Key Takeaways
1. Information is fundamentally bits plus context.
The only thing that distinguishes different data objects is the context in which we view them.
Bits need interpretation. At its core, all data within a computer system—from files on disk to programs in memory—is represented as bits (0s and 1s). The meaning of these bits is derived entirely from the context in which they are interpreted. The same sequence of bytes could represent an integer, a floating-point number, a character string, or even a machine instruction.
Context matters. This concept is crucial for programmers to understand. For example, a sequence of bytes representing a number might be treated as signed or unsigned, leading to vastly different interpretations. Similarly, the way a program handles data depends on its type, which the compiler uses to generate appropriate machine code.
Understanding representations. By understanding how different data types are represented at the bit level, programmers can write more reliable and efficient code. This knowledge helps avoid common pitfalls such as integer overflow, floating-point inaccuracies, and security vulnerabilities like buffer overflows.
2. Compilation systems translate human-readable programs into executable machine code.
On a Unix system, the translation from source file to object file is performed by a compiler driver.
From source to execution. The journey of a C program from its source code to its execution involves a series of transformations performed by the compilation system. This system typically consists of a preprocessor, compiler, assembler, and linker, each playing a vital role in converting the high-level code into low-level machine instructions.
Phases of compilation. The preprocessor handles directives like #include
, the compiler translates C code into assembly language, the assembler converts assembly code into relocatable object code, and the linker combines object files and libraries to produce an executable. Each phase adds complexity and detail, moving closer to the machine's understanding.
Executable object files. The final output of the compilation system is an executable object file, which contains the machine code instructions, data, and symbol information needed to run the program. This file is then loaded into memory by the operating system and executed by the processor.
3. Understanding compilation systems aids in optimizing performance and avoiding errors.
For simple programs such as
hello.c
, we can rely on the compilation system to produce correct and efficient machine code.
Compiler limitations. While modern compilers are sophisticated, they have limitations. Programmers need a basic understanding of machine-level code to make good coding decisions, such as choosing efficient data structures and algorithms.
Link-time errors. Some of the most perplexing programming errors are related to the linker, especially in large software systems. Understanding how linkers resolve references, handle static and dynamic libraries, and create position-independent code is crucial for avoiding these errors.
Security vulnerabilities. Buffer overflow vulnerabilities, a common source of security holes, arise from a lack of understanding of how data and control information are stored on the program stack. Programmers need to understand these concepts to write secure code.
4. Processors execute instructions stored in memory, managed by the operating system.
At its core is a word-size storage device (or register) called the program counter (PC).
The CPU's role. The central processing unit (CPU) is the engine that interprets and executes instructions stored in main memory. It operates by repeatedly fetching instructions, interpreting them, and performing simple operations on data.
Hardware organization. The CPU interacts with main memory, I/O devices, and other components through a system of buses. The register file, a small storage device within the CPU, holds frequently accessed data. The arithmetic/logic unit (ALU) performs arithmetic and logical operations.
Instruction execution. The CPU follows a simple instruction execution model, defined by its instruction set architecture (ISA). Instructions are executed in strict sequence, involving steps such as loading data, storing data, operating on data, and jumping to different instructions.
5. Cache memories are essential for bridging the processor-memory speed gap.
To deal with the processor-memory gap, system designers include smaller, faster storage devices called cache memories (or simply caches) that serve as temporary staging areas for information that the processor is likely to need in the near future.
The processor-memory gap. Due to physical laws, larger storage devices are slower than smaller storage devices. Faster devices are more expensive to build than their slower counterparts. This creates a significant gap between processor speed and memory access time.
Cache hierarchy. To bridge this gap, system designers use a hierarchy of storage devices called cache memories. Smaller, faster caches (L1, L2, L3) store frequently accessed data, allowing the processor to access them quickly.
Locality. Caching is made possible by locality, the tendency for programs to access data and code in localized regions. By exploiting temporal and spatial locality, caches can significantly improve program performance.
6. Storage devices are organized in a hierarchy based on speed, cost, and capacity.
As we move from the top of the hierarchy to the bottom, the devices become slower, larger, and less costly per byte.
The memory hierarchy. Computer systems organize storage devices into a hierarchy, with faster, smaller, and more expensive devices at the top and slower, larger, and less expensive devices at the bottom. This hierarchy includes registers, caches, main memory, solid-state disks, and rotating disks.
Caching at each level. Each level in the hierarchy serves as a cache for the next lower level. The register file caches data from the L1 cache, the L1 cache caches data from the L2 cache, and so on.
Exploiting the hierarchy. Programmers can improve performance by understanding and exploiting the memory hierarchy. This involves writing code that exhibits good locality and minimizing the number of accesses to slower storage devices.
7. The operating system manages hardware resources through abstractions like processes, virtual memory, and files.
We can think of the operating system as a layer of software interposed between the application program and the hardware.
Abstraction layer. The operating system (OS) acts as an intermediary between application programs and the hardware. It protects the hardware from misuse and provides applications with simple, uniform mechanisms for manipulating complex hardware devices.
Key abstractions. The OS achieves its goals through three fundamental abstractions: processes, virtual memory, and files. Processes provide the illusion of exclusive use of the processor, main memory, and I/O devices. Virtual memory provides the illusion of exclusive use of main memory. Files provide a uniform view of all I/O devices.
Kernel's role. The OS kernel manages these abstractions, handling context switches between processes, translating virtual addresses to physical addresses, and providing a uniform interface for accessing I/O devices.
8. Systems communicate with other systems using networks, viewed as I/O devices.
When the system copies a sequence of bytes from main memory to the network adapter, the data flow across the network to another machine, instead of, say, to a local disk drive.
Networks as I/O devices. From the perspective of an individual system, a network can be viewed as just another I/O device. Data can be copied from main memory to the network adapter for transmission to other machines.
Client-server model. Network applications are based on the client-server model, where clients request services from servers. This model relies on the ability to copy information over a network.
Telnet example. The telnet
application demonstrates how a network can be used to run programs remotely. The telnet client sends commands to the telnet server, which executes the commands and sends the output back to the client.
9. Amdahl's Law dictates the limits of performance improvement from optimizing a single component.
The main idea is that when we speed up one part of a system, the effect on the overall system performance depends on both how significant this part was and how much it sped up.
Diminishing returns. Amdahl's Law states that the overall speedup of a system is limited by the fraction of time that the improved component is used. Even if we make a significant improvement to a major part of the system, the net speedup will be less than the speedup for the one part.
Focus on the big picture. To significantly speed up the entire system, we must improve the speed of a very large fraction of the overall system. This requires identifying and optimizing the most time-consuming components.
General principle. Amdahl's Law is a general principle for improving any process, not just computer systems. It can guide efforts to reduce manufacturing costs or improve academic performance.
10. Concurrency and parallelism enhance system performance at multiple levels.
We use the term concurrency to refer to the general concept of a system with multiple, simultaneous activities, and the term parallelism to refer to the use of concurrency to make a system run faster.
Concurrency vs. Parallelism. Concurrency refers to the general concept of multiple, simultaneous activities in a system. Parallelism refers to the use of concurrency to make a system run faster.
Levels of Parallelism:
- Thread-Level Concurrency: Achieved through multiple processes or threads, enabling multiple users or tasks to run simultaneously.
- Instruction-Level Parallelism: Modern processors execute multiple instructions at once, improving performance.
- SIMD Parallelism: Single instructions operate on multiple data points simultaneously, speeding up image, sound, and video processing.
Multiprocessor systems. Multiprocessor systems, including multi-core processors and hyperthreading, allow for true parallel execution, improving system performance by reducing the need to simulate concurrency.
11. Abstractions are crucial for managing complexity in computer systems.
The use of abstractions is one of the most important concepts in computer science.
Simplifying complexity. Abstractions provide simplified views of complex systems, allowing programmers to use code without delving into its inner workings. This is a key aspect of good programming practice.
Examples of abstractions:
- Instruction Set Architecture (ISA): Provides an abstraction of the processor hardware.
- Operating System: Provides abstractions for I/O devices (files), program memory (virtual memory), and running programs (processes).
- Virtual Machines: Provides an abstraction of the entire computer, including the OS, processor, and programs.
Benefits of abstractions. Abstractions enable programmers to write code that is portable, reliable, and efficient, without needing to understand the underlying hardware and software in detail.
12. Number representation impacts program reliability and security.
Having a solid understanding of computer arithmetic is critical to writing reliable programs.
Finite approximations. Computer representations of numbers are finite approximations of integers and real numbers. This can lead to unexpected behavior, such as arithmetic overflow and floating-point inaccuracies.
Integer representations. Unsigned encodings represent nonnegative numbers, while two's-complement encodings represent signed integers. Understanding the properties of these representations is crucial for writing reliable code.
Floating-point representations. IEEE floating-point format is a base-2 version of scientific notation for representing real numbers. Understanding how floating-point numbers are represented and manipulated is essential for avoiding errors in numerical computations.
Last updated:
Review Summary
Computer Systems: A Programmer's Perspective is highly regarded for its comprehensive and clear explanations of computer systems concepts. Readers praise its practical approach, use of C examples, and coverage of topics like memory hierarchy and virtual memory. Many consider it essential reading for computer science students and professionals. The book is lauded for its ability to bridge theoretical concepts with real-world applications. While some find it challenging, most reviewers appreciate its depth and clarity. A few criticisms mention outdated information and potential issues with undefined behavior in code examples.
Similar Books
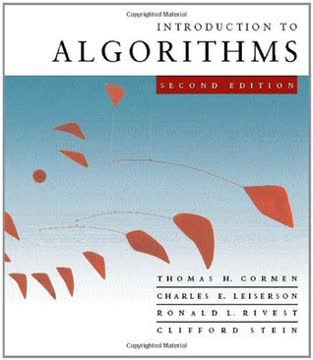
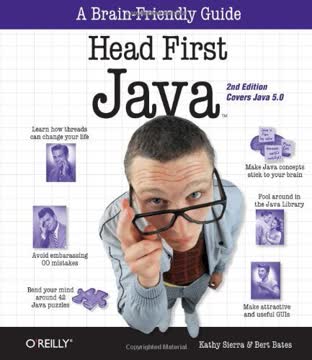
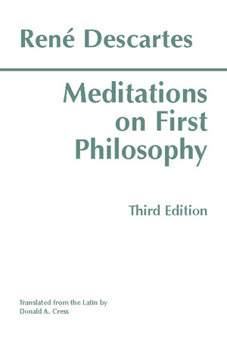
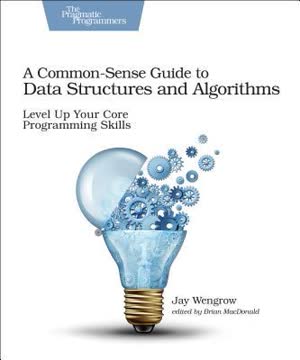
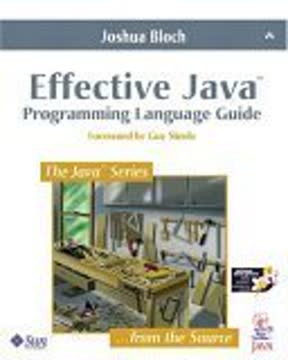
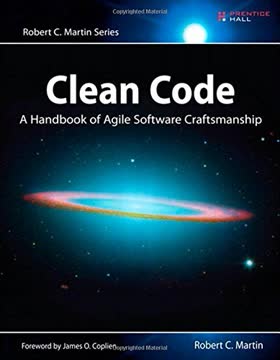
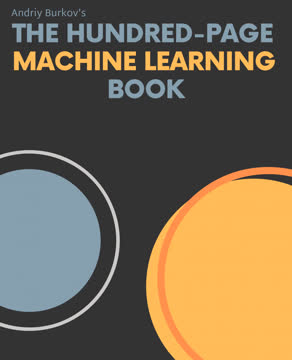
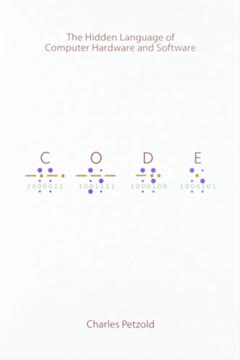
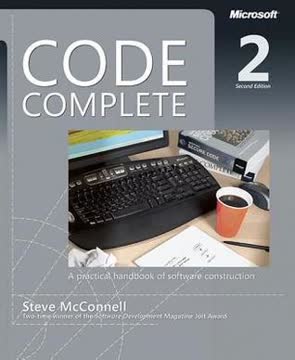
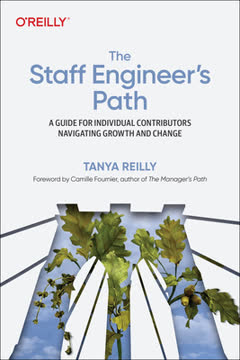
Download PDF
Download EPUB
.epub
digital book format is ideal for reading ebooks on phones, tablets, and e-readers.