Key Takeaways
1. Python's powerful string manipulation capabilities
"Python lets you overload operators by having your classes define special methods (i.e., methods whose names start and end with two underscores)."
Versatile string operations. Python offers a rich set of built-in string methods and functions that simplify text processing tasks. From basic operations like concatenation and slicing to more complex manipulations involving regular expressions, Python provides developers with powerful tools to handle strings efficiently.
Unicode support. Python's strong Unicode support enables seamless handling of international text and various character encodings. This feature is crucial for developing applications with global reach, ensuring proper display and processing of text in multiple languages.
String formatting options:
- f-strings (Python 3.6+)
- str.format() method
- %-formatting (older style)
These options provide flexibility in creating formatted strings for various purposes, from simple variable interpolation to complex alignment and padding operations.
2. Efficient file handling and directory operations
"Python separates the concepts of output (the print statement) and formatting (the % operator), but if you prefer to have these concepts together, they're easy to join, as this recipe shows."
Streamlined file I/O. Python's file handling capabilities are designed for simplicity and efficiency. The 'with' statement ensures proper file closure, while methods like read(), write(), and seek() provide fine-grained control over file operations.
Cross-platform compatibility. Python's os and os.path modules abstract away many platform-specific details, allowing developers to write code that works consistently across different operating systems. This feature is particularly valuable for tasks involving file and directory manipulation.
Key file and directory operations:
- Reading and writing files
- Searching for files and directories
- Modifying file attributes
- Compressing and archiving files
These operations, combined with Python's high-level abstractions, enable developers to create robust file management systems with minimal code.
3. Time and money: Precision in calculations and conversions
"Python 2.4 introduced support for decimal numbers (and you can retrofit that support into 2.3, see http://www.taniguetil.com.ar/facundo/bdvfiles/get decimal.html), making Python a good option even for computations where you must avoid using binary floats, as ones involving money so often are."
Decimal arithmetic for financial calculations. Python's decimal module provides precise decimal arithmetic, crucial for financial and monetary calculations where floating-point inaccuracies can lead to significant errors. This feature ensures that Python can be reliably used in financial applications and other domains requiring exact decimal representation.
Time manipulation and conversion. The datetime module offers a comprehensive set of tools for handling dates, times, and time zones. This functionality is essential for applications dealing with scheduling, time-based calculations, and data with temporal components.
Key features for time and money handling:
- Timezone-aware datetime objects
- Duration calculations with timedelta
- Currency formatting and conversion
- Precise arithmetic operations with Decimal objects
These features make Python a robust choice for developing applications in finance, scheduling, and other domains where precise time and monetary calculations are critical.
4. Python shortcuts for improved coding efficiency
"Once you get into the swing of Python, you'll find yourself constructing a lot of dictionaries."
Concise syntax for common operations. Python offers numerous syntactic shortcuts that allow developers to express complex operations succinctly. List comprehensions, generator expressions, and dictionary comprehensions are prime examples of how Python enables developers to write more readable and efficient code.
Built-in functions for common tasks. Python's standard library includes a wealth of built-in functions that simplify common programming tasks. Functions like map(), filter(), and zip() allow for functional-style programming, often reducing multi-line operations to a single, expressive line of code.
Efficiency-boosting Python features:
- Unpacking assignments
- Multiple assignment in a single line
- Conditional expressions (ternary operator)
- Slice notation for sequences
These features not only make code more concise but often lead to performance improvements by leveraging Python's optimized internals.
5. Mastering searching and sorting algorithms
"When you need to sort, find a way to use the built-in sort method of Python lists."
Optimized built-in sorting. Python's built-in sort() method and sorted() function use a highly optimized sorting algorithm (Timsort) that performs well across a wide range of input types and sizes. This implementation combines the best aspects of merge sort and insertion sort, providing excellent performance for both small and large datasets.
Customizable sorting behavior. The key parameter in sorting functions allows for flexible and efficient custom sorting based on specific attributes or computed values. This feature enables complex sorting operations without the need for verbose comparison functions.
Efficient searching techniques:
- Binary search with the bisect module
- Hash-based lookups with dictionaries
- Set operations for membership testing
These techniques, when applied appropriately, can significantly improve the performance of search operations in Python applications.
6. Object-oriented programming: Python's elegant approach
"To get the most out of Python's great OOP features, you should use them the Python way, rather than trying to mimic C++, Java, Smalltalk, or other languages you may be familiar with."
Simplicity in class definition. Python's class syntax is straightforward and intuitive, allowing developers to define classes with minimal boilerplate code. This simplicity encourages the use of OOP principles even in smaller scripts and applications.
Dynamic nature of Python classes. Python's dynamic typing extends to its OOP model, allowing for runtime modification of classes and objects. This flexibility enables powerful metaprogramming techniques and the implementation of design patterns that might be cumbersome in more static languages.
Key OOP features in Python:
- Multiple inheritance
- Properties and descriptors
- Method resolution order (MRO)
- Special methods for operator overloading
These features provide a rich toolset for designing complex systems while maintaining code readability and flexibility.
7. Advanced Python techniques for robust applications
"Metaclasses do not mean 'deep, dark black magic'."
Metaprogramming capabilities. Python's support for metaclasses and class decorators allows for powerful code generation and modification at runtime. These advanced features enable the creation of frameworks and libraries that can significantly reduce boilerplate code and enforce coding standards across large projects.
Context managers for resource management. The 'with' statement and context manager protocol provide a clean and pythonic way to manage resources, ensuring proper setup and teardown operations. This feature is particularly useful for file handling, database connections, and other scenarios requiring careful resource management.
Advanced Python techniques:
- Decorators for function and class modification
- Generators and coroutines for efficient iteration
- Abstract base classes for interface definition
- Type hinting for improved code clarity and tooling support
These advanced techniques, when used judiciously, can lead to more maintainable, efficient, and robust Python applications.
Last updated:
Review Summary
Python Cookbook receives mostly positive reviews, with readers praising its comprehensive coverage of Python 3 and practical recipes. Many find it useful for improving their coding skills and learning advanced techniques. The book is considered a valuable reference for experienced programmers, offering elegant solutions to common problems. Some criticisms include outdated content and the assumption of prior Python knowledge. Reviewers appreciate the book's structure, clear explanations, and focus on idiomatic Python. Overall, it's highly recommended for intermediate to advanced Python developers looking to enhance their skills.
Similar Books
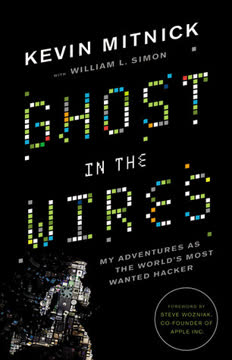
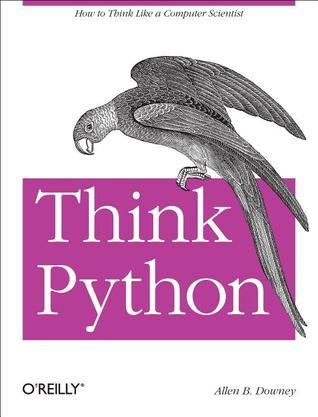
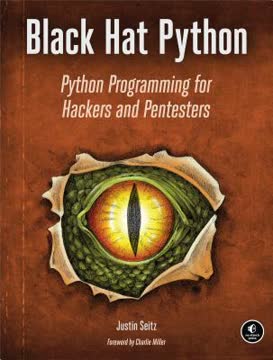
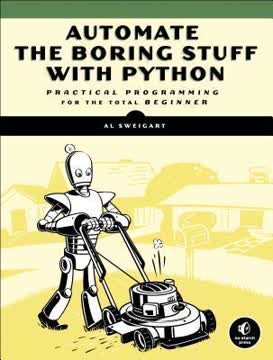
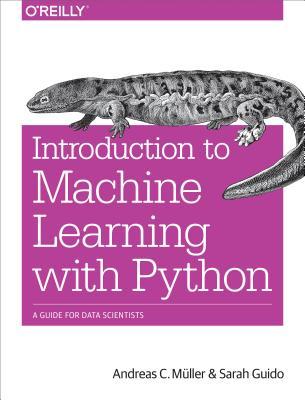
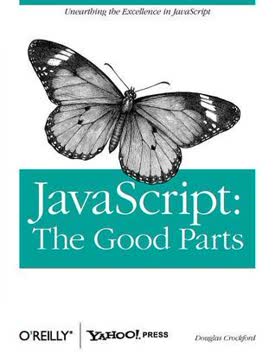
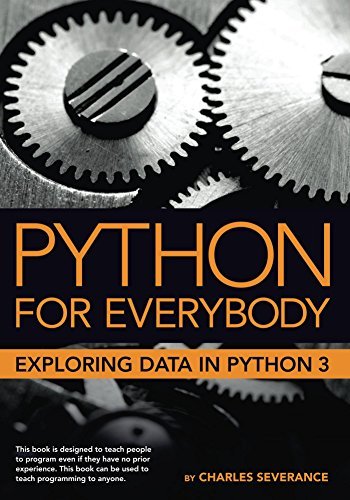
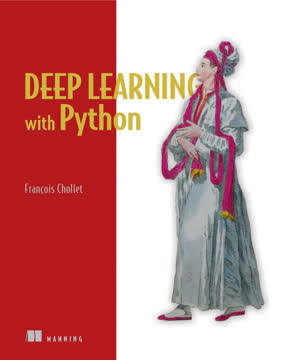
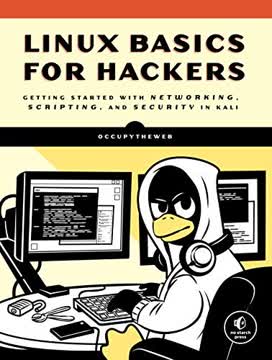
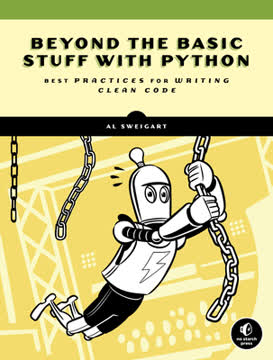
Download PDF
Download EPUB
.epub
digital book format is ideal for reading ebooks on phones, tablets, and e-readers.