Key Takeaways
1. Object-oriented design principles form the foundation of Python programming
"Objects are things that we can sense, feel, and manipulate."
Abstraction and encapsulation: Object-oriented programming (OOP) in Python revolves around creating abstract representations of real-world concepts. Objects encapsulate both data (attributes) and behavior (methods), allowing for modular and organized code. This approach promotes code reusability and maintainability by grouping related functionality together.
Identifying objects: When designing a Python program, start by identifying the key objects in your problem domain. Consider what data each object should hold and what actions it should be able to perform. This process of abstraction helps create a clear mental model of the system you're building.
- Examples of objects: Person, Car, BankAccount
- Attributes: name, age, balance
- Methods: drive(), deposit(), withdraw()
2. Classes and objects are the building blocks of OOP in Python
"A class is a blueprint for creating objects."
Class definition: In Python, classes are defined using the class
keyword, followed by the class name and a colon. The class body contains attribute definitions and method implementations. Methods are simply functions defined within the class, with the first parameter typically named self
, referring to the instance of the object.
Object instantiation: Objects are created from classes using the constructor syntax, which calls the __init__
method. This special method initializes the object's attributes and performs any necessary setup.
- Basic class structure:
class ClassName: def __init__(self, param1, param2): self.attribute1 = param1 self.attribute2 = param2 def method_name(self): # Method implementation
3. Inheritance and polymorphism enable code reuse and flexibility
"Inheritance provides abstraction."
Inheritance hierarchy: Python supports single and multiple inheritance, allowing classes to inherit attributes and methods from one or more parent classes. This creates a hierarchy of classes, promoting code reuse and organization.
Polymorphism: Derived classes can override methods from their parent classes, providing specialized implementations while maintaining a consistent interface. This enables polymorphic behavior, where objects of different classes can be treated uniformly if they share a common interface.
- Example of inheritance:
class Animal: def speak(self): pass class Dog(Animal): def speak(self): return "Woof!" class Cat(Animal): def speak(self): return "Meow!"
4. Python's built-in data structures offer powerful tools for organizing information
"Dictionaries are incredibly useful containers that allow us to map objects directly to other objects."
Versatile data structures: Python provides several built-in data structures, each with its own strengths and use cases. Lists offer ordered, mutable sequences, while tuples provide immutable sequences. Sets store unique elements, and dictionaries allow key-value mappings.
Choosing the right structure: Selecting the appropriate data structure can significantly impact your program's performance and readability. Consider the operations you'll need to perform on the data and choose accordingly.
- Common data structures:
- List:
[1, 2, 3]
- Tuple:
(1, 2, 3)
- Set:
{1, 2, 3}
- Dictionary:
{'a': 1, 'b': 2, 'c': 3}
- List:
5. Strings and serialization are crucial for data manipulation and storage
"Python strings are all represented in Unicode, a character definition standard that can represent virtually any character in any language on the planet."
String manipulation: Python offers a rich set of string manipulation methods, making it easy to process and transform text data. From simple operations like concatenation and slicing to more complex tasks like regular expression matching, Python provides powerful tools for working with strings.
Serialization: When it comes to storing complex data structures or transmitting them over networks, serialization is key. Python's pickle
module allows for easy serialization and deserialization of Python objects, while the json
module provides interoperability with other systems through the widely-used JSON format.
- String operations:
- Concatenation:
"Hello" + " " + "World"
- Formatting:
f"The answer is {42}"
- Splitting:
"a,b,c".split(',')
- Concatenation:
- Serialization examples:
- Pickle:
pickle.dumps(my_object)
- JSON:
json.dumps(my_dict)
- Pickle:
6. The iterator pattern and generators streamline data processing
"The iterator protocol is one of the most powerful design patterns."
Iterator protocol: Python's iterator protocol allows for efficient traversal of collections and custom objects. By implementing the __iter__()
and __next__()
methods, you can create objects that work seamlessly with Python's for
loops and other iteration constructs.
Generators: Python generators provide a concise way to create iterators. Using the yield
keyword, you can define functions that generate a sequence of values on-the-fly, saving memory and improving performance for large datasets or infinite sequences.
- Iterator example:
class CountUp: def __init__(self, start, end): self.current = start self.end = end def __iter__(self): return self def __next__(self): if self.current > self.end: raise StopIteration else: self.current += 1 return self.current - 1
- Generator example:
def count_up(start, end): current = start while current <= end: yield current current += 1
7. Design patterns provide elegant solutions to common programming challenges
"Design patterns are an attempt to bring this same formal definition for correctly designed structures to software engineering."
Common patterns: Design patterns offer reusable solutions to recurring problems in software design. Some commonly used patterns include:
- Singleton: Ensures a class has only one instance
- Factory: Provides an interface for creating objects in a superclass
- Observer: Defines a one-to-many dependency between objects
- Decorator: Adds new functionality to objects without altering their structure
Choosing patterns: When selecting a design pattern, consider the specific problem you're trying to solve and the long-term maintainability of your code. While patterns can greatly improve code organization, overuse or misapplication can lead to unnecessary complexity.
- Pattern selection criteria:
- Problem relevance
- Scalability requirements
- Team familiarity
- Performance considerations
8. Python's unique features simplify implementation of traditional design patterns
"Python is very good at blurring distinctions; it doesn't exactly help us to 'think outside the box'. Rather, it teaches us to stop thinking about the box."
Pythonic implementations: Many traditional design patterns can be implemented more elegantly in Python due to its dynamic nature and first-class functions. For example, the Strategy pattern can often be replaced with simple function passing, and the Decorator pattern is built into the language syntax.
Language-specific patterns: Python also introduces its own patterns and idioms that leverage its unique features. The context manager pattern, implemented using the with
statement, is a prime example of a Pythonic approach to resource management.
- Simplified pattern examples:
- Strategy: Use functions as arguments
- Decorator: Use the
@decorator
syntax - Context Manager: Implement
__enter__
and__exit__
methods
- Python-specific patterns:
- Generator expressions
- List comprehensions
- Descriptor protocol
Last updated:
Review Summary
Python 3 Object-oriented Programming receives generally positive reviews for its clear explanations of OOP concepts in Python. Readers appreciate its practical examples, design pattern coverage, and suitability for both beginners and experienced programmers. The book is praised for its engaging writing style and comprehensive content. Some reviewers note that certain examples may be outdated, and a few find the case studies abstract. Overall, it's recommended for those looking to improve their Python OOP skills, with many readers finding it valuable for understanding complex concepts and improving code structure.
Similar Books
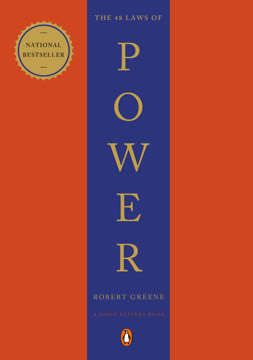
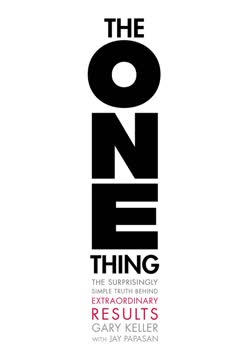
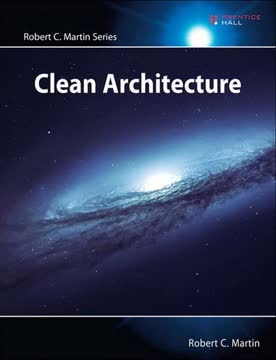
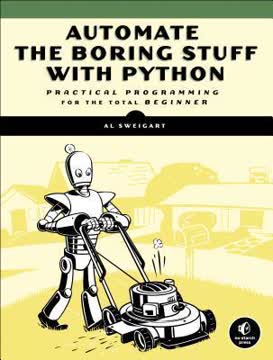
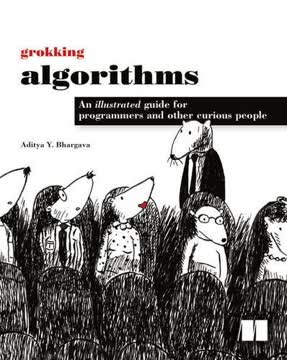

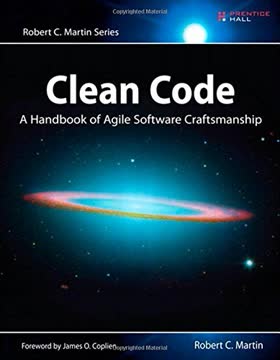
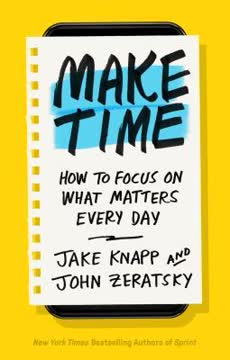
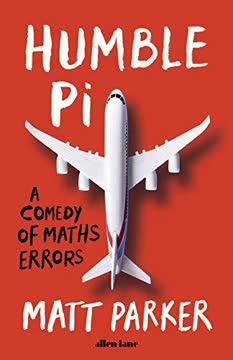
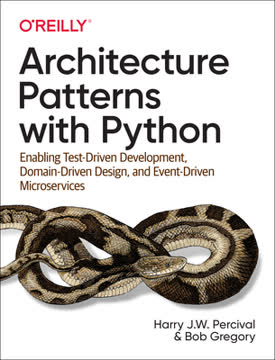
Download PDF
Download EPUB
.epub
digital book format is ideal for reading ebooks on phones, tablets, and e-readers.