Key Takeaways
1. Programs Mirror Human Problem-Solving
The single most important skill for a computer scientist is problem solving.
Computational Thinking. Programming is essentially a structured approach to problem-solving, mirroring how humans break down complex tasks into smaller, manageable steps. This involves formulating the problem, creatively devising solutions, and expressing those solutions clearly and accurately in a language the computer understands.
Basic Instructions. Every program, regardless of complexity, is built from a few fundamental instructions:
- Input: Gathering data.
- Output: Displaying results.
- Math: Performing calculations.
- Conditional execution: Making decisions.
- Repetition: Repeating actions.
Abstraction and Decomposition. Programming involves breaking down large, complex tasks into smaller, simpler subtasks until each subtask can be performed with one of the basic instructions. This process of abstraction and decomposition is key to managing complexity and creating effective programs.
2. Variables: Names Holding Values
A variable is a name that refers to a value.
Assignment and State. Variables are fundamental to programming, acting as named containers for values. Assignment statements create variables and assign them specific values, allowing programs to store and manipulate data. A state diagram visually represents variables and their corresponding values at a given point in time.
Naming Conventions. Choosing meaningful variable names is crucial for code readability and maintainability. Variable names should be descriptive, using lowercase letters and underscores to separate words (e.g., user_name
, total_count
). Avoid using keywords (e.g., class
, def
, while
) as variable names.
Expressions and Statements. Expressions combine values, variables, and operators to produce a result. Statements, on the other hand, are units of code that perform an action, such as creating a variable or displaying a value. Understanding the difference between expressions and statements is essential for writing correct and effective code.
3. Functions: Reusable Code Blocks
In the context of programming, a function is a named sequence of statements that performs a computation.
Definition and Calls. Functions are named blocks of code that perform specific tasks. Defining a function involves specifying its name, parameters (inputs), and the sequence of statements it executes. Calling a function executes those statements, potentially with different arguments each time.
Modularity and Reusability. Functions promote modularity by breaking down programs into smaller, self-contained units. This makes code easier to read, debug, and maintain. Functions also enable code reuse, as the same function can be called multiple times from different parts of the program.
Local Scope and Stack Diagrams. Variables defined inside a function are local, meaning they only exist within that function's scope. Parameters are also local variables. Stack diagrams are useful for visualizing the execution of functions, showing the function calls, local variables, and parameters at each step.
4. Interface Design: Functions Working Together
The interface of a function is a summary of how it is used: what are the parameters? What does the function do? And what is the return value?
Encapsulation and Generalization. Encapsulation involves wrapping a piece of code into a function, giving it a name and making it reusable. Generalization involves adding parameters to a function, making it more flexible and adaptable to different inputs.
Clean Interfaces. The interface of a function is a summary of how it is used, including its parameters, purpose, and return value. A clean interface allows users to interact with the function without needing to understand its internal workings.
Refactoring and Development Plans. Refactoring is the process of improving the structure and design of existing code without changing its functionality. A development plan provides a structured approach to writing programs, such as the "encapsulation and generalization" method, which involves starting with a small program, encapsulating it into a function, generalizing it with parameters, and repeating the process.
5. Conditionals & Recursion: Decision-Making
A boolean expression is an expression that is either true or false.
Conditional Execution. Conditional statements (if, elif, else) allow programs to execute different code blocks based on specific conditions. Boolean expressions, which evaluate to either True or False, are used to control the flow of execution.
Logical Operators. Logical operators (and, or, not) combine boolean expressions to create more complex conditions. These operators allow programs to make decisions based on multiple factors.
Recursion. Recursion is a powerful technique where a function calls itself within its own definition. Recursive functions must have a base case, which stops the recursion and prevents infinite loops. Stack diagrams are useful for visualizing the execution of recursive functions.
6. Fruitful Functions: Returning Results
When you call a fruitful function, you almost always want to do something with the result; for example, you might assign it to a variable or use it as part of an expression.
Return Values. Fruitful functions are functions that return a value, which can then be used in other parts of the program. The return
statement specifies the value that the function will return.
Incremental Development. Incremental development is a strategy for writing large functions by adding and testing small amounts of code at a time. This approach helps to avoid long debugging sessions and makes it easier to identify and fix errors.
Composition and Boolean Functions. Functions can be composed by calling one function from within another. Boolean functions return either True or False, and are often used to hide complex tests inside functions.
7. Iteration: Repeating Actions
Computers are often used to automate repetitive tasks.
Reassignment and Updating. Variables can be reassigned new values, and their values can be updated based on their previous values. This allows programs to track changing states and perform iterative calculations.
While Loops. The while
statement provides a way to repeat a block of code as long as a certain condition is true. The body of the loop should change the value of one or more variables so that the condition eventually becomes false and the loop terminates.
Break Statements and Algorithms. The break
statement allows you to exit a loop prematurely, based on a specific condition. Algorithms are step-by-step procedures for solving a category of problems, often involving iterative calculations.
8. Strings: Sequences of Characters
A string is a sequence of characters.
Indexing and Length. Strings are sequences of characters, and individual characters can be accessed using the bracket operator and an index. The len
function returns the number of characters in a string.
Traversal and Slices. Strings can be traversed using for
loops, allowing you to process each character individually. String slices allow you to extract portions of a string based on a range of indices.
Immutability and Methods. Strings are immutable, meaning their characters cannot be changed directly. However, string methods can be used to create new strings based on modifications of the original.
9. Word Play: Case Study
It is difficult to construct a solitary thought without using that most common symbol.
Reading and Processing. This case study focuses on solving word puzzles by searching for words with specific properties. It involves reading word lists from files, stripping whitespace and punctuation, and converting words to lowercase.
Search Functions. The exercises involve writing functions that search for words that meet certain criteria, such as containing no "e", avoiding certain letters, using only certain letters, or using all required letters.
Development Plan. The development plan involves reducing problems to previously solved problems, such as recognizing that uses_all
can be solved using uses_only
.
10. Lists: Mutable Sequences
A list is a sequence of values.
List Basics. Lists are versatile, mutable sequences that can hold elements of any type. They are created using square brackets and can be accessed using indices.
List Operations and Methods. Lists support various operations, including concatenation (+), repetition (*), and slicing. They also provide methods for adding elements (append
, extend
), removing elements (pop
, remove
, del
), and sorting (sort
).
Map, Filter, and Reduce. Common list operations can be expressed as combinations of map, filter, and reduce patterns. These patterns involve transforming elements, selecting elements based on a condition, and combining elements into a single value.
11. Dictionaries: Key-Value Mappings
A dictionary is like a list, but more general.
Dictionary Fundamentals. Dictionaries are data structures that map keys to values. Unlike lists, dictionaries are not ordered and are accessed using keys instead of indices.
Dictionary Operations. Dictionaries support operations like adding key-value pairs, accessing values using keys, checking for key existence, and iterating through keys. The get
method provides a way to access values with a default value if the key is not found.
Reverse Lookup and Memos. Reverse lookup involves finding the key associated with a given value, which requires searching the dictionary. Memos are previously computed values stored in a dictionary to avoid redundant calculations, improving efficiency.
12. Tuples: Immutable Sequences
A tuple is a sequence of values.
Tuple Basics. Tuples are immutable sequences, similar to lists but with the key difference that their elements cannot be modified after creation. They are defined using parentheses and commas.
Tuple Assignment. Tuple assignment allows you to assign values from a sequence to multiple variables simultaneously. This is a concise and elegant way to swap variable values or unpack elements from a list or tuple.
Variable-Length Arguments and Lists and Tuples. Functions can accept a variable number of arguments using the *args
syntax, which gathers the arguments into a tuple. The zip
function combines multiple sequences into a list of tuples, allowing you to iterate through them in parallel.
13. Data Structure Selection: Case Study
The practical goal of algorithm analysis is to predict the performance of different algorithms in order to guide design decisions.
Word Frequency Analysis. This case study involves analyzing word frequencies in a text file, demonstrating the use of dictionaries and lists to store and process data. It also introduces the concept of random numbers and their use in simulations.
Markov Analysis. Markov analysis is a technique for characterizing the probability of words following each other in a sequence. This involves building a dictionary that maps from prefixes to possible suffixes, allowing you to generate random text that mimics the style of the original text.
Data Structure Selection. The key takeaway is the importance of choosing appropriate data structures for specific tasks. Factors to consider include ease of implementation, runtime performance, and storage space.
14. Files: Persistent Storage
The goal of this book is to teach you to think like a computer scientist.
Reading and Writing Files. Files provide a way to store data persistently on a hard drive or other storage medium. Python provides functions for opening, reading, and writing text files.
Format Operator and Filenames and Paths. The format operator (%) allows you to create strings with formatted values. The os
module provides functions for working with filenames and paths, including finding the current directory, checking for file existence, and listing directory contents.
Catching Exceptions and Databases. The try
statement allows you to catch exceptions that might occur during file operations, preventing the program from crashing. The dbm
module provides an interface for creating and updating database files, which store data in a key-value format.
Last updated:
Review Summary
Think Python receives mostly positive reviews, praised for its clarity, conciseness, and beginner-friendly approach. Readers appreciate the practical exercises, free availability, and focus on computer science concepts. Some find it challenging for absolute beginners, noting math-heavy examples and exercises. The book is commended for its structured approach, covering Python basics and programming fundamentals. While a few reviewers found it frustrating or overly technical, many recommend it as an excellent introduction to Python and computer programming, suitable for both novices and those with prior experience.
Similar Books
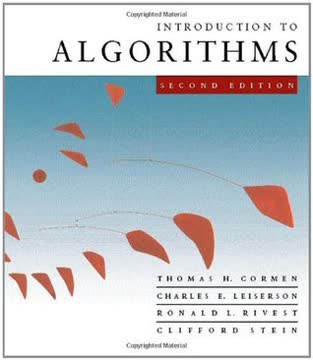
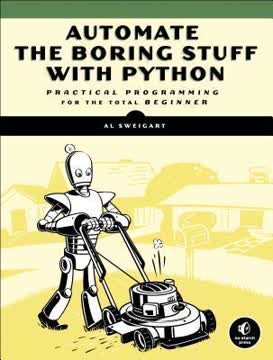
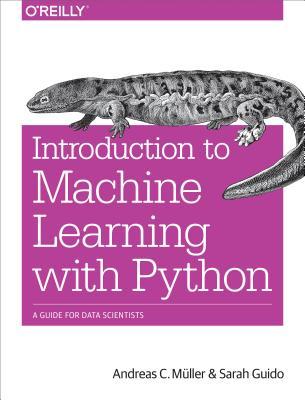
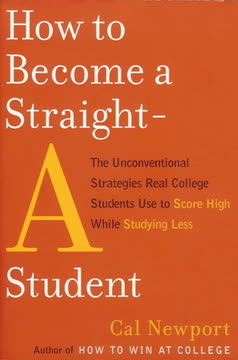
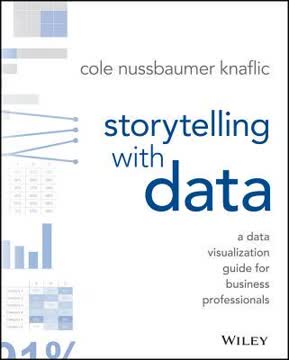
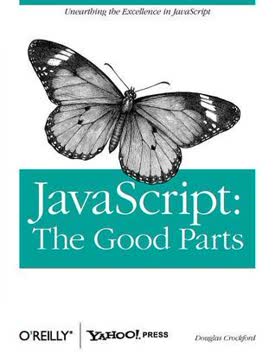
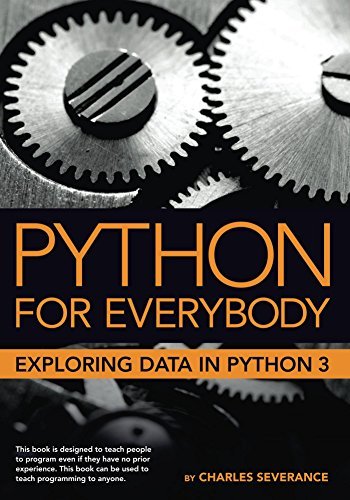
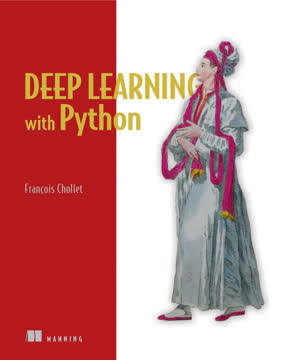
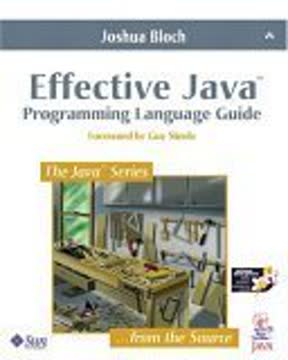
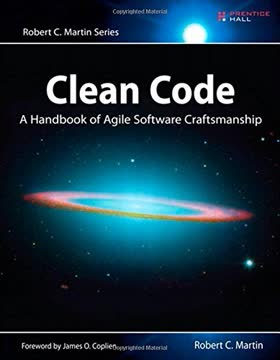