Key Takeaways
1. Computation is systematic problem-solving through algorithms
Computation plays a prominent role in society now.
Ubiquitous problem-solving. Computation is not limited to computers but occurs in many everyday activities. It involves systematically transforming a problem's initial state into a desired final state through a series of well-defined steps called an algorithm. This process is analogous to following a recipe or navigating using a map.
Algorithmic thinking. Understanding computation helps develop computational thinking skills, which are valuable for solving real-world problems more effectively. This includes breaking down complex problems into smaller, manageable subproblems, recognizing patterns, and devising step-by-step solutions. Examples of computational thinking in daily life include:
- Planning a trip with multiple stops
- Organizing a closet efficiently
- Optimizing a workout routine
Resource awareness. Computation requires resources such as time, energy, and memory. Efficient algorithms minimize resource usage, which is crucial for solving large-scale problems. This concept applies to both digital and physical world computations, from optimizing database queries to planning assembly line operations.
2. Algorithms manipulate representations to solve problems
The essence of a computation is the transformation of representation.
Abstraction and modeling. Algorithms operate on representations of real-world objects or concepts. These representations abstract away unnecessary details while preserving essential information needed for problem-solving. For example:
- A map represents geographical features as symbols
- A shopping list represents items as text
- A computer program represents data as binary digits
Transformation process. Algorithms systematically transform input representations into output representations that solve the given problem. This transformation often involves a series of smaller, intermediate steps. For instance, a GPS navigation algorithm might:
- Represent current location and destination as coordinates
- Transform road network into a graph
- Apply pathfinding algorithm to find optimal route
- Transform route back into user-friendly directions
Representation design. Choosing appropriate representations is crucial for effective problem-solving. Good representations make relevant information easily accessible and support efficient algorithmic operations. Poor representations can make problems unnecessarily difficult or even unsolvable.
3. Data structures organize information for efficient access
The choice of data structure is important for the efficiency of algorithms and is sometimes influenced by other considerations, such as the available space.
Organizing information. Data structures are specialized formats for storing and organizing data to support efficient access and modification. Common data structures include:
- Arrays: Fixed-size collections with fast random access
- Linked lists: Dynamic collections optimized for insertion and deletion
- Trees: Hierarchical structures for representing relationships
- Hash tables: Fast key-based lookup and storage
Performance trade-offs. Different data structures excel at different operations. Choosing the right data structure involves analyzing the specific requirements of the problem and the algorithms that will operate on the data. For example:
- Arrays allow fast access by index but slow insertion/deletion
- Linked lists allow fast insertion/deletion but slow random access
- Binary search trees offer balanced performance for many operations
Space considerations. In addition to time efficiency, data structures must consider space efficiency, especially when dealing with large amounts of data or constrained environments. Some data structures use extra space to improve access times, while others prioritize compact storage at the cost of slower operations.
4. Searching and sorting are fundamental algorithmic problems
Searching is a prevalent problem in real life, and it is also an important topic in computer science.
Ubiquitous operations. Searching and sorting are fundamental operations that underlie many computational tasks. Efficient search and sort algorithms are crucial for managing large datasets and enabling fast information retrieval. Real-world applications include:
- Database queries
- Spell checking
- Route planning
- Contact list management
Search strategies. Various search algorithms exist, each with different strengths:
- Linear search: Simple but inefficient for large datasets
- Binary search: Efficient for sorted data
- Hash-based search: Very fast for key-based lookups
- Graph search algorithms: For navigating complex relationships
Sorting techniques. Sorting algorithms organize data to enable more efficient operations, especially searching. Common sorting algorithms include:
- Quicksort: Efficient general-purpose sorting
- Mergesort: Stable sorting with guaranteed performance
- Heapsort: In-place sorting with good worst-case performance
- Bucket sort: Fast for uniformly distributed data
The choice of search or sort algorithm depends on factors such as data size, distribution, and required operation frequency.
5. Some problems are intractable, requiring approximation
There are computational problems that cannot be solved algorithmically—ever.
Computational limits. Some problems, known as intractable problems, cannot be solved efficiently by any known algorithm. These problems typically have exponential time complexity, meaning the time required to solve them grows extremely rapidly with input size. Examples include:
- The traveling salesman problem
- Optimal protein folding
- Factoring large numbers (important for cryptography)
Coping strategies. When faced with intractable problems, several approaches can be taken:
- Use approximation algorithms that find "good enough" solutions
- Limit input size to keep computation time manageable
- Use heuristics to guide search towards likely solutions
- Exploit problem-specific structure to reduce complexity
Theoretical implications. The existence of intractable problems has profound implications for computer science and mathematics. It demonstrates fundamental limits to computation and has led to important fields of study such as complexity theory and the P vs NP problem.
6. Recursion and loops enable powerful computational patterns
Recursion is a control structure but is also used in the definition of data organization.
Repetitive patterns. Recursion and loops are mechanisms for expressing repetitive computational patterns. They allow concise description of algorithms that operate on data of arbitrary size or depth. Key concepts include:
- Base case: Termination condition for recursion
- Recursive case: Self-referential step
- Loop invariant: Condition that holds true in each iteration
Problem decomposition. Recursive thinking often leads to elegant solutions for problems that can be naturally decomposed into smaller, similar subproblems. Examples include:
- Traversing tree-like structures (e.g., file systems)
- Divide-and-conquer algorithms (e.g., mergesort)
- Generating combinations or permutations
Implementation considerations. While recursion and loops can often be used interchangeably, each has strengths and weaknesses:
- Recursion: Often more intuitive for certain problems, but can be memory-intensive
- Loops: Generally more efficient, but can be harder to reason about for complex patterns
Understanding both approaches allows choosing the most appropriate tool for each situation.
7. Languages define syntax and semantics of computation
Any algorithm has to be expressed in some language.
Formal description. Programming languages provide formal ways to express algorithms and computations. They consist of:
- Syntax: Rules for forming valid statements
- Semantics: Rules for interpreting the meaning of statements
Language design. Different programming languages are designed for various purposes and paradigms:
- Imperative languages (e.g., C, Python): Describe computations as sequences of commands
- Functional languages (e.g., Haskell, Lisp): Express computations as mathematical functions
- Object-oriented languages (e.g., Java, C++): Organize code around data structures and their operations
- Domain-specific languages: Tailored for specific application areas (e.g., SQL for databases)
Abstraction levels. Languages can operate at different levels of abstraction:
- Machine code: Direct hardware instructions
- Assembly language: Human-readable low-level code
- High-level languages: More abstract, closer to natural language
- Visual programming languages: Use graphical elements to represent code
Higher-level languages increase programmer productivity but may sacrifice some control over low-level details.
8. Types and abstractions enhance reliability and understanding
Types structure the objects of a domain, and typing rules explain how they can be combined in meaningful ways.
Type systems. Types categorize data and operations, allowing compilers and runtime systems to catch errors and optimize code. Benefits of strong typing include:
- Early error detection
- Improved code readability
- Better documentation
- Enhanced refactoring support
Abstraction mechanisms. Programming languages provide various abstraction tools:
- Functions: Encapsulate reusable computations
- Classes and objects: Bundle data with related operations
- Modules: Organize code into logical units
- Interfaces: Define contracts for interaction
Software engineering impact. Types and abstractions are fundamental to building large, reliable software systems. They enable:
- Modular development: Teams can work on separate components
- Code reuse: Well-designed abstractions can be applied in multiple contexts
- Scalability: Systems can grow without becoming unmanageable
- Maintainability: Changes can be made with confidence in their impact
By providing a framework for organizing and reasoning about code, types and abstractions form the backbone of modern software engineering practices.
Last updated:
Review Summary
Once Upon an Algorithm receives mixed reviews. Readers appreciate its creative approach of using stories to explain computing concepts, but some find the execution uneven. The academic writing style and stretched analogies can make it challenging for beginners. However, many praise its clever examples and thorough explanations of computer science fundamentals. Some reviewers note it works better as a textbook supplement than a standalone introduction. Overall, it's seen as an ambitious attempt to make algorithms accessible, though not always successful in achieving this goal.
Similar Books
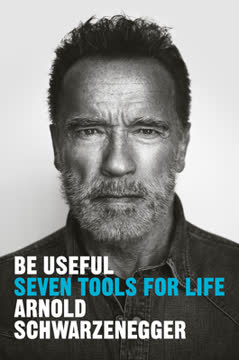
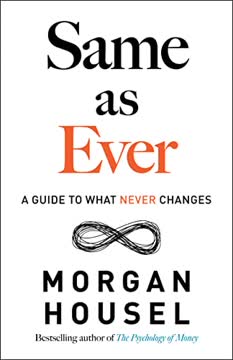
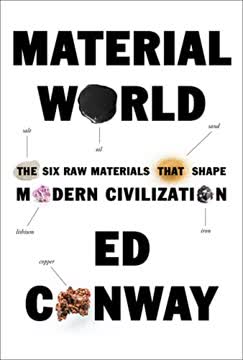
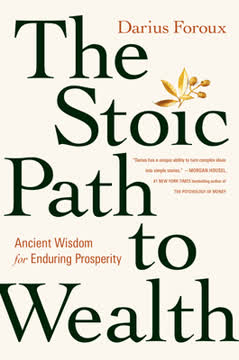
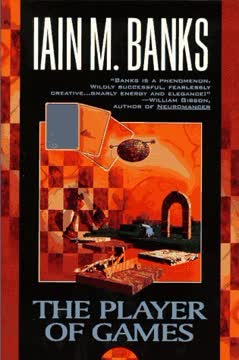
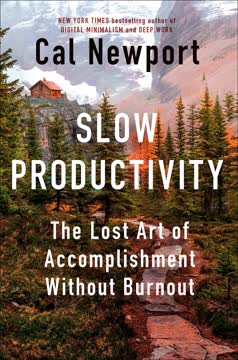
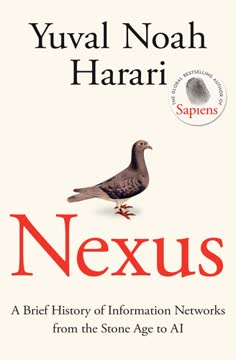
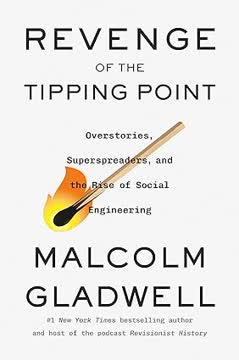
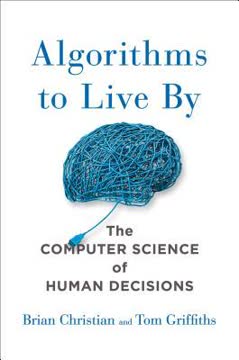
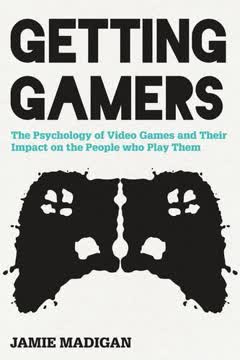
Download PDF
Download EPUB
.epub
digital book format is ideal for reading ebooks on phones, tablets, and e-readers.