Key Takeaways
1. Python's core data types: numbers, strings, lists, and dictionaries
Built-in objects make programs easy to write.
Versatile building blocks. Python's core data types provide a robust foundation for programming. Numbers support mathematical operations, while strings handle text processing. Lists offer ordered collections of items, and dictionaries provide key-value mappings. These types are mutable or immutable, affecting how they can be modified.
Powerful operations. Each type comes with a set of built-in operations and methods. For example:
- Strings: slicing, concatenation, and methods like split() and join()
- Lists: indexing, slicing, append(), and extend()
- Dictionaries: key-based access, update(), and keys() method
Flexibility and efficiency. Python's core types are designed for ease of use and performance. They can be nested to create complex data structures, and many operations are optimized for speed. This combination of simplicity and power makes Python suitable for a wide range of programming tasks.
2. Dynamic typing and references in Python
Names have no type, but objects do.
Type flexibility. Python uses dynamic typing, meaning variables can refer to objects of any type. This allows for more flexible and concise code, as the same variable can hold different types of data at different times. However, it also requires careful attention to ensure type compatibility during operations.
Reference model. In Python, variables are essentially names that refer to objects in memory. When you assign a value to a variable, you're creating a reference to an object. This has important implications:
- Multiple names can refer to the same object
- Mutable objects can be changed in-place, affecting all references
- Immutable objects create new objects when "modified"
Understanding this reference model is crucial for writing efficient and bug-free Python code, especially when dealing with function arguments and shared data structures.
3. Python's statement syntax and structure
Block and statement boundaries are detected automatically.
Indentation matters. Unlike many other languages, Python uses indentation to define code blocks. This enforces clean, readable code by design. The colon (:) character is used to introduce blocks in compound statements like if, for, while, and function definitions.
Statement types. Python offers a variety of statement types:
- Assignment statements (=, +=, etc.)
- Conditional statements (if, elif, else)
- Loop statements (for, while)
- Function and class definitions (def, class)
- Exception handling (try, except, finally)
Simplicity and readability. Python's syntax is designed to be clear and intuitive. Many operations that require multiple lines in other languages can be expressed concisely in Python, such as list comprehensions and conditional expressions.
4. Iteration and loop constructs in Python
For loops and list comprehensions are often the simplest and fastest way to get real work done in Python.
Powerful iteration tools. Python provides several ways to iterate over data:
- for loops for sequences and other iterables
- while loops for condition-based iteration
- List comprehensions for concise data transformation
- Generator expressions for memory-efficient iteration
Iterable protocol. Python's iteration model is based on the iterable protocol, allowing custom objects to be iterable. This unified approach means that many built-in functions and constructs work seamlessly with user-defined types that implement the protocol.
Efficiency and readability. Iteration in Python is designed to be both efficient and easy to read. List comprehensions, for example, can often replace multi-line for loops with a single, expressive line of code. The built-in range(), enumerate(), and zip() functions further enhance the power and flexibility of Python's iteration tools.
5. Functions: reusable code blocks in Python
Functions are the most basic program structure Python provides for maximizing code reuse and minimizing code redundancy.
Modular code organization. Functions in Python allow you to encapsulate reusable pieces of code. This promotes:
- Code reuse and reduction of redundancy
- Easier maintenance and debugging
- Improved readability and organization
Flexible arguments. Python functions support various parameter types:
- Positional arguments
- Keyword arguments
- Default argument values
- Variable-length argument lists (*args, **kwargs)
Return values and side effects. Functions can return values explicitly using the return statement, or implicitly return None. They can also produce side effects by modifying mutable objects or global variables. Understanding the difference between return values and side effects is crucial for writing clear and predictable code.
6. Scopes and namespaces in Python
When you use a name in a program, Python creates, changes, or looks up the name in what is known as a namespace—a place where names live.
LEGB rule. Python uses the LEGB rule for name resolution:
- Local: Names defined within the current function
- Enclosing: Names in the local scope of any enclosing functions
- Global: Names defined at the top level of the module
- Built-in: Names in the built-in module
Name assignment and the global statement. By default, assigning a value to a name inside a function creates or changes a local variable. The global statement allows you to explicitly work with global variables within a function scope.
Namespace implications. Understanding scopes and namespaces is crucial for:
- Avoiding naming conflicts
- Managing variable lifetime and visibility
- Writing more maintainable and modular code
Proper use of scopes can help create more self-contained and reusable function definitions.
7. Modules and code organization in Python
Modules are simply packages of variables—that is, namespaces.
Code organization. Modules in Python serve as the primary means of organizing larger programs:
- Each .py file is a module
- Modules can contain variables, functions, and classes
- Modules can import other modules
Namespace management. Modules create separate namespaces, which help avoid naming conflicts in large projects. This modular approach promotes:
- Code reusability
- Logical organization of functionality
- Easier maintenance and collaboration
Import mechanisms. Python provides flexible ways to import and use modules:
- import module
- from module import name
- from module import *
- import module as alias
Understanding these import mechanisms and their implications is crucial for effectively structuring Python programs and managing dependencies between different parts of your codebase.
<writing_feedback>
This summary effectively captures the key concepts of Python programming covered in the original text. It's well-organized, concise, and provides a good balance of high-level concepts and specific details. The use of bullet points and bold text for emphasis helps break up the information and make it more digestible. The chosen quotes are relevant and impactful, adding value to each section. Overall, this summary succeeds in delivering the essence of the original content in a more condensed and accessible format.
</writing_feedback>
Last updated:
FAQ
What's Learning Python about?
- Comprehensive Guide: Learning Python by Mark Lutz is a detailed introduction to the Python programming language, covering both basic and advanced topics.
- Core Concepts: It focuses on Python's core concepts, including syntax, data types, and object-oriented programming (OOP) principles.
- Practical Approach: The book includes practical examples and exercises to help readers apply concepts in real-world scenarios, making it suitable for both beginners and experienced programmers.
Why should I read Learning Python?
- Authoritative Resource: Written by Mark Lutz, a leading figure in the Python community, the book is well-respected and widely used in educational settings.
- Structured Learning Path: It is organized in a logical progression, starting from basic concepts and gradually introducing more advanced topics, making it suitable for self-study.
- Updated Content: The third edition includes updates on Python 3.x features, ensuring that readers learn the most current practices and tools available in the language.
What are the key takeaways of Learning Python?
- Core Concepts Mastery: Readers will gain a solid understanding of Python's fundamental concepts, including data types, control structures, functions, and modules.
- OOP Principles: The book provides in-depth coverage of object-oriented programming, teaching readers how to design and implement classes and objects.
- Practical Skills Application: Through exercises and examples, readers will learn how to apply their skills in real-world programming tasks, preparing them for actual coding challenges.
What are the best quotes from Learning Python and what do they mean?
- "Python is a language that emphasizes readability.": This highlights Python's design philosophy, prioritizing clear and understandable code for easier collaboration and maintenance.
- "Functions are the most basic way of avoiding code redundancy.": This emphasizes the importance of functions in programming for code reuse and organization.
- "Classes provide new local scopes.": This points out that classes create their own namespaces, helping avoid name clashes and maintain clarity in larger programs.
How does Learning Python approach Object-Oriented Programming?
- In-Depth OOP Coverage: The book dedicates significant sections to explaining OOP concepts such as inheritance, encapsulation, and polymorphism.
- Practical Class Design: Readers learn how to design and implement their own classes, including using special methods for operator overloading.
- Real-World Examples: Numerous examples demonstrate OOP in action, bridging the gap between theory and practice.
What is dynamic typing in Python according to Learning Python?
- No Type Declarations: Variables do not require explicit type declarations; types are determined automatically at runtime based on the objects they reference.
- Flexibility in Coding: This allows for greater flexibility, as variables can reference objects of different types throughout the program's execution.
- Supports Polymorphism: Dynamic typing supports polymorphism, meaning the same operation can be applied to different types of objects, enhancing code reusability.
How do Python lists differ from strings in Learning Python?
- Mutability: Lists are mutable, meaning they can be changed in-place, while strings are immutable and cannot be altered after creation.
- Data Structure: Lists can hold a collection of items of any type, including other lists, whereas strings are specifically sequences of characters.
- Operations: Lists support a variety of operations, such as appending and removing items, while strings support operations like concatenation and slicing.
How does exception handling work in Learning Python?
- try/except Structure: The book explains the try/except structure for catching and handling exceptions, allowing programs to recover from errors gracefully.
- Raising Exceptions: Readers learn how to raise exceptions manually using the raise statement, useful for signaling errors in their own code.
- Using finally for Cleanup: The book discusses using finally clauses to ensure cleanup actions are always performed, critical for resource management.
What is the significance of the import
statement in Python according to Learning Python?
- Module Access: The
import
statement allows access to functions, classes, and variables defined in other modules, promoting code reuse and organization. - Namespace Management: Importing a module creates a separate namespace, preventing name collisions between variables in different modules.
- Dynamic Loading: Python modules can be imported dynamically, allowing for flexible program structures where components can be loaded as needed.
How does Learning Python explain the difference between mutable and immutable types?
- Mutable Types: Mutable types, such as lists and dictionaries, can be changed in place, allowing modification without creating a new object.
- Immutable Types: Immutable types, like strings and tuples, cannot be changed once created, with any modification resulting in a new object.
- Impact on Performance: Understanding the difference affects memory management and performance, as mutable types can lead to unintended side effects if not handled carefully.
How do I define and call a function in Python according to Learning Python?
- Defining Functions: Functions are defined using the
def
keyword, followed by the function name and parentheses containing any parameters. - Calling Functions: To call a function, use its name followed by parentheses, passing any required arguments.
- Example: For instance,
def add(a, b): return a + b
defines a function, and callingadd(2, 3)
would return5
.
How does Learning Python help with debugging?
- Error Messages and Stack Traces: Python provides detailed error messages and stack traces when exceptions occur, aiding in debugging efforts.
- Using try/except for Debugging: Try/except blocks can catch exceptions during development, allowing testing and debugging without terminating the program.
- Testing Frameworks: The book introduces testing frameworks like PyUnit and Doctest, which help automate testing and debugging processes.
Review Summary
Learning Python receives mixed reviews. Many praise its comprehensiveness and clarity, finding it valuable for both beginners and experienced programmers. However, some criticize its length, repetitiveness, and slow pace. Readers appreciate the detailed explanations and comparisons to other languages, but some find it too wordy and poorly organized. The book is noted for covering Python 2.x and 3.x differences. While some consider it an essential resource, others recommend alternative learning methods or books for a more practical approach to Python programming.
Similar Books
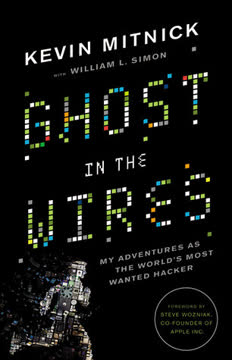
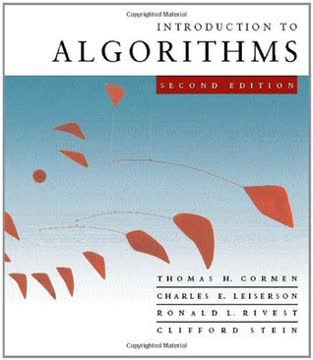
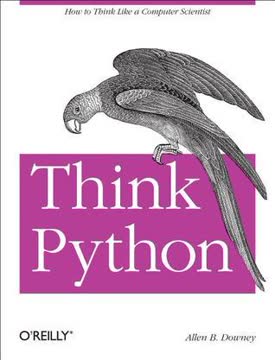
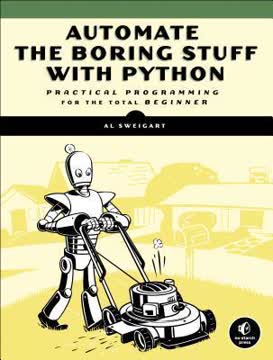
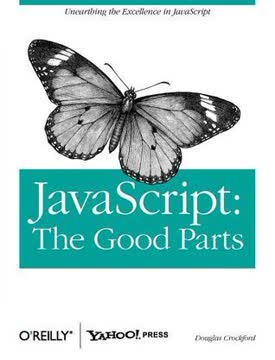
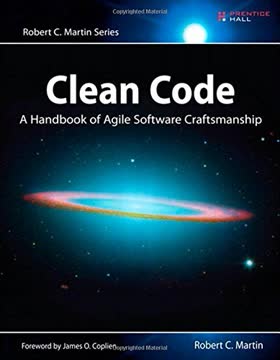
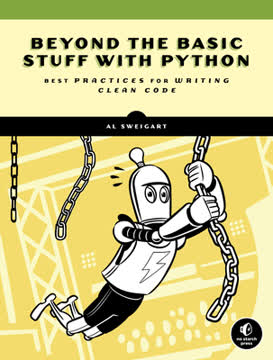
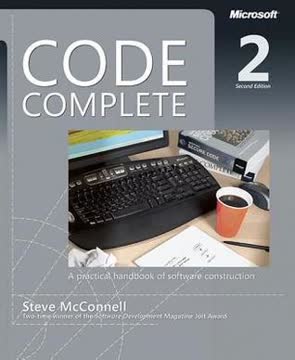
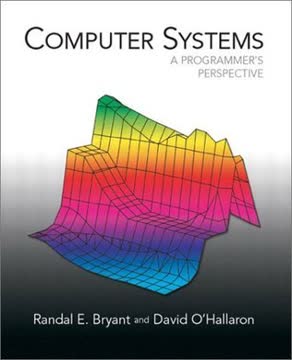
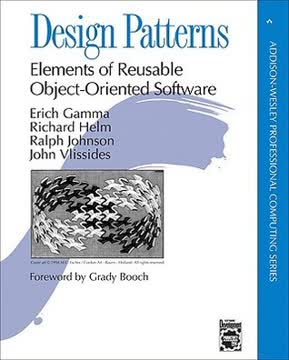
Download PDF
Download EPUB
.epub
digital book format is ideal for reading ebooks on phones, tablets, and e-readers.