Key Takeaways
1. Python's functions are first-class objects
Functions are objects—they can be assigned to variables, stored in data structures, passed to and returned from other functions.
Functions as data. In Python, functions are treated as first-class citizens, meaning they can be manipulated like any other object. This allows for powerful programming paradigms:
- Assigning functions to variables
- Storing functions in lists, dictionaries, or other data structures
- Passing functions as arguments to other functions
- Returning functions from other functions
- Defining functions inside other functions (nested functions)
This flexibility enables advanced programming techniques like higher-order functions, closures, and functional programming patterns, making Python a versatile and expressive language for complex problem-solving.
2. Decorators enhance and modify function behavior
Decorators define reusable building blocks you can apply to a callable to modify its behavior without permanently modifying the callable itself.
Syntactic sugar for modification. Decorators provide a clean and reusable way to extend or modify the behavior of functions or classes:
- They wrap a function, modifying its behavior without changing its source code
- Common uses include logging, timing, access control, and caching
- Decorators can be stacked, allowing multiple modifications to a single function
- They promote the principle of separation of concerns, keeping code modular
Decorators are implemented using the @
syntax, making the code more readable and maintainable. They leverage Python's first-class functions and closures to provide a powerful mechanism for meta-programming.
3. *args and **kwargs enable flexible function arguments
*args and **kwargs let you write functions with a variable number of arguments in Python.
Variable argument flexibility. These special syntax elements allow functions to accept any number of positional or keyword arguments:
*args
collects extra positional arguments into a tuple**kwargs
collects extra keyword arguments into a dictionary- They provide flexibility in function definitions, allowing for future expansion
- Useful for creating wrapper functions or APIs with optional parameters
This feature enables the creation of more general-purpose functions and helps in writing code that can adapt to changing requirements without modifying the function signature.
4. Comprehensions provide concise syntax for creating collections
Comprehensions are just fancy syntactic sugar for a simple for-loop pattern.
Elegant collection creation. List, set, and dictionary comprehensions offer a compact way to create collections based on existing iterables:
- They combine the creation and population of a collection in a single line
- Often more readable and faster than equivalent for loops
- Can include conditions for filtering elements
- Available for lists, sets, and dictionaries
Examples:
- List comprehension:
[x*2 for x in range(10) if x % 2 == 0]
- Set comprehension:
{word.lower() for word in words}
- Dictionary comprehension:
{k: v**2 for k, v in my_dict.items()}
While powerful, it's important to balance conciseness with readability, avoiding overly complex comprehensions that might obscure the code's intent.
5. Generator functions and expressions simplify iterator creation
Generators abstract away much of the boilerplate code needed when writing class-based iterators.
Effortless iteration. Generators provide a simple way to create iterators without the need for a full class implementation:
- Use the
yield
keyword to produce a series of values - Memory-efficient, as they generate values on-the-fly
- Can be used in for loops, list comprehensions, and other iterable contexts
- Generator expressions offer a concise syntax for simple generators
Generator functions:
python
def countdown(n):
while n > 0:
yield n
n -= 1
Generator expression:
python
squares = (x*x for x in range(10))
Generators are particularly useful for working with large datasets or infinite sequences, as they don't store all values in memory at once.
6. Dictionaries are versatile and powerful data structures
Dictionaries are the central data structure in Python.
Efficient key-value storage. Dictionaries provide fast, flexible, and feature-rich associative arrays:
- O(1) average time complexity for key lookup, insertion, and deletion
- Support any hashable object as keys (strings, numbers, tuples)
- Offer methods like
get()
for safe key access with default values - Can be used to emulate switch/case statements from other languages
Advanced dictionary features:
- OrderedDict: Remembers the order of inserted keys
- defaultdict: Provides default values for missing keys
- ChainMap: Searches multiple dictionaries as a single mapping
Dictionaries are fundamental to Python's implementation and are used extensively in the language itself and in many Python libraries and frameworks.
7. Effective looping techniques improve code readability
Writing C-style loops in Python is considered unpythonic.
Pythonic iteration. Python provides several idiomatic ways to loop over sequences and perform iterations:
- Use
for item in iterable
instead of indexing - Employ
enumerate()
when you need both index and value - Utilize
zip()
to iterate over multiple sequences simultaneously - Take advantage of the
itertools
module for complex iterations
Examples:
python
Instead of:
for i in range(len(items)):
print(i, items[i])
Use:
for i, item in enumerate(items):
print(i, item)
Looping over two lists:
for name, age in zip(names, ages):
print(f"{name} is {age} years old")
These techniques lead to more concise, readable, and efficient code, aligning with Python's philosophy of clarity and simplicity.
8. Object-oriented programming concepts optimize code organization
Abstract Base Classes (ABCs) ensure that derived classes implement particular methods from the base class.
Structured code design. Python's object-oriented features provide powerful tools for organizing and structuring code:
- Classes encapsulate data and behavior
- Inheritance allows for code reuse and specialization
- Abstract Base Classes define interfaces and enforce implementation
- Properties provide controlled access to attributes
- Special methods (dunder methods) allow customization of object behavior
Key OOP concepts in Python:
- Polymorphism through duck typing
- Multiple inheritance and method resolution order (MRO)
- Composition as an alternative to inheritance
- Metaclasses for advanced class creation control
Effective use of OOP principles leads to more maintainable, extensible, and modular code structures.
9. Data structures in Python offer diverse functionality
If you're not looking for parallel processing support, the implementation offered by collections.deque is an excellent default choice for implementing a FIFO queue data structure in Python.
Tailored data organization. Python provides a rich set of built-in and standard library data structures to suit various needs:
- Lists: Dynamic arrays for general-purpose sequences
- Tuples: Immutable sequences for fixed collections
- Sets: Unordered collections of unique elements
- Deques: Double-ended queues for efficient insertion/deletion at both ends
- Heapq: Priority queue implementation
- Counter: Multiset for counting hashable objects
Choosing the right data structure can significantly impact the performance and clarity of your code. Consider factors like:
- Required operations (e.g., frequent insertions, deletions, lookups)
- Memory usage
- Thread safety requirements
- Need for ordering or sorting
Understanding the characteristics and trade-offs of different data structures enables more efficient and elegant solutions to programming problems.
10. Efficient string formatting enhances code clarity
If your format strings are user-supplied, use Template Strings to avoid security issues. Otherwise, use Literal String Interpolation if you're on Python 3.6+, and "New Style" String Formatting if you're not.
Clear and secure string construction. Python offers multiple methods for string formatting, each with its own strengths:
- %-formatting: Old-style, still widely used
- str.format(): More readable and flexible
- f-strings: Concise and powerful, available in Python 3.6+
- Template strings: Safer for user-supplied formats
F-strings example:
python
name = "Alice"
age = 30
print(f"{name} is {age} years old")
Template strings for user input:
python
from string import Template
user_input = Template("Hello, $name!")
print(user_input.substitute(name="Bob"))
Choosing the appropriate string formatting method improves code readability, maintainability, and security, especially when dealing with user-supplied data or complex string constructions.
Last updated:
FAQ
What's "Python Tricks: A Buffet of Awesome Python Features" about?
- Overview: "Python Tricks" by Dan Bader is a guide to mastering Python by exploring its advanced features and idiomatic practices. It aims to elevate a Python developer's skills from intermediate to advanced.
- Content Structure: The book is structured into chapters that cover various Python features, including cleaner code patterns, effective functions, object-oriented programming, and data structures.
- Practical Examples: Each chapter includes practical examples and code snippets to illustrate the concepts, making it easier for readers to understand and apply them.
- Community Insights: The book also shares insights from the Python community, including feedback from experienced developers, to provide a well-rounded perspective on Python programming.
Why should I read "Python Tricks: A Buffet of Awesome Python Features"?
- Skill Enhancement: The book is designed to help Python developers become more effective, knowledgeable, and practical in their coding practices.
- Advanced Techniques: It introduces advanced Python features and idioms that can significantly improve code quality and efficiency.
- Real-World Application: The examples and tricks are applicable to real-world scenarios, making it a valuable resource for professional development.
- Community Engagement: Reading the book can also connect you with the broader Python community, as it includes insights and feedback from other developers.
What are the key takeaways of "Python Tricks: A Buffet of Awesome Python Features"?
- Cleaner Code Patterns: Learn how to write cleaner and more maintainable Python code using patterns like context managers and assertions.
- Effective Functions: Understand the power of first-class functions, decorators, and argument unpacking to create more flexible and reusable code.
- Object-Oriented Programming: Gain insights into Python's OOP features, including class and instance variables, abstract base classes, and namedtuples.
- Data Structures and Iteration: Explore Python's built-in data structures and learn how to use iterators, generators, and comprehensions effectively.
How does Dan Bader define a "Python Trick"?
- Teaching Tool: A Python Trick is a short code snippet meant to teach an aspect of Python with a simple illustration.
- Motivational Example: It serves as a motivating example, enabling readers to dig deeper and develop an intuitive understanding of Python.
- Community Origin: The concept started as a series of code screenshots shared on Twitter, which received positive feedback and led to the creation of the book.
- Accessible Learning: The tricks are designed to be accessible and easy to understand, making them suitable for developers looking to enhance their Python skills.
What are some patterns for cleaner Python code mentioned in the book?
- Assertions: Use assertions to automatically detect errors in your programs, making them more reliable and easier to debug.
- Complacent Comma Placement: Adopt a code style that avoids comma placement issues in lists, dicts, or set constants.
- Context Managers: Simplify resource management patterns using the with statement and context managers.
- Underscores and Dunders: Understand the meaning of single and double underscores in variable and method names to write more idiomatic Python code.
How does "Python Tricks" explain the use of decorators?
- Behavior Modification: Decorators allow you to extend and modify the behavior of a callable without permanently changing it.
- Reusable Building Blocks: They define reusable building blocks that can be applied to functions or classes to add functionality like logging or access control.
- Syntax and Stacking: The book explains the @ syntax for decorators and how multiple decorators can be stacked to accumulate their effects.
- Debugging Best Practices: It recommends using functools.wraps to carry over metadata from the undecorated callable to the decorated one for better debugging.
What are the differences between class, instance, and static methods in Python?
- Instance Methods: Require a class instance and can access the instance through the self parameter, allowing them to modify object state.
- Class Methods: Use the @classmethod decorator and take a cls parameter, allowing them to modify class state but not instance state.
- Static Methods: Use the @staticmethod decorator and do not take self or cls parameters, making them independent of class and instance state.
- Use Cases: The book provides examples of when to use each method type, emphasizing their role in communicating developer intent and maintaining code.
How does "Python Tricks" suggest handling dictionary default values?
- Avoid Explicit Checks: Instead of using explicit key in dict checks, use the get() method to provide a default value for missing keys.
- EAFP Principle: Follow the "easier to ask for forgiveness than permission" coding style by using try...except blocks to handle KeyError exceptions.
- Defaultdict Usage: In some cases, the collections.defaultdict class can be helpful for automatically handling missing keys with default values.
- Concise Implementation: The book provides examples of how to implement these techniques concisely and effectively.
What are some advanced string formatting techniques covered in the book?
- Old Style Formatting: Uses the %-operator for simple positional formatting, similar to printf-style functions in C.
- New Style Formatting: Introduced in Python 3, it uses the format() method for more powerful and flexible string formatting.
- Literal String Interpolation: Available in Python 3.6+, it allows embedded Python expressions inside string constants using f-strings.
- Template Strings: A simpler and less powerful mechanism for string formatting, useful for handling user-generated format strings safely.
How does "Python Tricks" explain the use of list comprehensions?
- Syntactic Sugar: List comprehensions are a concise way to create lists by iterating over a collection and applying an expression to each item.
- Template Pattern: The book provides a template pattern for transforming for-loops into list comprehensions and vice versa.
- Filtering Elements: Comprehensions can include conditions to filter elements, allowing for more complex list generation.
- Readability Considerations: While powerful, the book cautions against overusing comprehensions, especially with deep nesting, to maintain code readability.
What are some key quotes from "Python Tricks" and what do they mean?
- "Mastering Python programming isn’t just about grasping the theoretical aspects of the language." This emphasizes the importance of understanding and adopting the conventions and best practices used by the Python community.
- "A book that works like a buffet of awesome Python features (yum!) and keeps motivation levels high." This highlights the book's approach of presenting Python features in an engaging and motivating way.
- "Python’s assert statement is a debugging aid that tests a condition as an internal self-check in your program." This quote underscores the importance of using assertions for debugging and maintaining code reliability.
- "Decorators define reusable building blocks you can apply to a callable to modify its behavior without permanently modifying the callable itself." This explains the power and flexibility of decorators in enhancing code functionality.
How does "Python Tricks" address the use of virtual environments?
- Dependency Isolation: Virtual environments help isolate project dependencies, preventing version conflicts between packages and Python versions.
- Best Practice: The book recommends using virtual environments for all Python projects to keep dependencies separate and avoid headaches.
- Activation and Deactivation: It explains how to create, activate, and deactivate virtual environments to manage project-specific dependencies.
- Security Benefits: Using virtual environments reduces security risks by avoiding the need for superuser permissions when installing packages.
Review Summary
Python Tricks is highly praised for its concise yet insightful approach to intermediate and advanced Python topics. Readers appreciate its practical tips, clear explanations, and focus on best practices. Many found it helpful for filling knowledge gaps and improving coding skills. The book's conversational tone and systematic structure make it accessible and enjoyable. While some experienced developers found it less challenging, most agree it's an excellent resource for those looking to deepen their Python expertise and write more idiomatic code.
Similar Books
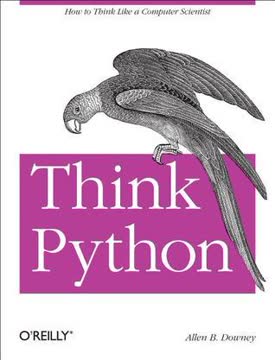
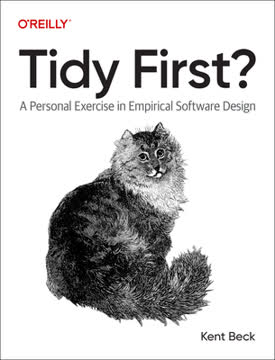
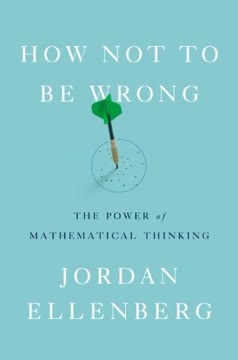
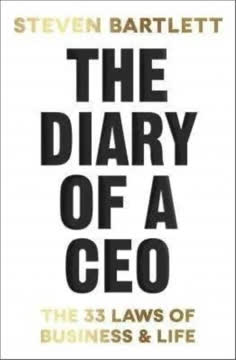
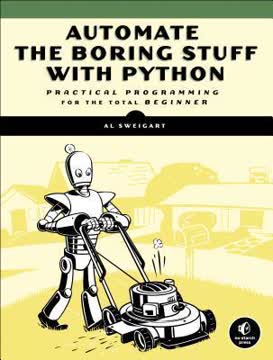
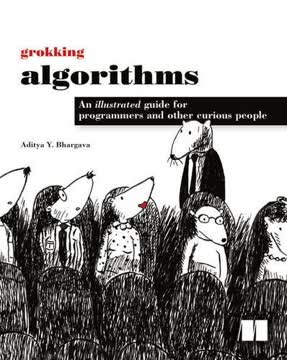
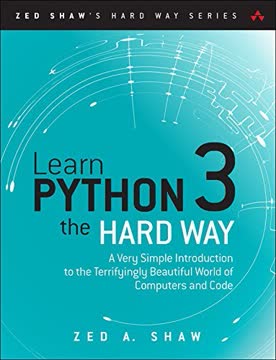
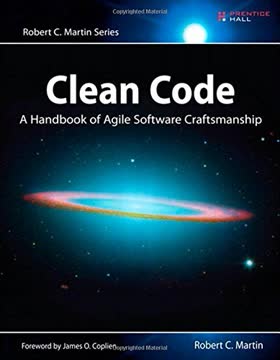
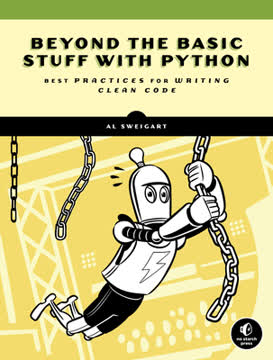
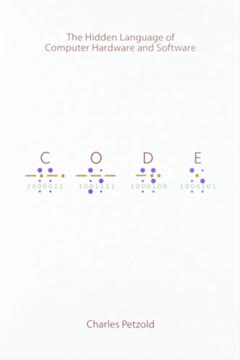
Download PDF
Download EPUB
.epub
digital book format is ideal for reading ebooks on phones, tablets, and e-readers.