Key Takeaways
1. Algorithms: The Unsung Heroes of Computing
But now that there are computers, there are even more algorithms, and algorithms lie at the heart of computing.
Algorithms are fundamental. Before computers existed, algorithms were the foundation of problem-solving. Now, with the proliferation of computers, algorithms are even more critical, serving as the core logic behind every computation. They are the well-defined procedures that transform inputs into desired outputs, acting as the essential tools for solving computational problems.
Ubiquitous applications. Algorithms are not just theoretical constructs; they are deeply embedded in our daily lives. From the Human Genome Project's data analysis to the Internet's routing protocols and search engines, algorithms are the driving force behind countless technologies. They are also essential for securing electronic commerce through cryptography and optimizing resource allocation in manufacturing and logistics.
Beyond sorting. While sorting is a common example used to illustrate algorithmic concepts, the range of problems algorithms can solve is vast. They can find the shortest path on a map, identify similarities between DNA strands, schedule tasks, and even determine the vertices of a convex hull. The possibilities are endless.
2. Efficiency Matters: Algorithms as a Critical Technology
Total system performance depends on choosing efficient algorithms as much as on choosing fast hardware.
Hardware isn't everything. While fast processors and ample memory are important, the efficiency of the algorithm used can have a far greater impact on performance. A poorly designed algorithm can negate the benefits of even the most powerful hardware.
Dramatic differences. The difference in efficiency between algorithms can be dramatic. For example, insertion sort, with its ‚.n2/ running time, pales in comparison to merge sort's ‚.n lg n/ time for large datasets. This difference becomes increasingly significant as the problem size grows.
Algorithms are a technology. Just like hardware, algorithms should be considered a technology. Investing in the development and selection of efficient algorithms is just as crucial as investing in faster processors or more memory. A skilled programmer understands the importance of algorithmic knowledge and technique.
3. Pseudocode: A Universal Language for Algorithms
The only requirement is that the specification must provide a precise description of the computational procedure to be followed.
Clarity over code. Pseudocode serves as a bridge between human understanding and machine execution. It is a way to express algorithms in a clear, concise, and unambiguous manner, without getting bogged down in the specifics of a particular programming language.
Expressive freedom. Unlike real code, pseudocode allows for the use of English phrases, mathematical notation, and other expressive methods to convey the essence of an algorithm. The goal is to communicate the algorithm's logic in the most accessible way possible.
Focus on logic. Pseudocode is not typically concerned with software engineering issues such as data abstraction, modularity, or error handling. It focuses solely on the computational procedure itself, allowing the reader to understand the algorithm's core logic without unnecessary distractions.
4. Insertion Sort: Simplicity and Incremental Design
Insertion sort works the way many people sort a hand of playing cards.
Incremental approach. Insertion sort is a simple sorting algorithm that builds a sorted array one element at a time. It iterates through the input, inserting each element into its correct position within the already sorted portion of the array.
Loop invariants. Loop invariants are crucial for understanding and proving the correctness of iterative algorithms. They define a property that holds true at the start of each iteration of a loop, allowing us to reason about the algorithm's behavior.
Correctness. The loop invariant for insertion sort states that at the start of each iteration, the subarray to the left of the current element is always sorted. By proving that this invariant holds true throughout the algorithm, we can demonstrate that insertion sort correctly sorts the entire array upon termination.
5. Merge Sort: Divide, Conquer, and Combine
The divide-and-conquer paradigm involves three steps at each level of the recursion.
Divide and conquer. Merge sort exemplifies the divide-and-conquer paradigm, breaking down the sorting problem into smaller subproblems, recursively sorting them, and then merging the sorted subproblems to produce the final sorted array.
Merging is key. The merging process, which combines two sorted subarrays into a single sorted array, is the heart of merge sort. This process takes linear time and is implemented by comparing elements from the two subarrays and placing them into the output array in sorted order.
Recurrences. The running time of merge sort can be described by a recurrence equation, which expresses the overall running time in terms of the running time on smaller inputs. Solving this recurrence reveals that merge sort has a worst-case running time of ‚.n lg n/.
6. Asymptotic Notation: Focusing on Growth
It is the rate of growth, or order of growth, of the running time that really interests us.
Ignoring details. Asymptotic notation provides a way to simplify the analysis of algorithms by focusing on the rate of growth of their running times, ignoring constant factors and lower-order terms. This allows us to compare the efficiency of different algorithms for large input sizes.
Theta, Big-O, and Omega. The most common asymptotic notations are:
- ‚-notation: Provides an asymptotically tight bound.
- O-notation: Provides an asymptotic upper bound.
- -notation: Provides an asymptotic lower bound.
Order of growth. By using asymptotic notation, we can compare algorithms based on their order of growth. An algorithm with a lower order of growth is generally considered more efficient for large inputs, even if it has a larger constant factor for small inputs.
7. Divide-and-Conquer: A Powerful Design Paradigm
Many useful algorithms are recursive in structure: to solve a given problem, they call themselves recursively one or more times to deal with closely related subproblems.
Recursive problem solving. Divide-and-conquer is a powerful technique for designing algorithms. It involves breaking a problem into smaller subproblems, solving the subproblems recursively, and then combining the solutions to the subproblems to solve the original problem.
Three steps:
- Divide: Break the problem into smaller subproblems.
- Conquer: Solve the subproblems recursively.
- Combine: Combine the solutions to the subproblems.
Recurrences. Divide-and-conquer algorithms often lead to recurrences that describe their running times. These recurrences can be solved using techniques such as the substitution method, recursion trees, or the master method.
8. Randomized Algorithms: Embracing Uncertainty
An algorithm whose behavior is determined not only by its input but by the values produced by a random-number generator is a randomized algorithm.
Randomness as a tool. Randomized algorithms use random choices during their execution to achieve better performance or to avoid worst-case scenarios. They can be particularly useful when the input distribution is unknown or when deterministic algorithms are too complex or inefficient.
Probabilistic analysis. Probabilistic analysis is used to determine the expected running time of an algorithm, where the expectation is taken over the distribution of random choices made by the algorithm. This is different from average-case analysis, where the expectation is taken over the distribution of inputs.
NP-Completeness. Randomized algorithms can be used to enforce a probability distribution on the inputs—thereby ensuring that no particular input always causes poor performance—or even to bound the error rate of algorithms that are allowed to produce incorrect results on a limited basis.
9. Data Structures: Organizing Information
A data structure is a way to store and organize data in order to facilitate access and modifications.
Efficient access. Data structures are fundamental to algorithm design, providing ways to store and organize data to facilitate efficient access and modification. The choice of data structure can significantly impact the performance of an algorithm.
Trade-offs. No single data structure is ideal for all purposes. Different data structures offer different trade-offs between storage space, access time, and the efficiency of various operations.
Examples. Common data structures include:
- Stacks and queues: Simple linear structures with specific access patterns.
- Linked lists: Flexible structures that allow for efficient insertion and deletion.
- Hash tables: Structures that provide fast average-case access to elements.
- Binary search trees: Tree-based structures that allow for efficient searching, insertion, and deletion.
10. NP-Completeness: Understanding Intractability
If you are called upon to produce an efficient algorithm for an NP-complete problem, you are likely to spend a lot of time in a fruitless search.
Hard problems. NP-complete problems are a class of problems for which no efficient (polynomial-time) solution is known. While no one has proven that efficient algorithms cannot exist, the lack of such solutions despite extensive research suggests that these problems are inherently difficult.
Reducibility. The remarkable property of NP-complete problems is that if an efficient algorithm exists for any one of them, then efficient algorithms exist for all of them. This relationship makes the lack of efficient solutions all the more tantalizing.
Approximation. If you encounter an NP-complete problem, it is often more productive to focus on developing an efficient algorithm that gives a good, but not necessarily optimal, solution. These are known as approximation algorithms.
Last updated:
FAQ
What's Introduction to Algorithms about?
- Comprehensive Guide: Introduction to Algorithms by Thomas H. Cormen is a detailed textbook that covers a wide range of algorithms and data structures, providing both theoretical foundations and practical applications.
- Focus on Design and Analysis: The book emphasizes the design and analysis of algorithms, including their efficiency and complexity, making it suitable for both undergraduate and graduate courses.
- Structured Learning Approach: It is organized into chapters that progressively build on each other, allowing readers to develop a deep understanding of algorithmic principles and their applications.
Why should I read Introduction to Algorithms?
- Foundational Knowledge: This book provides essential knowledge for anyone interested in computer science, programming, or software engineering.
- Widely Used Textbook: It is a standard reference in computer science education and is used in many university courses, making it a valuable resource for students and professionals alike.
- Real-World Applications: The algorithms discussed are applicable to real-world problems, making the knowledge gained from this book directly useful in software development and engineering.
What are the key takeaways of Introduction to Algorithms?
- Algorithm Efficiency: Understanding how to analyze the efficiency of algorithms using Big O notation is a crucial takeaway, as it helps in evaluating performance.
- Diverse Algorithm Techniques: The book covers various algorithmic strategies, including greedy algorithms, dynamic programming, and graph algorithms, each illustrated with examples and applications.
- Data Structures Importance: It emphasizes the relationship between algorithms and data structures, showing how the choice of data structure can significantly impact algorithm performance.
What are the best quotes from Introduction to Algorithms and what do they mean?
- "Algorithms lie at the heart of computing.": This quote emphasizes the fundamental role algorithms play in computer science and technology, underscoring their importance in problem-solving.
- "Efficiency is a design criterion.": This highlights the necessity of considering efficiency in algorithm design, as it directly impacts performance and resource utilization.
- "Understanding algorithms is essential for any programmer.": This quote stresses that a solid grasp of algorithms is crucial for effective programming and software development, as it enhances problem-solving skills.
How does Introduction to Algorithms define dynamic programming?
- Optimization Technique: Dynamic programming is defined as a method for solving complex problems by breaking them down into simpler subproblems, solving each subproblem just once, and storing their solutions.
- Overlapping Subproblems: The technique is effective when the problem has overlapping subproblems, meaning the same subproblems are solved multiple times, avoiding redundant calculations.
- Examples Provided: The book includes various examples, such as the matrix-chain multiplication problem, to demonstrate how dynamic programming can be applied to achieve efficient solutions.
What is the divide-and-conquer strategy in Introduction to Algorithms?
- Problem-Solving Method: Divide-and-conquer is a strategy where a problem is divided into smaller subproblems, solved independently, and then combined to form a solution to the original problem.
- Efficiency: This approach often leads to more efficient algorithms, as seen in sorting and searching algorithms, which can significantly reduce time complexity.
- Examples in Algorithms: The book provides examples of divide-and-conquer algorithms, such as mergesort and the closest pair of points, demonstrating its effectiveness in various scenarios.
What is the significance of the master theorem in Introduction to Algorithms?
- Solving Recurrences: The master theorem provides a method for solving recurrences of the form T(n) = aT(n/b) + f(n), which frequently arise in divide-and-conquer algorithms.
- Three Cases: It outlines three cases based on the relationship between f(n) and n^(log_b(a)), allowing for quick determination of asymptotic bounds.
- Widely Applicable: This theorem is a powerful tool for analyzing the running time of many algorithms, making it a crucial concept in the book.
How does Introduction to Algorithms approach graph algorithms?
- Graph Representation: The book discusses various ways to represent graphs, including adjacency lists and adjacency matrices, and explains the trade-offs between these representations.
- Key Algorithms: It covers essential graph algorithms, such as Dijkstra's algorithm for shortest paths, Kruskal's and Prim's algorithms for minimum spanning trees, and depth-first and breadth-first search.
- Complexity Analysis: The text provides a thorough analysis of the time and space complexity of graph algorithms, enabling readers to evaluate their efficiency.
What is the Bellman-Ford algorithm in Introduction to Algorithms?
- Single-Source Shortest Paths: The Bellman-Ford algorithm is designed to find the shortest paths from a single source vertex to all other vertices in a weighted graph.
- Handles Negative Weights: Unlike Dijkstra’s algorithm, it can handle graphs with negative-weight edges, making it versatile for various applications.
- Iterative Relaxation: The algorithm works by iteratively relaxing edges, ensuring that the shortest path estimates converge to the correct values.
What is the significance of the maximum-flow min-cut theorem in Introduction to Algorithms?
- Flow and Cuts Relationship: The max-flow min-cut theorem establishes a relationship between the maximum flow in a network and the minimum cut that separates the source from the sink.
- Equivalence: It states that the value of the maximum flow is equal to the capacity of the minimum cut, providing a powerful tool for analyzing flow networks.
- Applications: This theorem has numerous applications in network design, optimization, and resource allocation problems.
How does Introduction to Algorithms explain the concept of NP-completeness?
- Understanding Computational Limits: The NP-completeness section helps readers understand the limits of what can be efficiently computed, introducing problems that are easy to verify but hard to solve.
- Reduction Techniques: The text explains how to prove NP-completeness through reductions, providing a toolkit for identifying hard problems.
- Real-World Implications: Understanding NP-completeness has practical implications for algorithm development, informing decisions about which problems can be tackled with efficient algorithms.
What is the role of data structures in Introduction to Algorithms?
- Foundation for Algorithms: Data structures are presented as the backbone of algorithm design, influencing the efficiency and performance of algorithms.
- Variety of Structures: The book discusses various data structures, including arrays, linked lists, stacks, queues, trees, and hash tables, explaining their characteristics and use cases.
- Implementation and Analysis: Each data structure is accompanied by implementation details and performance analysis, helping readers understand how to effectively use them in conjunction with algorithms.
Review Summary
Introduction to Algorithms receives mixed reviews, with an overall high rating. Many praise it as comprehensive and essential for computer science, noting its thorough explanations and mathematical rigor. Critics argue it's too complex for beginners, focusing heavily on mathematical proofs rather than practical implementation. Some find the pseudocode difficult to understand. Supporters appreciate the detailed content and exercises, while detractors suggest alternative texts for learning algorithms. Despite criticisms, it's widely regarded as a fundamental resource for computer scientists and programmers seeking to improve their understanding of algorithms and data structures.
Similar Books
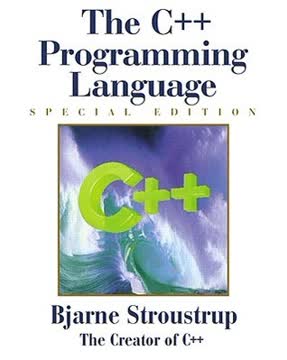
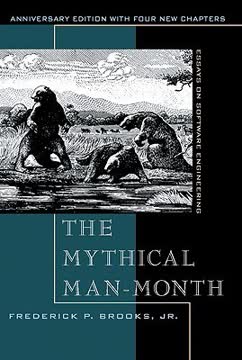
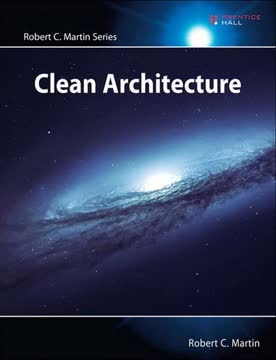
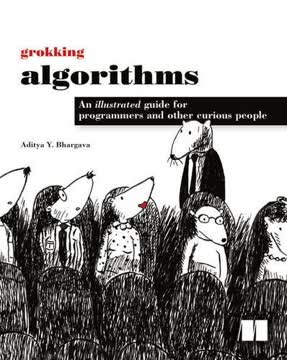
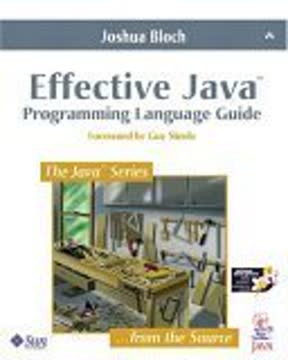
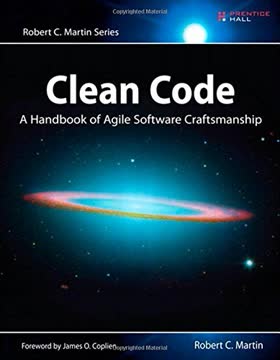
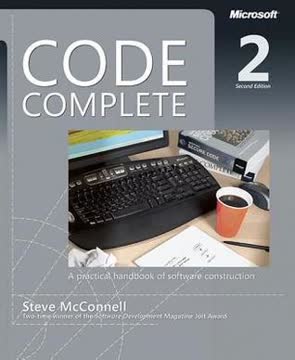
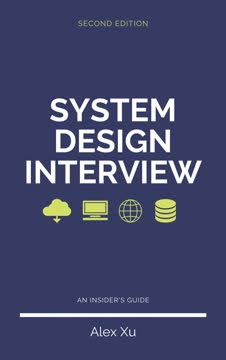
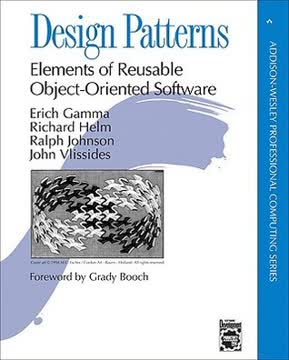
Download PDF
Download EPUB
.epub
digital book format is ideal for reading ebooks on phones, tablets, and e-readers.