Key Takeaways
1. Computer Science: Essential for Efficient Problem Solving
Computer science is everywhere, but it’s still taught as boring theory.
Practical Application. Computer science isn't just abstract theory; it's a crucial foundation for effective programming and problem-solving in the real world. Many coders lack formal computer science training, leading to inefficient solutions. This book aims to bridge that gap by presenting computer science concepts in a distilled, accessible format.
Computational Thinking. The core of computer science lies in computational thinking, which involves breaking down problems into computable systems. This approach is not limited to coding but can be applied to everyday situations, such as streamlining packing or speeding up cooking through parallelism.
Abundant Power, Scarce Skills. Computing power is readily available, but the ability to use it efficiently is scarce. By mastering computer science principles, individuals can unlock the full potential of machines and create innovative, effective solutions to complex problems.
2. Logic: The Bedrock of Computational Thinking
Coders work with logic so much it messes their minds.
Formal Logic. Logic is fundamental to computer science, enabling coders to deliberately solve problems. Formal logic provides a framework for reasoning about the validity of statements and relationships, using operators like AND, OR, NOT, and conditionals.
Boolean Algebra. Boolean algebra simplifies logical expressions, similar to how elementary algebra simplifies numerical expressions. De Morgan's Laws, for example, allow the transformation of ANDs into ORs and vice versa, aiding in the simplification of complex logical models.
Truth Tables. Truth tables offer a systematic way to analyze logical models by examining all possible configurations of variables. By constructing truth tables, one can determine the conditions under which a system will function correctly, as demonstrated in the "Fragile System" example.
3. Counting: Mastering the Art of Enumeration
It’s important to count things correctly—you’ll have to do it many times when working with computational problems.
Combinatorial Analysis. Counting techniques, including multiplications, permutations, combinations, and sums, are essential for solving computational problems. These tools allow us to determine the number of possible outcomes or configurations, which is crucial for estimating the feasibility of algorithms.
Factorials and Permutations. The factorial function (n!) calculates the number of ways to order n items. Permutations, which consider the order of selection, are used to count the number of ways to arrange m items out of n possible items.
Combinations and Sums. Combinations, denoted as "n choose m," calculate the number of ways to select m items out of n, regardless of order. Sums, expressed using the sigma (Σ) notation, are used to calculate the total number of possibilities in sequential events, as demonstrated in the "Flying Cheap" example.
4. Probability: Navigating the Realm of Chance
The principles of randomness will help you understand gambling, forecast the weather, or design a backup system with low risk of failure.
Calculating Odds. Probability principles help quantify the likelihood of events, enabling informed decision-making in various scenarios. The probability of an event is calculated as the number of ways the event can happen divided by the total number of possible outcomes.
Independent and Mutually Exclusive Events. Independent events have outcomes that do not influence each other, and their probabilities are multiplied to find the probability of both occurring. Mutually exclusive events cannot happen simultaneously, and their probabilities are summed to find the probability of either occurring.
Complementary Events and Gambler's Fallacy. Complementary events cover all possible outcomes, and the sum of their probabilities is 100%. It's crucial to avoid the gambler's fallacy, which incorrectly assumes that past events influence the outcome of independent events.
5. Complexity Analysis: Gauging Algorithmic Efficiency
In almost every computation, a variety of arrangements for the processes is possible.
Time Complexity. Time complexity, denoted as T(n), quantifies the number of operations an algorithm performs for an input of size n. Analyzing time complexity helps predict how execution time will grow as the input size increases.
Big-O Notation. Big-O notation expresses the dominant term of an algorithm's cost function in the worst case, providing a standardized way to represent time complexity. Algorithms with lower Big-O complexities, such as O(n log n), generally perform better than those with higher complexities, such as O(n^2), for large inputs.
Exponential Algorithms. Exponential time algorithms, with complexities like O(2^n), are considered "not runnable" due to their explosive growth. These algorithms are impractical for large inputs and should be avoided unless dealing with very small problem sizes.
6. Algorithm Design Strategies: A Toolkit for Problem Solving
If you find a good move, look for a better one.
Iteration and Recursion. Iteration uses loops to repeat a process until a condition is met, while recursion involves a function delegating work to clones of itself. Recursive algorithms are often simpler but can introduce computational overhead.
Brute Force and Backtracking. Brute force solves problems by inspecting all possible solution candidates, while backtracking optimizes the search by testing bad options and then stepping back. Backtracking is effective when choices restrain subsequent choices.
Heuristics and Divide and Conquer. Heuristics provide a reasonable way out by sacrificing optimality for speed, while divide and conquer breaks down problems into smaller, similar subproblems. Dynamic programming avoids redundant computations by identifying and memoizing repeated subproblems.
7. Data Structures: Organizing Information for Optimal Access
Good programmers worry about data structures and their relationships.
Abstract Data Types (ADTs). ADTs specify a group of operations for a given data type, hiding implementation details and promoting code reusability. Common ADTs include Stacks, Queues, Lists, Maps, and Sets.
Arrays and Linked Lists. Arrays store items in contiguous memory locations, providing instant access but limited flexibility. Linked lists use a chain of cells with pointers, allowing for easy insertion and deletion but slower access.
Trees and Hash Tables. Trees organize data hierarchically, with Binary Search Trees enabling efficient searching. Hash tables use hash functions to map data to memory locations, providing O(1) access time but requiring careful management of collisions.
8. Algorithms: Leveraging Pre-Existing Solutions
[Coding is] attractive not only because it can be economically and scientifically rewarding, but also because it can be an aesthetic experience much like composing poetry or music.
Sorting Algorithms. Sorting algorithms arrange data in a specific order, with simpler algorithms like Selection Sort and Insertion Sort being O(n^2) and more efficient algorithms like Merge Sort and Quicksort being O(n log n). Insertion Sort is very efficient at sorting nearly sorted datasets.
Searching Algorithms. Searching algorithms locate specific information in memory, with Sequential Search being O(n) and Binary Search being O(log n) for sorted data. Hash tables provide O(1) search time.
Graph Algorithms. Graph algorithms operate on data represented as nodes and edges, with Depth-First Search (DFS) and Breadth-First Search (BFS) exploring graphs in different ways. Dijkstra's Algorithm finds the shortest path between nodes.
9. Databases: Managing Vast Data Collections
While I am best known for my work on databases, my fundamental skills are those of an architect: analyzing requirements and constructing simple, but elegant, solutions.
Relational Databases. Relational databases organize data into tables with rows and columns, using primary and foreign keys to establish relationships. SQL is the standard query language for relational databases.
Non-Relational Databases (NoSQL). Non-relational databases offer more flexibility by ditching tabular relations and fixed schemas. Document stores, key-value stores, and graph databases are examples of NoSQL databases.
Distributed Databases. Distributed databases coordinate multiple computers to handle large datasets, high query loads, or mission-critical applications. Techniques include single-master replication, multi-master replication, and sharding.
10. Computer Architecture: Unveiling the Inner Workings
Any sufficiently advanced technology is indistinguishable from magic.
Processor and Memory. A computer consists of a processor (CPU) and memory (RAM). The memory stores instructions and data, while the processor fetches instructions and performs calculations.
CPU Operations. The CPU performs simple mathematical operations and moves data between the RAM and internal registers. The instruction set defines the operations a CPU can execute.
Memory Hierarchy. The memory hierarchy consists of CPU registers, L1/L2/L3 caches, RAM, and secondary storage (hard disk). Caches exploit temporal and spatial locality to reduce RAM access time.
11. Programming Languages: Bridging the Gap Between Human and Machine
When someone says: “I want a programming language in which I need only say what I wish done”, give him a lollipop.
Values, Expressions, and Statements. Programming languages manipulate information using values, expressions, and statements. Values represent information, expressions produce values, and statements instruct the computer.
Variables and Typing. Variables associate names with values, and typing assigns a data type to variables. Static typing requires explicit type declarations, while dynamic typing checks types at runtime.
Programming Paradigms. Programming paradigms offer different approaches to problem-solving, including imperative, object-oriented, and functional programming. Each paradigm has its strengths and weaknesses, influencing code structure and organization.
Last updated:
Review Summary
Computer Science Distilled receives mixed reviews, with an overall rating of 4.06 out of 5. Many readers praise it as an excellent introduction to computer science, highlighting its clear explanations and accessible approach. They appreciate its concise coverage of core concepts and its value for beginners and self-taught programmers. However, some critics argue that the book is too basic, lacks depth, and fails to adequately explain complex topics. Despite the criticism, many readers find it helpful for understanding CS fundamentals and recommend it as a starting point for those new to the field.
Similar Books
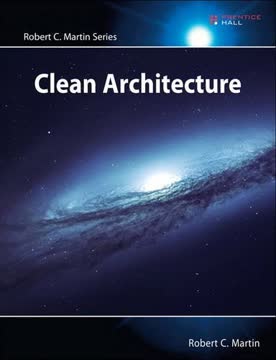
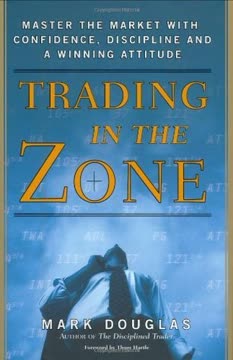
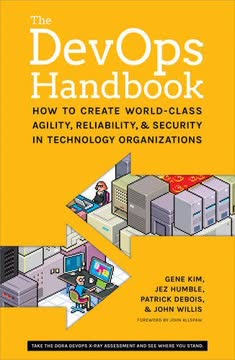
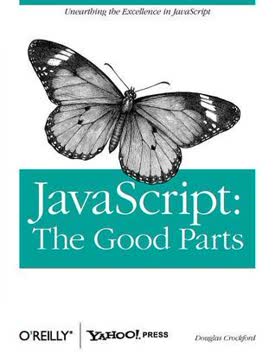
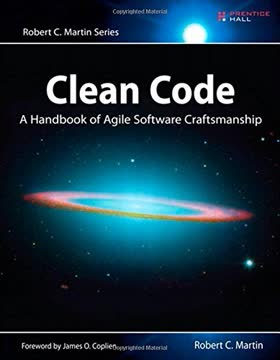
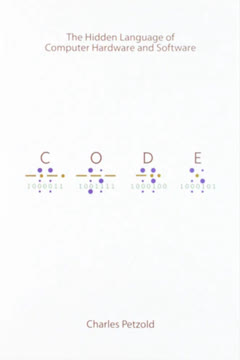
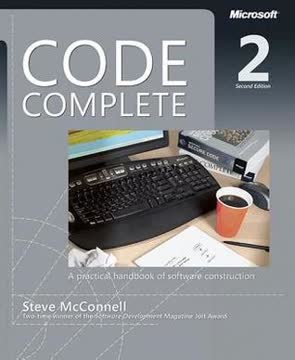
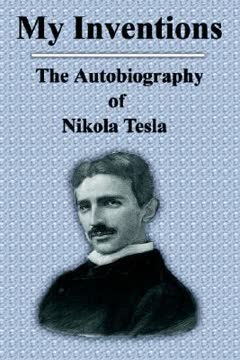
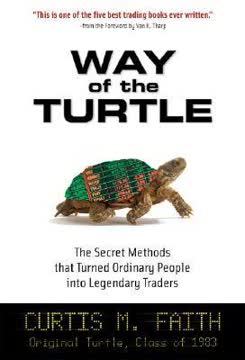
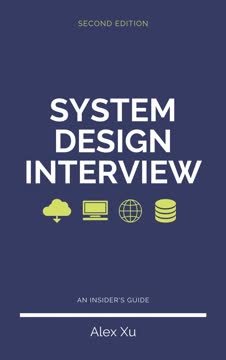