Key Takeaways
1. Master the Setup & Essential Tools
All you need is an editor, a Terminal, and Python.
Get the basics right. Before writing any code, ensure your computer is set up correctly. This means installing Python 3.6+, a programmer's text editor like Atom (avoiding IDEs like IDLE), and learning to use your operating system's command line interface (Terminal on macOS/Linux, PowerShell on Windows). These are your fundamental tools.
Practice terminal commands. The command line is where you'll run your Python scripts and interact with your computer like a programmer. Spend time learning basic commands for navigation and file management.
pwd
: Print working directory (where you are)cd
: Change directory (move to a different folder)ls
(ordir
on Windows): List directory contentsmkdir
: Make directoryrmdir
: Remove directory
Use a text editor. A programmer's text editor provides features like syntax highlighting that make writing code easier. Save your Python files with a .py
extension. Learn how to create, save, and open files using your chosen editor and navigate to them using the terminal.
2. Type Code Exactly & Cultivate Attention to Detail
Every single character has to match for it to work.
Precision is paramount. Programming demands extreme attention to detail. Computers are literal; they execute exactly what you type, not what you meant to type. Copying code samples character by character, including punctuation and spacing, trains your brain to focus on these crucial details.
Build muscle memory. Manually typing code, rather than copy-pasting, builds muscle memory and familiarity with the syntax and common symbols. This repetition is a core technique of the "hard way" approach, designed to build fundamental skills through practice.
Spot differences visually. A key skill is the ability to quickly spot differences between your code and the correct code. When your code doesn't work, compare it line by line to the example, looking for even the smallest discrepancies. Reading code backward or out loud can help you see it differently and catch errors.
3. Understand Errors and Learn to Debug
Stop staring and ask.
Errors are inevitable. You will write bugs. Even experienced programmers make mistakes. The computer isn't wrong; your code is. Learning to read and understand error messages is crucial for fixing problems. Python's traceback tells you the file, line number, and type of error.
Interrogate your code. Don't just stare at broken code hoping the answer appears. Actively seek information. Use print
statements to output the values of variables at different points in your program to see what's happening and where things go wrong. This is the most effective debugging technique.
Break it on purpose. Once your code works, try to break it in different ways. This game helps you understand how Python interprets your code and how different mistakes manifest as errors. Learning how to break code makes you better at fixing it.
4. Learn Basic Data Types & Operations
In programming, a variable is nothing more than a name for something...
Store information. Variables are names you give to pieces of data, like numbers or text. This makes your code easier to read and manage. Assign values to variables using the =
(equals) sign. Variable names should be descriptive and start with a letter or underscore.
Perform calculations. Programming languages include built-in ways to perform mathematical operations. Learn the symbols for basic math:
+
: Addition-
: Subtraction*
: Multiplication/
: Division%
: Modulus (remainder)<
: Less than>
: Greater than<=
: Less than or equal to>=
: Greater than or equal to==
: Equal to!=
: Not equal to
Work with text. Text is represented as "strings" in programming. Strings are sequences of characters enclosed in single ('
) or double ("
) quotes. You can embed variables inside strings using f-strings (prefixed with f
) and curly braces {}
. Special "escape sequences" like \n
(newline) or \t
(tab) allow you to include difficult-to-type characters in strings.
5. Work with Collections: Strings, Lists, and Dictionaries
A Dictionary (or dict) is a way to store data just like a list, but instead of using only numbers to get the data, you can use almost anything.
Ordered sequences. Lists are ordered collections of items, created using square brackets []
and commas. Items in a list are accessed by their numerical index, starting from 0 (cardinal numbers). Lists are useful for maintaining order and accessing elements by position.
Key-value mappings. Dictionaries (dicts) are unordered collections that store data as key-value pairs, created using curly braces {}
. You access values using their associated keys, which can be almost any immutable type (like strings or numbers), not just numerical indices. Dicts are useful for looking up information based on a specific identifier.
Manipulate collections. Both lists and dictionaries have built-in functions (methods) to modify and interact with their contents. For example, append()
adds an item to a list, pop()
removes and returns an item, and dictionary methods like get()
retrieve values safely. Understanding these methods is key to working with data structures.
6. Control Program Flow with Logic and Loops
An if-statement creates what is called a “branch” in the code.
Make decisions. if
statements allow your program to make decisions based on whether a Boolean expression (something that evaluates to True
or False
) is true. Code under an if
statement runs only if the condition is met. Use elif
(else if) for additional conditions and else
for a default action if none of the if
or elif
conditions are true.
Repeat actions. Loops allow you to repeat a block of code multiple times. for
loops iterate over a sequence of items (like a list or string), executing the code block for each item. while
loops continue executing a code block as long as a given Boolean expression remains True
.
Structure code blocks. In Python, code blocks associated with if
, elif
, else
, for
, and while
statements are defined by indentation (four spaces). A colon :
at the end of the statement line indicates the start of a new indented block. Correct indentation is critical for Python to understand your code's structure.
7. Organize Code with Functions
Functions do three things: 1. They name pieces of code... 2. They take arguments... 3. Using 1 and 2, they let you make your own “mini-scripts” or “tiny commands.”
Bundle code. Functions are named blocks of reusable code defined using the def
keyword. They allow you to group related instructions and give them a descriptive name, making your programs more organized and easier to understand.
Accept inputs. Functions can take arguments (inputs) specified within parentheses ()
after the function name in the def
line. These arguments act like variables that receive values when the function is called. This allows functions to perform operations on different data each time they are used.
Produce outputs. Functions can return a value using the return
keyword. This allows the result of the function's computation to be used elsewhere in your program, often assigned to a variable. Functions that don't explicitly return a value implicitly return None
.
8. Grasp Object-Oriented Concepts: Classes and Objects
A class is a way to take a grouping of functions and data and place them inside a container so you can access them with the . (dot) operator.
Blueprints for things. Classes are blueprints or templates for creating new types of "things" (objects). They define the properties (attributes, like variables) and behaviors (methods, like functions) that objects of that class will have. Define a class using the class
keyword.
Instances of blueprints. An object is a specific instance created from a class blueprint. You create an object by "instantiating" the class, which looks like calling the class name as a function (e.g., my_object = MyClass()
). Each object has its own unique set of data based on the class definition.
Initialize objects. The __init__
method is a special function inside a class that runs automatically when you create a new object. It's used to set up the object's initial state, often by assigning values to its attributes. The first parameter of any method in a class, including __init__
, is conventionally named self
, which refers to the object itself.
9. Navigate OOP Relationships: Is-A, Has-A, Inheritance, and Composition
Most of the uses of inheritance can be simplified or replaced with composition, and multiple inheritance should be avoided at all costs.
Inheritance (Is-A). Inheritance is a relationship where one class ("child" or "subclass") derives properties and behaviors from another class ("parent" or "superclass"). This is an "is-a" relationship (e.g., a Dog
is-a Animal
). The child class inherits methods and attributes from the parent, which can be used implicitly, overridden, or altered using super()
.
Composition (Has-A). Composition is a relationship where one class contains instances of other classes as parts. This is a "has-a" relationship (e.g., a Car
has-a Engine
). Instead of inheriting features, the class uses the functionality of the objects it contains by calling their methods.
Choose wisely. Both inheritance and composition promote code reuse, but composition is generally preferred for its flexibility and clarity, especially avoiding complex "multiple inheritance." Use inheritance when there's a clear hierarchy and shared core functionality; use composition to assemble objects from different parts.
10. Build Software Incrementally with Automated Tests
Testing a piece of software is definitely boring and tedious, so you might as well write a little bit of code to do it for you.
Automate verification. Automated tests are small pieces of code that check if other parts of your code are working as expected. Using a testing framework like nose
, you write test functions (starting with test_
) that use assertion functions (like assert_equal
) to verify outcomes.
Develop test-first. A powerful technique is "test-first development," where you write the test before writing the code it tests. You write a test that describes how the code should work, watch it fail, and then write just enough code to make the test pass. This ensures your code meets requirements and is testable.
Build confidence. Automated tests provide a safety net. When you make changes or add new features, running your tests quickly tells you if you've broken existing functionality. This allows you to refactor and improve your code with greater confidence, reducing the time spent manually checking everything.
11. Apply Knowledge to Build a Web Application
Most of what software does is the following: 1. Takes some kind of input from a person. 2. Changes it. 3. Prints out something to show how it changed.
Web frameworks simplify. Building web applications involves handling network requests, routing URLs, processing input from forms, and generating HTML responses. Frameworks like Flask provide tools and structure to manage these complexities, allowing you to focus on your application's logic.
Handle requests and responses. A web application waits for requests from a browser (like asking for a specific URL). Based on the URL and request method (GET for viewing, POST for submitting forms), the application runs specific Python code (view functions). This code processes any input, interacts with your game logic, and generates an HTML response to send back to the browser.
Use templates and sessions. Templates (like Jinja2 used by Flask) allow you to create dynamic HTML pages by embedding Python variables and logic within the HTML structure. Sessions provide a way to store information about a specific user across multiple requests, essential for maintaining game state as the user navigates between pages.
Last updated:
Review Summary
Learn Python 3 the Hard Way receives mixed reviews. Many find it helpful for beginners, praising its practical approach and emphasis on coding practice. The book's straightforward style and thorough coverage of basics are appreciated. However, some criticize the author's condescending tone and abrupt increase in difficulty towards the end. Readers note that while it's good for learning syntax, it may not be ideal for understanding more complex concepts. The book's outdated content and lack of explanation for certain topics are also mentioned as drawbacks.
Similar Books
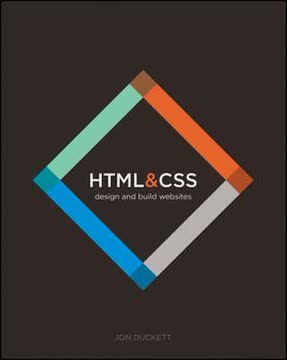
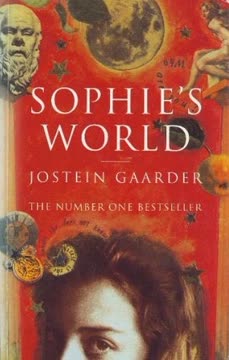
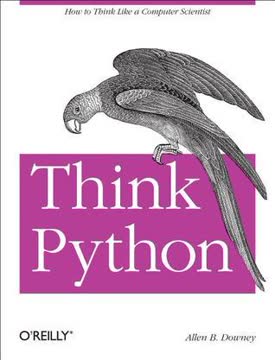
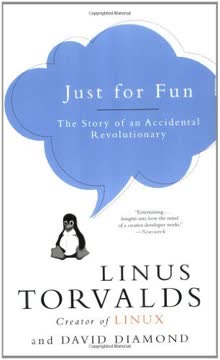
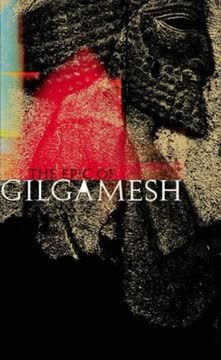
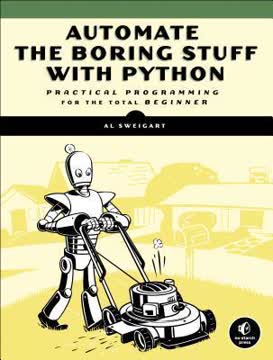
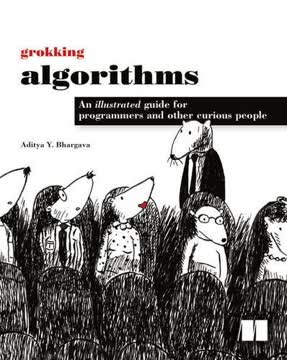
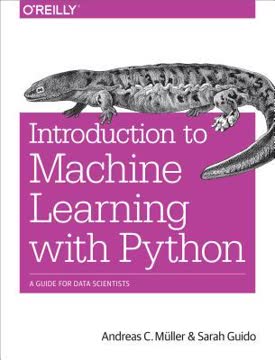
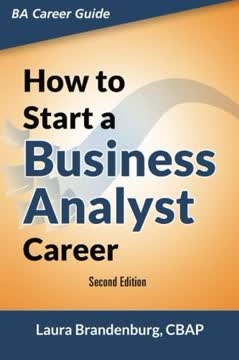
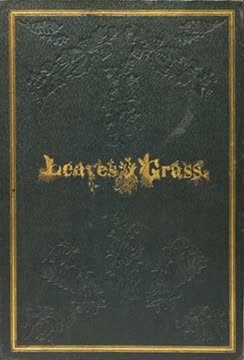
Download PDF
Download EPUB
.epub
digital book format is ideal for reading ebooks on phones, tablets, and e-readers.