Key Takeaways
1. Data Structures and Algorithms are Foundational for Efficient Code
Having the ability to write code that runs quickly is an important aspect of becoming a better software developer.
Efficiency Matters. Writing code that simply "works" is not enough. Experienced developers strive for code that is both maintainable and efficient. Efficiency, in this context, refers to how quickly the code executes and how effectively it utilizes resources. Data structures and algorithms are the building blocks for achieving this efficiency.
Organization Impacts Speed. The way data is organized (data structures) and the methods used to manipulate it (algorithms) have a profound impact on the speed of code. Choosing the right data structure and algorithm can make a difference of orders of magnitude in performance, especially when dealing with large datasets or high-traffic applications.
Arrays and Sets. Arrays and sets, while seemingly similar, demonstrate how data organization affects efficiency. Arrays allow direct access to elements via index, while sets enforce uniqueness. These differences lead to variations in the speed of operations like searching and insertion.
2. Time Complexity is Measured by Steps, Not Time
If you take away just one thing from this book, let it be this: when we measure how “fast” an operation takes, we do not refer to how fast the operation takes in terms of pure time, but instead in how many steps it takes.
Steps vs. Time. The true measure of an algorithm's speed is not the time it takes to execute, which can vary based on hardware, but the number of computational steps required. This provides a consistent and reliable metric for comparing algorithms.
Hardware Independence. Measuring speed in terms of steps allows for a hardware-independent comparison. An algorithm that takes fewer steps will generally be faster than one that takes more steps, regardless of the machine it's running on.
Time Complexity. The number of steps an operation takes is also known as its time complexity. Terms like speed, efficiency, performance, and runtime are used interchangeably to refer to this concept.
3. Big O Notation Classifies Algorithm Efficiency
Big O achieves consistency by focusing on the number of steps an algorithm takes, but in a specific way.
Standardized Measurement. Big O Notation provides a standardized way to express the efficiency of algorithms. It focuses on how the number of steps an algorithm takes grows relative to the input size (N).
Key Question. The core of Big O is answering the question: "If there are N data elements, how many steps will the algorithm take?" The answer is expressed within the parentheses of the Big O notation, such as O(N) or O(1).
Common Examples:
- O(1): Constant time, the algorithm takes the same number of steps regardless of input size.
- O(N): Linear time, the number of steps grows proportionally to the input size.
- O(log N): Logarithmic time, the number of steps increases much slower as the input size grows.
4. Big O Notation Ignores Constants and Lower Orders
Big O Notation does more than simply describe the number of steps an algorithm takes, such as with a hard number like 22 or 400. Rather, it’s an answer to that key question on your forehead: if there are N data elements, how many steps will the algorithm take?
Focus on Growth. Big O Notation is primarily concerned with how an algorithm's performance scales as the input data increases. It emphasizes the long-term trend rather than precise step counts.
Ignoring Constants. Constant factors are dropped in Big O Notation. An algorithm that takes 3N steps is still considered O(N). This is because the constant factor becomes insignificant as N grows very large.
Ignoring Lower Orders. When multiple orders of N are present, only the highest order is considered. For example, an algorithm that takes N^2 + N steps is simplified to O(N^2). This is because the N^2 term dominates the growth rate as N increases.
5. Sets Offer Unique Efficiency Trade-offs
Sets are important when you need to ensure that there is no duplicate data.
Uniqueness Constraint. Sets, unlike arrays, enforce the constraint that no duplicate values can be stored. This property is useful in scenarios where data integrity is paramount.
Insertion Overhead. The uniqueness constraint introduces an overhead during insertion. Before adding a new value, the set must first search to ensure that the value doesn't already exist. This search operation can impact insertion efficiency.
Trade-offs. While sets may have slower insertion compared to arrays, their ability to prevent duplicates can be crucial in certain applications. The choice between arrays and sets depends on the specific needs of the application.
6. Ordered Arrays Enable Binary Search
The big advantage of an ordered array over a classic array is that an ordered array allows for an alternative searching algorithm.
Maintaining Order. Ordered arrays require that values are always kept in sorted order. This constraint affects insertion efficiency, as new values must be placed in the correct position.
Binary Search. The primary advantage of ordered arrays is the ability to use binary search. This algorithm repeatedly divides the search space in half, leading to a much faster search time compared to linear search.
Logarithmic Time. Binary search has a time complexity of O(log N), which is significantly faster than the O(N) time complexity of linear search, especially for large datasets. This makes ordered arrays ideal for applications where searching is a frequent operation.
7. Recursion Can Simplify Complex Problems
Recurse Instead of Loop
Self-Reference. Recursion is a programming technique where a function calls itself within its own definition. This allows for solving problems by breaking them down into smaller, self-similar subproblems.
Base Case. Every recursive function must have a base case, which is a condition that stops the recursion and prevents it from running indefinitely. Without a base case, the function would call itself infinitely, leading to a stack overflow error.
Top-Down Thinking. Recursion encourages a top-down approach to problem-solving. Instead of focusing on the step-by-step details, you can focus on defining the problem in terms of smaller instances of itself.
8. Dynamic Programming Optimizes Recursive Algorithms
Dynamic Programming shows you how to optimize recursive code and prevent it from spiraling out of control.
Overlapping Subproblems. Recursive algorithms can sometimes lead to redundant calculations, especially when dealing with overlapping subproblems. This can result in exponential time complexity.
Memoization. Dynamic programming addresses this issue through memoization, a technique that involves storing the results of expensive function calls and reusing them when the same inputs occur again. This avoids redundant calculations and significantly improves performance.
Bottom-Up Approach. Another dynamic programming technique is the bottom-up approach, which involves solving the smallest subproblems first and then using their solutions to build up to the larger problem. This eliminates the need for recursion altogether.
9. Node-Based Structures Offer Flexibility
Speeding Up All the Things with Binary Search Trees
Non-Contiguous Memory. Node-based data structures, such as linked lists and trees, store data in nodes that can be dispersed throughout the computer's memory. This contrasts with arrays, which require contiguous blocks of memory.
Linked Lists. Linked lists consist of nodes, each containing a data element and a link to the next node. This structure allows for efficient insertion and deletion at the beginning of the list.
Trees. Trees are hierarchical data structures where each node can have multiple child nodes. Binary search trees, in particular, offer efficient search, insertion, and deletion operations while maintaining order.
10. Graphs Model Relationships and Connections
Graphs are useful when you need to ensure that there is no duplicate data.
Vertices and Edges. Graphs are data structures that consist of vertices (nodes) and edges (connections between vertices). They are ideal for representing relationships and networks.
Directed vs. Undirected. Graphs can be directed, where edges have a specific direction, or undirected, where edges represent mutual relationships. Social networks, maps, and dependency diagrams are common examples of graphs.
Graph Search. Graph search algorithms, such as depth-first search (DFS) and breadth-first search (BFS), are used to traverse and explore the connections within a graph. These algorithms have various applications, including finding paths, detecting cycles, and identifying connected components.
11. Space Complexity Matters for Memory Efficiency
Big O of Space Complexity
Memory Consumption. Space complexity measures the amount of memory an algorithm consumes relative to the input size. It's an important consideration when memory is limited or when dealing with large datasets.
Auxiliary Space. Space complexity typically refers to the auxiliary space used by an algorithm, which is the additional memory beyond the input data itself. This includes the space used for variables, data structures, and function calls.
Trade-offs. There is often a trade-off between time complexity and space complexity. An algorithm that is optimized for speed may consume more memory, and vice versa. Choosing the right algorithm involves balancing these competing factors.
12. Optimization Techniques Enhance Code Performance
Techniques for Code Optimization
Prerequisite: Determine Big O. Before optimizing code, it's crucial to first determine its current Big O notation. This provides a baseline for measuring the effectiveness of any optimizations.
Best-Imaginable Big O. Identify the best-imaginable Big O for the problem at hand. This serves as a target for optimization efforts. It may not always be achievable, but it provides a direction for improvement.
Magical Lookups. Consider whether using a hash table to enable O(1) lookups could improve performance. This technique can often eliminate nested loops and significantly reduce time complexity.
Last updated:
Review Summary
A Common-Sense Guide to Data Structures and Algorithms receives mostly positive reviews for its clear explanations and beginner-friendly approach. Readers appreciate the intuitive examples, diagrams, and step-by-step breakdowns of complex concepts. Many find it helpful for interview preparation and as a refresher for those with prior knowledge. Some criticisms include inconsistent programming language use, lack of depth in certain areas, and occasional errors. Overall, it's highly recommended for beginners and self-taught programmers, though more advanced readers may find it too basic.
Similar Books
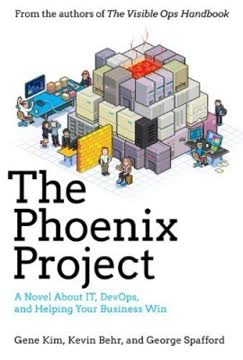
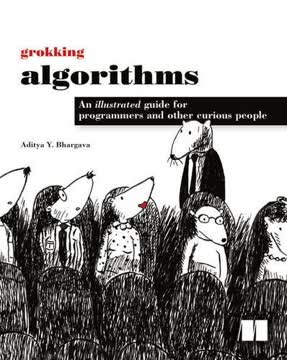
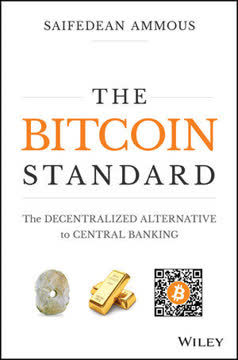
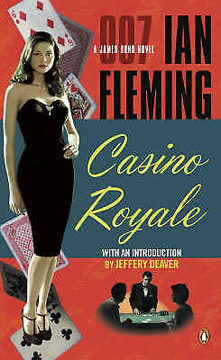
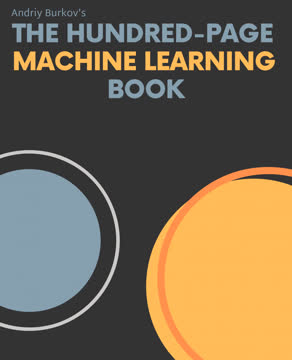
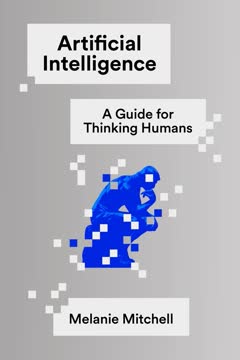
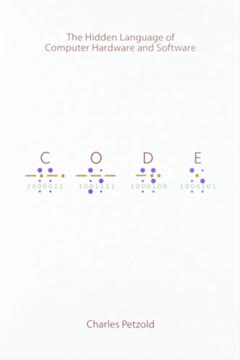
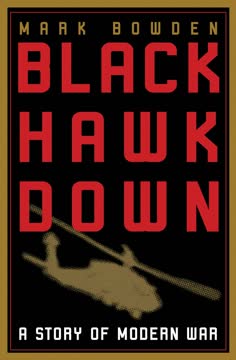
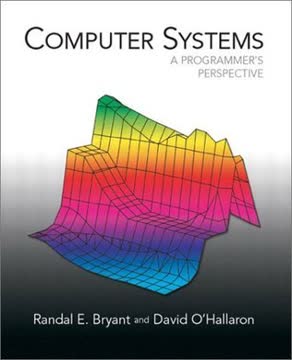
Download PDF
Download EPUB
.epub
digital book format is ideal for reading ebooks on phones, tablets, and e-readers.