Key Takeaways
1. Programming is Problem-Solving: Expressing Solutions for Computers
Programming is the art of expressing solutions to problems so that a computer can execute those solutions.
From Idea to Execution. Programming is fundamentally about translating human understanding into a language a computer can follow. It involves meticulously describing the steps required to solve a problem, accounting for every detail and potential scenario. This process demands precision, as computers lack the common sense to compensate for ambiguous instructions.
The Role of Programming Languages. Programming languages, like C++, provide the grammar and vocabulary needed to communicate with computers. These languages offer a structured way to define data and actions, enabling programmers to create complex programs from simple instructions. The goal is to teach the computer to perform complex tasks by providing a program.
Beyond Syntax: The Art of Problem Solving. While mastering the syntax of a programming language is essential, it's only one aspect of programming. The true art lies in understanding the problem, devising a solution, and expressing that solution in code. This requires a combination of analytical thinking, creativity, and attention to detail.
2. Objects, Types, and Values: The Foundation of Data Storage
A type defines a set of possible values and a set of operations (for an object).
Objects as Containers. To manipulate data, programs need to store it in memory. Objects serve as these storage locations, each having a specific type that dictates the kind of information it can hold. A named object is called a variable.
Types and Operations. The type of a variable determines not only the kind of data it can store (e.g., integers, floating-point numbers, characters, strings) but also the operations that can be performed on it. C++ offers a variety of built-in types, including int
, double
, char
, string
, and bool
, each with its own set of operators and behaviors.
Values and Literals. The data items stored in variables are called values. Each type has its own characteristic style of literals, such as 39
for integers, 3.5
for floating-point numbers, 'x'
for characters, and "Annemarie"
for strings. Understanding these basic concepts is crucial for writing programs that correctly store and manipulate data.
3. Computation: Expressions, Selection, and Iteration
The most basic building block of programs is an expression.
Expressions and Operators. Computation involves manipulating data to produce desired results. Expressions are the fundamental building blocks of computation, combining operands (data) with operators (actions) to calculate values. C++ provides a rich set of operators for arithmetic, comparison, logical operations, and more.
Selection: Choosing Among Alternatives. Programs often need to make decisions based on conditions. Selection statements, such as if
and switch
, allow programs to execute different code blocks depending on whether a condition is true or false. This enables programs to respond dynamically to different inputs and situations.
Iteration: Repeating Actions. Many tasks require repeating a set of actions multiple times. Iteration statements, such as while
and for
, provide a way to execute a block of code repeatedly until a certain condition is met. This is essential for processing large datasets, performing calculations, and creating dynamic behaviors.
4. Errors: The Inevitable Challenge and the Path to Robustness
The compiler is your friend; possibly, the compiler is the best friend you have when you program.
Types of Errors. Errors are an unavoidable part of programming. They can occur at various stages of the development process, including compile time (syntax and type errors), link time (missing definitions), and run time (logic errors and exceptions). Understanding the different types of errors is crucial for effective debugging.
The Compiler as a First Line of Defense. The compiler is a programmer's first line of defense against errors. It meticulously checks the code for syntax and type violations, preventing many mistakes from making it into the final program. While compilers can seem picky at times, their strictness ultimately saves programmers from a lot of self-inflicted problems.
Strategies for Error Handling. To produce robust software, programmers must adopt a proactive approach to error handling. This involves organizing code to minimize errors, using debugging and testing to eliminate most errors, and implementing mechanisms to handle the remaining errors gracefully. Type safety, initialization, and exception handling are key tools in this process.
5. Writing a Program: A Journey from Idea to Code
A program is written for two audiences.
The Programming Process. Writing a program is an iterative process that involves analysis, design, implementation, and testing. It's not simply about writing code; it's about understanding the problem, devising a solution, and expressing that solution in a clear, concise, and maintainable way.
The Importance of Readability. Code is not just for computers; it's also for humans. Writing readable code is crucial for collaboration, maintainability, and debugging. Comments, meaningful names, and consistent formatting all contribute to code that is easy to understand and modify.
The Value of Iteration. The best programs are rarely written in one go. Instead, they evolve through a series of versions, each building on the lessons learned from the previous one. This iterative approach allows programmers to refine their understanding of the problem and create more elegant and effective solutions.
6. Completing a Program: Refinement, Error Handling, and Style
Damn the torpedoes! Full speed ahead.
Beyond Basic Functionality. Once a program "basically works," the real work begins. Completing a program involves refining the user interface, implementing robust error handling, adding useful features, and restructuring the code for clarity and maintainability.
The Importance of Style. Code style is not just about aesthetics; it's about clarity, consistency, and maintainability. A well-styled program is easier to read, understand, and modify, reducing the likelihood of errors and making it easier to collaborate with other programmers.
The Role of Testing. Testing is an essential part of the software development process. It involves systematically exercising the program with a variety of inputs to identify errors and ensure that it meets its requirements. Testing should be an ongoing process, starting early in development and continuing throughout the program's lifecycle.
7. Technicalities: Functions, Declarations, and Scope
No amount of genius can overcome obsession with detail.
Functions as Building Blocks. Functions are the fundamental units of organization in C++. They allow programmers to break down complex tasks into smaller, more manageable pieces. Functions have a return type, a name, a parameter list, and a body.
Declarations and Definitions. A declaration introduces a name into a program, specifying its type. A definition, on the other hand, provides the full implementation of a function or the memory allocation for a variable. Every name must be declared before it can be used.
Scope: Controlling Visibility. Scope defines the region of program text where a name is valid. Different kinds of scopes (global, namespace, class, local) help to organize code and prevent name clashes. Keeping names as local as possible promotes code clarity and maintainability.
8. Technicalities: Classes, Encapsulation, and Abstraction
Code can be beautiful as well as useful.
User-Defined Types. Classes are the primary mechanism for creating user-defined types in C++. They allow programmers to define new data structures with their own data members and member functions. Classes are essential for representing complex concepts and building modular, reusable code.
Encapsulation: Hiding Implementation Details. Encapsulation involves bundling data and functions that operate on that data within a class, while hiding the internal implementation details from external users. This promotes data integrity, reduces dependencies, and makes code easier to maintain.
Abstraction: Simplifying Complexity. Abstraction involves presenting a simplified view of a complex system, hiding unnecessary details and focusing on essential features. Classes provide a powerful mechanism for abstraction, allowing programmers to create high-level interfaces that are easy to use and understand.
9. Input and Output Streams: Connecting Programs to the World
Science is what we have learned about how to keep from fooling ourselves.
The I/O Stream Model. The iostream library provides a unified and extensible framework for handling input and output in C++. It uses streams to represent sources and destinations of data, allowing programs to interact with a variety of devices and formats in a consistent way.
Files: Persistent Data Storage. Files are used to store data persistently on disks or other storage devices. The iostream library provides classes for reading from and writing to files, enabling programs to process large amounts of data and maintain information between program executions.
Error Handling: Anticipating the Unexpected. Input and output operations are prone to errors, such as invalid data formats, missing files, and device failures. Robust programs must anticipate these errors and handle them gracefully, providing informative error messages and preventing crashes.
10. A Display Model: Visualizing Data and User Interfaces
The world was black and white then. It didn´t turn color until sometime in the 1930s.
The Power of Graphics. Graphics provide a powerful way to visualize data, create engaging user interfaces, and enhance communication. By presenting information visually, programs can make complex concepts more accessible and intuitive.
The Pixel-Based Screen. Computer screens are composed of pixels, tiny spots of light that can be individually controlled. Graphics libraries provide tools for manipulating these pixels to create shapes, lines, text, and images.
The Window as a Canvas. A window is a rectangular area on the screen that serves as a canvas for drawing graphical objects. Programs can create and manipulate windows to display information and interact with users.
11. Graphics Classes: Building Blocks for Visual Representation
A language that doesn´t change the way you think isn´t worth learning.
Shapes as Fundamental Elements. Graphics libraries provide a set of basic shapes, such as lines, rectangles, circles, and polygons, that can be used to construct more complex graphical objects. These shapes serve as the building blocks for creating visual representations of data and user interfaces.
Attributes: Color and Style. Shapes can be customized by setting their attributes, such as color, line style, and fill color. These attributes allow programmers to control the appearance of graphical objects and create visually appealing displays.
Text and Images: Adding Information and Realism. Graphics libraries also provide tools for displaying text and images, allowing programs to incorporate textual information and realistic visuals into their displays. Text can be used to label axes, provide captions, and display instructions, while images can add realism and visual interest.
12. Class Design: Principles for Creating Effective Types
Functional, durable, beautiful.
Types as Representations of Concepts. Classes are used to represent concepts in code. A well-designed class should directly reflect the properties and behaviors of the concept it represents, making the code easier to understand and maintain.
Operations: Defining Behavior. Classes should provide a set of operations that allow users to interact with objects of that type in a meaningful way. These operations should be carefully chosen to be complete, minimal, and consistent.
Encapsulation: Protecting Data Integrity. Encapsulation involves hiding the internal implementation details of a class from external users, exposing only a well-defined interface. This protects the data from accidental corruption and allows the implementation to be changed without affecting user code.
13. Graphing Functions and Data: Visualizing Relationships
If it doesn’t have to produce correct results, I can make it arbitrarily fast.
The Importance of Visualization. Graphing data and functions is essential for understanding complex relationships and patterns. Visual representations can reveal insights that would be difficult or impossible to discern from raw data alone.
Axes: Providing Context. Axes provide a framework for interpreting graphs, defining the scale and units of measurement. Well-labeled axes are crucial for ensuring that graphs are clear and understandable.
Functions: Representing Mathematical Relationships. Functions can be graphed to visualize mathematical relationships and explore their properties. By plotting the values of a function over a range of inputs, programmers can gain insights into its behavior and characteristics.
14. Graphical User Interfaces: Enabling User Interaction
Computing is not about computers any more. It is about living.
Beyond Command Lines. Graphical user interfaces (GUIs) provide a more intuitive and user-friendly way to interact with programs than command-line interfaces. GUIs allow users to manipulate objects on the screen, press buttons, select from menus, and enter data in a variety of ways.
Widgets: Building Blocks of GUIs. Widgets are the basic building blocks of GUIs, providing interactive elements such as buttons, input boxes, output boxes, and menus. By combining these widgets, programmers can create complex and engaging user interfaces.
Callbacks: Responding to User Actions. Callbacks are functions that are executed in response to user actions, such as clicking a button or entering text in an input box. Callbacks enable programs to react dynamically to user input and provide a responsive user experience.
15. Vector and Free Store: Dynamic Memory Management
Use vector as the default!
The Need for Dynamic Memory. To create data structures that can grow and shrink as needed, programs must be able to allocate memory dynamically at runtime. This is achieved through the use of the free store, also known as the heap.
Pointers: Accessing Memory Directly. Pointers are variables that store memory addresses. They provide a low-level way to access and manipulate data in memory. However, pointers must be used with caution, as they can lead to memory leaks and other errors if not managed properly.
Destructors: Releasing Resources. Destructors are special functions that are automatically called when an object is destroyed. They are used to release any resources that the object may have acquired, such as memory allocated on the free store.
16. Arrays, Pointers, and References: Low-Level Memory Access
Caveat emptor!
Arrays: Contiguous Memory Blocks. Arrays are contiguous blocks of memory that can store a fixed number of elements of the same type. They provide efficient access to elements through subscripting but lack the flexibility of dynamic resizing.
Pointers: Navigating Memory. Pointers are variables that store memory addresses. They can be used to access and manipulate data in memory, but they also introduce the risk of memory errors if not used carefully.
References: Aliases for Objects. References provide an alternative way to access objects in memory. They are similar to pointers but offer a higher level of safety and convenience. References must be initialized to refer to an existing object and cannot be reassigned to refer to a different object.
17. Essential Operations: Managing Object Lifecycles
When someone says I want a programming language in which I need only say what I wish done, give him a lollipop.
The Importance of Control. To create robust and reliable programs, programmers must have precise control over the lifecycle of objects, including their creation, copying, moving, and destruction. This control is achieved through the use of essential operations.
Constructors: Initializing Objects. Constructors are special functions that are automatically called when an object is created. They are used to initialize the object's data members and establish its initial state.
Copy Operations: Creating Independent Copies. Copy constructors and copy assignment operators define how objects are copied. They are essential for ensuring that copies are independent of the original object and that changes to one do not affect the other.
Move Operations: Transferring Ownership. Move constructors and move assignment operators define how objects are moved. They are used to transfer ownership of resources from one object to another, avoiding unnecessary copying and improving performance.
Destructors: Releasing Resources. Destructors are special functions that are automatically called when an object is destroyed. They are used to release any resources that the object may have acquired, such as memory allocated on the free store.
18. Templates and Exceptions: Generic Programming and Error Handling
Success is never final.
Templates: Parameterizing Types. Templates provide a powerful mechanism for generic programming, allowing programmers to write code that works with a variety of types without having to write separate versions for each type. Templates enable code reuse and reduce code duplication.
Exceptions: Handling Errors Gracefully. Exceptions provide a structured way to handle errors in C++. They allow programs to separate the detection of errors from the handling of errors, making code more modular and easier to maintain.
Resource Management: Preventing Leaks. When dealing with dynamic memory allocation, it's crucial to ensure that all allocated memory is properly deallocated. Resource-management techniques, such as RAII (Resource Acquisition Is Initialization), help to prevent memory leaks and ensure that resources are released when they are no longer needed.
19. Containers and Iterators: The Power of the STL
Any problem in computer science can be solved with another layer of indirection. Except, of course, the problem of too many indirections.
The Standard Template Library (STL). The STL is a powerful and versatile part of the C++ standard library that provides a set of containers, algorithms, and iterators for working with data. The STL promotes code reuse, efficiency, and type safety.
Containers: Storing Data. Containers are data structures that hold collections of elements. The STL provides a variety of containers, such as vectors, lists, maps, and sets, each with its own strengths and weaknesses.
Iterators: Accessing Elements. Iterators are objects that provide a way to access the elements of a container. They provide a uniform interface for traversing different types of containers, allowing algorithms to work with a variety of data structures.
20. Maps and Sets: Associative Containers
Storing and processing data
Associative Containers. Maps and sets are associative containers that store elements in a sorted order. Maps store key-value pairs, while sets store unique elements. These containers provide efficient lookup, insertion, and deletion operations.
Maps: Key-Value Pairs. Maps are used to store associations between keys and values. They provide a way to look up a value based on its key. Maps are often used to implement dictionaries, symbol tables, and other data structures that require efficient key-based access.
Sets: Unique Elements. Sets are used to store unique elements in a sorted order. They provide a way to check for membership, insert new elements, and delete existing elements. Sets are often used to implement mathematical sets, collections of unique identifiers, and other data structures that require uniqueness.
21. Algorithms: The Power of the Standard Library
If it doesn´t have to produce correct results, I can make it arbitrarily fast.
Generic Algorithms. The STL provides a rich set of generic algorithms that can be used to perform common operations on containers, such as sorting, searching, copying, and transforming elements. These algorithms are designed to work with a variety of containers and element types, promoting code reuse and efficiency.
Function Objects: Customizing Algorithms. Function objects, also known as functors, are objects that can be called like functions. They provide a way to customize the behavior of STL algorithms, allowing programmers to specify their own comparison criteria, transformation functions, and other operations.
Numerical Algorithms. The STL also includes a set of numerical algorithms for performing mathematical operations on sequences of numbers, such as calculating sums, products, and inner products. These algorithms provide efficient and accurate ways to perform common numerical computations.
Last updated:
Review Summary
Programming: Principles and Practice Using C++ is generally well-regarded as an introduction to programming and C++. Many reviewers praise its comprehensive coverage, clear explanations, and practical exercises. It's considered suitable for beginners, though some find it challenging. The book's strengths include its focus on best practices, coverage of C++11/14 features, and the author's engaging writing style. Some criticisms mention inconsistent difficulty levels and outdated GUI examples. Overall, it's recommended for those seeking a solid foundation in programming and C++, especially with some prior coding experience.
Similar Books
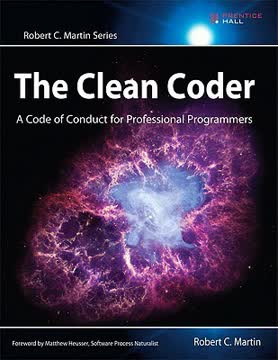
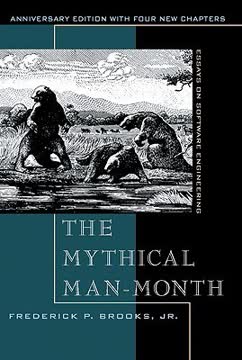
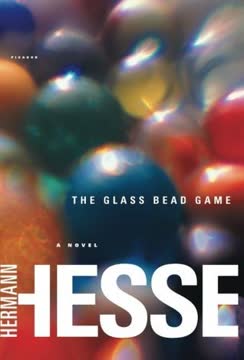
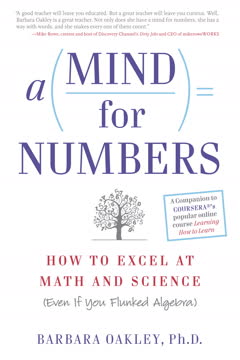
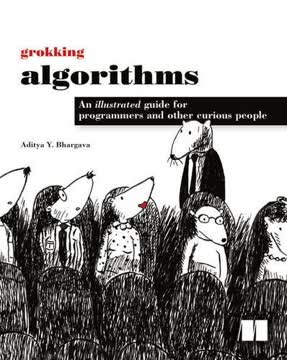
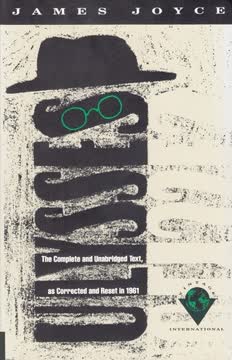
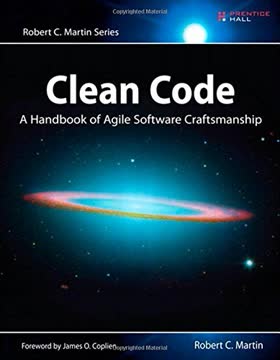
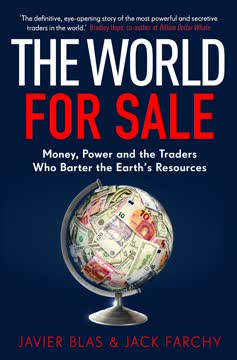
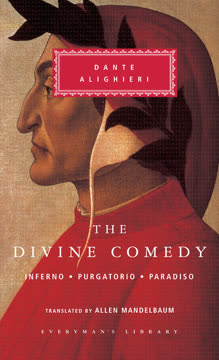
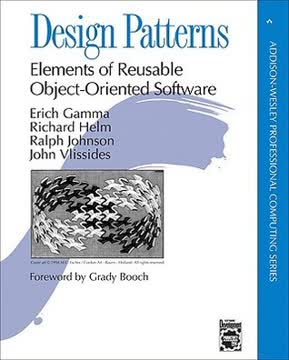
Download PDF
Download EPUB
.epub
digital book format is ideal for reading ebooks on phones, tablets, and e-readers.