Key Takeaways
1. Problem Solving is More Than Just Knowing Syntax
In essence, most programming books for beginners teach how to read a program, not how to write one.
Syntax vs. Problem Solving. Many aspiring programmers struggle because they focus solely on learning the syntax of a programming language, mistaking it for the ability to write original programs. Understanding syntax is essential, but it's merely the first step. The real challenge lies in problem-solving: the ability to translate a problem description into a working program.
Creative vs. Analytical Skills. Problem-solving requires a different set of mental muscles than learning syntax. Syntax acquisition is primarily an analytical, "left-brain" activity, involving memorization and comprehension. Writing original code, on the other hand, is a creative, "right-brain" activity, demanding ingenuity and the ability to combine learned tools in novel ways.
Beyond Cookbooks. Relying solely on "cookbook" examples or pre-written recipes can hinder the development of true problem-solving skills. While such resources can be valuable time-savers, they don't foster the deep understanding of ingredients, preparation methods, and cooking techniques that allows a great cook to create original meals. Similarly, a great programmer understands language syntax, application frameworks, algorithms, and software engineering principles, enabling them to craft original programs from specifications.
2. Formal Restatement Unlocks Hidden Solutions
Restating the problem in a more formal manner is a great technique for gaining insight into a problem.
Gaining New Perspectives. Restating a problem in different terms or from a different angle can reveal solutions that were previously hidden. It's like examining a hill from all sides before attempting to climb it, searching for the easiest path to the summit. This technique can clarify the true goal, which may not be what it initially seemed.
Constraints and Operations. Formal restatement involves identifying the constraints (unbreakable rules) and operations (possible actions) within the problem. By explicitly listing these elements, you can uncover potential solutions that might have been overlooked. For example, in the classic "fox, goose, and corn" riddle, formally stating the constraints and operations reveals the possibility of taking an item back across the river, a move that many initially fail to consider.
Thinking vs. Solving. Thinking about the problem itself can be as productive, or even more productive, than thinking about the solution. By formally restating the problem, you force yourself to analyze its components and relationships, often leading to valuable insights and a clearer path to a solution.
3. Divide and Conquer: Break Down Complexity
Finding a way to divide a problem into steps or phases can make the problem much easier.
Reducing Difficulty. Dividing a problem into smaller, more manageable pieces can significantly reduce its overall difficulty. The effort required to solve each piece is often far less than the effort required to solve the entire problem at once. This is because combining programming techniques is much trickier than using techniques alone.
Sorting Analogy. Consider alphabetizing 100 files. Alphabetizing four groups of 25 files individually is far less work than alphabetizing the entire set of 100 at once. This is because the work involved in inserting a single file grows as the number of files already filed grows.
Sequential vs. Combined Techniques. Code that employs a series of if
statements inside a while
loop that is itself inside a for
loop will be more difficult to write and read than a section of code that employs all those same control statements sequentially. By dividing the problem, you can simplify the code and reduce the cognitive load.
4. Analogies Bridge the Gap Between Problems
Expert problem solvers are quick to recognize an analogy, an exploitable similarity between a solved problem and an unsolved problem.
Exploiting Similarities. Recognizing analogies, or similarities between a current problem and a previously solved one, is a powerful problem-solving technique. The similarity may involve only a part of the problems, but it can provide valuable insights and shortcuts.
Quarrasi Lock Example. The Quarrasi lock problem, disguised with extraneous details and alien technology, is essentially the same as the "fox, goose, and corn" riddle. Recognizing this analogy allows you to translate the solution from the first problem rather than creating a new one.
Building a Solution Library. The more problems you solve and fully understand, the larger your storehouse of potential analogies becomes. This is why it's crucial to avoid shortcuts like copying code you don't fully understand. Every successful program you write is more than a solution to a current problem; it's a potential source of analogies to solve future problems.
5. Planning Prevents Frustration and Guides Progress
You must always have a plan, rather than engaging in directionless activity.
Eisenhower's Wisdom. "I have always found that plans are useless, but planning is indispensable." While the specifics of a plan may change as you encounter unforeseen challenges, the act of planning itself is crucial for success.
Setting Intermediate Goals. Without a plan, you have only one goal: solve the whole problem. This can lead to frustration, as there is no positive reinforcement from your efforts until the end. A plan allows you to set intermediate goals, track your progress, and build confidence.
Fleming's Discovery. The discovery of penicillin wasn't a lucky accident; it was the result of thorough and controlled experimentation. Planning allows you to recognize the importance of unexpected events and turn them into valuable insights.
6. Arrays: Fundamental Tools for Data Organization
The topics covered in this book represent areas in which I have most often seen new programmers struggle.
Collections of Variables. Arrays are fundamental data structures that organize variables of the same type under a single name, accessed by a numerical index. This organization enables efficient storage and retrieval of related data.
Basic Operations. Key array operations include storing values, copying arrays (or portions thereof), retrieving values by index, searching for specific values, sorting elements into a desired order, and computing statistics based on the array's contents.
Beyond Simple Data. Arrays can store complex data types, such as structures or objects, allowing you to organize and manipulate collections of related information. This versatility makes arrays a powerful tool for solving a wide range of programming problems.
7. Pointers: Mastering Dynamic Memory Management
C++ is the real deal — it’s programming without training wheels.
Dynamic Allocation. Pointers enable dynamic memory allocation, allowing you to create data structures with sizes determined at runtime. This flexibility is crucial when dealing with data sets of unknown or variable size.
Memory Sharing. Pointers facilitate memory sharing, allowing different parts of a program to access the same data without the overhead of copying. This can significantly improve performance, especially when working with large data structures.
Responsibility. Mastering pointers requires careful attention to memory management. You must allocate memory when needed, track pointers to allocated memory, and deallocate memory when it's no longer in use to avoid memory leaks and other errors.
8. Classes: Encapsulation for Reusability and Clarity
By studying these approaches, you can unlock your creativity.
Combining Data and Code. Classes encapsulate data and code that operates on that data into a single, reusable unit. This promotes modularity, organization, and code reuse.
Information Hiding. Classes enforce information hiding by controlling access to data members, preventing direct manipulation from outside the class. This protects the integrity of the data and allows you to change the implementation without affecting client code.
Extending the Language. Classes extend the language by creating new data types with custom behaviors. This allows you to model real-world objects and concepts in your code, making it more readable and maintainable.
9. Recursion: Elegant Solutions for Complex Structures
This book isn’t going to tell you precisely what to do; it’s going to help you develop your latent problem-solving abilities so that you will know what you should do.
Self-Referential Functions. Recursion is a technique where a function calls itself, either directly or indirectly. This allows you to solve complex problems by breaking them down into smaller, self-similar subproblems.
Base Cases and Recursive Steps. A recursive function must have a base case, which is a condition that stops the recursion and returns a value directly. It must also have a recursive step, which calls the function itself with a modified input.
Head vs. Tail Recursion. Head recursion performs the recursive call before other processing, while tail recursion performs the recursive call after other processing. The choice between the two can affect the efficiency and readability of the code.
10. Code Reuse: Balancing Efficiency and Understanding
The chief lesson here is the importance of recognizing analogies.
Avoiding Reinventing the Wheel. Code reuse is essential for efficient software development. Instead of writing everything from scratch, you can leverage existing components, such as algorithms, patterns, abstract data types, and libraries.
Good vs. Bad Reuse. Good reuse involves understanding the underlying concepts and adapting them to your specific problem. Bad reuse involves blindly copying code without understanding it, which can lead to errors and maintenance difficulties.
Building a Component Library. Strive to build a personal library of well-understood components that you can reuse in future projects. This will significantly increase your productivity and improve the quality of your code.
11. Master Your Strengths, Mitigate Your Weaknesses
My goal is for you and every other reader of this book to learn to systematically approach every programming task and to have the confidence that you will ultimately solve a given problem.
Self-Awareness is Key. The most effective problem-solving approach is one that leverages your strengths and compensates for your weaknesses. This requires honest self-appraisal and a willingness to adapt your techniques accordingly.
Coding and Design Weaknesses. Identify your common coding errors and design flaws. This will allow you to develop strategies to avoid these pitfalls and improve the overall quality of your code.
Leverage Your Talents. Recognize your programming strengths and design your master plan to maximize their impact. This will not only lead to better results but also make the problem-solving process more enjoyable and rewarding.
Last updated:
Review Summary
Think Like a Programmer receives mixed reviews, with an average rating of 3.86 out of 5. Readers appreciate its problem-solving approach and exercises, finding it useful for developing programming skills. Some praise the author's explanations and consider it a valuable resource for beginners. However, critics argue that the book's reliance on C++ limits its accessibility, and some find the content too basic or academic. The book's focus on logical thinking and breaking down problems is generally well-received, though opinions vary on its effectiveness for experienced programmers.
Similar Books
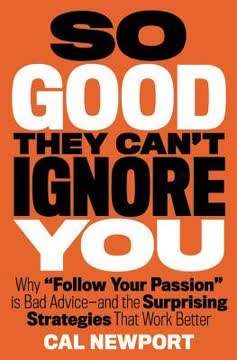
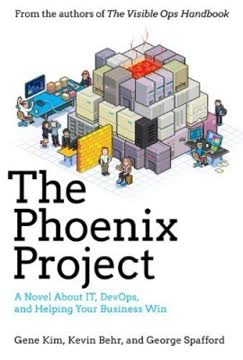
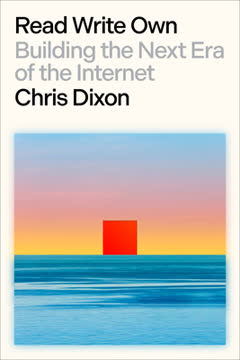
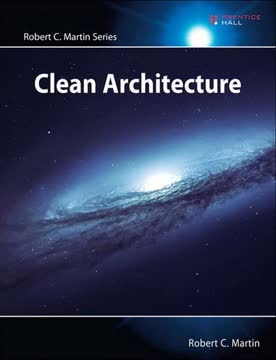
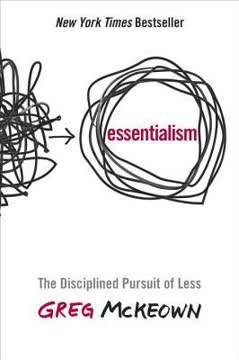
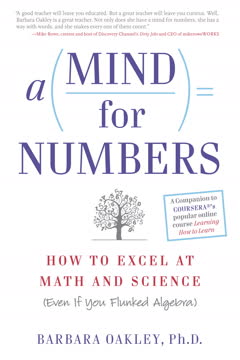
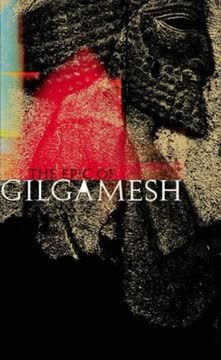
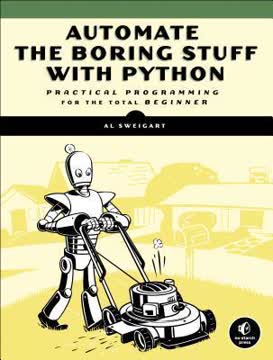
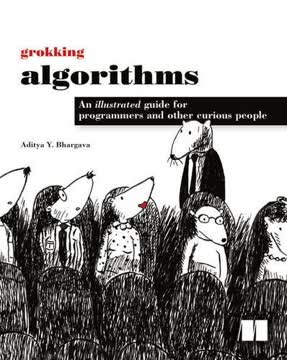
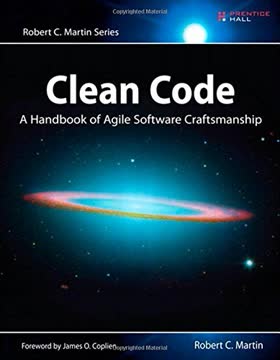
Download PDF
Download EPUB
.epub
digital book format is ideal for reading ebooks on phones, tablets, and e-readers.