Key Takeaways
1. Embrace Kotlin's functional programming features for cleaner code
The more of our code that is calculations, the better off we are.
Functional programming benefits: Kotlin encourages a more functional style of programming compared to Java. This approach leads to code that is easier to reason about, test, and maintain. By favoring immutability and pure functions, developers can reduce the complexity of their codebase and minimize unexpected side effects.
Key functional features:
- Lambda expressions
- Higher-order functions
- Immutable data structures
- Extension functions
- Sequence operations
By leveraging these features, developers can write more concise and expressive code that is less prone to errors and easier to understand.
2. Leverage Kotlin's type system to improve null safety and error handling
Kotlin's support for nullability makes sure that we take account of absence when we have to and are not overwhelmed by it when we don't.
Null safety advantages: Kotlin's type system distinguishes between nullable and non-nullable types, forcing developers to handle potential null cases explicitly. This approach significantly reduces the risk of null pointer exceptions, a common source of runtime errors in Java.
Improved error handling:
- Use of the Elvis operator (?:) for providing default values
- Safe call operator (?.) for safely accessing properties of nullable objects
- Smart casts for automatic type inference after null checks
By embracing Kotlin's null safety features, developers can write more robust code with fewer runtime errors and improved readability.
3. Refactor Java code to idiomatic Kotlin gradually and safely
Refactoring is often a process of exploration. We have a goal in mind, but don't always know how it will turn out.
Gradual refactoring approach: When migrating from Java to Kotlin, it's essential to take a step-by-step approach. This allows for maintaining a functional codebase throughout the transition while gradually introducing Kotlin idioms and best practices.
Refactoring strategies:
- Convert Java files to Kotlin one at a time
- Use IntelliJ's automatic Java-to-Kotlin converter as a starting point
- Gradually introduce Kotlin-specific features like data classes and extension functions
- Refactor towards more functional programming patterns
- Continuously run tests to ensure functionality is preserved
By following this approach, teams can safely transition their codebase to Kotlin while minimizing the risk of introducing bugs or breaking existing functionality.
4. Use extension functions to enhance existing types without inheritance
We find that Kotlin's lightweight syntax for closures offered a way to define the structure of their application and distinguish between object graphs instantiated for the whole application, for each HTTP request, or for each database transaction.
Extension function benefits: Kotlin's extension functions allow developers to add new functionality to existing classes without modifying their source code or using inheritance. This feature promotes a more modular and flexible codebase.
Use cases for extension functions:
- Adding utility methods to standard library classes
- Grouping related functionality for domain-specific operations
- Improving readability by allowing method chaining
- Creating domain-specific languages (DSLs)
By leveraging extension functions, developers can write more expressive and maintainable code while avoiding the pitfalls of excessive inheritance or utility classes.
5. Replace mutable state with immutable data and transformations
We like calculations because they are so much easier to work with, but ultimately our software needs to have an effect on the world, which is an action.
Immutability advantages: Favoring immutable data structures and transformations over mutable state leads to more predictable and easier-to-reason-about code. This approach aligns well with functional programming principles and can help reduce bugs related to unexpected state changes.
Strategies for immutability:
- Use data classes for representing immutable data
- Prefer val over var for variable declarations
- Use copy() for creating modified versions of immutable objects
- Leverage collection transformation functions like map, filter, and reduce
By adopting these practices, developers can create more robust and thread-safe code that is less prone to side effects and easier to test.
6. Simplify error handling with sealed classes and result types
We want our code to be simple and flexible. To this end, libraries need to hide implementation details from client code, and we want to be able to substitute one implementation of some functionality with another.
Improved error handling: Kotlin's sealed classes and result types offer a more expressive and type-safe way to handle errors compared to traditional exception handling. This approach allows for better compile-time checks and more explicit error handling in the code.
Benefits of using result types:
- Forced handling of potential error cases
- Improved readability and maintainability
- Better separation of happy path and error handling logic
- Easier composition of operations that may fail
By adopting this approach, developers can create more robust and predictable error handling mechanisms in their applications.
7. Move I/O operations to the edges of your system for better testability
By moving I/O to the outside of our systems, we can reduce the ways that both actions and errors complicate our code.
Improved testability: Pushing I/O operations to the boundaries of your application makes the core business logic easier to test and reason about. This separation of concerns allows for better unit testing and simplified error handling.
Strategies for managing I/O:
- Use dependency injection to provide I/O dependencies
- Create abstractions for I/O operations
- Use interfaces to decouple I/O from business logic
- Leverage functional programming concepts to separate pure functions from I/O effects
By following these practices, developers can create more modular and testable code that is less tightly coupled to external dependencies.
8. Prefer composition over inheritance with Kotlin's delegation
In Kotlin, we usually implement functions not with objects but with, well, functions.
Composition benefits: Kotlin's delegation feature provides a powerful alternative to inheritance, allowing for more flexible and maintainable code structures. This approach aligns well with the principle of favoring composition over inheritance.
Advantages of delegation:
- Improved code reusability
- Reduced coupling between classes
- More flexible object composition
- Easier to implement the decorator pattern
By leveraging delegation, developers can create more modular and easier-to-maintain code structures that are less prone to the pitfalls of deep inheritance hierarchies.
9. Utilize Kotlin's concise syntax for more expressive and readable code
We prefer expressions as opposed to statements. Expressions are declarative: we declare what we want the function to calculate and let the Kotlin compiler and runtime decide how to compute that calculation.
Concise syntax benefits: Kotlin's syntax offers numerous shortcuts and language features that allow developers to express complex operations more concisely. This leads to more readable and maintainable code.
Key syntax features:
- String templates
- Data classes
- Default and named arguments
- When expressions
- Smart casts
- Single-expression functions
By taking advantage of these features, developers can write more expressive code that is easier to understand and maintain.
10. Apply functional programming concepts to simplify complex operations
Functional programming does not eliminate mutable state but instead makes it the responsibility of the runtime.
Functional simplification: Adopting functional programming concepts in Kotlin can lead to simpler and more predictable code, especially when dealing with complex operations or data transformations.
Key functional concepts:
- Higher-order functions
- Function composition
- Immutability
- Recursion
- Lazy evaluation
By applying these concepts, developers can create more modular and reusable code that is easier to reason about and less prone to side effects.
Last updated:
Review Summary
Java to Kotlin receives high praise from readers, with an average rating of 4.60 out of 5. Reviewers appreciate the book's approach to guiding Java developers towards Kotlin, explaining language differences and refactoring techniques. The authors' pragmatic view on programming and focus on best practices are commended. Readers find value in the book's discussion of "language grain" and its practical examples. While some found early chapters challenging, most agree it's an excellent resource for Java developers interested in Kotlin, offering insights beyond basic language transition.
Similar Books
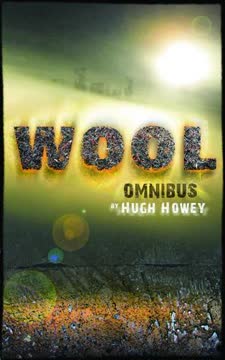
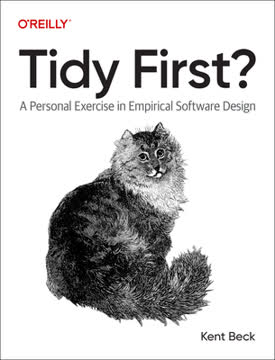
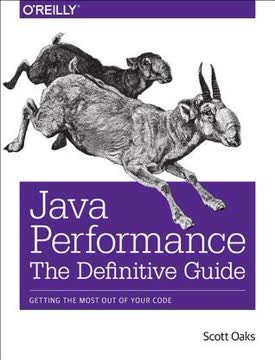
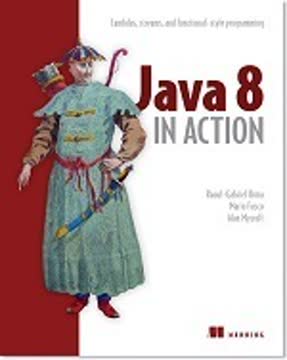
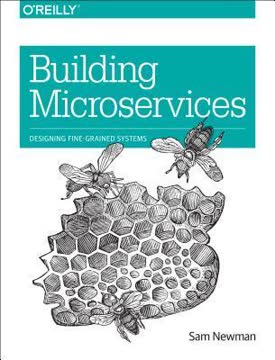
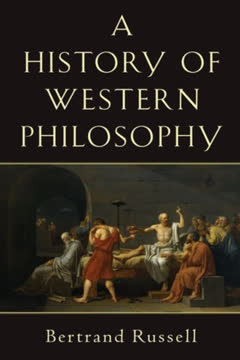
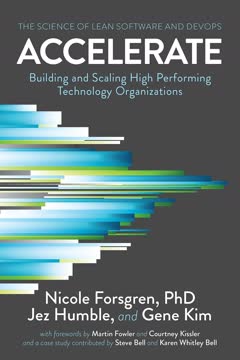
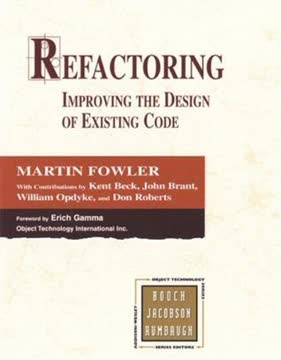
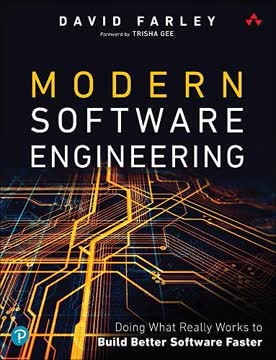
Download PDF
Download EPUB
.epub
digital book format is ideal for reading ebooks on phones, tablets, and e-readers.