Key Takeaways
1. Master Python's built-in data structures and functions
Python has long been a popular raw data manipulation language in part due to its ease of use for string and text processing.
Fundamental building blocks. Python's built-in data structures like lists, tuples, dictionaries, and sets form the foundation for data analysis. Lists and tuples store ordered sequences, while dictionaries and sets offer fast lookups and unique value storage. These structures support various operations:
- List operations: append, extend, insert, remove
- Dictionary methods: keys(), values(), items()
- Set operations: union, intersection, difference
Python's built-in functions, such as len(), range(), zip(), and enumerate(), provide powerful tools for data manipulation. List comprehensions offer a concise way to create new lists based on existing ones, often replacing traditional for loops.
2. Leverage NumPy for efficient numerical computing
NumPy internally stores data in a contiguous block of memory, independent of other built-in Python objects.
High-performance arrays. NumPy's ndarray is the cornerstone of numerical computing in Python, offering:
- Efficient storage and operations on large arrays
- Broadcasting capabilities for working with arrays of different shapes
- Vectorized operations that eliminate the need for explicit loops
NumPy's universal functions (ufuncs) provide fast element-wise array operations, such as np.sqrt(), np.exp(), and np.maximum(). These functions can operate on entire arrays at once, significantly improving performance compared to pure Python implementations.
Linear algebra operations, random number generation, and Fourier transforms are also available in NumPy, making it an essential tool for scientific computing and data analysis.
3. Utilize pandas for data manipulation and analysis
pandas will be a major tool of interest throughout much of the rest of the book.
Data structures for analysis. Pandas introduces two primary data structures:
- Series: 1-dimensional labeled array
- DataFrame: 2-dimensional labeled data structure with columns of potentially different types
These structures offer powerful indexing and data alignment capabilities. Key features include:
- Handling of missing data
- Merging and joining datasets
- Reshaping and pivoting data
- Time series functionality
Pandas excels at loading data from various sources (CSV, Excel, databases) and provides tools for data cleaning, transformation, and analysis. Its integration with NumPy allows for seamless transitions between data manipulation and numerical computations.
4. Create insightful visualizations with matplotlib and seaborn
matplotlib is a desktop plotting package designed for creating plots and figures suitable for publication.
Visual data exploration. Matplotlib provides a MATLAB-like plotting interface in Python, offering:
- Line plots, scatter plots, bar charts, histograms, and more
- Customizable plot elements (colors, labels, legends, etc.)
- Support for multiple plot types in a single figure
Seaborn, built on top of matplotlib, offers:
- Statistical data visualization
- Built-in themes for attractive plots
- High-level interface for common plot types
Together, these libraries enable the creation of publication-quality visualizations for data exploration and presentation. The integration with pandas allows for easy plotting of DataFrame and Series objects.
5. Handle time series data effectively
Time series data is an important form of structured data in many different fields, such as finance, economics, ecology, neuroscience, and physics.
Temporal data analysis. Pandas provides robust tools for working with time-based data:
- DatetimeIndex and PeriodIndex for time-based indexing
- Resampling and frequency conversion
- Rolling window calculations
- Time zone handling
These features allow for efficient analysis of time series data, including:
- Date range generation
- Shifting data
- Lagging and leading operations
- Period-based analysis
The ability to handle various time frequencies (daily, monthly, quarterly) and perform calendar-based calculations makes pandas particularly useful for financial and economic data analysis.
6. Perform data aggregation and group operations
Categorizing a dataset and applying a function to each group, whether an aggregation or transformation, can be a critical component of a data analysis workflow.
Group-based analysis. Pandas' groupby functionality enables powerful data aggregation and transformation:
- Splitting data into groups based on one or more keys
- Applying functions to each group
- Combining results into a new data structure
Common operations include:
- Aggregations: sum, mean, count, etc.
- Transformations: standardization, ranking, etc.
- Custom functions applied to groups
This functionality is particularly useful for summarizing large datasets, computing group-level statistics, and performing complex data transformations based on categorical variables.
7. Integrate pandas with modeling libraries
pandas is generally oriented toward working with arrays of dates, whether used as an axis index or a column in a DataFrame.
Data preparation for modeling. Pandas facilitates the transition between data manipulation and statistical modeling:
- Easy conversion between pandas objects and NumPy arrays
- Support for categorical data and dummy variable creation
- Integration with Patsy for model formula specification
These features allow for seamless integration with modeling libraries like statsmodels and scikit-learn. Pandas' data structures can be easily transformed into the format required by these libraries, streamlining the modeling process.
8. Explore statistical modeling with statsmodels
statsmodels is a Python library for fitting many kinds of statistical models, performing statistical tests, and data exploration and visualization.
Statistical analysis tools. Statsmodels offers a wide range of statistical models and tests:
- Linear regression models
- Time series analysis
- Generalized linear models
- Hypothesis tests
The library provides both a formula-based API (similar to R) and an array-based API, allowing for flexible model specification. It also offers comprehensive model diagnostics and results interpretation tools.
9. Implement machine learning with scikit-learn
scikit-learn is one of the most widely used and trusted general-purpose Python machine learning toolkits.
Machine learning workflows. Scikit-learn provides a consistent API for various machine learning tasks:
- Supervised learning: classification, regression
- Unsupervised learning: clustering, dimensionality reduction
- Model selection and evaluation
- Data preprocessing and feature engineering
Key features include:
- Consistent fit/predict API across models
- Cross-validation tools
- Pipeline creation for end-to-end workflows
- Extensive documentation and examples
The library's integration with pandas and NumPy allows for seamless incorporation of machine learning techniques into data analysis workflows.
Last updated:
FAQ
What's Python for Data Analysis about?
- Focus on Data Manipulation: The book is centered on manipulating, processing, cleaning, and analyzing data using Python. It provides a comprehensive guide to the Python programming language and its data-oriented library ecosystem.
- Tools and Libraries: It emphasizes essential libraries like pandas, NumPy, and Jupyter, which are crucial for data analysis tasks. These tools are foundational for anyone looking to become an effective data analyst.
- Practical Approach: The book is designed to be practical, offering hands-on examples and code snippets that readers can directly apply to their data analysis projects.
Why should I read Python for Data Analysis?
- Comprehensive Resource: The book is a key resource for university courses and professionals, covering essential tools and techniques for data analysis in Python.
- Authoritative Source: Written by Wes McKinney, the creator of pandas, it offers insights directly from an expert, making it a valuable resource.
- Updated Content: The third edition is updated with current versions of Python, NumPy, and pandas, ensuring readers learn the most relevant practices.
What are the key takeaways of Python for Data Analysis?
- Data Wrangling Skills: Readers will learn how to manipulate and clean data effectively using pandas, focusing on reshaping, merging, and aggregating data.
- Understanding NumPy: The book provides a solid foundation in NumPy, crucial for numerical computing in Python, enhancing data analysis capabilities.
- Visualization Techniques: It covers basic data visualization using matplotlib, allowing readers to present their data analysis results effectively.
What are the best quotes from Python for Data Analysis and what do they mean?
- "Python has become a popular and widespread language for data analysis.": Highlights Python's growing importance in data science, indicating its value for future career opportunities.
- "It’s a good idea to be familiar with the documentation for the various statistics or machine learning frameworks.": Emphasizes the importance of staying updated with the latest tools and libraries in the evolving field of data science.
- "The programming skills you have developed here will stay relevant for a long time into the future.": Reassures readers that the skills learned will remain applicable, making it a worthwhile endeavor.
How does Python for Data Analysis approach data wrangling?
- Step-by-Step Guidance: The book provides a structured approach to data wrangling, starting with data loading and cleaning, making it easy to follow.
- Use of Real Datasets: By using real datasets, it allows readers to practice data wrangling techniques in a realistic context, reinforcing concepts.
- Focus on pandas: It extensively covers pandas, detailing its functionalities for data manipulation, crucial for effective data wrangling in Python.
What are the essential Python libraries discussed in Python for Data Analysis?
- NumPy: Fundamental for numerical computing, providing support for multidimensional arrays and mathematical functions, essential for efficient data manipulation.
- pandas: Emphasized for data manipulation and analysis, particularly for working with structured data, introducing key data structures like Series and DataFrame.
- matplotlib: Used for creating visualizations, the book provides guidance on using it to visualize data effectively.
How does Python for Data Analysis help with data cleaning?
- Data Preparation Techniques: Covers techniques for cleaning and preparing data, including handling missing values, filtering, and transforming data.
- Using pandas for Cleaning: Provides practical examples of using pandas to clean data, such as removing duplicates and filling in missing values.
- Real-World Examples: Includes real-world datasets and scenarios, allowing readers to see how data cleaning is applied in practice.
What is the significance of the DataFrame in Python for Data Analysis?
- Tabular Data Structure: DataFrame is a two-dimensional, size-mutable, and potentially heterogeneous tabular data structure with labeled axes.
- Data Manipulation: Allows for easy manipulation of data, including filtering, grouping, and aggregating, with numerous examples provided.
- Integration with Other Libraries: Integrates well with other libraries like NumPy and matplotlib, facilitating complex data analysis tasks.
How does Python for Data Analysis address missing data?
- Identifying Missing Values: Discusses methods for identifying and handling missing data, emphasizing the importance of recognizing missing values.
- Filling and Dropping: Covers techniques for filling missing values and dropping rows or columns with missing data, allowing for dataset-specific approaches.
- Using pandas Functions: Demonstrates how to use pandas functions like
isna()
andfillna()
to manage missing data effectively.
What is the groupby method in pandas as explained in Python for Data Analysis?
- Data Aggregation: The
groupby
method is used to split data into groups based on criteria, allowing for aggregation and transformation. - Flexible Grouping: Supports grouping by one or more columns, with various aggregation functions like mean, sum, and count.
- Example Usage: For instance,
df.groupby("key").mean()
computes the mean of each group defined by unique values in the "key" column.
How can I create a pivot table in pandas as described in Python for Data Analysis?
- Using
pivot_table
: Allows summarizing data by one or more keys, arranging data in a rectangular format. - Aggregation Functions: Specify aggregation functions like mean, sum, or count to compute statistics for the pivot table.
- Example:
df.pivot_table(index="day", columns="smoker", values="tip_pct", aggfunc="mean")
creates a pivot table showing average tip percentages by day and smoking status.
How do I visualize data using pandas as per Python for Data Analysis?
- Built-in Plotting: Pandas has built-in plotting capabilities through the
plot
attribute, simplifying visualizations directly from DataFrames and Series. - Integration with Matplotlib: Integrates well with matplotlib, allowing for customization of plots using its extensive features.
- Example:
df.plot(kind="bar")
creates a bar plot of the DataFrame, demonstrating the ease of visualization with pandas.
Review Summary
Python for Data Analysis receives mostly positive reviews for its comprehensive coverage of pandas and data manipulation in Python. Readers praise its practical examples and clear explanations, particularly for those transitioning from other languages. Some criticize the focus on pandas over broader data analysis concepts and the use of random datasets. The book is considered valuable for learning data wrangling but may be too verbose for experienced users. Overall, it's seen as a useful resource for mastering pandas and Python-based data analysis.
Similar Books
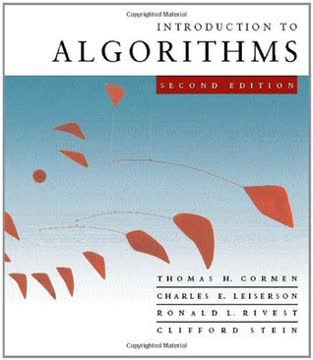
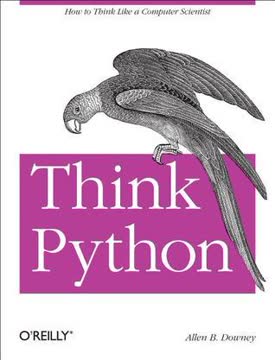
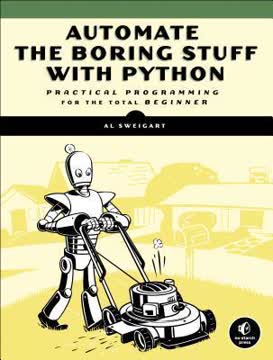
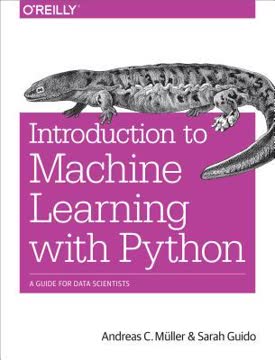
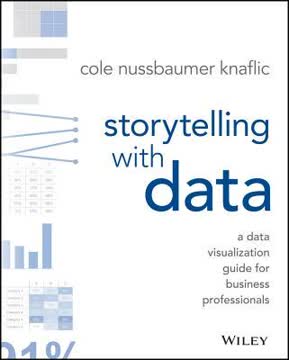
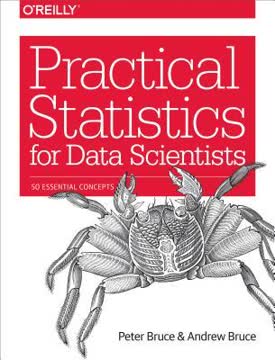
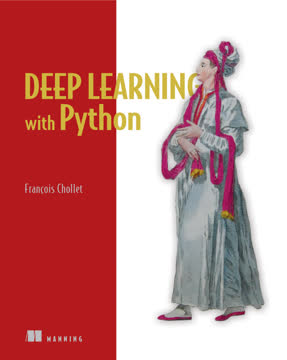
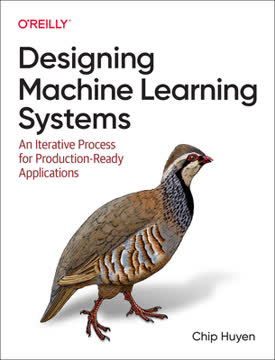
Download PDF
Download EPUB
.epub
digital book format is ideal for reading ebooks on phones, tablets, and e-readers.