to components. This is useful for creating dynamic UIs that display different content based on the URL.
6. Testing ensures React component reliability and code quality.
A nice side effect of working with React is that it can make you a stronger JavaScript developer by promoting patterns that are readable, reusable, and testable.
Importance of testing. Testing is a crucial aspect of software development that ensures the reliability and quality of React components. Unit tests verify that individual components function as expected, while integration tests ensure that different parts of the application work together seamlessly.
Popular testing frameworks:
- Jest: A JavaScript testing framework that comes pre-configured with Create React App.
- React Testing Library: A library that provides utilities for testing React components in a user-centric way.
- Enzyme: A JavaScript testing utility for React that makes it easier to assert, manipulate, and traverse your React Components’ output.
Test-Driven Development (TDD). TDD is a development practice where tests are written before the code. This approach helps developers clarify requirements, design better code, and ensure that all functionality is thoroughly tested.
7. Data fetching strategies are crucial for dynamic React applications.
With the modern web, we need to perform asynchronous tasks.
Asynchronous operations. Modern web applications often need to fetch data from external APIs or perform other asynchronous operations. React provides several techniques for handling these operations, including:
Data fetching techniques:
- Fetch API: A built-in JavaScript API for making HTTP requests.
- Axios: A popular third-party library for making HTTP requests.
- useEffect Hook: Used to perform side effects, such as data fetching, in functional components.
Handling loading and error states. When fetching data, it's important to handle loading and error states gracefully. This can be achieved by using state variables to track the status of the request and rendering appropriate UI elements based on the current state.
8. Suspense handles asynchronous operations for better user experience.
More recently in 2019, we saw the release of Hooks, a new way of adding and sharing stateful logic across components.
Declarative data fetching. Suspense is a React feature that allows developers to declaratively specify how to handle asynchronous operations, such as data fetching. It provides a way to suspend rendering until the data is available, improving the user experience by preventing flickering or incomplete UIs.
Key components of Suspense:
- Suspense component: Wraps components that may suspend rendering.
- fallback prop: Specifies the UI to render while the component is suspended.
- lazy function: Used to load components asynchronously.
Error boundaries. Error boundaries are components that can catch JavaScript errors anywhere in their child component tree, log those errors, and display a fallback UI. They provide a way to prevent errors from crashing the entire application and improve the user experience by providing informative error messages.
9. Webpack optimizes React applications for production.
As an example, let’s look at an arrow function with some default arguments:
Module bundling. Webpack is a module bundler that takes all of the different files in a JavaScript project (JavaScript, CSS, images, etc.) and transforms them into a single file or a set of files that can be deployed to a web server.
Benefits of Webpack:
- Code splitting: Divides the code into smaller chunks that can be loaded on demand, improving initial load time.
- Minification: Removes unnecessary characters from the code, reducing file size.
- Asset optimization: Optimizes images and other assets to improve performance.
- Hot Module Replacement (HMR): Allows developers to see changes in the code without refreshing the page.
Loaders and plug-ins. Webpack uses loaders to transform different types of files and plug-ins to perform additional tasks, such as minification and code splitting.
10. ESLint and Prettier maintain code consistency and readability.
Many of the features that are included in the latest JavaScript syntax are present because they support functional programming techniques.
Code linting. ESLint is a tool that analyzes JavaScript code for potential errors, style violations, and other issues. It helps developers maintain code quality and consistency by enforcing coding standards.
Code formatting. Prettier is an opinionated code formatter that automatically formats code according to a set of predefined rules. It helps developers maintain code readability and consistency by eliminating debates over code style.
Benefits of ESLint and Prettier:
- Improved code quality: ESLint helps identify potential errors and enforce coding standards.
- Increased readability: Prettier ensures that code is consistently formatted, making it easier to read and understand.
- Reduced code review time: By automating code formatting and linting, ESLint and Prettier reduce the amount of time spent on code reviews.
Last updated:
Review Summary
Learning React receives mostly positive reviews, with an average rating of 4.06 out of 5. Readers appreciate its comprehensive coverage of React concepts, including functional programming, hooks, and Redux. The book is praised for its clear explanations and practical examples. Some criticisms include outdated information, code errors, and difficulty following examples. Several reviewers recommend it for beginners, while others suggest it's better suited for those with some React experience. Overall, it's considered a solid introduction to React, though some prefer official documentation for up-to-date information.
Similar Books
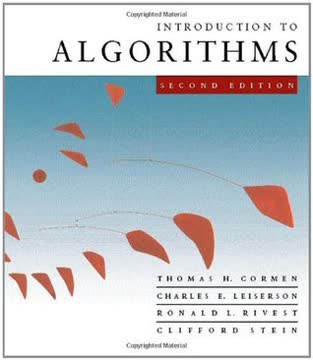
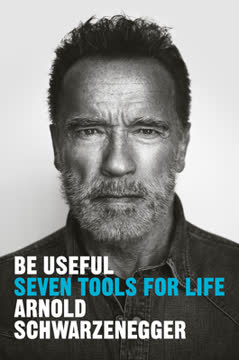
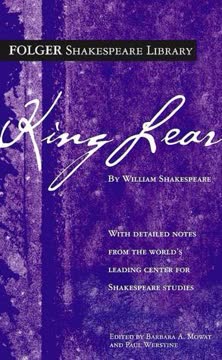
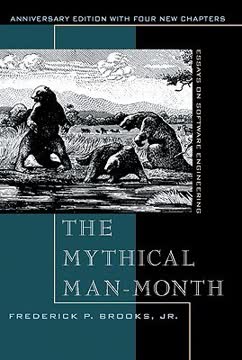
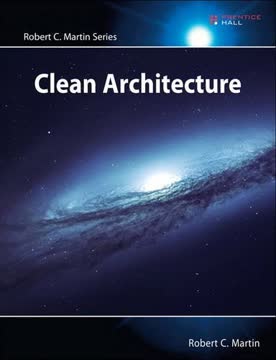
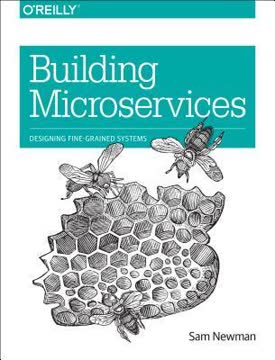
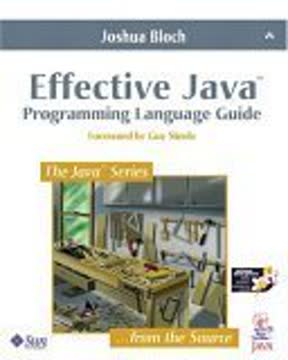
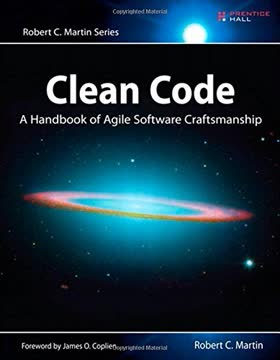
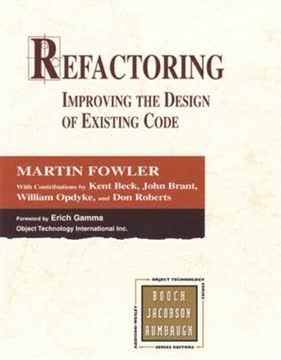
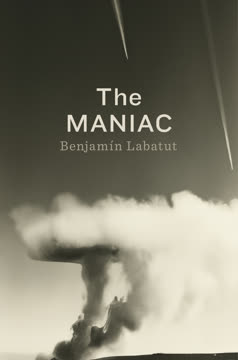
Download EPUB
.epub
digital book format is ideal for reading ebooks on phones, tablets, and e-readers.