Key Takeaways
1. Clean code is essential for sustainable software development
"Clean code always looks like it was written by someone who cares."
Code quality matters. Clean code is not just about aesthetics; it's about creating software that can be easily understood, maintained, and extended over time. When code is clean, it becomes a pleasure to work with, reducing the cognitive load on developers and allowing them to focus on solving problems rather than deciphering cryptic implementations.
Technical debt is costly. Neglecting code cleanliness leads to accumulating technical debt, which can severely impede future development and cause projects to grind to a halt. By consistently writing clean code, teams can avoid the pitfalls of rushed, messy implementations and ensure their software remains adaptable to changing requirements.
Characteristics of clean code:
- Readable and expressive
- Well-organized and structured
- Follows consistent conventions
- Easy to understand and modify
- Thoroughly tested
2. Meaningful names are crucial for code readability and maintainability
"The name of a variable, function, or class, should answer all the big questions."
Names convey intent. Choosing appropriate names for variables, functions, and classes is one of the most critical aspects of writing clean code. Good names should clearly communicate the purpose and behavior of the code element they represent, making it easier for other developers (including your future self) to understand the code's functionality at a glance.
Avoid cryptic abbreviations. While it may be tempting to use short, abbreviated names to save typing, this often leads to confusion and reduced readability. Opt for descriptive, unambiguous names that fully convey the meaning and purpose of the code element. Remember that modern IDEs make it easy to work with longer names through auto-completion and refactoring tools.
Guidelines for choosing good names:
- Use intention-revealing names
- Avoid disinformation or misleading names
- Make meaningful distinctions
- Use pronounceable names
- Use searchable names
- Avoid encodings or prefixes (e.g., Hungarian notation)
- Choose names at the appropriate level of abstraction
3. Functions should be small, focused, and do one thing well
"Functions should do one thing. They should do it well. They should do it only."
Small is beautiful. Keep functions short and focused on a single task or concept. Ideally, functions should be no more than 20 lines long, with most being much shorter. This makes them easier to understand, test, and maintain. When a function grows too large or tries to do too much, it's a sign that it should be broken down into smaller, more focused functions.
Single responsibility principle. Each function should have a clear, singular purpose. If you find yourself using words like "and" or "or" to describe what a function does, it's likely doing too much and should be split into multiple functions. This approach not only makes the code more readable but also more reusable and easier to refactor.
Characteristics of good functions:
- Do one thing
- Have descriptive names
- Take few arguments (ideally none to three)
- Don't use flag arguments
- Have no side effects
- Follow the Single Responsibility Principle
- Operate at a single level of abstraction
4. Comments should be used sparingly and only when necessary
"The proper use of comments is to compensate for our failure to express ourselves in code."
Code should be self-explanatory. Strive to make your code so clear and expressive that it doesn't need comments to explain what it's doing. Good code with clear names and structure is often more effective than comments in conveying intent. When you feel the need to write a comment, first consider if you can refactor the code to make it more self-evident.
Comments can become outdated. One of the dangers of relying on comments is that they can become outdated as the code evolves, leading to confusion and misunderstandings. By focusing on writing clear, self-documenting code, you reduce the risk of misleading future developers (including yourself) with stale comments.
Appropriate uses of comments:
- Legal comments (copyright, etc.)
- Explaining intent when it can't be expressed in code
- Clarifying complex algorithms or formulas
- Warning of consequences
- TODO comments (sparingly)
- Javadoc for public APIs
5. Proper formatting enhances code readability and comprehension
"Code formatting is about communication, and communication is the professional developer's first order of business."
Consistency is key. Establish and adhere to a consistent formatting style throughout your codebase. This includes conventions for indentation, line breaks, whitespace, and organization of code elements. Consistent formatting makes it easier for developers to quickly scan and understand code, reducing cognitive load and improving productivity.
Vertical formatting matters. Organize your code vertically to enhance readability. Keep related concepts close together and separate unrelated concepts. Use blank lines to create "paragraphs" in your code, grouping related statements and separating different thoughts. This vertical organization helps readers quickly grasp the structure and flow of the code.
Formatting guidelines:
- Consistent indentation (spaces or tabs)
- Proper line breaks and whitespace
- Vertical alignment of related elements
- Logical grouping of related code
- Consistent naming conventions
- Limiting line length (e.g., 80-120 characters)
- Proper placement of braces and parentheses
6. Error handling should be thorough and separate from main logic
"Error handling is important, but if it obscures logic, it's wrong."
Separate concerns. Error handling code should be separated from the main logic of your functions. This separation makes both the happy path and the error handling clearer and easier to understand. Consider using exceptions to handle errors, as they allow you to keep the main logic clean and uncluttered.
Be specific and informative. When errors occur, provide clear and specific error messages that help identify the problem and potential solutions. Avoid generic error messages that leave users or other developers guessing about what went wrong. Additionally, consider logging detailed error information for debugging purposes.
Error handling best practices:
- Use exceptions rather than return codes
- Create informative error messages
- Define exception classes in terms of a caller's needs
- Don't return null
- Don't pass null
- Provide context with exceptions
- Wrap third-party APIs to handle their specific exceptions
7. Unit tests are vital for maintaining code quality and facilitating changes
"Test code is just as important as production code."
Tests enable confidence. A comprehensive suite of unit tests provides confidence that your code works as expected and allows you to make changes without fear of breaking existing functionality. Tests act as a safety net, catching regressions and ensuring that your code continues to behave correctly as it evolves.
Write tests first. Following the Test-Driven Development (TDD) approach, write tests before implementing the actual code. This practice helps you think through the design and requirements of your code before diving into implementation. It also ensures that your code is testable from the start, promoting better design and more modular, loosely coupled code.
Characteristics of good unit tests:
- Fast: Tests should run quickly
- Independent: Tests should not depend on each other
- Repeatable: Tests should produce the same results each time
- Self-validating: Tests should have a boolean output (pass/fail)
- Timely: Tests should be written just before the production code
- Readable and maintainable
- Cover edge cases and boundary conditions
8. Classes should be small, focused, and adhere to single responsibility principle
"The first rule of classes is that they should be small. The second rule of classes is that they should be smaller than that."
Single Responsibility Principle. Each class should have one, and only one, reason to change. This principle encourages you to create small, focused classes that are easier to understand, test, and maintain. When a class tries to do too much, it becomes difficult to modify and more prone to bugs.
Cohesion is crucial. Aim for high cohesion within your classes, meaning that the methods and properties of a class should be closely related and work together to fulfill the class's purpose. Low cohesion often indicates that a class is trying to do too much and should be split into multiple smaller, more focused classes.
Guidelines for creating good classes:
- Keep classes small (aim for 100-200 lines)
- Follow the Single Responsibility Principle
- Maintain high cohesion between methods and properties
- Limit the number of instance variables
- Prefer composition over inheritance
- Use dependency injection to manage dependencies
- Follow the Open-Closed Principle (open for extension, closed for modification)
9. Systems should be designed with clean boundaries and separation of concerns
"An optimal system architecture consists of modularized domains of concern, each of which is implemented with Plain Old Java (or other) Objects."
Clear boundaries. Design your system with clear boundaries between different components or modules. This separation allows each part of the system to evolve independently and makes it easier to understand, test, and maintain individual components. Use interfaces and abstract classes to define these boundaries and hide implementation details.
Dependency management. Carefully manage dependencies between different parts of your system. Use dependency injection and inversion of control to decouple components and make them more modular. This approach makes it easier to change or replace parts of the system without affecting others.
Strategies for clean system design:
- Use layered architecture (e.g., presentation, business logic, data access)
- Apply the Dependency Inversion Principle
- Utilize design patterns to solve common problems
- Create abstractions to hide implementation details
- Use aspect-oriented programming for cross-cutting concerns
- Implement a plugin architecture for extensibility
- Separate configuration from code
10. Continuous improvement through refactoring is key to maintaining clean code
"The act of writing clean code is an exercise in taking something that works and cleaning it until it conforms to the rules."
Refactoring is ongoing. Clean code is not achieved in a single pass but through continuous improvement and refactoring. As you gain new insights or as requirements change, be prepared to revisit and refine your code. Regular refactoring helps prevent the accumulation of technical debt and keeps your codebase maintainable.
Boy Scout Rule. Follow the Boy Scout Rule: "Leave the campground cleaner than you found it." Whenever you work on a piece of code, make an effort to improve it, even if it's just a small enhancement. Over time, these small improvements add up to significant gains in code quality and maintainability.
Refactoring techniques:
- Extract method: Break long methods into smaller, focused ones
- Rename: Improve names of variables, methods, and classes
- Move method: Relocate methods to more appropriate classes
- Extract class: Split large classes into smaller, focused ones
- Inline method: Simplify code by removing unnecessary abstractions
- Replace conditional with polymorphism
- Introduce parameter object: Group related parameters
- Remove duplication: Eliminate repeated code
Last updated:
Review Summary
The Robert C. Martin Clean Code Collection receives mostly positive reviews, with an average rating of 4.48/5. Readers praise it as essential for software developers, offering valuable insights on coding practices and professional behavior. Many consider it a must-read, appreciating its guidance on clean code and craftsmanship. Some criticize it for being opinionated and outdated in parts. The book's focus on Java and specific programming paradigms is noted. Overall, it's seen as beneficial for both new and experienced programmers, though some suggest reading it with a critical mindset.
Similar Books
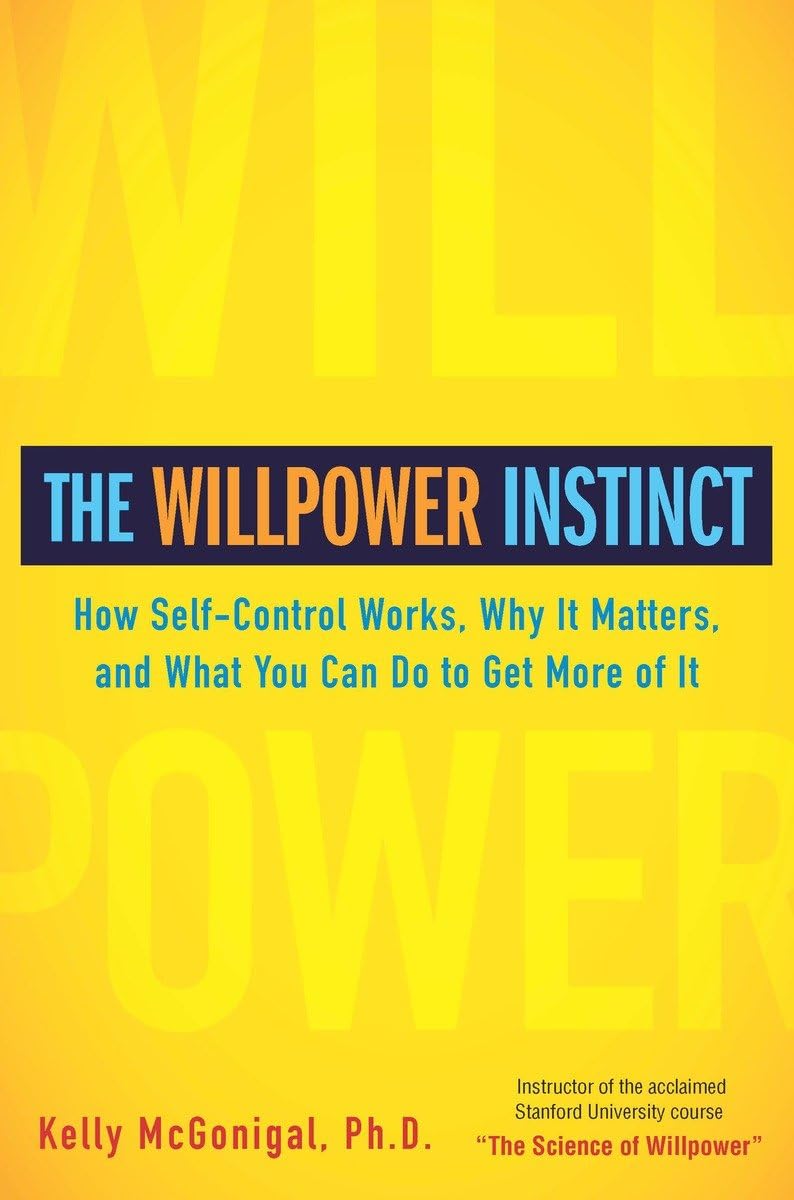
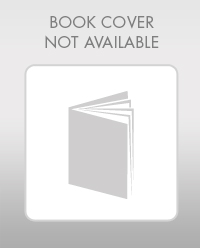
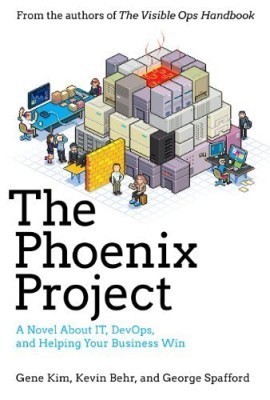
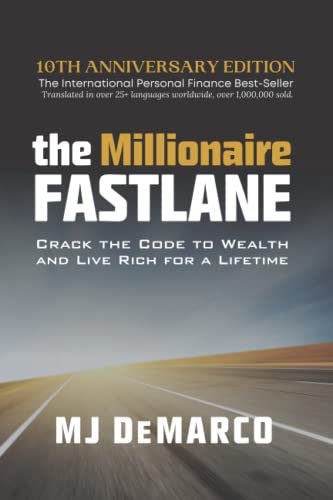
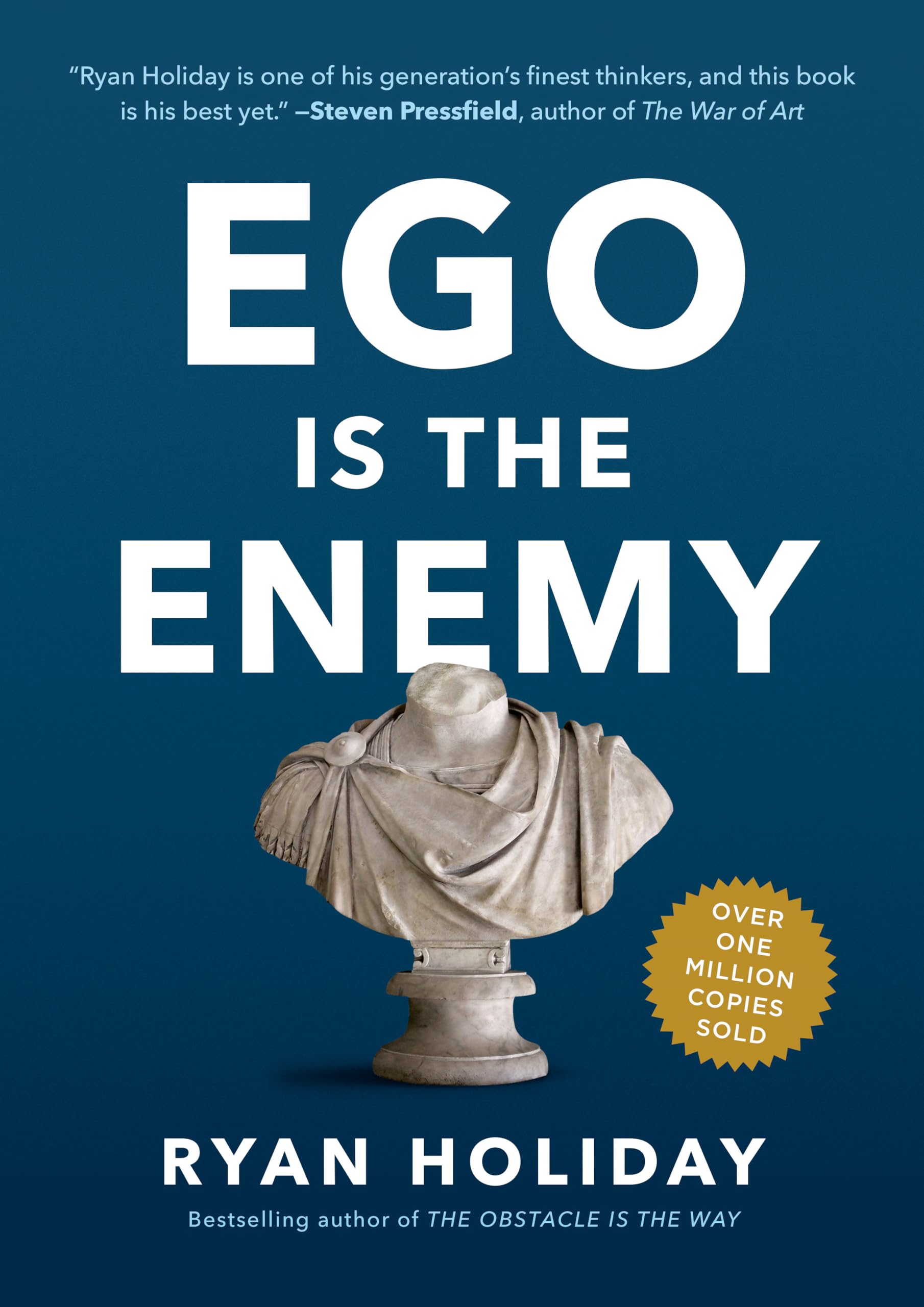
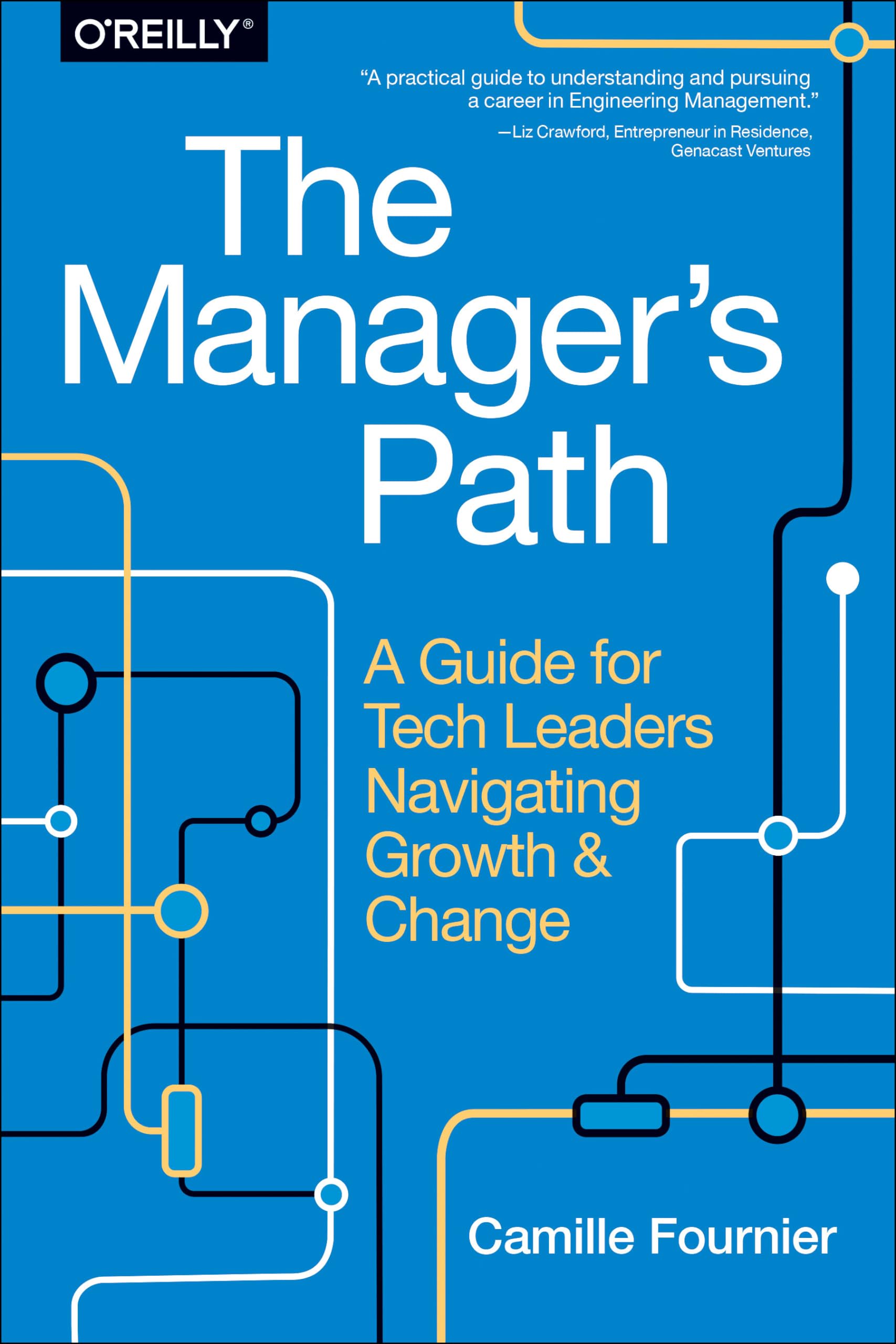
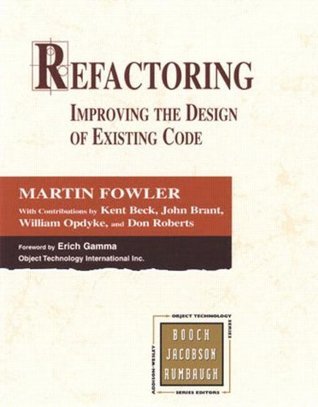
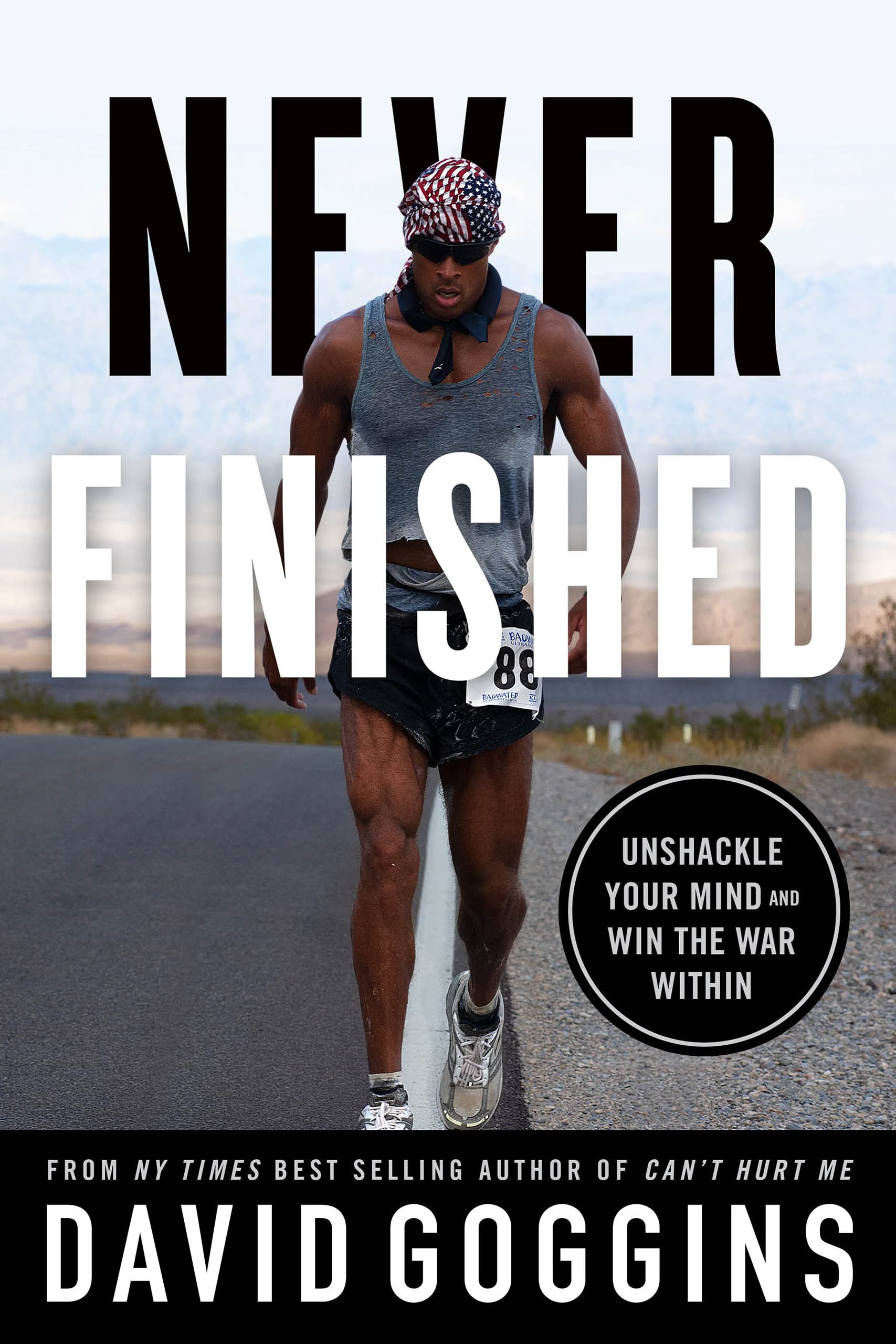
Download PDF
Download EPUB
.epub
digital book format is ideal for reading ebooks on phones, tablets, and e-readers.