Key Takeaways
1. Decorators: Enhancing Functions with Syntactic Sugar
A decorator is a callable that takes another function as an argument (the decorated function).
Syntactic elegance. Decorators provide a clean and readable way to modify or enhance the behavior of functions without altering their core logic. They are applied using the "@" syntax, which is essentially syntactic sugar for passing a function as an argument to another function. This allows for powerful metaprogramming techniques, where you can add functionality such as logging, timing, or access control to existing functions.
Execution timing. One crucial aspect of decorators is that they are executed immediately when a module is loaded, typically at import time. This means they can be used to register functions, modify class or function attributes, or perform other setup tasks before the decorated function is actually called. However, the decorated function itself is only executed when explicitly invoked.
Common use cases for decorators:
- Logging and debugging
- Timing and performance analysis
- Access control and authentication
- Caching and memoization
- Input validation and type checking
2. Closures: Capturing and Preserving State in Nested Functions
A closure is a function that retains the bindings of the free variables that exist when the function is defined, so that they can be used later when the function is invoked and the defining scope is no longer available.
Preserving context. Closures are a powerful feature in Python that allow inner functions to capture and remember the state of variables in their outer scope, even after the outer function has finished executing. This enables the creation of function factories and the implementation of data hiding and encapsulation without using classes.
Practical applications. Closures are fundamental to many programming patterns in Python, including decorators, callbacks, and certain functional programming techniques. They provide a way to create functions with "memory," which can be particularly useful for maintaining state across function calls or creating specialized functions based on runtime parameters.
Key points about closures:
- They "close over" variables in their lexical scope
- The enclosed variables are stored in the function's closure attribute
- Closures enable partial function application and currying
- They are the basis for many advanced Python features and design patterns
3. Variable Scope Rules: Understanding Local, Global, and Nonlocal Variables
If there is a nonlocal x declaration, x comes from and is assigned to the x local variable of the nearest surrounding function where x is defined.
Scope hierarchy. Python's variable scope rules define how names are looked up in nested functions. Understanding these rules is crucial for writing correct and efficient code, especially when dealing with closures and decorators. The order of lookup goes from local, to enclosing functions, to global, and finally to built-in scopes.
The nonlocal keyword. Introduced in Python 3, nonlocal allows inner functions to assign to variables in their enclosing scope. This is particularly important for closures that need to modify state captured from their outer scope. Without nonlocal, attempting to assign to an outer scope variable would instead create a new local variable, potentially leading to unexpected behavior.
Python's variable scope rules:
- Local scope (function)
- Enclosing functions (nonlocal)
- Global scope (module)
- Built-in scope (Python's built-in names)
- The global keyword can be used to indicate that a name refers to a global variable
- nonlocal is used for variables in enclosing (but non-global) scopes
4. Implementing Decorators: From Simple to Parameterized
To accept parameters, the new register decorator must be called as a function
Nested functions. Implementing decorators typically involves nested function definitions. The outermost function (the decorator itself) takes the function to be decorated as an argument. It then defines and returns an inner function that wraps the original function, adding the desired functionality.
Parameterized decorators. To create decorators that accept arguments, you need to add another layer of nesting. The outermost function becomes a decorator factory that takes the parameters and returns the actual decorator. This allows for more flexible and configurable decorators, but also increases complexity.
Structure of a basic decorator:
- Decorator function (takes the function to be decorated)
- Wrapper function (adds functionality and calls original function)
Structure of a parameterized decorator:
- Decorator factory (takes decorator parameters)
- Decorator function (takes the function to be decorated)
- Wrapper function (adds functionality and calls original function)
5. Standard Library Decorators: Leveraging Built-in Power Tools
functools.cache was added in Python 3.9. If you need to run these examples in Python 3.8, replace @cache with @lru_cache.
Ready-made solutions. Python's standard library provides several powerful decorators that solve common programming problems. These include property for creating managed attributes, classmethod and staticmethod for altering method behavior, and various tools in the functools module for optimization and function manipulation.
Optimization decorators. The functools module offers particularly useful decorators for performance optimization. The @cache decorator (and its predecessor @lru_cache) provides memoization, storing function results to avoid repeated computations. The @singledispatch decorator enables you to create generic functions with behavior that varies based on the type of the first argument.
Key standard library decorators:
- @property: Create managed attributes
- @classmethod: Define methods that operate on the class, not instances
- @staticmethod: Define methods that don't need access to class or instance
- @functools.cache: Memoize function results
- @functools.lru_cache: Memoize with a size-limited cache
- @functools.singledispatch: Create type-based dispatching functions
6. Single Dispatch Generic Functions: Polymorphism in Python
The advantage of @singledispatch is supporting modular extension: each module can register a specialized function for each type it supports.
Type-based dispatching. The @singledispatch decorator provides a way to create functions that behave differently based on the type of their first argument. This enables a form of function overloading or ad-hoc polymorphism in Python, which is particularly useful when you need to implement operations that vary across different types.
Extensibility. One of the key benefits of @singledispatch is that it allows for modular and extensible code. New type-specific implementations can be registered at any time, even for types defined in third-party libraries. This makes it possible to adapt and extend functionality without modifying the original code.
Benefits of @singledispatch:
- Enables type-based function dispatching
- Allows for easy extension with new types
- Supports registration of implementations for abstract base classes
- Maintains clean and modular code structure
Usage pattern:
- Decorate the base function with @singledispatch
- Register type-specific implementations with @base_function.register(type)
7. Memoization and Caching: Optimizing Function Performance
functools.cache implements memoization: an optimization technique that works by saving the results of previous invocations of an expensive function, avoiding repeat computations on previously used arguments.
Performance boost. Memoization is a powerful optimization technique that can dramatically improve the performance of functions with expensive computations, especially when they are called repeatedly with the same arguments. The @functools.cache and @functools.lru_cache decorators provide easy-to-use implementations of this technique.
Trade-offs. While caching can provide significant speed improvements, it comes with trade-offs in terms of memory usage. The @cache decorator stores all results indefinitely, which can lead to high memory consumption for long-running processes. The @lru_cache decorator provides more control with its maxsize parameter, allowing you to limit the cache size and automatically evict least recently used entries.
When to use memoization:
- Functions with expensive computations
- Pure functions (same input always produces same output)
- Functions called repeatedly with the same arguments
Considerations:
- Memory usage vs. Computation time
- Cache size and eviction policies (@lru_cache)
- Thread safety in concurrent environments
8. The Art of Gradual Typing: Balancing Flexibility and Safety
Type hints are optional at all levels: you can have entire packages with no type hints, you can silence the type checker when you import one of those packages into a module where you use type hints, and you can add special comments to make the type checker ignore specific lines in your code.
Enhanced tooling. Python's gradual typing system, introduced with PEP 484, allows developers to add type hints to their code incrementally. These hints are used by static type checkers and IDEs to catch potential errors early and provide better code completion and refactoring support. However, they do not affect the runtime behavior of the program.
Flexibility preserved. The gradual nature of Python's typing system means that you can add type hints where they provide the most value, without being forced to annotate every part of your codebase. This preserves Python's flexibility and ease of use, while still allowing for increased safety and tooling support in critical or complex areas of your code.
Benefits of gradual typing:
- Catch errors earlier in the development process
- Improve code readability and self-documentation
- Enable better IDE support and tooling
- Facilitate easier refactoring and maintenance
Challenges and considerations:
- Learning curve for type hint syntax and concepts
- Potential for over-complication in simple scripts
- Need for balance between flexibility and strictness
- Compatibility with third-party libraries
</most_relevant_traits>
Last updated:
Review Summary
Fluent Python receives overwhelmingly positive reviews, praised for its in-depth coverage of advanced Python topics. Readers appreciate the clear explanations, practical examples, and insights into Python's design philosophy. Many consider it essential for intermediate to advanced programmers seeking to deepen their understanding of the language. The book is noted for its comprehensive approach, covering topics like data models, concurrency, and metaprogramming. While some found it challenging, most agree it's a valuable resource for mastering Python's intricacies and writing idiomatic code.
Similar Books
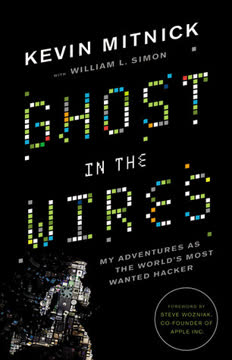
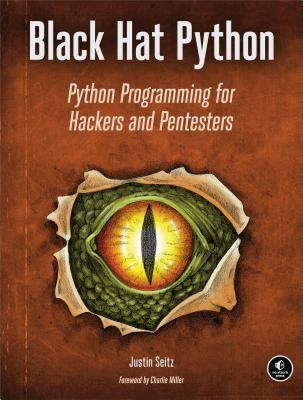
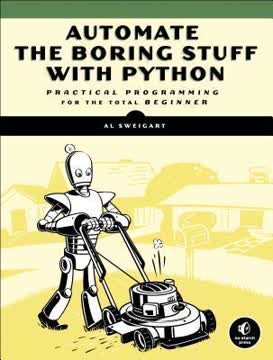
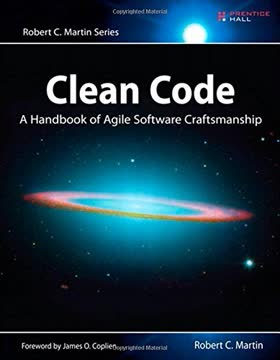


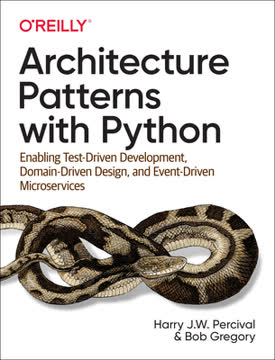
Download PDF
Download EPUB
.epub
digital book format is ideal for reading ebooks on phones, tablets, and e-readers.